Querying the database in a Django unit test
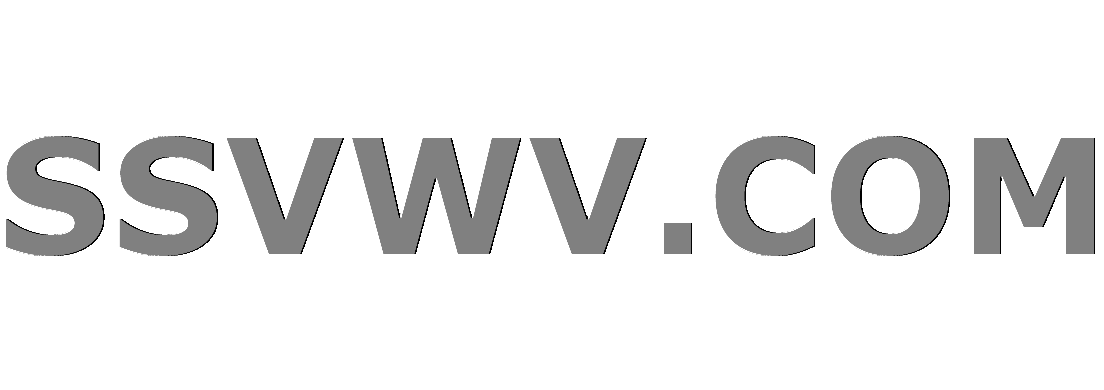
Multi tool use
up vote
1
down vote
favorite
I am creating a web application which has a POST
endpoint, that does two things:
- Saves the
POST
ed data (a university review) in the database. - Redirects the user to an overview page.
Here is the code for it:
if request.method == 'POST':
review = Review(university=university,
user=User.objects.get(pk=1),
summary=request.POST['summary'])
review.save()
return HttpResponseRedirect(reverse('university_overview', args=(university_id,)))
I haven't yet implemented passing the user data to the endpoint, and that's why I'm saving everything under the user with pk=1
.
My test is as follows:
class UniversityAddReviewTestCase(TestCase):
def setUp(self):
user = User.objects.create(username="username", password="password", email="email")
university = University.objects.create(name="Oxford University", country="UK", info="Meh", rating="9")
Review.objects.create(university=university, summary="Very nice", user_id=user.id)
Review.objects.create(university=university, summary="Very bad", user_id=user.id)
new_review = {
'summary': 'It was okay.'
}
self.response = Client().post('/%s/reviews/add' % university.id, new_review)
def test_database_updated(self):
self.assertEqual(len(Review.objects.all()), 3)
The result is this:
File ".../core/views.py", line 20, in detail
user=User.objects.get(pk=1),
File ".../ENV/lib/python3.6/site-packages/django/db/models/manager.py", line 82, in manager_method
return getattr(self.get_queryset(), name)(*args, **kwargs)
File ".../ENV/lib/python3.6/site-packages/django/db/models/query.py", line 403, in get
self.model._meta.object_name
django.contrib.auth.models.DoesNotExist: User matching query does not exist.
Why is this happening? I know the user I'm creating has a pk=1
, as when I actually print it during the test it's 1.
python django unit-testing
add a comment |
up vote
1
down vote
favorite
I am creating a web application which has a POST
endpoint, that does two things:
- Saves the
POST
ed data (a university review) in the database. - Redirects the user to an overview page.
Here is the code for it:
if request.method == 'POST':
review = Review(university=university,
user=User.objects.get(pk=1),
summary=request.POST['summary'])
review.save()
return HttpResponseRedirect(reverse('university_overview', args=(university_id,)))
I haven't yet implemented passing the user data to the endpoint, and that's why I'm saving everything under the user with pk=1
.
My test is as follows:
class UniversityAddReviewTestCase(TestCase):
def setUp(self):
user = User.objects.create(username="username", password="password", email="email")
university = University.objects.create(name="Oxford University", country="UK", info="Meh", rating="9")
Review.objects.create(university=university, summary="Very nice", user_id=user.id)
Review.objects.create(university=university, summary="Very bad", user_id=user.id)
new_review = {
'summary': 'It was okay.'
}
self.response = Client().post('/%s/reviews/add' % university.id, new_review)
def test_database_updated(self):
self.assertEqual(len(Review.objects.all()), 3)
The result is this:
File ".../core/views.py", line 20, in detail
user=User.objects.get(pk=1),
File ".../ENV/lib/python3.6/site-packages/django/db/models/manager.py", line 82, in manager_method
return getattr(self.get_queryset(), name)(*args, **kwargs)
File ".../ENV/lib/python3.6/site-packages/django/db/models/query.py", line 403, in get
self.model._meta.object_name
django.contrib.auth.models.DoesNotExist: User matching query does not exist.
Why is this happening? I know the user I'm creating has a pk=1
, as when I actually print it during the test it's 1.
python django unit-testing
is this the only place in your tests where you create a user?
– Henry Woody
Nov 19 at 23:39
no, I have other tests that create users as well
– pavlos163
Nov 19 at 23:49
it is however the only user in the specificTestCase
class
– pavlos163
Nov 19 at 23:50
oh and are there are test methods in thisTestCase
besidestest_database_updated
?
– Henry Woody
Nov 19 at 23:52
there is atest_html
method that only doesself.assertRedirects(self.response, "/4/overview/")
– pavlos163
Nov 20 at 0:08
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am creating a web application which has a POST
endpoint, that does two things:
- Saves the
POST
ed data (a university review) in the database. - Redirects the user to an overview page.
Here is the code for it:
if request.method == 'POST':
review = Review(university=university,
user=User.objects.get(pk=1),
summary=request.POST['summary'])
review.save()
return HttpResponseRedirect(reverse('university_overview', args=(university_id,)))
I haven't yet implemented passing the user data to the endpoint, and that's why I'm saving everything under the user with pk=1
.
My test is as follows:
class UniversityAddReviewTestCase(TestCase):
def setUp(self):
user = User.objects.create(username="username", password="password", email="email")
university = University.objects.create(name="Oxford University", country="UK", info="Meh", rating="9")
Review.objects.create(university=university, summary="Very nice", user_id=user.id)
Review.objects.create(university=university, summary="Very bad", user_id=user.id)
new_review = {
'summary': 'It was okay.'
}
self.response = Client().post('/%s/reviews/add' % university.id, new_review)
def test_database_updated(self):
self.assertEqual(len(Review.objects.all()), 3)
The result is this:
File ".../core/views.py", line 20, in detail
user=User.objects.get(pk=1),
File ".../ENV/lib/python3.6/site-packages/django/db/models/manager.py", line 82, in manager_method
return getattr(self.get_queryset(), name)(*args, **kwargs)
File ".../ENV/lib/python3.6/site-packages/django/db/models/query.py", line 403, in get
self.model._meta.object_name
django.contrib.auth.models.DoesNotExist: User matching query does not exist.
Why is this happening? I know the user I'm creating has a pk=1
, as when I actually print it during the test it's 1.
python django unit-testing
I am creating a web application which has a POST
endpoint, that does two things:
- Saves the
POST
ed data (a university review) in the database. - Redirects the user to an overview page.
Here is the code for it:
if request.method == 'POST':
review = Review(university=university,
user=User.objects.get(pk=1),
summary=request.POST['summary'])
review.save()
return HttpResponseRedirect(reverse('university_overview', args=(university_id,)))
I haven't yet implemented passing the user data to the endpoint, and that's why I'm saving everything under the user with pk=1
.
My test is as follows:
class UniversityAddReviewTestCase(TestCase):
def setUp(self):
user = User.objects.create(username="username", password="password", email="email")
university = University.objects.create(name="Oxford University", country="UK", info="Meh", rating="9")
Review.objects.create(university=university, summary="Very nice", user_id=user.id)
Review.objects.create(university=university, summary="Very bad", user_id=user.id)
new_review = {
'summary': 'It was okay.'
}
self.response = Client().post('/%s/reviews/add' % university.id, new_review)
def test_database_updated(self):
self.assertEqual(len(Review.objects.all()), 3)
The result is this:
File ".../core/views.py", line 20, in detail
user=User.objects.get(pk=1),
File ".../ENV/lib/python3.6/site-packages/django/db/models/manager.py", line 82, in manager_method
return getattr(self.get_queryset(), name)(*args, **kwargs)
File ".../ENV/lib/python3.6/site-packages/django/db/models/query.py", line 403, in get
self.model._meta.object_name
django.contrib.auth.models.DoesNotExist: User matching query does not exist.
Why is this happening? I know the user I'm creating has a pk=1
, as when I actually print it during the test it's 1.
python django unit-testing
python django unit-testing
asked Nov 19 at 22:08


pavlos163
510738
510738
is this the only place in your tests where you create a user?
– Henry Woody
Nov 19 at 23:39
no, I have other tests that create users as well
– pavlos163
Nov 19 at 23:49
it is however the only user in the specificTestCase
class
– pavlos163
Nov 19 at 23:50
oh and are there are test methods in thisTestCase
besidestest_database_updated
?
– Henry Woody
Nov 19 at 23:52
there is atest_html
method that only doesself.assertRedirects(self.response, "/4/overview/")
– pavlos163
Nov 20 at 0:08
add a comment |
is this the only place in your tests where you create a user?
– Henry Woody
Nov 19 at 23:39
no, I have other tests that create users as well
– pavlos163
Nov 19 at 23:49
it is however the only user in the specificTestCase
class
– pavlos163
Nov 19 at 23:50
oh and are there are test methods in thisTestCase
besidestest_database_updated
?
– Henry Woody
Nov 19 at 23:52
there is atest_html
method that only doesself.assertRedirects(self.response, "/4/overview/")
– pavlos163
Nov 20 at 0:08
is this the only place in your tests where you create a user?
– Henry Woody
Nov 19 at 23:39
is this the only place in your tests where you create a user?
– Henry Woody
Nov 19 at 23:39
no, I have other tests that create users as well
– pavlos163
Nov 19 at 23:49
no, I have other tests that create users as well
– pavlos163
Nov 19 at 23:49
it is however the only user in the specific
TestCase
class– pavlos163
Nov 19 at 23:50
it is however the only user in the specific
TestCase
class– pavlos163
Nov 19 at 23:50
oh and are there are test methods in this
TestCase
besides test_database_updated
?– Henry Woody
Nov 19 at 23:52
oh and are there are test methods in this
TestCase
besides test_database_updated
?– Henry Woody
Nov 19 at 23:52
there is a
test_html
method that only does self.assertRedirects(self.response, "/4/overview/")
– pavlos163
Nov 20 at 0:08
there is a
test_html
method that only does self.assertRedirects(self.response, "/4/overview/")
– pavlos163
Nov 20 at 0:08
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
pk
is defined by the database. Something could make it not equal to 1 in your test.
Try this in your setUp method
user = User.objects.create_user(
username="username",
password="password",
email="test@example.com",
id=1
)
assert user.pk == 1
That worked, thank you! Is there a convention around usingcreate_user
instead of justcreate
?
– pavlos163
Nov 20 at 0:11
create_user
is a method available to the User manager. It hashes the password and a few other actions. Creating a user with justcreate
would result in a user that cannot log in via standard login forms because the password saved in the database in not hashed. That was not the fix though. The fix was specifying id=1
– rikAtee
Nov 20 at 7:51
Yes, I realised, it was a curiosity question :D Thank you.
– pavlos163
Nov 20 at 19:10
add a comment |
up vote
0
down vote
Take advantage of using self
and then you can try this instead:
class UniversityAddReviewTestCase(TestCase):
def setUp(self):
self.user = User.objects.create(
username="username",
password="password",
email="email")
....
and then
if request.method == 'POST':
review = Review(
university=university,
user=self.user,
summary=request.POST['summary'])
review.save()
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
pk
is defined by the database. Something could make it not equal to 1 in your test.
Try this in your setUp method
user = User.objects.create_user(
username="username",
password="password",
email="test@example.com",
id=1
)
assert user.pk == 1
That worked, thank you! Is there a convention around usingcreate_user
instead of justcreate
?
– pavlos163
Nov 20 at 0:11
create_user
is a method available to the User manager. It hashes the password and a few other actions. Creating a user with justcreate
would result in a user that cannot log in via standard login forms because the password saved in the database in not hashed. That was not the fix though. The fix was specifying id=1
– rikAtee
Nov 20 at 7:51
Yes, I realised, it was a curiosity question :D Thank you.
– pavlos163
Nov 20 at 19:10
add a comment |
up vote
1
down vote
accepted
pk
is defined by the database. Something could make it not equal to 1 in your test.
Try this in your setUp method
user = User.objects.create_user(
username="username",
password="password",
email="test@example.com",
id=1
)
assert user.pk == 1
That worked, thank you! Is there a convention around usingcreate_user
instead of justcreate
?
– pavlos163
Nov 20 at 0:11
create_user
is a method available to the User manager. It hashes the password and a few other actions. Creating a user with justcreate
would result in a user that cannot log in via standard login forms because the password saved in the database in not hashed. That was not the fix though. The fix was specifying id=1
– rikAtee
Nov 20 at 7:51
Yes, I realised, it was a curiosity question :D Thank you.
– pavlos163
Nov 20 at 19:10
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
pk
is defined by the database. Something could make it not equal to 1 in your test.
Try this in your setUp method
user = User.objects.create_user(
username="username",
password="password",
email="test@example.com",
id=1
)
assert user.pk == 1
pk
is defined by the database. Something could make it not equal to 1 in your test.
Try this in your setUp method
user = User.objects.create_user(
username="username",
password="password",
email="test@example.com",
id=1
)
assert user.pk == 1
answered Nov 19 at 22:45
rikAtee
4,68542956
4,68542956
That worked, thank you! Is there a convention around usingcreate_user
instead of justcreate
?
– pavlos163
Nov 20 at 0:11
create_user
is a method available to the User manager. It hashes the password and a few other actions. Creating a user with justcreate
would result in a user that cannot log in via standard login forms because the password saved in the database in not hashed. That was not the fix though. The fix was specifying id=1
– rikAtee
Nov 20 at 7:51
Yes, I realised, it was a curiosity question :D Thank you.
– pavlos163
Nov 20 at 19:10
add a comment |
That worked, thank you! Is there a convention around usingcreate_user
instead of justcreate
?
– pavlos163
Nov 20 at 0:11
create_user
is a method available to the User manager. It hashes the password and a few other actions. Creating a user with justcreate
would result in a user that cannot log in via standard login forms because the password saved in the database in not hashed. That was not the fix though. The fix was specifying id=1
– rikAtee
Nov 20 at 7:51
Yes, I realised, it was a curiosity question :D Thank you.
– pavlos163
Nov 20 at 19:10
That worked, thank you! Is there a convention around using
create_user
instead of just create
?– pavlos163
Nov 20 at 0:11
That worked, thank you! Is there a convention around using
create_user
instead of just create
?– pavlos163
Nov 20 at 0:11
create_user
is a method available to the User manager. It hashes the password and a few other actions. Creating a user with just create
would result in a user that cannot log in via standard login forms because the password saved in the database in not hashed. That was not the fix though. The fix was specifying id=1– rikAtee
Nov 20 at 7:51
create_user
is a method available to the User manager. It hashes the password and a few other actions. Creating a user with just create
would result in a user that cannot log in via standard login forms because the password saved in the database in not hashed. That was not the fix though. The fix was specifying id=1– rikAtee
Nov 20 at 7:51
Yes, I realised, it was a curiosity question :D Thank you.
– pavlos163
Nov 20 at 19:10
Yes, I realised, it was a curiosity question :D Thank you.
– pavlos163
Nov 20 at 19:10
add a comment |
up vote
0
down vote
Take advantage of using self
and then you can try this instead:
class UniversityAddReviewTestCase(TestCase):
def setUp(self):
self.user = User.objects.create(
username="username",
password="password",
email="email")
....
and then
if request.method == 'POST':
review = Review(
university=university,
user=self.user,
summary=request.POST['summary'])
review.save()
add a comment |
up vote
0
down vote
Take advantage of using self
and then you can try this instead:
class UniversityAddReviewTestCase(TestCase):
def setUp(self):
self.user = User.objects.create(
username="username",
password="password",
email="email")
....
and then
if request.method == 'POST':
review = Review(
university=university,
user=self.user,
summary=request.POST['summary'])
review.save()
add a comment |
up vote
0
down vote
up vote
0
down vote
Take advantage of using self
and then you can try this instead:
class UniversityAddReviewTestCase(TestCase):
def setUp(self):
self.user = User.objects.create(
username="username",
password="password",
email="email")
....
and then
if request.method == 'POST':
review = Review(
university=university,
user=self.user,
summary=request.POST['summary'])
review.save()
Take advantage of using self
and then you can try this instead:
class UniversityAddReviewTestCase(TestCase):
def setUp(self):
self.user = User.objects.create(
username="username",
password="password",
email="email")
....
and then
if request.method == 'POST':
review = Review(
university=university,
user=self.user,
summary=request.POST['summary'])
review.save()
answered Nov 19 at 23:40


Marcelo Duarte Fernandes
111
111
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53383346%2fquerying-the-database-in-a-django-unit-test%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1zABHxgiRomngMg,t2MzZIM1nc1FkgnRjBj6Te7hmpSFNTJbRsggIoe6Kzt,2SRRr xjNAzBnh,LL,IsC
is this the only place in your tests where you create a user?
– Henry Woody
Nov 19 at 23:39
no, I have other tests that create users as well
– pavlos163
Nov 19 at 23:49
it is however the only user in the specific
TestCase
class– pavlos163
Nov 19 at 23:50
oh and are there are test methods in this
TestCase
besidestest_database_updated
?– Henry Woody
Nov 19 at 23:52
there is a
test_html
method that only doesself.assertRedirects(self.response, "/4/overview/")
– pavlos163
Nov 20 at 0:08