Components not appearing in ContentPane
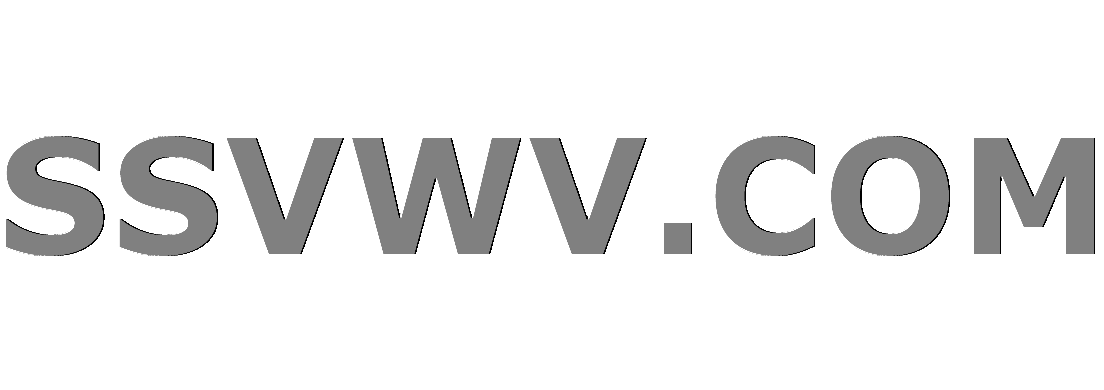
Multi tool use
In my application I have a list of 6 Jlabels
, which are added to the contentPane
in a for
loop. After that I add 2 JButtons
- one for removing all the labels and the second one for adding them again:
public class Test {
private JFrame frame;
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Test window = new Test();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public Test() {
initialize();
}
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 960, 620);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.setLocationRelativeTo(null);
frame.getContentPane().setLayout(null);
frame.getContentPane().setBackground(new Color(30, 30, 30));
LinkedList<JLabel> labels = new LinkedList<>();
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setSize(280, 50);
labels.setBackground(new Color(75, 75, 75));
labels.setOpaque(true);
}
Button buttonAdd = new JButton("Add");
buttonAdd.setBounds(310, 15, 150, 50);
buttonAdd.addMouseListener(new MouseAdapter() {
@Override
public final void mouseClicked(MouseEvent event) {
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setLocation(15, 15+50*i);
frame.getContentPane().add(labels.get(i));
}
}
});
Button buttonRemove = new JButton("Remove");
buttonRemove.setBounds(310, 15, 150, 50);
buttonRemove.addMouseListener(new MouseAdapter() {
@Override
public final void mouseClicked(MouseEvent event) {
for(int i = 0 ; i < 6 ; i++) {
frame.getContentPane().remove(labels.get(i));
}
}
});
}
}
When I added the 6 labels outside of linsteners they were properly added to the ContentPane
and displayed. Yet, when I try to do this via the buttons, upon clicking the buttonAdd
nothing happens. They do not get displayed.
I tried messing with the hierarchy, setting the indices manually but nothing worked. I suspect the MouseListeners but I have no idea why this doesn't work.
java swing components containers jlabel
|
show 2 more comments
In my application I have a list of 6 Jlabels
, which are added to the contentPane
in a for
loop. After that I add 2 JButtons
- one for removing all the labels and the second one for adding them again:
public class Test {
private JFrame frame;
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Test window = new Test();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public Test() {
initialize();
}
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 960, 620);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.setLocationRelativeTo(null);
frame.getContentPane().setLayout(null);
frame.getContentPane().setBackground(new Color(30, 30, 30));
LinkedList<JLabel> labels = new LinkedList<>();
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setSize(280, 50);
labels.setBackground(new Color(75, 75, 75));
labels.setOpaque(true);
}
Button buttonAdd = new JButton("Add");
buttonAdd.setBounds(310, 15, 150, 50);
buttonAdd.addMouseListener(new MouseAdapter() {
@Override
public final void mouseClicked(MouseEvent event) {
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setLocation(15, 15+50*i);
frame.getContentPane().add(labels.get(i));
}
}
});
Button buttonRemove = new JButton("Remove");
buttonRemove.setBounds(310, 15, 150, 50);
buttonRemove.addMouseListener(new MouseAdapter() {
@Override
public final void mouseClicked(MouseEvent event) {
for(int i = 0 ; i < 6 ; i++) {
frame.getContentPane().remove(labels.get(i));
}
}
});
}
}
When I added the 6 labels outside of linsteners they were properly added to the ContentPane
and displayed. Yet, when I try to do this via the buttons, upon clicking the buttonAdd
nothing happens. They do not get displayed.
I tried messing with the hierarchy, setting the indices manually but nothing worked. I suspect the MouseListeners but I have no idea why this doesn't work.
java swing components containers jlabel
Is the listener working? Does it print something to the console using sysout? Try this: alvinalexander.com/java/jbutton-listener-pressed-actionlistener
– Marvin Klar
Nov 20 at 22:36
Yes, I have tried printing out something likeSystem.out.println("foo");
and it did showfoo
in the console.
– Virginia
Nov 20 at 22:42
But you called 'remove' instead of 'add' :D didn't saw this first.
– Marvin Klar
Nov 20 at 22:44
little typo, it still doesn't work though :(
– Virginia
Nov 20 at 22:47
1
(1-) 1) The code you posted doesn't compile. Post actual code that you are testing. We don't have time to guess what you really are testing. 2) You should be adding anActionListener
to the button, not a MouseListener.
– camickr
Nov 21 at 0:29
|
show 2 more comments
In my application I have a list of 6 Jlabels
, which are added to the contentPane
in a for
loop. After that I add 2 JButtons
- one for removing all the labels and the second one for adding them again:
public class Test {
private JFrame frame;
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Test window = new Test();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public Test() {
initialize();
}
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 960, 620);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.setLocationRelativeTo(null);
frame.getContentPane().setLayout(null);
frame.getContentPane().setBackground(new Color(30, 30, 30));
LinkedList<JLabel> labels = new LinkedList<>();
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setSize(280, 50);
labels.setBackground(new Color(75, 75, 75));
labels.setOpaque(true);
}
Button buttonAdd = new JButton("Add");
buttonAdd.setBounds(310, 15, 150, 50);
buttonAdd.addMouseListener(new MouseAdapter() {
@Override
public final void mouseClicked(MouseEvent event) {
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setLocation(15, 15+50*i);
frame.getContentPane().add(labels.get(i));
}
}
});
Button buttonRemove = new JButton("Remove");
buttonRemove.setBounds(310, 15, 150, 50);
buttonRemove.addMouseListener(new MouseAdapter() {
@Override
public final void mouseClicked(MouseEvent event) {
for(int i = 0 ; i < 6 ; i++) {
frame.getContentPane().remove(labels.get(i));
}
}
});
}
}
When I added the 6 labels outside of linsteners they were properly added to the ContentPane
and displayed. Yet, when I try to do this via the buttons, upon clicking the buttonAdd
nothing happens. They do not get displayed.
I tried messing with the hierarchy, setting the indices manually but nothing worked. I suspect the MouseListeners but I have no idea why this doesn't work.
java swing components containers jlabel
In my application I have a list of 6 Jlabels
, which are added to the contentPane
in a for
loop. After that I add 2 JButtons
- one for removing all the labels and the second one for adding them again:
public class Test {
private JFrame frame;
public static void main(String args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Test window = new Test();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public Test() {
initialize();
}
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 960, 620);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.setLocationRelativeTo(null);
frame.getContentPane().setLayout(null);
frame.getContentPane().setBackground(new Color(30, 30, 30));
LinkedList<JLabel> labels = new LinkedList<>();
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setSize(280, 50);
labels.setBackground(new Color(75, 75, 75));
labels.setOpaque(true);
}
Button buttonAdd = new JButton("Add");
buttonAdd.setBounds(310, 15, 150, 50);
buttonAdd.addMouseListener(new MouseAdapter() {
@Override
public final void mouseClicked(MouseEvent event) {
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setLocation(15, 15+50*i);
frame.getContentPane().add(labels.get(i));
}
}
});
Button buttonRemove = new JButton("Remove");
buttonRemove.setBounds(310, 15, 150, 50);
buttonRemove.addMouseListener(new MouseAdapter() {
@Override
public final void mouseClicked(MouseEvent event) {
for(int i = 0 ; i < 6 ; i++) {
frame.getContentPane().remove(labels.get(i));
}
}
});
}
}
When I added the 6 labels outside of linsteners they were properly added to the ContentPane
and displayed. Yet, when I try to do this via the buttons, upon clicking the buttonAdd
nothing happens. They do not get displayed.
I tried messing with the hierarchy, setting the indices manually but nothing worked. I suspect the MouseListeners but I have no idea why this doesn't work.
java swing components containers jlabel
java swing components containers jlabel
edited Nov 20 at 23:11


Andrew Thompson
153k27163338
153k27163338
asked Nov 20 at 22:29
Virginia
165
165
Is the listener working? Does it print something to the console using sysout? Try this: alvinalexander.com/java/jbutton-listener-pressed-actionlistener
– Marvin Klar
Nov 20 at 22:36
Yes, I have tried printing out something likeSystem.out.println("foo");
and it did showfoo
in the console.
– Virginia
Nov 20 at 22:42
But you called 'remove' instead of 'add' :D didn't saw this first.
– Marvin Klar
Nov 20 at 22:44
little typo, it still doesn't work though :(
– Virginia
Nov 20 at 22:47
1
(1-) 1) The code you posted doesn't compile. Post actual code that you are testing. We don't have time to guess what you really are testing. 2) You should be adding anActionListener
to the button, not a MouseListener.
– camickr
Nov 21 at 0:29
|
show 2 more comments
Is the listener working? Does it print something to the console using sysout? Try this: alvinalexander.com/java/jbutton-listener-pressed-actionlistener
– Marvin Klar
Nov 20 at 22:36
Yes, I have tried printing out something likeSystem.out.println("foo");
and it did showfoo
in the console.
– Virginia
Nov 20 at 22:42
But you called 'remove' instead of 'add' :D didn't saw this first.
– Marvin Klar
Nov 20 at 22:44
little typo, it still doesn't work though :(
– Virginia
Nov 20 at 22:47
1
(1-) 1) The code you posted doesn't compile. Post actual code that you are testing. We don't have time to guess what you really are testing. 2) You should be adding anActionListener
to the button, not a MouseListener.
– camickr
Nov 21 at 0:29
Is the listener working? Does it print something to the console using sysout? Try this: alvinalexander.com/java/jbutton-listener-pressed-actionlistener
– Marvin Klar
Nov 20 at 22:36
Is the listener working? Does it print something to the console using sysout? Try this: alvinalexander.com/java/jbutton-listener-pressed-actionlistener
– Marvin Klar
Nov 20 at 22:36
Yes, I have tried printing out something like
System.out.println("foo");
and it did show foo
in the console.– Virginia
Nov 20 at 22:42
Yes, I have tried printing out something like
System.out.println("foo");
and it did show foo
in the console.– Virginia
Nov 20 at 22:42
But you called 'remove' instead of 'add' :D didn't saw this first.
– Marvin Klar
Nov 20 at 22:44
But you called 'remove' instead of 'add' :D didn't saw this first.
– Marvin Klar
Nov 20 at 22:44
little typo, it still doesn't work though :(
– Virginia
Nov 20 at 22:47
little typo, it still doesn't work though :(
– Virginia
Nov 20 at 22:47
1
1
(1-) 1) The code you posted doesn't compile. Post actual code that you are testing. We don't have time to guess what you really are testing. 2) You should be adding an
ActionListener
to the button, not a MouseListener.– camickr
Nov 21 at 0:29
(1-) 1) The code you posted doesn't compile. Post actual code that you are testing. We don't have time to guess what you really are testing. 2) You should be adding an
ActionListener
to the button, not a MouseListener.– camickr
Nov 21 at 0:29
|
show 2 more comments
1 Answer
1
active
oldest
votes
First things first;
-Anything modifies the GUI has to be done in Event Dispatch Thread(EDT). You can read more why it is needed from this answer.
You have to call:
Test.this.frame.revalidate();
Test.this.frame.repaint();
Like below:
for ( int i = 0; i < 6; i++ )
{
final JLabel l = labels.get( i );
l.setLocation( 15, 15 + (50 * i) );
Test.this.frame.getContentPane().add( l );
}
Test.this.frame.revalidate();
Test.this.frame.repaint();
Asides this I see in your code you use Button
instead of JButton
, I am assuming it is just a typing mistake. It should be JButton
. Also
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setSize(280, 50);
labels.setBackground(new Color(75, 75, 75));
labels.setOpaque(true);
}
this piece of code is just wrong, labels is a list
not a JLabel
. Define local variable
JLabel labelToAdd = labels.get(i)
labelToAdd.setSize(280, 50);
labelToAdd.setBackground(new Color(75, 75, 75));
labelToAdd.setOpaque(true);
1
Anything modifies the GUI has to be done in Event Dispatch Thread(EDT).
- correct, but code executed from within a listener does execute on the EDT, so there is no need to use the invokeLater().
– camickr
Nov 21 at 0:21
Thank you for correcting me, I modified my answer.
– Bleach
Nov 21 at 8:51
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402551%2fcomponents-not-appearing-in-contentpane%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
First things first;
-Anything modifies the GUI has to be done in Event Dispatch Thread(EDT). You can read more why it is needed from this answer.
You have to call:
Test.this.frame.revalidate();
Test.this.frame.repaint();
Like below:
for ( int i = 0; i < 6; i++ )
{
final JLabel l = labels.get( i );
l.setLocation( 15, 15 + (50 * i) );
Test.this.frame.getContentPane().add( l );
}
Test.this.frame.revalidate();
Test.this.frame.repaint();
Asides this I see in your code you use Button
instead of JButton
, I am assuming it is just a typing mistake. It should be JButton
. Also
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setSize(280, 50);
labels.setBackground(new Color(75, 75, 75));
labels.setOpaque(true);
}
this piece of code is just wrong, labels is a list
not a JLabel
. Define local variable
JLabel labelToAdd = labels.get(i)
labelToAdd.setSize(280, 50);
labelToAdd.setBackground(new Color(75, 75, 75));
labelToAdd.setOpaque(true);
1
Anything modifies the GUI has to be done in Event Dispatch Thread(EDT).
- correct, but code executed from within a listener does execute on the EDT, so there is no need to use the invokeLater().
– camickr
Nov 21 at 0:21
Thank you for correcting me, I modified my answer.
– Bleach
Nov 21 at 8:51
add a comment |
First things first;
-Anything modifies the GUI has to be done in Event Dispatch Thread(EDT). You can read more why it is needed from this answer.
You have to call:
Test.this.frame.revalidate();
Test.this.frame.repaint();
Like below:
for ( int i = 0; i < 6; i++ )
{
final JLabel l = labels.get( i );
l.setLocation( 15, 15 + (50 * i) );
Test.this.frame.getContentPane().add( l );
}
Test.this.frame.revalidate();
Test.this.frame.repaint();
Asides this I see in your code you use Button
instead of JButton
, I am assuming it is just a typing mistake. It should be JButton
. Also
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setSize(280, 50);
labels.setBackground(new Color(75, 75, 75));
labels.setOpaque(true);
}
this piece of code is just wrong, labels is a list
not a JLabel
. Define local variable
JLabel labelToAdd = labels.get(i)
labelToAdd.setSize(280, 50);
labelToAdd.setBackground(new Color(75, 75, 75));
labelToAdd.setOpaque(true);
1
Anything modifies the GUI has to be done in Event Dispatch Thread(EDT).
- correct, but code executed from within a listener does execute on the EDT, so there is no need to use the invokeLater().
– camickr
Nov 21 at 0:21
Thank you for correcting me, I modified my answer.
– Bleach
Nov 21 at 8:51
add a comment |
First things first;
-Anything modifies the GUI has to be done in Event Dispatch Thread(EDT). You can read more why it is needed from this answer.
You have to call:
Test.this.frame.revalidate();
Test.this.frame.repaint();
Like below:
for ( int i = 0; i < 6; i++ )
{
final JLabel l = labels.get( i );
l.setLocation( 15, 15 + (50 * i) );
Test.this.frame.getContentPane().add( l );
}
Test.this.frame.revalidate();
Test.this.frame.repaint();
Asides this I see in your code you use Button
instead of JButton
, I am assuming it is just a typing mistake. It should be JButton
. Also
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setSize(280, 50);
labels.setBackground(new Color(75, 75, 75));
labels.setOpaque(true);
}
this piece of code is just wrong, labels is a list
not a JLabel
. Define local variable
JLabel labelToAdd = labels.get(i)
labelToAdd.setSize(280, 50);
labelToAdd.setBackground(new Color(75, 75, 75));
labelToAdd.setOpaque(true);
First things first;
-Anything modifies the GUI has to be done in Event Dispatch Thread(EDT). You can read more why it is needed from this answer.
You have to call:
Test.this.frame.revalidate();
Test.this.frame.repaint();
Like below:
for ( int i = 0; i < 6; i++ )
{
final JLabel l = labels.get( i );
l.setLocation( 15, 15 + (50 * i) );
Test.this.frame.getContentPane().add( l );
}
Test.this.frame.revalidate();
Test.this.frame.repaint();
Asides this I see in your code you use Button
instead of JButton
, I am assuming it is just a typing mistake. It should be JButton
. Also
for(int i = 0 ; i < 6 ; i++) {
labels.get(i).setSize(280, 50);
labels.setBackground(new Color(75, 75, 75));
labels.setOpaque(true);
}
this piece of code is just wrong, labels is a list
not a JLabel
. Define local variable
JLabel labelToAdd = labels.get(i)
labelToAdd.setSize(280, 50);
labelToAdd.setBackground(new Color(75, 75, 75));
labelToAdd.setOpaque(true);
edited Nov 21 at 8:52
answered Nov 20 at 23:07


Bleach
93111
93111
1
Anything modifies the GUI has to be done in Event Dispatch Thread(EDT).
- correct, but code executed from within a listener does execute on the EDT, so there is no need to use the invokeLater().
– camickr
Nov 21 at 0:21
Thank you for correcting me, I modified my answer.
– Bleach
Nov 21 at 8:51
add a comment |
1
Anything modifies the GUI has to be done in Event Dispatch Thread(EDT).
- correct, but code executed from within a listener does execute on the EDT, so there is no need to use the invokeLater().
– camickr
Nov 21 at 0:21
Thank you for correcting me, I modified my answer.
– Bleach
Nov 21 at 8:51
1
1
Anything modifies the GUI has to be done in Event Dispatch Thread(EDT).
- correct, but code executed from within a listener does execute on the EDT, so there is no need to use the invokeLater().– camickr
Nov 21 at 0:21
Anything modifies the GUI has to be done in Event Dispatch Thread(EDT).
- correct, but code executed from within a listener does execute on the EDT, so there is no need to use the invokeLater().– camickr
Nov 21 at 0:21
Thank you for correcting me, I modified my answer.
– Bleach
Nov 21 at 8:51
Thank you for correcting me, I modified my answer.
– Bleach
Nov 21 at 8:51
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402551%2fcomponents-not-appearing-in-contentpane%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
I849MSc4zRd N K4kuhtxkikvaI lEwbq
Is the listener working? Does it print something to the console using sysout? Try this: alvinalexander.com/java/jbutton-listener-pressed-actionlistener
– Marvin Klar
Nov 20 at 22:36
Yes, I have tried printing out something like
System.out.println("foo");
and it did showfoo
in the console.– Virginia
Nov 20 at 22:42
But you called 'remove' instead of 'add' :D didn't saw this first.
– Marvin Klar
Nov 20 at 22:44
little typo, it still doesn't work though :(
– Virginia
Nov 20 at 22:47
1
(1-) 1) The code you posted doesn't compile. Post actual code that you are testing. We don't have time to guess what you really are testing. 2) You should be adding an
ActionListener
to the button, not a MouseListener.– camickr
Nov 21 at 0:29