Match string in between two strings
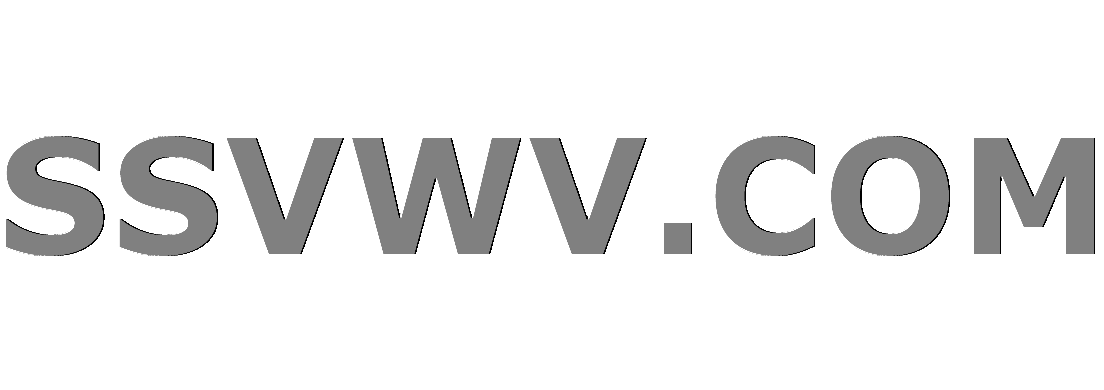
Multi tool use
If I have a string like this:
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
I want to get the strings between each of the substrings "play" and "in", so basically an array with "the Ukelele" and "the Guitar".
Right now I'm doing:
var test = str.match("play(.*)in");
But that's returning the string between the first "play" and last "in", so I get "the Ukulele in Lebanon. Play the Guitar" instead of 2 separate strings. Does anyone know how to globally search a string for all occurrences of a substring between a starting and ending string?
javascript regex string string-matching
add a comment |
If I have a string like this:
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
I want to get the strings between each of the substrings "play" and "in", so basically an array with "the Ukelele" and "the Guitar".
Right now I'm doing:
var test = str.match("play(.*)in");
But that's returning the string between the first "play" and last "in", so I get "the Ukulele in Lebanon. Play the Guitar" instead of 2 separate strings. Does anyone know how to globally search a string for all occurrences of a substring between a starting and ending string?
javascript regex string string-matching
2
str.match("play(.*)in")
==>str.match(/play(.*?)in/g)
– Tushar
Feb 26 '16 at 5:43
add a comment |
If I have a string like this:
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
I want to get the strings between each of the substrings "play" and "in", so basically an array with "the Ukelele" and "the Guitar".
Right now I'm doing:
var test = str.match("play(.*)in");
But that's returning the string between the first "play" and last "in", so I get "the Ukulele in Lebanon. Play the Guitar" instead of 2 separate strings. Does anyone know how to globally search a string for all occurrences of a substring between a starting and ending string?
javascript regex string string-matching
If I have a string like this:
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
I want to get the strings between each of the substrings "play" and "in", so basically an array with "the Ukelele" and "the Guitar".
Right now I'm doing:
var test = str.match("play(.*)in");
But that's returning the string between the first "play" and last "in", so I get "the Ukulele in Lebanon. Play the Guitar" instead of 2 separate strings. Does anyone know how to globally search a string for all occurrences of a substring between a starting and ending string?
javascript regex string string-matching
javascript regex string string-matching
edited Feb 26 '16 at 5:48


Tushar
67.8k1195121
67.8k1195121
asked Feb 26 '16 at 5:42


MarksCodeMarksCode
1,18311755
1,18311755
2
str.match("play(.*)in")
==>str.match(/play(.*?)in/g)
– Tushar
Feb 26 '16 at 5:43
add a comment |
2
str.match("play(.*)in")
==>str.match(/play(.*?)in/g)
– Tushar
Feb 26 '16 at 5:43
2
2
str.match("play(.*)in")
==> str.match(/play(.*?)in/g)
– Tushar
Feb 26 '16 at 5:43
str.match("play(.*)in")
==> str.match(/play(.*?)in/g)
– Tushar
Feb 26 '16 at 5:43
add a comment |
4 Answers
4
active
oldest
votes
You can use the regex
plays*(.*?)s*in
- Use the
/
as delimiters for regex literal syntax - Use the lazy group to match minimal possible
Demo:
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
var regex = /plays*(.*?)s*in/g;
var matches = ;
while (m = regex.exec(str)) {
matches.push(m[1]);
}
document.body.innerHTML = '<pre>' + JSON.stringify(matches, 0, 4) + '</pre>';
I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:25
thanks this is perfect ;)
– STEEL
May 13 '18 at 16:41
add a comment |
/bplays+(.+?)s+inb/ig
might be more specific and might work better for you.
I believe there may be some issues with the regexes offered previously. For instance, /plays*(.*?)s*in/g
will find a match within "displaying photographs in sequence". Of course this is not what you want. One of the problems is that there is nothing specifying that "play" should be a discrete word. It needs a word boundary before it and at least one instance of white space after it (it can't be optional). Similarly, the white space after the capture group should not be optional.
The other expression offered at the time I added this, /play (.+?) in/g
, lacks the word boundary token before "play" and after "in", so it will contain a match in "display blue ink". This is not what you want.
As to your expression, it was missing the word boundary and white space tokens as well. But as another mentioned, it also needed the wildcard to be lazy. Otherwise, given your example string, your match would start with the first instance of "play" and end with the 2nd instance of "in".
If issues with my offered expression are found, would appreciate feedback.
add a comment |
You are so close to the right answer. There are a few things you may be overlooking:
- You need your match to be non-greedy, this can be accomplished by using the
?
operator - Do not use the
String.match()
method as it's proven to match the entirety of the pattern and does not pay attention to capturing groups as you would expect. An alternative is to useRegExp.exec()
orString.replace()
, but using replace would require a little more work, so stick to building your own array with exec
var str = "display the Ukulele in Lebanon. play the Guitar in Lebanon.";
var re = /bplay (.+?) inb/g;
var matches = ;
var match;
while ( match = re.exec(str) ){
matches[ matches.length ] = match[1];
}
document.getElementById('demo').innerHTML = JSON.stringify( matches );
<pre id="demo"></pre>
Thankyou sir, this is an excellent answer. Another user gave me the regex of/plays*(.*?)s*in/g
but yours looks much simpler. The syntax looks pretty messy so I'm still trying to understand it.
– MarksCode
Feb 26 '16 at 6:07
I was busy typing and din't notice that @Tushar came to almost the same answer, except for the value assignment to the array. In JavaScript you can use `,
s, or
` to all reference a space. Just be careful elsewhere, like Perl, where the ` ` could be ignored. Alsos
refers to more than just whitespace, it could mean a tab or a newline character.
– vol7ron
Feb 26 '16 at 6:11
@vol7ron: I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:23
@Jon thanks, you're correct, this could use word boundaries. Keep in mind that even word boundaries could have issues with hyphenations. The most robust solution would require many more lines of logic - or a negative lookbehind (which I don't think ECMAScript RegEx permits). So this also requires the OP to be more specific about the string(s) being evaluated. That said, theb
would be a good thing to include.
– vol7ron
Mar 1 '16 at 3:39
@vol7ron: Yes,b
can have issues with many special characters. It's possible that I'm making more of this than needs to be as the string the OP is dealing with may vary little from what he provided above, in which caseb
would be unnecessary. Also, his question may have been really just about greedy vs lazy. But I suppose that while the issue of potential problems withb
has been raised (and as you alluded, without knowing more about possible variation in his input string), maybe the following would be safer:/(?:s|^)plays+(.+?)s+ins/ig
.
– Jon
Mar 1 '16 at 4:17
add a comment |
A victim of greedy matching.
.* finds the longest possible match,
while .*? finds the shortest possible match.
For the example given str will be an array or 3 strings containing:
the Ukelele
the Guitar
Lebanon
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f35644346%2fmatch-string-in-between-two-strings%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use the regex
plays*(.*?)s*in
- Use the
/
as delimiters for regex literal syntax - Use the lazy group to match minimal possible
Demo:
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
var regex = /plays*(.*?)s*in/g;
var matches = ;
while (m = regex.exec(str)) {
matches.push(m[1]);
}
document.body.innerHTML = '<pre>' + JSON.stringify(matches, 0, 4) + '</pre>';
I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:25
thanks this is perfect ;)
– STEEL
May 13 '18 at 16:41
add a comment |
You can use the regex
plays*(.*?)s*in
- Use the
/
as delimiters for regex literal syntax - Use the lazy group to match minimal possible
Demo:
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
var regex = /plays*(.*?)s*in/g;
var matches = ;
while (m = regex.exec(str)) {
matches.push(m[1]);
}
document.body.innerHTML = '<pre>' + JSON.stringify(matches, 0, 4) + '</pre>';
I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:25
thanks this is perfect ;)
– STEEL
May 13 '18 at 16:41
add a comment |
You can use the regex
plays*(.*?)s*in
- Use the
/
as delimiters for regex literal syntax - Use the lazy group to match minimal possible
Demo:
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
var regex = /plays*(.*?)s*in/g;
var matches = ;
while (m = regex.exec(str)) {
matches.push(m[1]);
}
document.body.innerHTML = '<pre>' + JSON.stringify(matches, 0, 4) + '</pre>';
You can use the regex
plays*(.*?)s*in
- Use the
/
as delimiters for regex literal syntax - Use the lazy group to match minimal possible
Demo:
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
var regex = /plays*(.*?)s*in/g;
var matches = ;
while (m = regex.exec(str)) {
matches.push(m[1]);
}
document.body.innerHTML = '<pre>' + JSON.stringify(matches, 0, 4) + '</pre>';
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
var regex = /plays*(.*?)s*in/g;
var matches = ;
while (m = regex.exec(str)) {
matches.push(m[1]);
}
document.body.innerHTML = '<pre>' + JSON.stringify(matches, 0, 4) + '</pre>';
var str = "play the Ukulele in Lebanon. play the Guitar in Lebanon.";
var regex = /plays*(.*?)s*in/g;
var matches = ;
while (m = regex.exec(str)) {
matches.push(m[1]);
}
document.body.innerHTML = '<pre>' + JSON.stringify(matches, 0, 4) + '</pre>';
edited Feb 26 '16 at 5:46
answered Feb 26 '16 at 5:44


TusharTushar
67.8k1195121
67.8k1195121
I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:25
thanks this is perfect ;)
– STEEL
May 13 '18 at 16:41
add a comment |
I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:25
thanks this is perfect ;)
– STEEL
May 13 '18 at 16:41
I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:25
I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:25
thanks this is perfect ;)
– STEEL
May 13 '18 at 16:41
thanks this is perfect ;)
– STEEL
May 13 '18 at 16:41
add a comment |
/bplays+(.+?)s+inb/ig
might be more specific and might work better for you.
I believe there may be some issues with the regexes offered previously. For instance, /plays*(.*?)s*in/g
will find a match within "displaying photographs in sequence". Of course this is not what you want. One of the problems is that there is nothing specifying that "play" should be a discrete word. It needs a word boundary before it and at least one instance of white space after it (it can't be optional). Similarly, the white space after the capture group should not be optional.
The other expression offered at the time I added this, /play (.+?) in/g
, lacks the word boundary token before "play" and after "in", so it will contain a match in "display blue ink". This is not what you want.
As to your expression, it was missing the word boundary and white space tokens as well. But as another mentioned, it also needed the wildcard to be lazy. Otherwise, given your example string, your match would start with the first instance of "play" and end with the 2nd instance of "in".
If issues with my offered expression are found, would appreciate feedback.
add a comment |
/bplays+(.+?)s+inb/ig
might be more specific and might work better for you.
I believe there may be some issues with the regexes offered previously. For instance, /plays*(.*?)s*in/g
will find a match within "displaying photographs in sequence". Of course this is not what you want. One of the problems is that there is nothing specifying that "play" should be a discrete word. It needs a word boundary before it and at least one instance of white space after it (it can't be optional). Similarly, the white space after the capture group should not be optional.
The other expression offered at the time I added this, /play (.+?) in/g
, lacks the word boundary token before "play" and after "in", so it will contain a match in "display blue ink". This is not what you want.
As to your expression, it was missing the word boundary and white space tokens as well. But as another mentioned, it also needed the wildcard to be lazy. Otherwise, given your example string, your match would start with the first instance of "play" and end with the 2nd instance of "in".
If issues with my offered expression are found, would appreciate feedback.
add a comment |
/bplays+(.+?)s+inb/ig
might be more specific and might work better for you.
I believe there may be some issues with the regexes offered previously. For instance, /plays*(.*?)s*in/g
will find a match within "displaying photographs in sequence". Of course this is not what you want. One of the problems is that there is nothing specifying that "play" should be a discrete word. It needs a word boundary before it and at least one instance of white space after it (it can't be optional). Similarly, the white space after the capture group should not be optional.
The other expression offered at the time I added this, /play (.+?) in/g
, lacks the word boundary token before "play" and after "in", so it will contain a match in "display blue ink". This is not what you want.
As to your expression, it was missing the word boundary and white space tokens as well. But as another mentioned, it also needed the wildcard to be lazy. Otherwise, given your example string, your match would start with the first instance of "play" and end with the 2nd instance of "in".
If issues with my offered expression are found, would appreciate feedback.
/bplays+(.+?)s+inb/ig
might be more specific and might work better for you.
I believe there may be some issues with the regexes offered previously. For instance, /plays*(.*?)s*in/g
will find a match within "displaying photographs in sequence". Of course this is not what you want. One of the problems is that there is nothing specifying that "play" should be a discrete word. It needs a word boundary before it and at least one instance of white space after it (it can't be optional). Similarly, the white space after the capture group should not be optional.
The other expression offered at the time I added this, /play (.+?) in/g
, lacks the word boundary token before "play" and after "in", so it will contain a match in "display blue ink". This is not what you want.
As to your expression, it was missing the word boundary and white space tokens as well. But as another mentioned, it also needed the wildcard to be lazy. Otherwise, given your example string, your match would start with the first instance of "play" and end with the 2nd instance of "in".
If issues with my offered expression are found, would appreciate feedback.
edited Mar 1 '16 at 3:29
answered Mar 1 '16 at 3:22


JonJon
236139
236139
add a comment |
add a comment |
You are so close to the right answer. There are a few things you may be overlooking:
- You need your match to be non-greedy, this can be accomplished by using the
?
operator - Do not use the
String.match()
method as it's proven to match the entirety of the pattern and does not pay attention to capturing groups as you would expect. An alternative is to useRegExp.exec()
orString.replace()
, but using replace would require a little more work, so stick to building your own array with exec
var str = "display the Ukulele in Lebanon. play the Guitar in Lebanon.";
var re = /bplay (.+?) inb/g;
var matches = ;
var match;
while ( match = re.exec(str) ){
matches[ matches.length ] = match[1];
}
document.getElementById('demo').innerHTML = JSON.stringify( matches );
<pre id="demo"></pre>
Thankyou sir, this is an excellent answer. Another user gave me the regex of/plays*(.*?)s*in/g
but yours looks much simpler. The syntax looks pretty messy so I'm still trying to understand it.
– MarksCode
Feb 26 '16 at 6:07
I was busy typing and din't notice that @Tushar came to almost the same answer, except for the value assignment to the array. In JavaScript you can use `,
s, or
` to all reference a space. Just be careful elsewhere, like Perl, where the ` ` could be ignored. Alsos
refers to more than just whitespace, it could mean a tab or a newline character.
– vol7ron
Feb 26 '16 at 6:11
@vol7ron: I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:23
@Jon thanks, you're correct, this could use word boundaries. Keep in mind that even word boundaries could have issues with hyphenations. The most robust solution would require many more lines of logic - or a negative lookbehind (which I don't think ECMAScript RegEx permits). So this also requires the OP to be more specific about the string(s) being evaluated. That said, theb
would be a good thing to include.
– vol7ron
Mar 1 '16 at 3:39
@vol7ron: Yes,b
can have issues with many special characters. It's possible that I'm making more of this than needs to be as the string the OP is dealing with may vary little from what he provided above, in which caseb
would be unnecessary. Also, his question may have been really just about greedy vs lazy. But I suppose that while the issue of potential problems withb
has been raised (and as you alluded, without knowing more about possible variation in his input string), maybe the following would be safer:/(?:s|^)plays+(.+?)s+ins/ig
.
– Jon
Mar 1 '16 at 4:17
add a comment |
You are so close to the right answer. There are a few things you may be overlooking:
- You need your match to be non-greedy, this can be accomplished by using the
?
operator - Do not use the
String.match()
method as it's proven to match the entirety of the pattern and does not pay attention to capturing groups as you would expect. An alternative is to useRegExp.exec()
orString.replace()
, but using replace would require a little more work, so stick to building your own array with exec
var str = "display the Ukulele in Lebanon. play the Guitar in Lebanon.";
var re = /bplay (.+?) inb/g;
var matches = ;
var match;
while ( match = re.exec(str) ){
matches[ matches.length ] = match[1];
}
document.getElementById('demo').innerHTML = JSON.stringify( matches );
<pre id="demo"></pre>
Thankyou sir, this is an excellent answer. Another user gave me the regex of/plays*(.*?)s*in/g
but yours looks much simpler. The syntax looks pretty messy so I'm still trying to understand it.
– MarksCode
Feb 26 '16 at 6:07
I was busy typing and din't notice that @Tushar came to almost the same answer, except for the value assignment to the array. In JavaScript you can use `,
s, or
` to all reference a space. Just be careful elsewhere, like Perl, where the ` ` could be ignored. Alsos
refers to more than just whitespace, it could mean a tab or a newline character.
– vol7ron
Feb 26 '16 at 6:11
@vol7ron: I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:23
@Jon thanks, you're correct, this could use word boundaries. Keep in mind that even word boundaries could have issues with hyphenations. The most robust solution would require many more lines of logic - or a negative lookbehind (which I don't think ECMAScript RegEx permits). So this also requires the OP to be more specific about the string(s) being evaluated. That said, theb
would be a good thing to include.
– vol7ron
Mar 1 '16 at 3:39
@vol7ron: Yes,b
can have issues with many special characters. It's possible that I'm making more of this than needs to be as the string the OP is dealing with may vary little from what he provided above, in which caseb
would be unnecessary. Also, his question may have been really just about greedy vs lazy. But I suppose that while the issue of potential problems withb
has been raised (and as you alluded, without knowing more about possible variation in his input string), maybe the following would be safer:/(?:s|^)plays+(.+?)s+ins/ig
.
– Jon
Mar 1 '16 at 4:17
add a comment |
You are so close to the right answer. There are a few things you may be overlooking:
- You need your match to be non-greedy, this can be accomplished by using the
?
operator - Do not use the
String.match()
method as it's proven to match the entirety of the pattern and does not pay attention to capturing groups as you would expect. An alternative is to useRegExp.exec()
orString.replace()
, but using replace would require a little more work, so stick to building your own array with exec
var str = "display the Ukulele in Lebanon. play the Guitar in Lebanon.";
var re = /bplay (.+?) inb/g;
var matches = ;
var match;
while ( match = re.exec(str) ){
matches[ matches.length ] = match[1];
}
document.getElementById('demo').innerHTML = JSON.stringify( matches );
<pre id="demo"></pre>
You are so close to the right answer. There are a few things you may be overlooking:
- You need your match to be non-greedy, this can be accomplished by using the
?
operator - Do not use the
String.match()
method as it's proven to match the entirety of the pattern and does not pay attention to capturing groups as you would expect. An alternative is to useRegExp.exec()
orString.replace()
, but using replace would require a little more work, so stick to building your own array with exec
var str = "display the Ukulele in Lebanon. play the Guitar in Lebanon.";
var re = /bplay (.+?) inb/g;
var matches = ;
var match;
while ( match = re.exec(str) ){
matches[ matches.length ] = match[1];
}
document.getElementById('demo').innerHTML = JSON.stringify( matches );
<pre id="demo"></pre>
var str = "display the Ukulele in Lebanon. play the Guitar in Lebanon.";
var re = /bplay (.+?) inb/g;
var matches = ;
var match;
while ( match = re.exec(str) ){
matches[ matches.length ] = match[1];
}
document.getElementById('demo').innerHTML = JSON.stringify( matches );
<pre id="demo"></pre>
var str = "display the Ukulele in Lebanon. play the Guitar in Lebanon.";
var re = /bplay (.+?) inb/g;
var matches = ;
var match;
while ( match = re.exec(str) ){
matches[ matches.length ] = match[1];
}
document.getElementById('demo').innerHTML = JSON.stringify( matches );
<pre id="demo"></pre>
edited Mar 1 '16 at 3:42
answered Feb 26 '16 at 6:02
vol7ronvol7ron
24.9k1587149
24.9k1587149
Thankyou sir, this is an excellent answer. Another user gave me the regex of/plays*(.*?)s*in/g
but yours looks much simpler. The syntax looks pretty messy so I'm still trying to understand it.
– MarksCode
Feb 26 '16 at 6:07
I was busy typing and din't notice that @Tushar came to almost the same answer, except for the value assignment to the array. In JavaScript you can use `,
s, or
` to all reference a space. Just be careful elsewhere, like Perl, where the ` ` could be ignored. Alsos
refers to more than just whitespace, it could mean a tab or a newline character.
– vol7ron
Feb 26 '16 at 6:11
@vol7ron: I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:23
@Jon thanks, you're correct, this could use word boundaries. Keep in mind that even word boundaries could have issues with hyphenations. The most robust solution would require many more lines of logic - or a negative lookbehind (which I don't think ECMAScript RegEx permits). So this also requires the OP to be more specific about the string(s) being evaluated. That said, theb
would be a good thing to include.
– vol7ron
Mar 1 '16 at 3:39
@vol7ron: Yes,b
can have issues with many special characters. It's possible that I'm making more of this than needs to be as the string the OP is dealing with may vary little from what he provided above, in which caseb
would be unnecessary. Also, his question may have been really just about greedy vs lazy. But I suppose that while the issue of potential problems withb
has been raised (and as you alluded, without knowing more about possible variation in his input string), maybe the following would be safer:/(?:s|^)plays+(.+?)s+ins/ig
.
– Jon
Mar 1 '16 at 4:17
add a comment |
Thankyou sir, this is an excellent answer. Another user gave me the regex of/plays*(.*?)s*in/g
but yours looks much simpler. The syntax looks pretty messy so I'm still trying to understand it.
– MarksCode
Feb 26 '16 at 6:07
I was busy typing and din't notice that @Tushar came to almost the same answer, except for the value assignment to the array. In JavaScript you can use `,
s, or
` to all reference a space. Just be careful elsewhere, like Perl, where the ` ` could be ignored. Alsos
refers to more than just whitespace, it could mean a tab or a newline character.
– vol7ron
Feb 26 '16 at 6:11
@vol7ron: I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:23
@Jon thanks, you're correct, this could use word boundaries. Keep in mind that even word boundaries could have issues with hyphenations. The most robust solution would require many more lines of logic - or a negative lookbehind (which I don't think ECMAScript RegEx permits). So this also requires the OP to be more specific about the string(s) being evaluated. That said, theb
would be a good thing to include.
– vol7ron
Mar 1 '16 at 3:39
@vol7ron: Yes,b
can have issues with many special characters. It's possible that I'm making more of this than needs to be as the string the OP is dealing with may vary little from what he provided above, in which caseb
would be unnecessary. Also, his question may have been really just about greedy vs lazy. But I suppose that while the issue of potential problems withb
has been raised (and as you alluded, without knowing more about possible variation in his input string), maybe the following would be safer:/(?:s|^)plays+(.+?)s+ins/ig
.
– Jon
Mar 1 '16 at 4:17
Thankyou sir, this is an excellent answer. Another user gave me the regex of
/plays*(.*?)s*in/g
but yours looks much simpler. The syntax looks pretty messy so I'm still trying to understand it.– MarksCode
Feb 26 '16 at 6:07
Thankyou sir, this is an excellent answer. Another user gave me the regex of
/plays*(.*?)s*in/g
but yours looks much simpler. The syntax looks pretty messy so I'm still trying to understand it.– MarksCode
Feb 26 '16 at 6:07
I was busy typing and din't notice that @Tushar came to almost the same answer, except for the value assignment to the array. In JavaScript you can use `
,
s, or
` to all reference a space. Just be careful elsewhere, like Perl, where the ` ` could be ignored. Also s
refers to more than just whitespace, it could mean a tab or a newline character.– vol7ron
Feb 26 '16 at 6:11
I was busy typing and din't notice that @Tushar came to almost the same answer, except for the value assignment to the array. In JavaScript you can use `
,
s, or
` to all reference a space. Just be careful elsewhere, like Perl, where the ` ` could be ignored. Also s
refers to more than just whitespace, it could mean a tab or a newline character.– vol7ron
Feb 26 '16 at 6:11
@vol7ron: I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:23
@vol7ron: I found some possible issues in your expression. I referenced it in my answer.
– Jon
Mar 1 '16 at 3:23
@Jon thanks, you're correct, this could use word boundaries. Keep in mind that even word boundaries could have issues with hyphenations. The most robust solution would require many more lines of logic - or a negative lookbehind (which I don't think ECMAScript RegEx permits). So this also requires the OP to be more specific about the string(s) being evaluated. That said, the
b
would be a good thing to include.– vol7ron
Mar 1 '16 at 3:39
@Jon thanks, you're correct, this could use word boundaries. Keep in mind that even word boundaries could have issues with hyphenations. The most robust solution would require many more lines of logic - or a negative lookbehind (which I don't think ECMAScript RegEx permits). So this also requires the OP to be more specific about the string(s) being evaluated. That said, the
b
would be a good thing to include.– vol7ron
Mar 1 '16 at 3:39
@vol7ron: Yes,
b
can have issues with many special characters. It's possible that I'm making more of this than needs to be as the string the OP is dealing with may vary little from what he provided above, in which case b
would be unnecessary. Also, his question may have been really just about greedy vs lazy. But I suppose that while the issue of potential problems with b
has been raised (and as you alluded, without knowing more about possible variation in his input string), maybe the following would be safer: /(?:s|^)plays+(.+?)s+ins/ig
.– Jon
Mar 1 '16 at 4:17
@vol7ron: Yes,
b
can have issues with many special characters. It's possible that I'm making more of this than needs to be as the string the OP is dealing with may vary little from what he provided above, in which case b
would be unnecessary. Also, his question may have been really just about greedy vs lazy. But I suppose that while the issue of potential problems with b
has been raised (and as you alluded, without knowing more about possible variation in his input string), maybe the following would be safer: /(?:s|^)plays+(.+?)s+ins/ig
.– Jon
Mar 1 '16 at 4:17
add a comment |
A victim of greedy matching.
.* finds the longest possible match,
while .*? finds the shortest possible match.
For the example given str will be an array or 3 strings containing:
the Ukelele
the Guitar
Lebanon
add a comment |
A victim of greedy matching.
.* finds the longest possible match,
while .*? finds the shortest possible match.
For the example given str will be an array or 3 strings containing:
the Ukelele
the Guitar
Lebanon
add a comment |
A victim of greedy matching.
.* finds the longest possible match,
while .*? finds the shortest possible match.
For the example given str will be an array or 3 strings containing:
the Ukelele
the Guitar
Lebanon
A victim of greedy matching.
.* finds the longest possible match,
while .*? finds the shortest possible match.
For the example given str will be an array or 3 strings containing:
the Ukelele
the Guitar
Lebanon
answered Feb 26 '16 at 5:51
Arif BurhanArif Burhan
423311
423311
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f35644346%2fmatch-string-in-between-two-strings%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0,3,5tamaRsC jDZ0u951J,Ef,Fqq6ZsW82EHZxKMx8PSf,nd0UCW8p,NwUv2MVf zSIiEjgkaE3vyslHV,VTq,aQk
2
str.match("play(.*)in")
==>str.match(/play(.*?)in/g)
– Tushar
Feb 26 '16 at 5:43