How to customize individual text in Flutter TextFields or TextFormFields in flutter
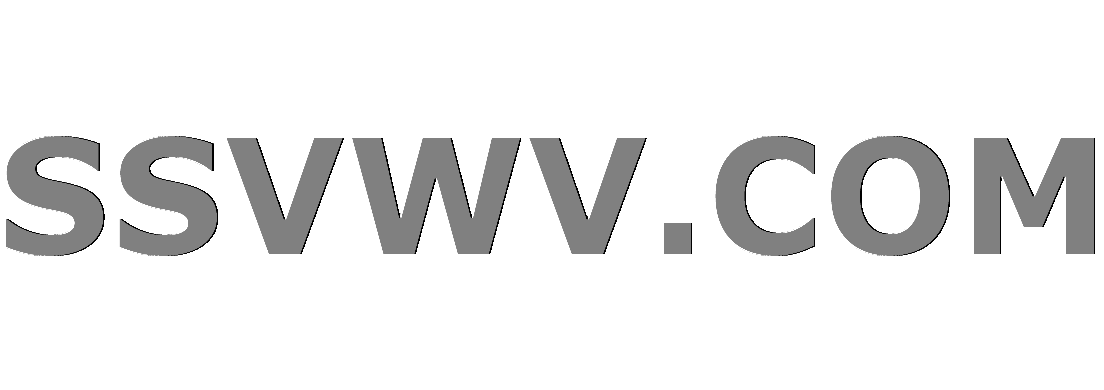
Multi tool use
up vote
-1
down vote
favorite
I'm trying to style text as the user types into the TextField or TextFormField. For example links get underlined or emails get highlighted. Is there a way to do this? This is the approach I took, but I am having trouble formatting the string as shown in the code
class _MyCustomFormState extends State<MyCustomForm> {
final myController = TextEditingController();
@override
void initState() {
super.initState();
myController.addListener(_textListener);
}
_textListener(){
//Checks if the new string typed is an email and highlights it
List<String> _stringList = _controller.text.split(" ");
String _lastString = _stringList.last;
if(_isEmail(_lastString)){
//Format _lastString (this is the problem)
}
//Remove the last string and add the formatted version
_stringList.removeLast();
_stringList.add(_lastString);
_controller.text = _stringList.join(" ");
}
Widget _textField(){
return TextFormField(
keyboardType: TextInputType.multiline,
maxLines: null,
controller: _controller,);
}
}

add a comment |
up vote
-1
down vote
favorite
I'm trying to style text as the user types into the TextField or TextFormField. For example links get underlined or emails get highlighted. Is there a way to do this? This is the approach I took, but I am having trouble formatting the string as shown in the code
class _MyCustomFormState extends State<MyCustomForm> {
final myController = TextEditingController();
@override
void initState() {
super.initState();
myController.addListener(_textListener);
}
_textListener(){
//Checks if the new string typed is an email and highlights it
List<String> _stringList = _controller.text.split(" ");
String _lastString = _stringList.last;
if(_isEmail(_lastString)){
//Format _lastString (this is the problem)
}
//Remove the last string and add the formatted version
_stringList.removeLast();
_stringList.add(_lastString);
_controller.text = _stringList.join(" ");
}
Widget _textField(){
return TextFormField(
keyboardType: TextInputType.multiline,
maxLines: null,
controller: _controller,);
}
}

Please specify what you have tried and if possible paste your code for reference.
– Arnold Parge
Nov 19 at 16:07
For underlined links you can useTextStyle
. For highlightning you can useRichText
, if I understood you right
– Andrey Turkovsky
Nov 19 at 16:34
@ArnoldParge I have attached the approach I am using.
– T.Abs
Nov 20 at 20:40
@AndreyTurkovsky that would work if I was assigning a style to the widget. However I am only trying to format one word in a string as shown in the sample code (just attached)
– T.Abs
Nov 20 at 20:41
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I'm trying to style text as the user types into the TextField or TextFormField. For example links get underlined or emails get highlighted. Is there a way to do this? This is the approach I took, but I am having trouble formatting the string as shown in the code
class _MyCustomFormState extends State<MyCustomForm> {
final myController = TextEditingController();
@override
void initState() {
super.initState();
myController.addListener(_textListener);
}
_textListener(){
//Checks if the new string typed is an email and highlights it
List<String> _stringList = _controller.text.split(" ");
String _lastString = _stringList.last;
if(_isEmail(_lastString)){
//Format _lastString (this is the problem)
}
//Remove the last string and add the formatted version
_stringList.removeLast();
_stringList.add(_lastString);
_controller.text = _stringList.join(" ");
}
Widget _textField(){
return TextFormField(
keyboardType: TextInputType.multiline,
maxLines: null,
controller: _controller,);
}
}

I'm trying to style text as the user types into the TextField or TextFormField. For example links get underlined or emails get highlighted. Is there a way to do this? This is the approach I took, but I am having trouble formatting the string as shown in the code
class _MyCustomFormState extends State<MyCustomForm> {
final myController = TextEditingController();
@override
void initState() {
super.initState();
myController.addListener(_textListener);
}
_textListener(){
//Checks if the new string typed is an email and highlights it
List<String> _stringList = _controller.text.split(" ");
String _lastString = _stringList.last;
if(_isEmail(_lastString)){
//Format _lastString (this is the problem)
}
//Remove the last string and add the formatted version
_stringList.removeLast();
_stringList.add(_lastString);
_controller.text = _stringList.join(" ");
}
Widget _textField(){
return TextFormField(
keyboardType: TextInputType.multiline,
maxLines: null,
controller: _controller,);
}
}


edited Nov 24 at 22:22
asked Nov 19 at 16:04
T.Abs
11
11
Please specify what you have tried and if possible paste your code for reference.
– Arnold Parge
Nov 19 at 16:07
For underlined links you can useTextStyle
. For highlightning you can useRichText
, if I understood you right
– Andrey Turkovsky
Nov 19 at 16:34
@ArnoldParge I have attached the approach I am using.
– T.Abs
Nov 20 at 20:40
@AndreyTurkovsky that would work if I was assigning a style to the widget. However I am only trying to format one word in a string as shown in the sample code (just attached)
– T.Abs
Nov 20 at 20:41
add a comment |
Please specify what you have tried and if possible paste your code for reference.
– Arnold Parge
Nov 19 at 16:07
For underlined links you can useTextStyle
. For highlightning you can useRichText
, if I understood you right
– Andrey Turkovsky
Nov 19 at 16:34
@ArnoldParge I have attached the approach I am using.
– T.Abs
Nov 20 at 20:40
@AndreyTurkovsky that would work if I was assigning a style to the widget. However I am only trying to format one word in a string as shown in the sample code (just attached)
– T.Abs
Nov 20 at 20:41
Please specify what you have tried and if possible paste your code for reference.
– Arnold Parge
Nov 19 at 16:07
Please specify what you have tried and if possible paste your code for reference.
– Arnold Parge
Nov 19 at 16:07
For underlined links you can use
TextStyle
. For highlightning you can use RichText
, if I understood you right– Andrey Turkovsky
Nov 19 at 16:34
For underlined links you can use
TextStyle
. For highlightning you can use RichText
, if I understood you right– Andrey Turkovsky
Nov 19 at 16:34
@ArnoldParge I have attached the approach I am using.
– T.Abs
Nov 20 at 20:40
@ArnoldParge I have attached the approach I am using.
– T.Abs
Nov 20 at 20:40
@AndreyTurkovsky that would work if I was assigning a style to the widget. However I am only trying to format one word in a string as shown in the sample code (just attached)
– T.Abs
Nov 20 at 20:41
@AndreyTurkovsky that would work if I was assigning a style to the widget. However I am only trying to format one word in a string as shown in the sample code (just attached)
– T.Abs
Nov 20 at 20:41
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378523%2fhow-to-customize-individual-text-in-flutter-textfields-or-textformfields-in-flut%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
camcy 1ElTwY,DBCwIk0wZZH Knj,tfyBQL4AUbSe,yzYywvLjLRHEtJh J,XwPL,GREGnO3237urQXzrBog8E N8FT33rd Q,tMN Klrr9X E
Please specify what you have tried and if possible paste your code for reference.
– Arnold Parge
Nov 19 at 16:07
For underlined links you can use
TextStyle
. For highlightning you can useRichText
, if I understood you right– Andrey Turkovsky
Nov 19 at 16:34
@ArnoldParge I have attached the approach I am using.
– T.Abs
Nov 20 at 20:40
@AndreyTurkovsky that would work if I was assigning a style to the widget. However I am only trying to format one word in a string as shown in the sample code (just attached)
– T.Abs
Nov 20 at 20:41