Map iteration not working in JSP with RESTful method returning type Viewable
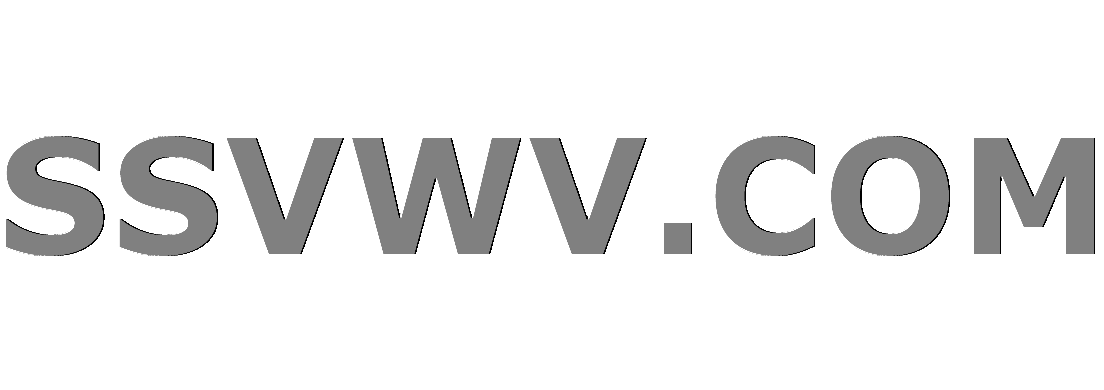
Multi tool use
I'm certain this is a really messy way of doing things but:
I have a Map of Maps to try and return data in the form of a Viewable to be used in the context of a user list front-end using JSP.
However, the iteration through said Map of Maps in my JSP file isn't happening and so nothing gets put out.
Here's My Code
JSP
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%--
Document : all-users
Created on : Nov 22, 2018, 5:13:55 AM
Author : cubsy
--%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<style><%@include file="css/users-page.css"%></style>
<title>Users</title>
</head>
<body>
<!-- screen top -->
<div class="header">
<h1>Cubapp</h1>
<p>A new twitter style forum service</p>
</div>
<div class="navbar">
<a href="#">My Account</a>
<a href="#">All Accounts</a>
<a href="#">Messages</a>
<a href="#" class="right">Sign Out</a>
</div>
<!-- screen top -->
<div class="row">
<div class="side"></div>
<div class="main">
<!-- This is where the user boxes will go inside the "main" flex field -->
<c:forEach items="${map}" var="user">
<p>Key: ${user.key}</p>
<div class="card">
<div class="userProfile">
<p>User</p>
</div>
<div class="container">
<h4><b>${user.username}</b></h4>
<p>${user.firstname} ${user.lastname}</p>
</div>
</div>
</c:forEach>
</div>
</div>
</body>
</html>
UserResource Class(Handles network calls)
package fish.payara.cubappmessenger.resource;
import fish.payara.cubappmessenger.base.User;
import fish.payara.cubappmessenger.service.UserService;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.ws.rs.Consumes;
import javax.ws.rs.FormParam;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status;
import javax.ws.rs.core.UriBuilder;
import javax.ws.rs.core.UriInfo;
import org.glassfish.jersey.server.mvc.Viewable;
@Path("users")
public class UserResource {
UserService uService = new UserService();
List<String> quotes = new ArrayList<>();
@GET
@Path("/signup")
public Viewable loginPage() {
Map<String, String> quote = new HashMap<>();
String q = "Welcome Home Lads!";
quote.put("quote", q);
return new Viewable("/signup", quote);
}
@GET
@Path("/{userId}")
public Viewable getUserById(@PathParam("userId") String id) {
Map<String, String> userInfo = new HashMap<>();
userInfo.put("username", uService.getUserById(id).getUsername());
userInfo.put("firstname", uService.getUserById(id).getFirstName());
userInfo.put("lastname", uService.getUserById(id).getLastName());
return new Viewable("/test", userInfo);
}
@GET
public Viewable getUsers() {
//List<Map<String, String>> u = new ArrayList<>();
Map<String, Map<String, String>> map = new HashMap<>();
for (int i=0; i<uService.getAllUsers().size(); i++) {
Map<String, String> newUser = new HashMap<>();
User user = uService.getAllUsers().get(i);
newUser.put("username", user.getUsername());
newUser.put("firstname", user.getFirstName());
newUser.put("lastname", user.getLastName());
map.put(user.getUsername(), newUser);
}
return new Viewable("/all-users", map);
}
@POST
@Path("/newuser")
public Response addNewUser(@FormParam("username") String username,
@FormParam("firstname") String fname,
@FormParam("lastname") String sname,
@Context UriInfo uriInfo) {
UriBuilder builder = uriInfo.getBaseUriBuilder();
builder.path("/users/"+username);
User u = new User(username, fname, sname);
uService.addUser(u);
return Response.seeOther(builder.build()).build();
}
}
UserService Class(Handles connection to the Database - connection does work as tests with other elements interacting with the database have been and still are successful)
package fish.payara.cubappmessenger.service;
import fish.payara.cubappmessenger.base.Message;
import fish.payara.cubappmessenger.base.User;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class UserService {
Connection con = null;
public UserService() {
String url, username, password;
url = "jdbc:mysql://localhost:3306/Cubapp";
username = "root";
password = "Huskyanna234";
try {
Class.forName("com.mysql.jdbc.Driver");
con = DriverManager.getConnection(url, username, password);
} catch (Exception e) {
System.out.println(e);
}
}
//===============User Handling===============
public List<User> getAllUsers() {
List<User> users = new ArrayList<>();
String sql = "select * from Users";
try {
//Statement statement = con.createStatement();
Statement statement = con.createStatement();
ResultSet rs = statement.executeQuery(sql);
while(rs.next()) {
User u = new User();
u.setUsername(rs.getString("username"));
u.setFirstName(rs.getString("firstName"));
u.setLastName(rs.getString("lastName"));
users.add(u);
}
} catch (Exception e) {
System.out.println(e);
}
return users;
}
public User getUserById(String id) {
User u = new User();
String sql = "select * from Users where username='"+id+"'";
try {
// Statement statement = con.createStatement();
Statement statement = con.createStatement();
ResultSet rs = statement.executeQuery(sql);
if(rs.next()) {
u.setUsername(rs.getString("username"));
u.setFirstName(rs.getString("firstName"));
u.setLastName(rs.getString("lastName"));
}
} catch (Exception e) {
System.out.println(e);
}
return u;
}
public User addUser(User user) {
String sql = "insert into Users values (?,?,?)";
try {
//PreparedStatement statement = con.prepareStatement(sql);
PreparedStatement statement = con.prepareStatement(sql);
statement.setString(1, user.getUsername());
statement.setString(2, user.getFirstName());
statement.setString(3, user.getLastName());
statement.executeUpdate();
} catch (Exception e) {
System.out.println(e);
}
return user;
}
//=================User Handling=================
}
I imagine I've just made a stupid syntax error or something, I'm new to Java based web development but any help will be appreciated.
java rest jsp jax-rs java-ee-7
add a comment |
I'm certain this is a really messy way of doing things but:
I have a Map of Maps to try and return data in the form of a Viewable to be used in the context of a user list front-end using JSP.
However, the iteration through said Map of Maps in my JSP file isn't happening and so nothing gets put out.
Here's My Code
JSP
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%--
Document : all-users
Created on : Nov 22, 2018, 5:13:55 AM
Author : cubsy
--%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<style><%@include file="css/users-page.css"%></style>
<title>Users</title>
</head>
<body>
<!-- screen top -->
<div class="header">
<h1>Cubapp</h1>
<p>A new twitter style forum service</p>
</div>
<div class="navbar">
<a href="#">My Account</a>
<a href="#">All Accounts</a>
<a href="#">Messages</a>
<a href="#" class="right">Sign Out</a>
</div>
<!-- screen top -->
<div class="row">
<div class="side"></div>
<div class="main">
<!-- This is where the user boxes will go inside the "main" flex field -->
<c:forEach items="${map}" var="user">
<p>Key: ${user.key}</p>
<div class="card">
<div class="userProfile">
<p>User</p>
</div>
<div class="container">
<h4><b>${user.username}</b></h4>
<p>${user.firstname} ${user.lastname}</p>
</div>
</div>
</c:forEach>
</div>
</div>
</body>
</html>
UserResource Class(Handles network calls)
package fish.payara.cubappmessenger.resource;
import fish.payara.cubappmessenger.base.User;
import fish.payara.cubappmessenger.service.UserService;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.ws.rs.Consumes;
import javax.ws.rs.FormParam;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status;
import javax.ws.rs.core.UriBuilder;
import javax.ws.rs.core.UriInfo;
import org.glassfish.jersey.server.mvc.Viewable;
@Path("users")
public class UserResource {
UserService uService = new UserService();
List<String> quotes = new ArrayList<>();
@GET
@Path("/signup")
public Viewable loginPage() {
Map<String, String> quote = new HashMap<>();
String q = "Welcome Home Lads!";
quote.put("quote", q);
return new Viewable("/signup", quote);
}
@GET
@Path("/{userId}")
public Viewable getUserById(@PathParam("userId") String id) {
Map<String, String> userInfo = new HashMap<>();
userInfo.put("username", uService.getUserById(id).getUsername());
userInfo.put("firstname", uService.getUserById(id).getFirstName());
userInfo.put("lastname", uService.getUserById(id).getLastName());
return new Viewable("/test", userInfo);
}
@GET
public Viewable getUsers() {
//List<Map<String, String>> u = new ArrayList<>();
Map<String, Map<String, String>> map = new HashMap<>();
for (int i=0; i<uService.getAllUsers().size(); i++) {
Map<String, String> newUser = new HashMap<>();
User user = uService.getAllUsers().get(i);
newUser.put("username", user.getUsername());
newUser.put("firstname", user.getFirstName());
newUser.put("lastname", user.getLastName());
map.put(user.getUsername(), newUser);
}
return new Viewable("/all-users", map);
}
@POST
@Path("/newuser")
public Response addNewUser(@FormParam("username") String username,
@FormParam("firstname") String fname,
@FormParam("lastname") String sname,
@Context UriInfo uriInfo) {
UriBuilder builder = uriInfo.getBaseUriBuilder();
builder.path("/users/"+username);
User u = new User(username, fname, sname);
uService.addUser(u);
return Response.seeOther(builder.build()).build();
}
}
UserService Class(Handles connection to the Database - connection does work as tests with other elements interacting with the database have been and still are successful)
package fish.payara.cubappmessenger.service;
import fish.payara.cubappmessenger.base.Message;
import fish.payara.cubappmessenger.base.User;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class UserService {
Connection con = null;
public UserService() {
String url, username, password;
url = "jdbc:mysql://localhost:3306/Cubapp";
username = "root";
password = "Huskyanna234";
try {
Class.forName("com.mysql.jdbc.Driver");
con = DriverManager.getConnection(url, username, password);
} catch (Exception e) {
System.out.println(e);
}
}
//===============User Handling===============
public List<User> getAllUsers() {
List<User> users = new ArrayList<>();
String sql = "select * from Users";
try {
//Statement statement = con.createStatement();
Statement statement = con.createStatement();
ResultSet rs = statement.executeQuery(sql);
while(rs.next()) {
User u = new User();
u.setUsername(rs.getString("username"));
u.setFirstName(rs.getString("firstName"));
u.setLastName(rs.getString("lastName"));
users.add(u);
}
} catch (Exception e) {
System.out.println(e);
}
return users;
}
public User getUserById(String id) {
User u = new User();
String sql = "select * from Users where username='"+id+"'";
try {
// Statement statement = con.createStatement();
Statement statement = con.createStatement();
ResultSet rs = statement.executeQuery(sql);
if(rs.next()) {
u.setUsername(rs.getString("username"));
u.setFirstName(rs.getString("firstName"));
u.setLastName(rs.getString("lastName"));
}
} catch (Exception e) {
System.out.println(e);
}
return u;
}
public User addUser(User user) {
String sql = "insert into Users values (?,?,?)";
try {
//PreparedStatement statement = con.prepareStatement(sql);
PreparedStatement statement = con.prepareStatement(sql);
statement.setString(1, user.getUsername());
statement.setString(2, user.getFirstName());
statement.setString(3, user.getLastName());
statement.executeUpdate();
} catch (Exception e) {
System.out.println(e);
}
return user;
}
//=================User Handling=================
}
I imagine I've just made a stupid syntax error or something, I'm new to Java based web development but any help will be appreciated.
java rest jsp jax-rs java-ee-7
nothing gets put out.: I'm sure something gets puts out. What is, precisely? Anyway, I would restart all of this from scratch. Java is an OO, type-safe language. Define a User class, with getters. If you want to display a user, then store a User object in a request attribute. If you want to display several users, then store a List<User> in a request attribute.
– JB Nizet
Nov 23 '18 at 11:50
My assumption is that the jsp forEach is taking the count to be 0 and thus never executing the code within that forEach tag - I have a User class with getters and setters which are used and accessed in the UserService class and work correctly as they should. My issue is getting the jsp front end to render the data from my restful methods on request and not just to output raw JSON or XML data in my browser window
– Cuba Stanley
Nov 23 '18 at 12:06
The JSP EL has been designed to read data from Java beans. I don't understand why you think you need to use maps. Anyway, don't use assumptions. Inspect the HTML code that is actually been generated. Use your debugger. Add traces to the code. Display the complete map.
– JB Nizet
Nov 23 '18 at 12:10
add a comment |
I'm certain this is a really messy way of doing things but:
I have a Map of Maps to try and return data in the form of a Viewable to be used in the context of a user list front-end using JSP.
However, the iteration through said Map of Maps in my JSP file isn't happening and so nothing gets put out.
Here's My Code
JSP
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%--
Document : all-users
Created on : Nov 22, 2018, 5:13:55 AM
Author : cubsy
--%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<style><%@include file="css/users-page.css"%></style>
<title>Users</title>
</head>
<body>
<!-- screen top -->
<div class="header">
<h1>Cubapp</h1>
<p>A new twitter style forum service</p>
</div>
<div class="navbar">
<a href="#">My Account</a>
<a href="#">All Accounts</a>
<a href="#">Messages</a>
<a href="#" class="right">Sign Out</a>
</div>
<!-- screen top -->
<div class="row">
<div class="side"></div>
<div class="main">
<!-- This is where the user boxes will go inside the "main" flex field -->
<c:forEach items="${map}" var="user">
<p>Key: ${user.key}</p>
<div class="card">
<div class="userProfile">
<p>User</p>
</div>
<div class="container">
<h4><b>${user.username}</b></h4>
<p>${user.firstname} ${user.lastname}</p>
</div>
</div>
</c:forEach>
</div>
</div>
</body>
</html>
UserResource Class(Handles network calls)
package fish.payara.cubappmessenger.resource;
import fish.payara.cubappmessenger.base.User;
import fish.payara.cubappmessenger.service.UserService;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.ws.rs.Consumes;
import javax.ws.rs.FormParam;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status;
import javax.ws.rs.core.UriBuilder;
import javax.ws.rs.core.UriInfo;
import org.glassfish.jersey.server.mvc.Viewable;
@Path("users")
public class UserResource {
UserService uService = new UserService();
List<String> quotes = new ArrayList<>();
@GET
@Path("/signup")
public Viewable loginPage() {
Map<String, String> quote = new HashMap<>();
String q = "Welcome Home Lads!";
quote.put("quote", q);
return new Viewable("/signup", quote);
}
@GET
@Path("/{userId}")
public Viewable getUserById(@PathParam("userId") String id) {
Map<String, String> userInfo = new HashMap<>();
userInfo.put("username", uService.getUserById(id).getUsername());
userInfo.put("firstname", uService.getUserById(id).getFirstName());
userInfo.put("lastname", uService.getUserById(id).getLastName());
return new Viewable("/test", userInfo);
}
@GET
public Viewable getUsers() {
//List<Map<String, String>> u = new ArrayList<>();
Map<String, Map<String, String>> map = new HashMap<>();
for (int i=0; i<uService.getAllUsers().size(); i++) {
Map<String, String> newUser = new HashMap<>();
User user = uService.getAllUsers().get(i);
newUser.put("username", user.getUsername());
newUser.put("firstname", user.getFirstName());
newUser.put("lastname", user.getLastName());
map.put(user.getUsername(), newUser);
}
return new Viewable("/all-users", map);
}
@POST
@Path("/newuser")
public Response addNewUser(@FormParam("username") String username,
@FormParam("firstname") String fname,
@FormParam("lastname") String sname,
@Context UriInfo uriInfo) {
UriBuilder builder = uriInfo.getBaseUriBuilder();
builder.path("/users/"+username);
User u = new User(username, fname, sname);
uService.addUser(u);
return Response.seeOther(builder.build()).build();
}
}
UserService Class(Handles connection to the Database - connection does work as tests with other elements interacting with the database have been and still are successful)
package fish.payara.cubappmessenger.service;
import fish.payara.cubappmessenger.base.Message;
import fish.payara.cubappmessenger.base.User;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class UserService {
Connection con = null;
public UserService() {
String url, username, password;
url = "jdbc:mysql://localhost:3306/Cubapp";
username = "root";
password = "Huskyanna234";
try {
Class.forName("com.mysql.jdbc.Driver");
con = DriverManager.getConnection(url, username, password);
} catch (Exception e) {
System.out.println(e);
}
}
//===============User Handling===============
public List<User> getAllUsers() {
List<User> users = new ArrayList<>();
String sql = "select * from Users";
try {
//Statement statement = con.createStatement();
Statement statement = con.createStatement();
ResultSet rs = statement.executeQuery(sql);
while(rs.next()) {
User u = new User();
u.setUsername(rs.getString("username"));
u.setFirstName(rs.getString("firstName"));
u.setLastName(rs.getString("lastName"));
users.add(u);
}
} catch (Exception e) {
System.out.println(e);
}
return users;
}
public User getUserById(String id) {
User u = new User();
String sql = "select * from Users where username='"+id+"'";
try {
// Statement statement = con.createStatement();
Statement statement = con.createStatement();
ResultSet rs = statement.executeQuery(sql);
if(rs.next()) {
u.setUsername(rs.getString("username"));
u.setFirstName(rs.getString("firstName"));
u.setLastName(rs.getString("lastName"));
}
} catch (Exception e) {
System.out.println(e);
}
return u;
}
public User addUser(User user) {
String sql = "insert into Users values (?,?,?)";
try {
//PreparedStatement statement = con.prepareStatement(sql);
PreparedStatement statement = con.prepareStatement(sql);
statement.setString(1, user.getUsername());
statement.setString(2, user.getFirstName());
statement.setString(3, user.getLastName());
statement.executeUpdate();
} catch (Exception e) {
System.out.println(e);
}
return user;
}
//=================User Handling=================
}
I imagine I've just made a stupid syntax error or something, I'm new to Java based web development but any help will be appreciated.
java rest jsp jax-rs java-ee-7
I'm certain this is a really messy way of doing things but:
I have a Map of Maps to try and return data in the form of a Viewable to be used in the context of a user list front-end using JSP.
However, the iteration through said Map of Maps in my JSP file isn't happening and so nothing gets put out.
Here's My Code
JSP
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%--
Document : all-users
Created on : Nov 22, 2018, 5:13:55 AM
Author : cubsy
--%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<style><%@include file="css/users-page.css"%></style>
<title>Users</title>
</head>
<body>
<!-- screen top -->
<div class="header">
<h1>Cubapp</h1>
<p>A new twitter style forum service</p>
</div>
<div class="navbar">
<a href="#">My Account</a>
<a href="#">All Accounts</a>
<a href="#">Messages</a>
<a href="#" class="right">Sign Out</a>
</div>
<!-- screen top -->
<div class="row">
<div class="side"></div>
<div class="main">
<!-- This is where the user boxes will go inside the "main" flex field -->
<c:forEach items="${map}" var="user">
<p>Key: ${user.key}</p>
<div class="card">
<div class="userProfile">
<p>User</p>
</div>
<div class="container">
<h4><b>${user.username}</b></h4>
<p>${user.firstname} ${user.lastname}</p>
</div>
</div>
</c:forEach>
</div>
</div>
</body>
</html>
UserResource Class(Handles network calls)
package fish.payara.cubappmessenger.resource;
import fish.payara.cubappmessenger.base.User;
import fish.payara.cubappmessenger.service.UserService;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.ws.rs.Consumes;
import javax.ws.rs.FormParam;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status;
import javax.ws.rs.core.UriBuilder;
import javax.ws.rs.core.UriInfo;
import org.glassfish.jersey.server.mvc.Viewable;
@Path("users")
public class UserResource {
UserService uService = new UserService();
List<String> quotes = new ArrayList<>();
@GET
@Path("/signup")
public Viewable loginPage() {
Map<String, String> quote = new HashMap<>();
String q = "Welcome Home Lads!";
quote.put("quote", q);
return new Viewable("/signup", quote);
}
@GET
@Path("/{userId}")
public Viewable getUserById(@PathParam("userId") String id) {
Map<String, String> userInfo = new HashMap<>();
userInfo.put("username", uService.getUserById(id).getUsername());
userInfo.put("firstname", uService.getUserById(id).getFirstName());
userInfo.put("lastname", uService.getUserById(id).getLastName());
return new Viewable("/test", userInfo);
}
@GET
public Viewable getUsers() {
//List<Map<String, String>> u = new ArrayList<>();
Map<String, Map<String, String>> map = new HashMap<>();
for (int i=0; i<uService.getAllUsers().size(); i++) {
Map<String, String> newUser = new HashMap<>();
User user = uService.getAllUsers().get(i);
newUser.put("username", user.getUsername());
newUser.put("firstname", user.getFirstName());
newUser.put("lastname", user.getLastName());
map.put(user.getUsername(), newUser);
}
return new Viewable("/all-users", map);
}
@POST
@Path("/newuser")
public Response addNewUser(@FormParam("username") String username,
@FormParam("firstname") String fname,
@FormParam("lastname") String sname,
@Context UriInfo uriInfo) {
UriBuilder builder = uriInfo.getBaseUriBuilder();
builder.path("/users/"+username);
User u = new User(username, fname, sname);
uService.addUser(u);
return Response.seeOther(builder.build()).build();
}
}
UserService Class(Handles connection to the Database - connection does work as tests with other elements interacting with the database have been and still are successful)
package fish.payara.cubappmessenger.service;
import fish.payara.cubappmessenger.base.Message;
import fish.payara.cubappmessenger.base.User;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class UserService {
Connection con = null;
public UserService() {
String url, username, password;
url = "jdbc:mysql://localhost:3306/Cubapp";
username = "root";
password = "Huskyanna234";
try {
Class.forName("com.mysql.jdbc.Driver");
con = DriverManager.getConnection(url, username, password);
} catch (Exception e) {
System.out.println(e);
}
}
//===============User Handling===============
public List<User> getAllUsers() {
List<User> users = new ArrayList<>();
String sql = "select * from Users";
try {
//Statement statement = con.createStatement();
Statement statement = con.createStatement();
ResultSet rs = statement.executeQuery(sql);
while(rs.next()) {
User u = new User();
u.setUsername(rs.getString("username"));
u.setFirstName(rs.getString("firstName"));
u.setLastName(rs.getString("lastName"));
users.add(u);
}
} catch (Exception e) {
System.out.println(e);
}
return users;
}
public User getUserById(String id) {
User u = new User();
String sql = "select * from Users where username='"+id+"'";
try {
// Statement statement = con.createStatement();
Statement statement = con.createStatement();
ResultSet rs = statement.executeQuery(sql);
if(rs.next()) {
u.setUsername(rs.getString("username"));
u.setFirstName(rs.getString("firstName"));
u.setLastName(rs.getString("lastName"));
}
} catch (Exception e) {
System.out.println(e);
}
return u;
}
public User addUser(User user) {
String sql = "insert into Users values (?,?,?)";
try {
//PreparedStatement statement = con.prepareStatement(sql);
PreparedStatement statement = con.prepareStatement(sql);
statement.setString(1, user.getUsername());
statement.setString(2, user.getFirstName());
statement.setString(3, user.getLastName());
statement.executeUpdate();
} catch (Exception e) {
System.out.println(e);
}
return user;
}
//=================User Handling=================
}
I imagine I've just made a stupid syntax error or something, I'm new to Java based web development but any help will be appreciated.
java rest jsp jax-rs java-ee-7
java rest jsp jax-rs java-ee-7
asked Nov 23 '18 at 11:42


Cuba StanleyCuba Stanley
11
11
nothing gets put out.: I'm sure something gets puts out. What is, precisely? Anyway, I would restart all of this from scratch. Java is an OO, type-safe language. Define a User class, with getters. If you want to display a user, then store a User object in a request attribute. If you want to display several users, then store a List<User> in a request attribute.
– JB Nizet
Nov 23 '18 at 11:50
My assumption is that the jsp forEach is taking the count to be 0 and thus never executing the code within that forEach tag - I have a User class with getters and setters which are used and accessed in the UserService class and work correctly as they should. My issue is getting the jsp front end to render the data from my restful methods on request and not just to output raw JSON or XML data in my browser window
– Cuba Stanley
Nov 23 '18 at 12:06
The JSP EL has been designed to read data from Java beans. I don't understand why you think you need to use maps. Anyway, don't use assumptions. Inspect the HTML code that is actually been generated. Use your debugger. Add traces to the code. Display the complete map.
– JB Nizet
Nov 23 '18 at 12:10
add a comment |
nothing gets put out.: I'm sure something gets puts out. What is, precisely? Anyway, I would restart all of this from scratch. Java is an OO, type-safe language. Define a User class, with getters. If you want to display a user, then store a User object in a request attribute. If you want to display several users, then store a List<User> in a request attribute.
– JB Nizet
Nov 23 '18 at 11:50
My assumption is that the jsp forEach is taking the count to be 0 and thus never executing the code within that forEach tag - I have a User class with getters and setters which are used and accessed in the UserService class and work correctly as they should. My issue is getting the jsp front end to render the data from my restful methods on request and not just to output raw JSON or XML data in my browser window
– Cuba Stanley
Nov 23 '18 at 12:06
The JSP EL has been designed to read data from Java beans. I don't understand why you think you need to use maps. Anyway, don't use assumptions. Inspect the HTML code that is actually been generated. Use your debugger. Add traces to the code. Display the complete map.
– JB Nizet
Nov 23 '18 at 12:10
nothing gets put out.: I'm sure something gets puts out. What is, precisely? Anyway, I would restart all of this from scratch. Java is an OO, type-safe language. Define a User class, with getters. If you want to display a user, then store a User object in a request attribute. If you want to display several users, then store a List<User> in a request attribute.
– JB Nizet
Nov 23 '18 at 11:50
nothing gets put out.: I'm sure something gets puts out. What is, precisely? Anyway, I would restart all of this from scratch. Java is an OO, type-safe language. Define a User class, with getters. If you want to display a user, then store a User object in a request attribute. If you want to display several users, then store a List<User> in a request attribute.
– JB Nizet
Nov 23 '18 at 11:50
My assumption is that the jsp forEach is taking the count to be 0 and thus never executing the code within that forEach tag - I have a User class with getters and setters which are used and accessed in the UserService class and work correctly as they should. My issue is getting the jsp front end to render the data from my restful methods on request and not just to output raw JSON or XML data in my browser window
– Cuba Stanley
Nov 23 '18 at 12:06
My assumption is that the jsp forEach is taking the count to be 0 and thus never executing the code within that forEach tag - I have a User class with getters and setters which are used and accessed in the UserService class and work correctly as they should. My issue is getting the jsp front end to render the data from my restful methods on request and not just to output raw JSON or XML data in my browser window
– Cuba Stanley
Nov 23 '18 at 12:06
The JSP EL has been designed to read data from Java beans. I don't understand why you think you need to use maps. Anyway, don't use assumptions. Inspect the HTML code that is actually been generated. Use your debugger. Add traces to the code. Display the complete map.
– JB Nizet
Nov 23 '18 at 12:10
The JSP EL has been designed to read data from Java beans. I don't understand why you think you need to use maps. Anyway, don't use assumptions. Inspect the HTML code that is actually been generated. Use your debugger. Add traces to the code. Display the complete map.
– JB Nizet
Nov 23 '18 at 12:10
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446057%2fmap-iteration-not-working-in-jsp-with-restful-method-returning-type-viewable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446057%2fmap-iteration-not-working-in-jsp-with-restful-method-returning-type-viewable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VgBCG5Zpojlnk,vhM HImzrScv1zAAMGAwr8zQOLGfhkmAk,oplT0aSE99ciRjjJ dXS1CWfsfxXUSDTxybBAOm0CF
nothing gets put out.: I'm sure something gets puts out. What is, precisely? Anyway, I would restart all of this from scratch. Java is an OO, type-safe language. Define a User class, with getters. If you want to display a user, then store a User object in a request attribute. If you want to display several users, then store a List<User> in a request attribute.
– JB Nizet
Nov 23 '18 at 11:50
My assumption is that the jsp forEach is taking the count to be 0 and thus never executing the code within that forEach tag - I have a User class with getters and setters which are used and accessed in the UserService class and work correctly as they should. My issue is getting the jsp front end to render the data from my restful methods on request and not just to output raw JSON or XML data in my browser window
– Cuba Stanley
Nov 23 '18 at 12:06
The JSP EL has been designed to read data from Java beans. I don't understand why you think you need to use maps. Anyway, don't use assumptions. Inspect the HTML code that is actually been generated. Use your debugger. Add traces to the code. Display the complete map.
– JB Nizet
Nov 23 '18 at 12:10