Android - NetworkInterface vs WifiManager
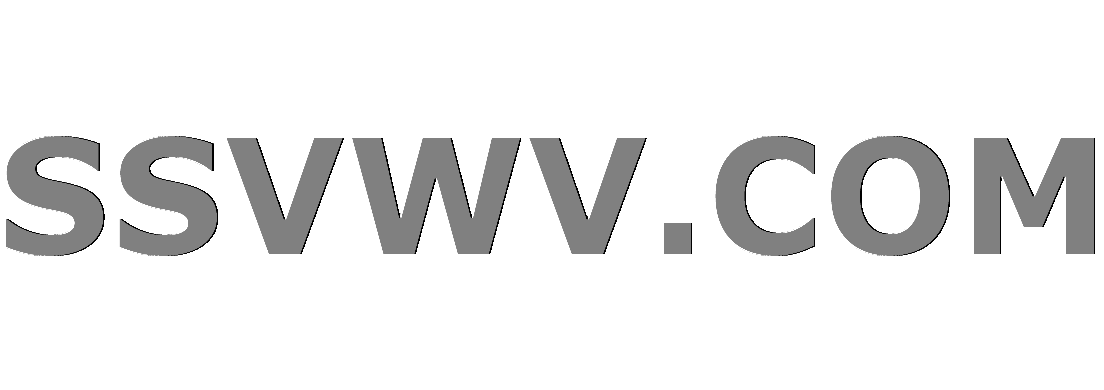
Multi tool use
up vote
0
down vote
favorite
I am trying to show the ip address of of connected network(WiFi/Ethernet). I am confusing by two ip address. I can see two approaches. Get IP using WiFi info and Get IP using NetworkInterface. But I am getting two different IP's. Which one I need to use. Please let me know.
Using NetworkInterface:
private void getIpUsingNetworkInterface() {
String ipAddress = null;
try {
for (Enumeration<NetworkInterface> en = NetworkInterface.getNetworkInterfaces(); en.hasMoreElements(); ) {
NetworkInterface intf = en.nextElement();
for (Enumeration<InetAddress> enumIpAddr = intf.getInetAddresses(); enumIpAddr.hasMoreElements(); ) {
InetAddress inetAddress = enumIpAddr.nextElement();
if (!inetAddress.isLoopbackAddress()) {
ipAddress = inetAddress.getHostAddress().toString();
}
}
}
Log.i("ipaddress==> ", "" + ipAddress);
Log.i("", "");
} catch (SocketException e) {
e.printStackTrace();
}
}
Using WifiManager:
private void getIPUsingWiFiManager() {
final WifiManager wifiManager = (WifiManager) context.getApplicationContext().getSystemService(context.WIFI_SERVICE);
WifiInfo wifiInfo = wifiManager.getConnectionInfo();
byte myIPAddress = BigInteger.valueOf(wifiInfo.getIpAddress()).toByteArray();
InetAddress myInetIP = InetAddress.getByAddress(reverseByte(myIPAddress));
String ipAddress = myInetIP.getHostAddress();
}
And also I can get IP address from DhcpInfo.
DhcpInfo dhcp = wifiManager.getDhcpInfo();
String ipAddress = dhcp.ipAddress;
Please let me know which is the best and correct way to get the IP address of connected network.

add a comment |
up vote
0
down vote
favorite
I am trying to show the ip address of of connected network(WiFi/Ethernet). I am confusing by two ip address. I can see two approaches. Get IP using WiFi info and Get IP using NetworkInterface. But I am getting two different IP's. Which one I need to use. Please let me know.
Using NetworkInterface:
private void getIpUsingNetworkInterface() {
String ipAddress = null;
try {
for (Enumeration<NetworkInterface> en = NetworkInterface.getNetworkInterfaces(); en.hasMoreElements(); ) {
NetworkInterface intf = en.nextElement();
for (Enumeration<InetAddress> enumIpAddr = intf.getInetAddresses(); enumIpAddr.hasMoreElements(); ) {
InetAddress inetAddress = enumIpAddr.nextElement();
if (!inetAddress.isLoopbackAddress()) {
ipAddress = inetAddress.getHostAddress().toString();
}
}
}
Log.i("ipaddress==> ", "" + ipAddress);
Log.i("", "");
} catch (SocketException e) {
e.printStackTrace();
}
}
Using WifiManager:
private void getIPUsingWiFiManager() {
final WifiManager wifiManager = (WifiManager) context.getApplicationContext().getSystemService(context.WIFI_SERVICE);
WifiInfo wifiInfo = wifiManager.getConnectionInfo();
byte myIPAddress = BigInteger.valueOf(wifiInfo.getIpAddress()).toByteArray();
InetAddress myInetIP = InetAddress.getByAddress(reverseByte(myIPAddress));
String ipAddress = myInetIP.getHostAddress();
}
And also I can get IP address from DhcpInfo.
DhcpInfo dhcp = wifiManager.getDhcpInfo();
String ipAddress = dhcp.ipAddress;
Please let me know which is the best and correct way to get the IP address of connected network.

Plot twist: a single network interface can have many IP addresses.
– m0skit0
Nov 19 at 12:27
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to show the ip address of of connected network(WiFi/Ethernet). I am confusing by two ip address. I can see two approaches. Get IP using WiFi info and Get IP using NetworkInterface. But I am getting two different IP's. Which one I need to use. Please let me know.
Using NetworkInterface:
private void getIpUsingNetworkInterface() {
String ipAddress = null;
try {
for (Enumeration<NetworkInterface> en = NetworkInterface.getNetworkInterfaces(); en.hasMoreElements(); ) {
NetworkInterface intf = en.nextElement();
for (Enumeration<InetAddress> enumIpAddr = intf.getInetAddresses(); enumIpAddr.hasMoreElements(); ) {
InetAddress inetAddress = enumIpAddr.nextElement();
if (!inetAddress.isLoopbackAddress()) {
ipAddress = inetAddress.getHostAddress().toString();
}
}
}
Log.i("ipaddress==> ", "" + ipAddress);
Log.i("", "");
} catch (SocketException e) {
e.printStackTrace();
}
}
Using WifiManager:
private void getIPUsingWiFiManager() {
final WifiManager wifiManager = (WifiManager) context.getApplicationContext().getSystemService(context.WIFI_SERVICE);
WifiInfo wifiInfo = wifiManager.getConnectionInfo();
byte myIPAddress = BigInteger.valueOf(wifiInfo.getIpAddress()).toByteArray();
InetAddress myInetIP = InetAddress.getByAddress(reverseByte(myIPAddress));
String ipAddress = myInetIP.getHostAddress();
}
And also I can get IP address from DhcpInfo.
DhcpInfo dhcp = wifiManager.getDhcpInfo();
String ipAddress = dhcp.ipAddress;
Please let me know which is the best and correct way to get the IP address of connected network.

I am trying to show the ip address of of connected network(WiFi/Ethernet). I am confusing by two ip address. I can see two approaches. Get IP using WiFi info and Get IP using NetworkInterface. But I am getting two different IP's. Which one I need to use. Please let me know.
Using NetworkInterface:
private void getIpUsingNetworkInterface() {
String ipAddress = null;
try {
for (Enumeration<NetworkInterface> en = NetworkInterface.getNetworkInterfaces(); en.hasMoreElements(); ) {
NetworkInterface intf = en.nextElement();
for (Enumeration<InetAddress> enumIpAddr = intf.getInetAddresses(); enumIpAddr.hasMoreElements(); ) {
InetAddress inetAddress = enumIpAddr.nextElement();
if (!inetAddress.isLoopbackAddress()) {
ipAddress = inetAddress.getHostAddress().toString();
}
}
}
Log.i("ipaddress==> ", "" + ipAddress);
Log.i("", "");
} catch (SocketException e) {
e.printStackTrace();
}
}
Using WifiManager:
private void getIPUsingWiFiManager() {
final WifiManager wifiManager = (WifiManager) context.getApplicationContext().getSystemService(context.WIFI_SERVICE);
WifiInfo wifiInfo = wifiManager.getConnectionInfo();
byte myIPAddress = BigInteger.valueOf(wifiInfo.getIpAddress()).toByteArray();
InetAddress myInetIP = InetAddress.getByAddress(reverseByte(myIPAddress));
String ipAddress = myInetIP.getHostAddress();
}
And also I can get IP address from DhcpInfo.
DhcpInfo dhcp = wifiManager.getDhcpInfo();
String ipAddress = dhcp.ipAddress;
Please let me know which is the best and correct way to get the IP address of connected network.


asked Nov 19 at 12:24
New Developer
587932
587932
Plot twist: a single network interface can have many IP addresses.
– m0skit0
Nov 19 at 12:27
add a comment |
Plot twist: a single network interface can have many IP addresses.
– m0skit0
Nov 19 at 12:27
Plot twist: a single network interface can have many IP addresses.
– m0skit0
Nov 19 at 12:27
Plot twist: a single network interface can have many IP addresses.
– m0skit0
Nov 19 at 12:27
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374593%2fandroid-networkinterface-vs-wifimanager%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1fGqP1NWw,NW3qUzpQZNAje0eaa2fJ8XsbZV3C meP3M,qbfl2eI04,ePl5PF 3IfBwy3z tpw2s9fT4 QTkDwa6XU0z79g,XQv7
Plot twist: a single network interface can have many IP addresses.
– m0skit0
Nov 19 at 12:27