Screen recorder producing huge AVI files
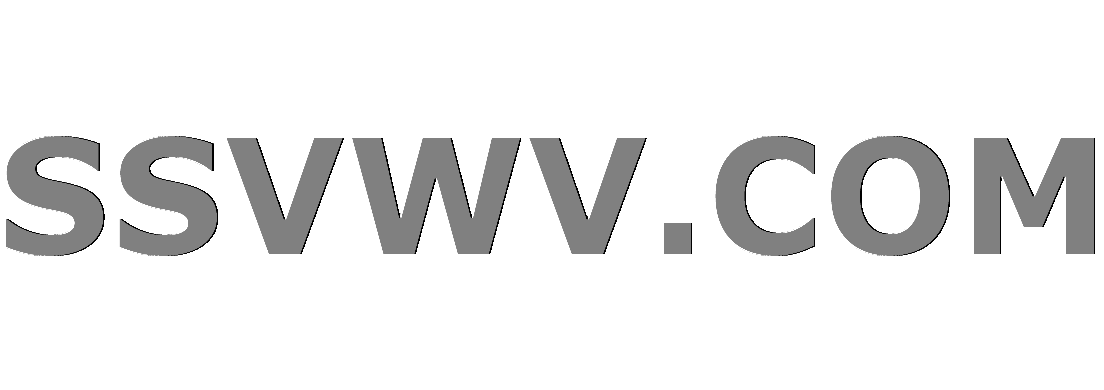
Multi tool use
up vote
3
down vote
favorite
I'm currently trying to make a screen recorder in C#, and so far it works but the problem is that something as simple as a 20second video will take about 1GB of space. I have it setup so a timer continuously takes screenshots with this method:
void takeScreenshot()
{
Rectangle bounds = Screen.FromControl(this).Bounds;
using (Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
{
using (Graphics g = Graphics.FromImage(bitmap))
{
//Add screen to bitmap:
g.CopyFromScreen(new Point(bounds.Left, bounds.Top), Point.Empty, bounds.Size);
}
//Create and save screenshot:
string name = path + "//screenshot-" + fileCount + ".jpeg";
bitmap.Save(name, ImageFormat.Jpeg);
inputImageSequence.Add(name);
fileCount++;
//Dispose of bitmap:
bitmap.Dispose();
}
}
And then it stores those pictures in a temporary folder in the D://
drive, and then when it's done it takes all the pictures and creates an AVI video out of them like this:
//Set bounds of video to screen size:
Rectangle bounds = Screen.FromControl(this).Bounds;
int width = bounds.Width;
int height = bounds.Height;
var framRate = 5;
using (var vFWriter = new VideoFileWriter())
{
//Create new video file:
vFWriter.Open(outputPath+"//video.avi", width, height, framRate, VideoCodec.Raw);
//Make each screenshot into a video frame:
foreach (var imageLocation in inputImageSequence)
{
Bitmap imageFrame = System.Drawing.Image.FromFile(imageLocation) as Bitmap;
vFWriter.WriteVideoFrame(imageFrame);
imageFrame.Dispose();
}
vFWriter.Close();
}
//Delete the screenshots and temporary folder:
DeletePath(path);
Any help on reducing the inefficiency of this is appreciated, I'm fairly new to this kind of programming.
c# compression video
New contributor
BenCompSci is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
3
down vote
favorite
I'm currently trying to make a screen recorder in C#, and so far it works but the problem is that something as simple as a 20second video will take about 1GB of space. I have it setup so a timer continuously takes screenshots with this method:
void takeScreenshot()
{
Rectangle bounds = Screen.FromControl(this).Bounds;
using (Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
{
using (Graphics g = Graphics.FromImage(bitmap))
{
//Add screen to bitmap:
g.CopyFromScreen(new Point(bounds.Left, bounds.Top), Point.Empty, bounds.Size);
}
//Create and save screenshot:
string name = path + "//screenshot-" + fileCount + ".jpeg";
bitmap.Save(name, ImageFormat.Jpeg);
inputImageSequence.Add(name);
fileCount++;
//Dispose of bitmap:
bitmap.Dispose();
}
}
And then it stores those pictures in a temporary folder in the D://
drive, and then when it's done it takes all the pictures and creates an AVI video out of them like this:
//Set bounds of video to screen size:
Rectangle bounds = Screen.FromControl(this).Bounds;
int width = bounds.Width;
int height = bounds.Height;
var framRate = 5;
using (var vFWriter = new VideoFileWriter())
{
//Create new video file:
vFWriter.Open(outputPath+"//video.avi", width, height, framRate, VideoCodec.Raw);
//Make each screenshot into a video frame:
foreach (var imageLocation in inputImageSequence)
{
Bitmap imageFrame = System.Drawing.Image.FromFile(imageLocation) as Bitmap;
vFWriter.WriteVideoFrame(imageFrame);
imageFrame.Dispose();
}
vFWriter.Close();
}
//Delete the screenshots and temporary folder:
DeletePath(path);
Any help on reducing the inefficiency of this is appreciated, I'm fairly new to this kind of programming.
c# compression video
New contributor
BenCompSci is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I'm currently trying to make a screen recorder in C#, and so far it works but the problem is that something as simple as a 20second video will take about 1GB of space. I have it setup so a timer continuously takes screenshots with this method:
void takeScreenshot()
{
Rectangle bounds = Screen.FromControl(this).Bounds;
using (Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
{
using (Graphics g = Graphics.FromImage(bitmap))
{
//Add screen to bitmap:
g.CopyFromScreen(new Point(bounds.Left, bounds.Top), Point.Empty, bounds.Size);
}
//Create and save screenshot:
string name = path + "//screenshot-" + fileCount + ".jpeg";
bitmap.Save(name, ImageFormat.Jpeg);
inputImageSequence.Add(name);
fileCount++;
//Dispose of bitmap:
bitmap.Dispose();
}
}
And then it stores those pictures in a temporary folder in the D://
drive, and then when it's done it takes all the pictures and creates an AVI video out of them like this:
//Set bounds of video to screen size:
Rectangle bounds = Screen.FromControl(this).Bounds;
int width = bounds.Width;
int height = bounds.Height;
var framRate = 5;
using (var vFWriter = new VideoFileWriter())
{
//Create new video file:
vFWriter.Open(outputPath+"//video.avi", width, height, framRate, VideoCodec.Raw);
//Make each screenshot into a video frame:
foreach (var imageLocation in inputImageSequence)
{
Bitmap imageFrame = System.Drawing.Image.FromFile(imageLocation) as Bitmap;
vFWriter.WriteVideoFrame(imageFrame);
imageFrame.Dispose();
}
vFWriter.Close();
}
//Delete the screenshots and temporary folder:
DeletePath(path);
Any help on reducing the inefficiency of this is appreciated, I'm fairly new to this kind of programming.
c# compression video
New contributor
BenCompSci is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I'm currently trying to make a screen recorder in C#, and so far it works but the problem is that something as simple as a 20second video will take about 1GB of space. I have it setup so a timer continuously takes screenshots with this method:
void takeScreenshot()
{
Rectangle bounds = Screen.FromControl(this).Bounds;
using (Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
{
using (Graphics g = Graphics.FromImage(bitmap))
{
//Add screen to bitmap:
g.CopyFromScreen(new Point(bounds.Left, bounds.Top), Point.Empty, bounds.Size);
}
//Create and save screenshot:
string name = path + "//screenshot-" + fileCount + ".jpeg";
bitmap.Save(name, ImageFormat.Jpeg);
inputImageSequence.Add(name);
fileCount++;
//Dispose of bitmap:
bitmap.Dispose();
}
}
And then it stores those pictures in a temporary folder in the D://
drive, and then when it's done it takes all the pictures and creates an AVI video out of them like this:
//Set bounds of video to screen size:
Rectangle bounds = Screen.FromControl(this).Bounds;
int width = bounds.Width;
int height = bounds.Height;
var framRate = 5;
using (var vFWriter = new VideoFileWriter())
{
//Create new video file:
vFWriter.Open(outputPath+"//video.avi", width, height, framRate, VideoCodec.Raw);
//Make each screenshot into a video frame:
foreach (var imageLocation in inputImageSequence)
{
Bitmap imageFrame = System.Drawing.Image.FromFile(imageLocation) as Bitmap;
vFWriter.WriteVideoFrame(imageFrame);
imageFrame.Dispose();
}
vFWriter.Close();
}
//Delete the screenshots and temporary folder:
DeletePath(path);
Any help on reducing the inefficiency of this is appreciated, I'm fairly new to this kind of programming.
c# compression video
c# compression video
New contributor
BenCompSci is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
BenCompSci is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 17 mins ago


t3chb0t
33.7k745108
33.7k745108
New contributor
BenCompSci is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 4 hours ago


BenCompSci
162
162
New contributor
BenCompSci is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
BenCompSci is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
BenCompSci is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
takeScreenshot()
- Based on the .NET Naming Guidelines methods should be named using
PascalCase
casingtakeScreenshot()
=>TakeScreenshot()
- You are enclosing the usage of the
Bitmap
inside ausing
statement which is the way to go but callingDispose()
on thatBitmap
is superflous because that is what ausing
statement is doing.
If you have two
using
statements without any code between you can stack them which saves one level of indentation like so (already removed theDispose()
)
using (Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
using (Graphics g = Graphics.FromImage(bitmap))
{
//Add screen to bitmap:
g.CopyFromScreen(new Point(bounds.Left, bounds.Top), Point.Empty, bounds.Size);
//Create and save screenshot:
string name = path + "//screenshot-" + fileCount + ".jpeg";
bitmap.Save(name, ImageFormat.Jpeg);
inputImageSequence.Add(name);
fileCount++;
}
- Comments should explain why something is done in the way it is done. Let the code itself tell what is done by using meaningful named things. In this way your comments won't lie like e.g
//Create and save screenshot:
which isn't what that code does. Saving yes but no creating of a screenshot is taking place.
Creating the video
- Try to be consitent. Here you create a
Bitmap
and callDispose()
instead of using ausing
statement. In addition, sometime you usevar
and sometimes you use the concrete type althought the type is seen at first glance. - If you are using the
as
operator you should always add anull
check for that object because anas
operator won't throw an exception but the resulting object may benull
which will trigger an exception soewhere else.
Implementing these points will look like so
Rectangle bounds = Screen.FromControl(this).Bounds;
var width = bounds.Width;
var height = bounds.Height;
var framRate = 5;
using (var vFWriter = new VideoFileWriter())
{
vFWriter.Open(outputPath+"//video.avi", width, height, framRate, VideoCodec.Raw);
foreach (var imageLocation in inputImageSequence)
{
using(var imageFrame = (Bitmap)System.Drawing.Image.FromFile(imageLocation))
{
vFWriter.WriteVideoFrame(imageFrame);
}
}
vFWriter.Close();
}
DeletePath(path);
My guess about the big file size is that you are using the VideoCodec.Raw
. Try to change it to some other codec and see if this helps.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
takeScreenshot()
- Based on the .NET Naming Guidelines methods should be named using
PascalCase
casingtakeScreenshot()
=>TakeScreenshot()
- You are enclosing the usage of the
Bitmap
inside ausing
statement which is the way to go but callingDispose()
on thatBitmap
is superflous because that is what ausing
statement is doing.
If you have two
using
statements without any code between you can stack them which saves one level of indentation like so (already removed theDispose()
)
using (Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
using (Graphics g = Graphics.FromImage(bitmap))
{
//Add screen to bitmap:
g.CopyFromScreen(new Point(bounds.Left, bounds.Top), Point.Empty, bounds.Size);
//Create and save screenshot:
string name = path + "//screenshot-" + fileCount + ".jpeg";
bitmap.Save(name, ImageFormat.Jpeg);
inputImageSequence.Add(name);
fileCount++;
}
- Comments should explain why something is done in the way it is done. Let the code itself tell what is done by using meaningful named things. In this way your comments won't lie like e.g
//Create and save screenshot:
which isn't what that code does. Saving yes but no creating of a screenshot is taking place.
Creating the video
- Try to be consitent. Here you create a
Bitmap
and callDispose()
instead of using ausing
statement. In addition, sometime you usevar
and sometimes you use the concrete type althought the type is seen at first glance. - If you are using the
as
operator you should always add anull
check for that object because anas
operator won't throw an exception but the resulting object may benull
which will trigger an exception soewhere else.
Implementing these points will look like so
Rectangle bounds = Screen.FromControl(this).Bounds;
var width = bounds.Width;
var height = bounds.Height;
var framRate = 5;
using (var vFWriter = new VideoFileWriter())
{
vFWriter.Open(outputPath+"//video.avi", width, height, framRate, VideoCodec.Raw);
foreach (var imageLocation in inputImageSequence)
{
using(var imageFrame = (Bitmap)System.Drawing.Image.FromFile(imageLocation))
{
vFWriter.WriteVideoFrame(imageFrame);
}
}
vFWriter.Close();
}
DeletePath(path);
My guess about the big file size is that you are using the VideoCodec.Raw
. Try to change it to some other codec and see if this helps.
add a comment |
up vote
2
down vote
takeScreenshot()
- Based on the .NET Naming Guidelines methods should be named using
PascalCase
casingtakeScreenshot()
=>TakeScreenshot()
- You are enclosing the usage of the
Bitmap
inside ausing
statement which is the way to go but callingDispose()
on thatBitmap
is superflous because that is what ausing
statement is doing.
If you have two
using
statements without any code between you can stack them which saves one level of indentation like so (already removed theDispose()
)
using (Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
using (Graphics g = Graphics.FromImage(bitmap))
{
//Add screen to bitmap:
g.CopyFromScreen(new Point(bounds.Left, bounds.Top), Point.Empty, bounds.Size);
//Create and save screenshot:
string name = path + "//screenshot-" + fileCount + ".jpeg";
bitmap.Save(name, ImageFormat.Jpeg);
inputImageSequence.Add(name);
fileCount++;
}
- Comments should explain why something is done in the way it is done. Let the code itself tell what is done by using meaningful named things. In this way your comments won't lie like e.g
//Create and save screenshot:
which isn't what that code does. Saving yes but no creating of a screenshot is taking place.
Creating the video
- Try to be consitent. Here you create a
Bitmap
and callDispose()
instead of using ausing
statement. In addition, sometime you usevar
and sometimes you use the concrete type althought the type is seen at first glance. - If you are using the
as
operator you should always add anull
check for that object because anas
operator won't throw an exception but the resulting object may benull
which will trigger an exception soewhere else.
Implementing these points will look like so
Rectangle bounds = Screen.FromControl(this).Bounds;
var width = bounds.Width;
var height = bounds.Height;
var framRate = 5;
using (var vFWriter = new VideoFileWriter())
{
vFWriter.Open(outputPath+"//video.avi", width, height, framRate, VideoCodec.Raw);
foreach (var imageLocation in inputImageSequence)
{
using(var imageFrame = (Bitmap)System.Drawing.Image.FromFile(imageLocation))
{
vFWriter.WriteVideoFrame(imageFrame);
}
}
vFWriter.Close();
}
DeletePath(path);
My guess about the big file size is that you are using the VideoCodec.Raw
. Try to change it to some other codec and see if this helps.
add a comment |
up vote
2
down vote
up vote
2
down vote
takeScreenshot()
- Based on the .NET Naming Guidelines methods should be named using
PascalCase
casingtakeScreenshot()
=>TakeScreenshot()
- You are enclosing the usage of the
Bitmap
inside ausing
statement which is the way to go but callingDispose()
on thatBitmap
is superflous because that is what ausing
statement is doing.
If you have two
using
statements without any code between you can stack them which saves one level of indentation like so (already removed theDispose()
)
using (Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
using (Graphics g = Graphics.FromImage(bitmap))
{
//Add screen to bitmap:
g.CopyFromScreen(new Point(bounds.Left, bounds.Top), Point.Empty, bounds.Size);
//Create and save screenshot:
string name = path + "//screenshot-" + fileCount + ".jpeg";
bitmap.Save(name, ImageFormat.Jpeg);
inputImageSequence.Add(name);
fileCount++;
}
- Comments should explain why something is done in the way it is done. Let the code itself tell what is done by using meaningful named things. In this way your comments won't lie like e.g
//Create and save screenshot:
which isn't what that code does. Saving yes but no creating of a screenshot is taking place.
Creating the video
- Try to be consitent. Here you create a
Bitmap
and callDispose()
instead of using ausing
statement. In addition, sometime you usevar
and sometimes you use the concrete type althought the type is seen at first glance. - If you are using the
as
operator you should always add anull
check for that object because anas
operator won't throw an exception but the resulting object may benull
which will trigger an exception soewhere else.
Implementing these points will look like so
Rectangle bounds = Screen.FromControl(this).Bounds;
var width = bounds.Width;
var height = bounds.Height;
var framRate = 5;
using (var vFWriter = new VideoFileWriter())
{
vFWriter.Open(outputPath+"//video.avi", width, height, framRate, VideoCodec.Raw);
foreach (var imageLocation in inputImageSequence)
{
using(var imageFrame = (Bitmap)System.Drawing.Image.FromFile(imageLocation))
{
vFWriter.WriteVideoFrame(imageFrame);
}
}
vFWriter.Close();
}
DeletePath(path);
My guess about the big file size is that you are using the VideoCodec.Raw
. Try to change it to some other codec and see if this helps.
takeScreenshot()
- Based on the .NET Naming Guidelines methods should be named using
PascalCase
casingtakeScreenshot()
=>TakeScreenshot()
- You are enclosing the usage of the
Bitmap
inside ausing
statement which is the way to go but callingDispose()
on thatBitmap
is superflous because that is what ausing
statement is doing.
If you have two
using
statements without any code between you can stack them which saves one level of indentation like so (already removed theDispose()
)
using (Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
using (Graphics g = Graphics.FromImage(bitmap))
{
//Add screen to bitmap:
g.CopyFromScreen(new Point(bounds.Left, bounds.Top), Point.Empty, bounds.Size);
//Create and save screenshot:
string name = path + "//screenshot-" + fileCount + ".jpeg";
bitmap.Save(name, ImageFormat.Jpeg);
inputImageSequence.Add(name);
fileCount++;
}
- Comments should explain why something is done in the way it is done. Let the code itself tell what is done by using meaningful named things. In this way your comments won't lie like e.g
//Create and save screenshot:
which isn't what that code does. Saving yes but no creating of a screenshot is taking place.
Creating the video
- Try to be consitent. Here you create a
Bitmap
and callDispose()
instead of using ausing
statement. In addition, sometime you usevar
and sometimes you use the concrete type althought the type is seen at first glance. - If you are using the
as
operator you should always add anull
check for that object because anas
operator won't throw an exception but the resulting object may benull
which will trigger an exception soewhere else.
Implementing these points will look like so
Rectangle bounds = Screen.FromControl(this).Bounds;
var width = bounds.Width;
var height = bounds.Height;
var framRate = 5;
using (var vFWriter = new VideoFileWriter())
{
vFWriter.Open(outputPath+"//video.avi", width, height, framRate, VideoCodec.Raw);
foreach (var imageLocation in inputImageSequence)
{
using(var imageFrame = (Bitmap)System.Drawing.Image.FromFile(imageLocation))
{
vFWriter.WriteVideoFrame(imageFrame);
}
}
vFWriter.Close();
}
DeletePath(path);
My guess about the big file size is that you are using the VideoCodec.Raw
. Try to change it to some other codec and see if this helps.
answered 1 hour ago


Heslacher
44.6k460154
44.6k460154
add a comment |
add a comment |
BenCompSci is a new contributor. Be nice, and check out our Code of Conduct.
BenCompSci is a new contributor. Be nice, and check out our Code of Conduct.
BenCompSci is a new contributor. Be nice, and check out our Code of Conduct.
BenCompSci is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f208582%2fscreen-recorder-producing-huge-avi-files%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FvSpEaiuyZdnSdR3e dV,GZUV20l CuC10 ILFbzNjeru,6ePT,G6iRav8yypTmuFO0p9gTYFcsIY6AQ8cjLBXcOqIF,YBfL1