Trying to create roughly even distances between randomly chosen sites on a 2D plane
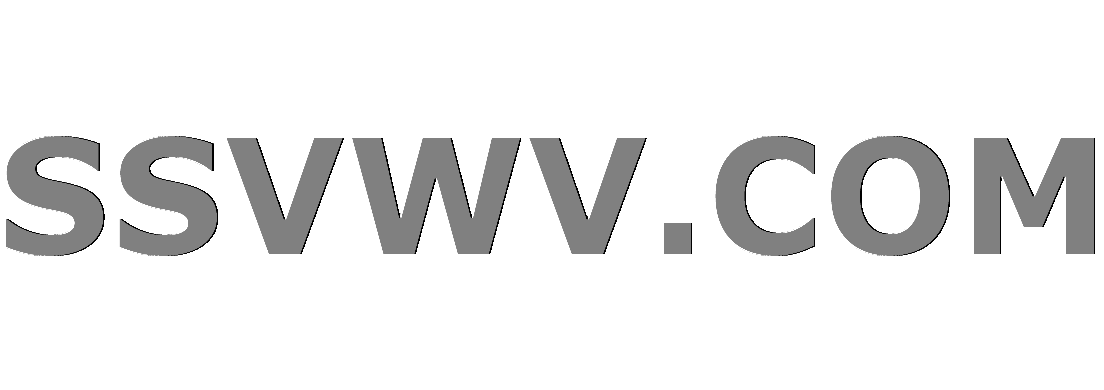
Multi tool use
$begingroup$
I included JavaFX as a tag because that's what I use to display the sites, but you shouldn't need to understand JavaFX to answer my question. I included a full working example, but you probably only need to look at the randomlyChoosePoints()
method at the bottom of my example.
I am trying to create a simplistic sweep-line algorithm to find the shortest distance between any pair of a group of randomly chosen sites on a 2D plane.
How can I randomly generate points on a 2D plane and have them be fairly close to equal in distance from each-other without being perfectly equal in distance?
I am trying to do this because I want to use this code to help me generate a random map using a voronoi diagram later on.
public class MCVE extends Application {
private final static int WIDTH = 400;
private final static int HEIGHT = 500;
private final static int AMOUNT_OF_SITES = 50;
private final static SplittableRandom RANDOM = new SplittableRandom();
@Override
public void start(Stage primaryStage) {
// Create the canvas
Canvas canvas = new Canvas(WIDTH, HEIGHT);
GraphicsContext gc = canvas.getGraphicsContext2D();
drawShapes(gc);
// Add the canvas to the window
Group root = new Group();
root.getChildren().add(canvas);
primaryStage.setScene(new Scene(root));
// Show the window
primaryStage.setTitle("Sweep-Line Test");
primaryStage.show();
}
/**
* Draws shapes on the Canvas object.
*
* @param gc
*/
private void drawShapes(GraphicsContext gc) {
gc.setFill(Color.BLACK); // sites should be black
// create random sites
HashSet<int> siteSet = randomlyChoosePoints();
// add the random sites to the displayed window
for(int i : siteSet) {
gc.fillRect(i[0], i[1], 5, 5);
}
}
private HashSet<int> randomlyChoosePoints() {
// create a HashSet to hold the sites as two-value int arrays.
HashSet<int> siteSet = new HashSet<>();
int point; // holds x and y coordinates
for(int i = 0; i < AMOUNT_OF_SITES; i++) {
// randomly choose the coordinates and add them to the HashSet
point = new int {RANDOM.nextInt(WIDTH), RANDOM.nextInt(HEIGHT)};
siteSet.add(point);
}
return siteSet;
}
}
java random javafx
$endgroup$
add a comment |
$begingroup$
I included JavaFX as a tag because that's what I use to display the sites, but you shouldn't need to understand JavaFX to answer my question. I included a full working example, but you probably only need to look at the randomlyChoosePoints()
method at the bottom of my example.
I am trying to create a simplistic sweep-line algorithm to find the shortest distance between any pair of a group of randomly chosen sites on a 2D plane.
How can I randomly generate points on a 2D plane and have them be fairly close to equal in distance from each-other without being perfectly equal in distance?
I am trying to do this because I want to use this code to help me generate a random map using a voronoi diagram later on.
public class MCVE extends Application {
private final static int WIDTH = 400;
private final static int HEIGHT = 500;
private final static int AMOUNT_OF_SITES = 50;
private final static SplittableRandom RANDOM = new SplittableRandom();
@Override
public void start(Stage primaryStage) {
// Create the canvas
Canvas canvas = new Canvas(WIDTH, HEIGHT);
GraphicsContext gc = canvas.getGraphicsContext2D();
drawShapes(gc);
// Add the canvas to the window
Group root = new Group();
root.getChildren().add(canvas);
primaryStage.setScene(new Scene(root));
// Show the window
primaryStage.setTitle("Sweep-Line Test");
primaryStage.show();
}
/**
* Draws shapes on the Canvas object.
*
* @param gc
*/
private void drawShapes(GraphicsContext gc) {
gc.setFill(Color.BLACK); // sites should be black
// create random sites
HashSet<int> siteSet = randomlyChoosePoints();
// add the random sites to the displayed window
for(int i : siteSet) {
gc.fillRect(i[0], i[1], 5, 5);
}
}
private HashSet<int> randomlyChoosePoints() {
// create a HashSet to hold the sites as two-value int arrays.
HashSet<int> siteSet = new HashSet<>();
int point; // holds x and y coordinates
for(int i = 0; i < AMOUNT_OF_SITES; i++) {
// randomly choose the coordinates and add them to the HashSet
point = new int {RANDOM.nextInt(WIDTH), RANDOM.nextInt(HEIGHT)};
siteSet.add(point);
}
return siteSet;
}
}
java random javafx
$endgroup$
add a comment |
$begingroup$
I included JavaFX as a tag because that's what I use to display the sites, but you shouldn't need to understand JavaFX to answer my question. I included a full working example, but you probably only need to look at the randomlyChoosePoints()
method at the bottom of my example.
I am trying to create a simplistic sweep-line algorithm to find the shortest distance between any pair of a group of randomly chosen sites on a 2D plane.
How can I randomly generate points on a 2D plane and have them be fairly close to equal in distance from each-other without being perfectly equal in distance?
I am trying to do this because I want to use this code to help me generate a random map using a voronoi diagram later on.
public class MCVE extends Application {
private final static int WIDTH = 400;
private final static int HEIGHT = 500;
private final static int AMOUNT_OF_SITES = 50;
private final static SplittableRandom RANDOM = new SplittableRandom();
@Override
public void start(Stage primaryStage) {
// Create the canvas
Canvas canvas = new Canvas(WIDTH, HEIGHT);
GraphicsContext gc = canvas.getGraphicsContext2D();
drawShapes(gc);
// Add the canvas to the window
Group root = new Group();
root.getChildren().add(canvas);
primaryStage.setScene(new Scene(root));
// Show the window
primaryStage.setTitle("Sweep-Line Test");
primaryStage.show();
}
/**
* Draws shapes on the Canvas object.
*
* @param gc
*/
private void drawShapes(GraphicsContext gc) {
gc.setFill(Color.BLACK); // sites should be black
// create random sites
HashSet<int> siteSet = randomlyChoosePoints();
// add the random sites to the displayed window
for(int i : siteSet) {
gc.fillRect(i[0], i[1], 5, 5);
}
}
private HashSet<int> randomlyChoosePoints() {
// create a HashSet to hold the sites as two-value int arrays.
HashSet<int> siteSet = new HashSet<>();
int point; // holds x and y coordinates
for(int i = 0; i < AMOUNT_OF_SITES; i++) {
// randomly choose the coordinates and add them to the HashSet
point = new int {RANDOM.nextInt(WIDTH), RANDOM.nextInt(HEIGHT)};
siteSet.add(point);
}
return siteSet;
}
}
java random javafx
$endgroup$
I included JavaFX as a tag because that's what I use to display the sites, but you shouldn't need to understand JavaFX to answer my question. I included a full working example, but you probably only need to look at the randomlyChoosePoints()
method at the bottom of my example.
I am trying to create a simplistic sweep-line algorithm to find the shortest distance between any pair of a group of randomly chosen sites on a 2D plane.
How can I randomly generate points on a 2D plane and have them be fairly close to equal in distance from each-other without being perfectly equal in distance?
I am trying to do this because I want to use this code to help me generate a random map using a voronoi diagram later on.
public class MCVE extends Application {
private final static int WIDTH = 400;
private final static int HEIGHT = 500;
private final static int AMOUNT_OF_SITES = 50;
private final static SplittableRandom RANDOM = new SplittableRandom();
@Override
public void start(Stage primaryStage) {
// Create the canvas
Canvas canvas = new Canvas(WIDTH, HEIGHT);
GraphicsContext gc = canvas.getGraphicsContext2D();
drawShapes(gc);
// Add the canvas to the window
Group root = new Group();
root.getChildren().add(canvas);
primaryStage.setScene(new Scene(root));
// Show the window
primaryStage.setTitle("Sweep-Line Test");
primaryStage.show();
}
/**
* Draws shapes on the Canvas object.
*
* @param gc
*/
private void drawShapes(GraphicsContext gc) {
gc.setFill(Color.BLACK); // sites should be black
// create random sites
HashSet<int> siteSet = randomlyChoosePoints();
// add the random sites to the displayed window
for(int i : siteSet) {
gc.fillRect(i[0], i[1], 5, 5);
}
}
private HashSet<int> randomlyChoosePoints() {
// create a HashSet to hold the sites as two-value int arrays.
HashSet<int> siteSet = new HashSet<>();
int point; // holds x and y coordinates
for(int i = 0; i < AMOUNT_OF_SITES; i++) {
// randomly choose the coordinates and add them to the HashSet
point = new int {RANDOM.nextInt(WIDTH), RANDOM.nextInt(HEIGHT)};
siteSet.add(point);
}
return siteSet;
}
}
java random javafx
java random javafx
asked 2 mins ago


LuminousNutriaLuminousNutria
1265
1265
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f212286%2ftrying-to-create-roughly-even-distances-between-randomly-chosen-sites-on-a-2d-pl%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f212286%2ftrying-to-create-roughly-even-distances-between-randomly-chosen-sites-on-a-2d-pl%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YF7yOwwO,FvuvbJdz cdKL,ShkkODR9yt 9oKAs,Fe7QE2poFW05pDD6KOLb75ZtwLF7o6 cL a0IQ3qw PECTdZ,oqiRoz