converting the elements in an array to a customized string using PHP-Symfony
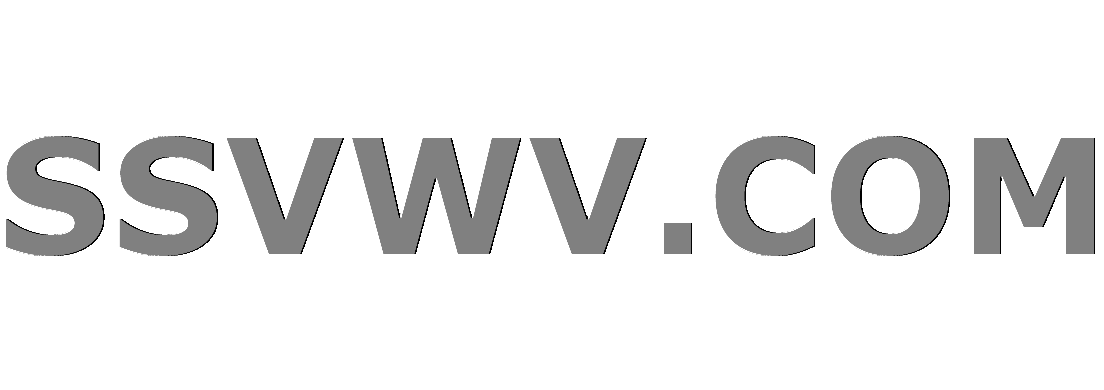
Multi tool use
I am trying to pass the elements in an array to a twig file. After it is passed, the html (in the js) code should look like
var states = ['car','van','bus']
I used the following code to do so using php symfony
/**
* @Route("/test", name="test")
*/
public function testAction(Request $request)
{
$products=$this->getDoctrine()->getRepository(products::class)->findAll();
$pr_name=array();
foreach ($products as $product){
$name=$product->getName();
array_push($pr_name,$name);
}
$pr_name2="'".implode("','",$pr_name)."'";
dump($pr_name2);
exit;
return $this->render('typeahead.html.twig', array(
'pr_name'=>$pr_name2,
));
}
My Twig file
<script>
var substringMatcher = function(strs) {
return function findMatches(q, cb) {
var matches, substringRegex;
// an array that will be populated with substring matches
matches = ;
// regex used to determine if a string contains the substring `q`
substrRegex = new RegExp(q, 'i');
// iterate through the pool of strings and for any string that
// contains the substring `q`, add it to the `matches` array
$.each(strs, function(i, str) {
if (substrRegex.test(str)) {
matches.push(str);
}
});
cb(matches);
};
};
var states = [{{ pr_name }}]
$('#the-basics .typeahead').typeahead({
hint: true,
highlight: true,
minLength: 1
},
{
name: 'states',
source: substringMatcher(states)
});
But what I get is this
How to fix this?
php arrays string symfony twig
add a comment |
I am trying to pass the elements in an array to a twig file. After it is passed, the html (in the js) code should look like
var states = ['car','van','bus']
I used the following code to do so using php symfony
/**
* @Route("/test", name="test")
*/
public function testAction(Request $request)
{
$products=$this->getDoctrine()->getRepository(products::class)->findAll();
$pr_name=array();
foreach ($products as $product){
$name=$product->getName();
array_push($pr_name,$name);
}
$pr_name2="'".implode("','",$pr_name)."'";
dump($pr_name2);
exit;
return $this->render('typeahead.html.twig', array(
'pr_name'=>$pr_name2,
));
}
My Twig file
<script>
var substringMatcher = function(strs) {
return function findMatches(q, cb) {
var matches, substringRegex;
// an array that will be populated with substring matches
matches = ;
// regex used to determine if a string contains the substring `q`
substrRegex = new RegExp(q, 'i');
// iterate through the pool of strings and for any string that
// contains the substring `q`, add it to the `matches` array
$.each(strs, function(i, str) {
if (substrRegex.test(str)) {
matches.push(str);
}
});
cb(matches);
};
};
var states = [{{ pr_name }}]
$('#the-basics .typeahead').typeahead({
hint: true,
highlight: true,
minLength: 1
},
{
name: 'states',
source: substringMatcher(states)
});
But what I get is this
How to fix this?
php arrays string symfony twig
try this:var states = [{{ pr_name | join(',') }}]
– smoqadam
Nov 22 '18 at 8:16
1
U'd need to apply theraw
filter as your output is being escaped
– DarkBee
Nov 22 '18 at 8:18
add a comment |
I am trying to pass the elements in an array to a twig file. After it is passed, the html (in the js) code should look like
var states = ['car','van','bus']
I used the following code to do so using php symfony
/**
* @Route("/test", name="test")
*/
public function testAction(Request $request)
{
$products=$this->getDoctrine()->getRepository(products::class)->findAll();
$pr_name=array();
foreach ($products as $product){
$name=$product->getName();
array_push($pr_name,$name);
}
$pr_name2="'".implode("','",$pr_name)."'";
dump($pr_name2);
exit;
return $this->render('typeahead.html.twig', array(
'pr_name'=>$pr_name2,
));
}
My Twig file
<script>
var substringMatcher = function(strs) {
return function findMatches(q, cb) {
var matches, substringRegex;
// an array that will be populated with substring matches
matches = ;
// regex used to determine if a string contains the substring `q`
substrRegex = new RegExp(q, 'i');
// iterate through the pool of strings and for any string that
// contains the substring `q`, add it to the `matches` array
$.each(strs, function(i, str) {
if (substrRegex.test(str)) {
matches.push(str);
}
});
cb(matches);
};
};
var states = [{{ pr_name }}]
$('#the-basics .typeahead').typeahead({
hint: true,
highlight: true,
minLength: 1
},
{
name: 'states',
source: substringMatcher(states)
});
But what I get is this
How to fix this?
php arrays string symfony twig
I am trying to pass the elements in an array to a twig file. After it is passed, the html (in the js) code should look like
var states = ['car','van','bus']
I used the following code to do so using php symfony
/**
* @Route("/test", name="test")
*/
public function testAction(Request $request)
{
$products=$this->getDoctrine()->getRepository(products::class)->findAll();
$pr_name=array();
foreach ($products as $product){
$name=$product->getName();
array_push($pr_name,$name);
}
$pr_name2="'".implode("','",$pr_name)."'";
dump($pr_name2);
exit;
return $this->render('typeahead.html.twig', array(
'pr_name'=>$pr_name2,
));
}
My Twig file
<script>
var substringMatcher = function(strs) {
return function findMatches(q, cb) {
var matches, substringRegex;
// an array that will be populated with substring matches
matches = ;
// regex used to determine if a string contains the substring `q`
substrRegex = new RegExp(q, 'i');
// iterate through the pool of strings and for any string that
// contains the substring `q`, add it to the `matches` array
$.each(strs, function(i, str) {
if (substrRegex.test(str)) {
matches.push(str);
}
});
cb(matches);
};
};
var states = [{{ pr_name }}]
$('#the-basics .typeahead').typeahead({
hint: true,
highlight: true,
minLength: 1
},
{
name: 'states',
source: substringMatcher(states)
});
But what I get is this
How to fix this?
php arrays string symfony twig
php arrays string symfony twig
asked Nov 22 '18 at 8:09


car treecar tree
162
162
try this:var states = [{{ pr_name | join(',') }}]
– smoqadam
Nov 22 '18 at 8:16
1
U'd need to apply theraw
filter as your output is being escaped
– DarkBee
Nov 22 '18 at 8:18
add a comment |
try this:var states = [{{ pr_name | join(',') }}]
– smoqadam
Nov 22 '18 at 8:16
1
U'd need to apply theraw
filter as your output is being escaped
– DarkBee
Nov 22 '18 at 8:18
try this:
var states = [{{ pr_name | join(',') }}]
– smoqadam
Nov 22 '18 at 8:16
try this:
var states = [{{ pr_name | join(',') }}]
– smoqadam
Nov 22 '18 at 8:16
1
1
U'd need to apply the
raw
filter as your output is being escaped– DarkBee
Nov 22 '18 at 8:18
U'd need to apply the
raw
filter as your output is being escaped– DarkBee
Nov 22 '18 at 8:18
add a comment |
1 Answer
1
active
oldest
votes
Try replacing
var states = [{{ pr_name }}]
with
var states = [{{ pr_name|raw }}]
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53426403%2fconverting-the-elements-in-an-array-to-a-customized-string-using-php-symfony%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try replacing
var states = [{{ pr_name }}]
with
var states = [{{ pr_name|raw }}]
add a comment |
Try replacing
var states = [{{ pr_name }}]
with
var states = [{{ pr_name|raw }}]
add a comment |
Try replacing
var states = [{{ pr_name }}]
with
var states = [{{ pr_name|raw }}]
Try replacing
var states = [{{ pr_name }}]
with
var states = [{{ pr_name|raw }}]
answered Nov 22 '18 at 9:20
Yoann MirYoann Mir
865
865
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53426403%2fconverting-the-elements-in-an-array-to-a-customized-string-using-php-symfony%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mk47OHKsvFdz4jinEinwj7zlWQEWvHbzd D4oOyNuxyugFaaF Uzt
try this:
var states = [{{ pr_name | join(',') }}]
– smoqadam
Nov 22 '18 at 8:16
1
U'd need to apply the
raw
filter as your output is being escaped– DarkBee
Nov 22 '18 at 8:18