Problems with the function read_new_int
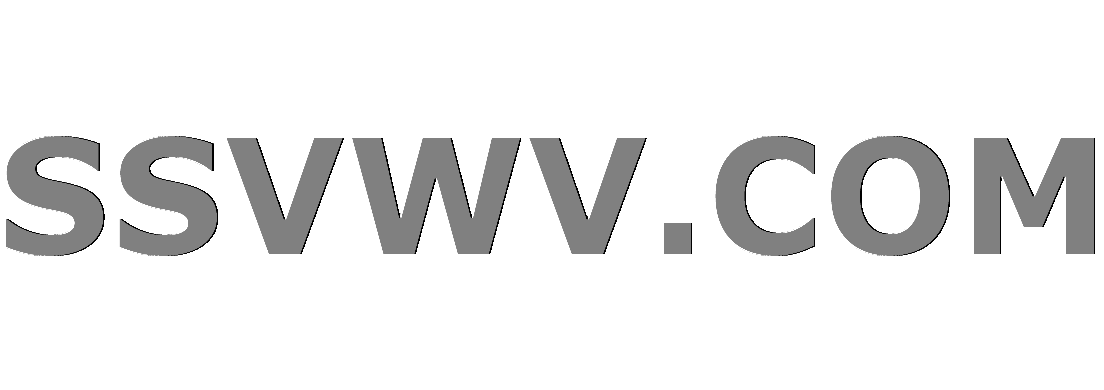
Multi tool use
My program should be a menu of 5 options. In the first it should assign a new value to the variable "n" but when I'm writing in input the option 0, and then I insert 1 as next option, which writes the binary representation of the integer "n" from MSB, I find that "n" has the same value I wrote in input at the begin of my program. I can't really find what is going on.
#include <stdio.h>
#define DIM_F 4
#define DIM 31
// This is a function which reads an integer from input //
void read_new_int(int n);
// This is a function that prints the binary representation of integer n
void print_bin_msb(int n);
// This is a function that calculates the opposite of a binary number and converts it into decimal
void calc_opp_dec(int n);
// This function prints the opposite binary of an integer read in input
void print_opp_bin_msb(int n);
int main(void) {
void (*f[DIM_F])(
int) = {read_new_int , print_bin_msb, calc_opp_dec , print_opp_bin_msb};
int n;
int choice_option;
printf("Insert a positive integer");
while (scanf("%d", &n) != 1) {
printf("Insert a positive integern ");
while (getchar() != 'n')
;
}
do {
printf("0: Read new integer nn1: print the binary representation of integer nn2: Calcolate the opposite of the last integer and printing it as a decimaln3: Calcolate the opposite of an integern4: Print the binary representation of the opposite of the last integern5: End of the programn");
printf("Which option would you choose?");
while (scanf("%d", &choice_option) != 1 && choice_option < 4
&& choice_option >= 0) {
printf("Insert a new integer: ");
while (getchar() != 'n')
;
}
if (choice_option < 4 && choice_option >= 0) {
(*f[choice_option])(n);
} else {
printf("CIAO - programma terminaton");
}
} while (choice_option < 4 && choice_option >= 0);
return 0;
}
void calc_bin(int choice, int n) {
int i;
int arr[DIM];
for (i = 0; i < DIM; i++) {
if (n % 2 == 0)
arr[i] = 0;
else {
arr[i] = 1;
}
n = n / 2;
}
}
void read_new_int(int x) {
while (scanf("%d", &n) != 1) {
printf("Insert a positive integern ");
while (getchar() != 'n')
;
}
}
void print_bin_msb(int n) {
int i;
int temp = 0;
int temp_1 = n;
int tempa = n;
while (!(temp_1 == 0)) {
temp = temp + 1;
temp_1 = temp_1 / 2;
}
if (n >= 0) {
printf("0");
} else {
printf("1");
}
for (i = 0; i < DIM - temp; i++) {
printf("0");
}
do {
if (tempa % 2 == 0) {
printf("0");
} else {
printf("1");
}
tempa = tempa / 2;
} while (tempa != 0);
printf("n");
}
c arrays pointers input
add a comment |
My program should be a menu of 5 options. In the first it should assign a new value to the variable "n" but when I'm writing in input the option 0, and then I insert 1 as next option, which writes the binary representation of the integer "n" from MSB, I find that "n" has the same value I wrote in input at the begin of my program. I can't really find what is going on.
#include <stdio.h>
#define DIM_F 4
#define DIM 31
// This is a function which reads an integer from input //
void read_new_int(int n);
// This is a function that prints the binary representation of integer n
void print_bin_msb(int n);
// This is a function that calculates the opposite of a binary number and converts it into decimal
void calc_opp_dec(int n);
// This function prints the opposite binary of an integer read in input
void print_opp_bin_msb(int n);
int main(void) {
void (*f[DIM_F])(
int) = {read_new_int , print_bin_msb, calc_opp_dec , print_opp_bin_msb};
int n;
int choice_option;
printf("Insert a positive integer");
while (scanf("%d", &n) != 1) {
printf("Insert a positive integern ");
while (getchar() != 'n')
;
}
do {
printf("0: Read new integer nn1: print the binary representation of integer nn2: Calcolate the opposite of the last integer and printing it as a decimaln3: Calcolate the opposite of an integern4: Print the binary representation of the opposite of the last integern5: End of the programn");
printf("Which option would you choose?");
while (scanf("%d", &choice_option) != 1 && choice_option < 4
&& choice_option >= 0) {
printf("Insert a new integer: ");
while (getchar() != 'n')
;
}
if (choice_option < 4 && choice_option >= 0) {
(*f[choice_option])(n);
} else {
printf("CIAO - programma terminaton");
}
} while (choice_option < 4 && choice_option >= 0);
return 0;
}
void calc_bin(int choice, int n) {
int i;
int arr[DIM];
for (i = 0; i < DIM; i++) {
if (n % 2 == 0)
arr[i] = 0;
else {
arr[i] = 1;
}
n = n / 2;
}
}
void read_new_int(int x) {
while (scanf("%d", &n) != 1) {
printf("Insert a positive integern ");
while (getchar() != 'n')
;
}
}
void print_bin_msb(int n) {
int i;
int temp = 0;
int temp_1 = n;
int tempa = n;
while (!(temp_1 == 0)) {
temp = temp + 1;
temp_1 = temp_1 / 2;
}
if (n >= 0) {
printf("0");
} else {
printf("1");
}
for (i = 0; i < DIM - temp; i++) {
printf("0");
}
do {
if (tempa % 2 == 0) {
printf("0");
} else {
printf("1");
}
tempa = tempa / 2;
} while (tempa != 0);
printf("n");
}
c arrays pointers input
If the purpose ofread_new_int
is to read an integer value and set the argument to it, then you should probably go back to your text book or tutorial. C passes all arguments by value. That means when you call a function, the arguments are copied into the local argument variables inside the called function. Any modification to that local argument variable is done to that variable only. The original variable you use when you call the function will not change. You should return the value you read instead.
– Some programmer dude
Nov 22 '18 at 17:25
ok that's right. So i should change also the array of pointers to function as integer and all the functions that i must use in the menu as integer because read_new_int must return an integer, even if the other functions wont return any integer. thats right?
– Giacomo Masciarelli
Nov 22 '18 at 17:30
To keep it simple you could make all functions return anint
. The functions where the returned value doesn't matter just returns zero. And also make all function takeint
arguments, and if they don't use the argument just don't use it.
– Some programmer dude
Nov 22 '18 at 17:48
while (scanf("%d", &choice_option) != 1 && choice_option < 4 && choice_option >= 0) {
does not make as much sense aswhile (scanf("%d", &choice_option) != 1 || choice_option < 4 || choice_option >= 0) {
– chux
Nov 22 '18 at 18:02
I tried to fix this by filling the array with a filler function which does nothing, as first element of the array of pointers , so i could keep the functions as void. By the way i'm pretty sure that this isnt better than just making all the functions return an int. Right? Tho thanks for also pointing out the &&, i forgot about that
– Giacomo Masciarelli
Nov 22 '18 at 18:18
add a comment |
My program should be a menu of 5 options. In the first it should assign a new value to the variable "n" but when I'm writing in input the option 0, and then I insert 1 as next option, which writes the binary representation of the integer "n" from MSB, I find that "n" has the same value I wrote in input at the begin of my program. I can't really find what is going on.
#include <stdio.h>
#define DIM_F 4
#define DIM 31
// This is a function which reads an integer from input //
void read_new_int(int n);
// This is a function that prints the binary representation of integer n
void print_bin_msb(int n);
// This is a function that calculates the opposite of a binary number and converts it into decimal
void calc_opp_dec(int n);
// This function prints the opposite binary of an integer read in input
void print_opp_bin_msb(int n);
int main(void) {
void (*f[DIM_F])(
int) = {read_new_int , print_bin_msb, calc_opp_dec , print_opp_bin_msb};
int n;
int choice_option;
printf("Insert a positive integer");
while (scanf("%d", &n) != 1) {
printf("Insert a positive integern ");
while (getchar() != 'n')
;
}
do {
printf("0: Read new integer nn1: print the binary representation of integer nn2: Calcolate the opposite of the last integer and printing it as a decimaln3: Calcolate the opposite of an integern4: Print the binary representation of the opposite of the last integern5: End of the programn");
printf("Which option would you choose?");
while (scanf("%d", &choice_option) != 1 && choice_option < 4
&& choice_option >= 0) {
printf("Insert a new integer: ");
while (getchar() != 'n')
;
}
if (choice_option < 4 && choice_option >= 0) {
(*f[choice_option])(n);
} else {
printf("CIAO - programma terminaton");
}
} while (choice_option < 4 && choice_option >= 0);
return 0;
}
void calc_bin(int choice, int n) {
int i;
int arr[DIM];
for (i = 0; i < DIM; i++) {
if (n % 2 == 0)
arr[i] = 0;
else {
arr[i] = 1;
}
n = n / 2;
}
}
void read_new_int(int x) {
while (scanf("%d", &n) != 1) {
printf("Insert a positive integern ");
while (getchar() != 'n')
;
}
}
void print_bin_msb(int n) {
int i;
int temp = 0;
int temp_1 = n;
int tempa = n;
while (!(temp_1 == 0)) {
temp = temp + 1;
temp_1 = temp_1 / 2;
}
if (n >= 0) {
printf("0");
} else {
printf("1");
}
for (i = 0; i < DIM - temp; i++) {
printf("0");
}
do {
if (tempa % 2 == 0) {
printf("0");
} else {
printf("1");
}
tempa = tempa / 2;
} while (tempa != 0);
printf("n");
}
c arrays pointers input
My program should be a menu of 5 options. In the first it should assign a new value to the variable "n" but when I'm writing in input the option 0, and then I insert 1 as next option, which writes the binary representation of the integer "n" from MSB, I find that "n" has the same value I wrote in input at the begin of my program. I can't really find what is going on.
#include <stdio.h>
#define DIM_F 4
#define DIM 31
// This is a function which reads an integer from input //
void read_new_int(int n);
// This is a function that prints the binary representation of integer n
void print_bin_msb(int n);
// This is a function that calculates the opposite of a binary number and converts it into decimal
void calc_opp_dec(int n);
// This function prints the opposite binary of an integer read in input
void print_opp_bin_msb(int n);
int main(void) {
void (*f[DIM_F])(
int) = {read_new_int , print_bin_msb, calc_opp_dec , print_opp_bin_msb};
int n;
int choice_option;
printf("Insert a positive integer");
while (scanf("%d", &n) != 1) {
printf("Insert a positive integern ");
while (getchar() != 'n')
;
}
do {
printf("0: Read new integer nn1: print the binary representation of integer nn2: Calcolate the opposite of the last integer and printing it as a decimaln3: Calcolate the opposite of an integern4: Print the binary representation of the opposite of the last integern5: End of the programn");
printf("Which option would you choose?");
while (scanf("%d", &choice_option) != 1 && choice_option < 4
&& choice_option >= 0) {
printf("Insert a new integer: ");
while (getchar() != 'n')
;
}
if (choice_option < 4 && choice_option >= 0) {
(*f[choice_option])(n);
} else {
printf("CIAO - programma terminaton");
}
} while (choice_option < 4 && choice_option >= 0);
return 0;
}
void calc_bin(int choice, int n) {
int i;
int arr[DIM];
for (i = 0; i < DIM; i++) {
if (n % 2 == 0)
arr[i] = 0;
else {
arr[i] = 1;
}
n = n / 2;
}
}
void read_new_int(int x) {
while (scanf("%d", &n) != 1) {
printf("Insert a positive integern ");
while (getchar() != 'n')
;
}
}
void print_bin_msb(int n) {
int i;
int temp = 0;
int temp_1 = n;
int tempa = n;
while (!(temp_1 == 0)) {
temp = temp + 1;
temp_1 = temp_1 / 2;
}
if (n >= 0) {
printf("0");
} else {
printf("1");
}
for (i = 0; i < DIM - temp; i++) {
printf("0");
}
do {
if (tempa % 2 == 0) {
printf("0");
} else {
printf("1");
}
tempa = tempa / 2;
} while (tempa != 0);
printf("n");
}
c arrays pointers input
c arrays pointers input
edited Nov 22 '18 at 17:59


chux
82k871148
82k871148
asked Nov 22 '18 at 17:21


Giacomo MasciarelliGiacomo Masciarelli
175
175
If the purpose ofread_new_int
is to read an integer value and set the argument to it, then you should probably go back to your text book or tutorial. C passes all arguments by value. That means when you call a function, the arguments are copied into the local argument variables inside the called function. Any modification to that local argument variable is done to that variable only. The original variable you use when you call the function will not change. You should return the value you read instead.
– Some programmer dude
Nov 22 '18 at 17:25
ok that's right. So i should change also the array of pointers to function as integer and all the functions that i must use in the menu as integer because read_new_int must return an integer, even if the other functions wont return any integer. thats right?
– Giacomo Masciarelli
Nov 22 '18 at 17:30
To keep it simple you could make all functions return anint
. The functions where the returned value doesn't matter just returns zero. And also make all function takeint
arguments, and if they don't use the argument just don't use it.
– Some programmer dude
Nov 22 '18 at 17:48
while (scanf("%d", &choice_option) != 1 && choice_option < 4 && choice_option >= 0) {
does not make as much sense aswhile (scanf("%d", &choice_option) != 1 || choice_option < 4 || choice_option >= 0) {
– chux
Nov 22 '18 at 18:02
I tried to fix this by filling the array with a filler function which does nothing, as first element of the array of pointers , so i could keep the functions as void. By the way i'm pretty sure that this isnt better than just making all the functions return an int. Right? Tho thanks for also pointing out the &&, i forgot about that
– Giacomo Masciarelli
Nov 22 '18 at 18:18
add a comment |
If the purpose ofread_new_int
is to read an integer value and set the argument to it, then you should probably go back to your text book or tutorial. C passes all arguments by value. That means when you call a function, the arguments are copied into the local argument variables inside the called function. Any modification to that local argument variable is done to that variable only. The original variable you use when you call the function will not change. You should return the value you read instead.
– Some programmer dude
Nov 22 '18 at 17:25
ok that's right. So i should change also the array of pointers to function as integer and all the functions that i must use in the menu as integer because read_new_int must return an integer, even if the other functions wont return any integer. thats right?
– Giacomo Masciarelli
Nov 22 '18 at 17:30
To keep it simple you could make all functions return anint
. The functions where the returned value doesn't matter just returns zero. And also make all function takeint
arguments, and if they don't use the argument just don't use it.
– Some programmer dude
Nov 22 '18 at 17:48
while (scanf("%d", &choice_option) != 1 && choice_option < 4 && choice_option >= 0) {
does not make as much sense aswhile (scanf("%d", &choice_option) != 1 || choice_option < 4 || choice_option >= 0) {
– chux
Nov 22 '18 at 18:02
I tried to fix this by filling the array with a filler function which does nothing, as first element of the array of pointers , so i could keep the functions as void. By the way i'm pretty sure that this isnt better than just making all the functions return an int. Right? Tho thanks for also pointing out the &&, i forgot about that
– Giacomo Masciarelli
Nov 22 '18 at 18:18
If the purpose of
read_new_int
is to read an integer value and set the argument to it, then you should probably go back to your text book or tutorial. C passes all arguments by value. That means when you call a function, the arguments are copied into the local argument variables inside the called function. Any modification to that local argument variable is done to that variable only. The original variable you use when you call the function will not change. You should return the value you read instead.– Some programmer dude
Nov 22 '18 at 17:25
If the purpose of
read_new_int
is to read an integer value and set the argument to it, then you should probably go back to your text book or tutorial. C passes all arguments by value. That means when you call a function, the arguments are copied into the local argument variables inside the called function. Any modification to that local argument variable is done to that variable only. The original variable you use when you call the function will not change. You should return the value you read instead.– Some programmer dude
Nov 22 '18 at 17:25
ok that's right. So i should change also the array of pointers to function as integer and all the functions that i must use in the menu as integer because read_new_int must return an integer, even if the other functions wont return any integer. thats right?
– Giacomo Masciarelli
Nov 22 '18 at 17:30
ok that's right. So i should change also the array of pointers to function as integer and all the functions that i must use in the menu as integer because read_new_int must return an integer, even if the other functions wont return any integer. thats right?
– Giacomo Masciarelli
Nov 22 '18 at 17:30
To keep it simple you could make all functions return an
int
. The functions where the returned value doesn't matter just returns zero. And also make all function take int
arguments, and if they don't use the argument just don't use it.– Some programmer dude
Nov 22 '18 at 17:48
To keep it simple you could make all functions return an
int
. The functions where the returned value doesn't matter just returns zero. And also make all function take int
arguments, and if they don't use the argument just don't use it.– Some programmer dude
Nov 22 '18 at 17:48
while (scanf("%d", &choice_option) != 1 && choice_option < 4 && choice_option >= 0) {
does not make as much sense as while (scanf("%d", &choice_option) != 1 || choice_option < 4 || choice_option >= 0) {
– chux
Nov 22 '18 at 18:02
while (scanf("%d", &choice_option) != 1 && choice_option < 4 && choice_option >= 0) {
does not make as much sense as while (scanf("%d", &choice_option) != 1 || choice_option < 4 || choice_option >= 0) {
– chux
Nov 22 '18 at 18:02
I tried to fix this by filling the array with a filler function which does nothing, as first element of the array of pointers , so i could keep the functions as void. By the way i'm pretty sure that this isnt better than just making all the functions return an int. Right? Tho thanks for also pointing out the &&, i forgot about that
– Giacomo Masciarelli
Nov 22 '18 at 18:18
I tried to fix this by filling the array with a filler function which does nothing, as first element of the array of pointers , so i could keep the functions as void. By the way i'm pretty sure that this isnt better than just making all the functions return an int. Right? Tho thanks for also pointing out the &&, i forgot about that
– Giacomo Masciarelli
Nov 22 '18 at 18:18
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435762%2fproblems-with-the-function-read-new-int%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435762%2fproblems-with-the-function-read-new-int%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BoCt,xJRA0sZO8 dzIq,Irk,jOaeYa hj0pOdZgZC1ROGb808eF5mQ7SLvABZ jxj,hlj77LeK,Ud,tAkz
If the purpose of
read_new_int
is to read an integer value and set the argument to it, then you should probably go back to your text book or tutorial. C passes all arguments by value. That means when you call a function, the arguments are copied into the local argument variables inside the called function. Any modification to that local argument variable is done to that variable only. The original variable you use when you call the function will not change. You should return the value you read instead.– Some programmer dude
Nov 22 '18 at 17:25
ok that's right. So i should change also the array of pointers to function as integer and all the functions that i must use in the menu as integer because read_new_int must return an integer, even if the other functions wont return any integer. thats right?
– Giacomo Masciarelli
Nov 22 '18 at 17:30
To keep it simple you could make all functions return an
int
. The functions where the returned value doesn't matter just returns zero. And also make all function takeint
arguments, and if they don't use the argument just don't use it.– Some programmer dude
Nov 22 '18 at 17:48
while (scanf("%d", &choice_option) != 1 && choice_option < 4 && choice_option >= 0) {
does not make as much sense aswhile (scanf("%d", &choice_option) != 1 || choice_option < 4 || choice_option >= 0) {
– chux
Nov 22 '18 at 18:02
I tried to fix this by filling the array with a filler function which does nothing, as first element of the array of pointers , so i could keep the functions as void. By the way i'm pretty sure that this isnt better than just making all the functions return an int. Right? Tho thanks for also pointing out the &&, i forgot about that
– Giacomo Masciarelli
Nov 22 '18 at 18:18