Uncheck checkbox onclick of its lable in Angular 5
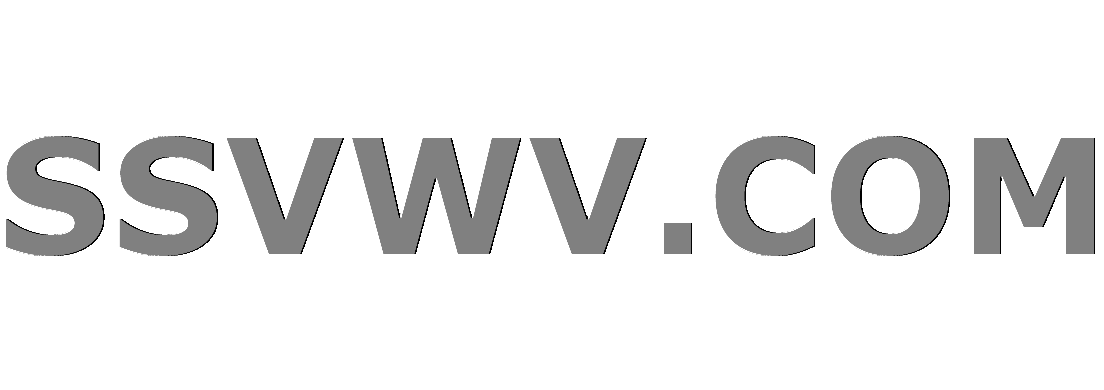
Multi tool use
Following my previous question: ngFor length in Angular 5
I was able to fix the error in the previous question.
Now I have a list of checkboxes in which I want to uncheck the selected checkbox by on its label click. Something like this: https://run.plnkr.co/plunks/5WkoJK/.
Below is my code
categories.component.html
<input [(ngModel)]="searchText" placeholder="search text goes here">
<span class="filter-clear" *ngIf="searchText.length>0" (click)="clearFilter()">X</span>
<ul class="list-unstyled scrollbar">
<li *ngFor="let category of categories| filter : searchText; let i = index">
<label class="custom-control custom-checkbox">
<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.selected" value="{{category}}" (click)="check(category, $event)" class="custom-control-input">
<small class="custom-control-indicator"></small>
<small class="custom-control-label">{{ category }}</small>
</label>
</li>
</ul>
<div (click)="deleteCategory(category)" class="selected-game" *ngFor="let category of myarray" >
<span>{{category}}</span>
</div>
categories.component.ts
import { Component, NgModule, OnInit, Input, Output, EventEmitter } from '@angular/core';
import { Feeds } from '../shared/services/feeds';
import { FeedsService } from '../shared/services/feeds.service';
import { Categories } from '../shared/services/categories';
import { CategoriesService } from '../shared/services/categories.service';
import { MyService } from '../shared/services/my-service.service';
import { Pipe, PipeTransform } from '@angular/core';
import { FilterPipe } from '../shared/pipes/category-type.pipe';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
@Component({
selector: 'app-categories',
templateUrl: './categories.component.html',
styleUrls: ['./categories.component.scss']
})
export class CategoriesComponent implements OnInit {
categories: Categories;
feeds: Feeds;
myarray = ;
myobj = {
'categories': this.myarray
};
searchText = '';
selected_count = 0;
constructor(private categoriesService: CategoriesService, private myService: MyService) {
}
ngOnInit() {
this.getCategories();
}
clearFilter() {
this.searchText = '';
}
// Clearing All Selections
clearSelection() {
this.searchText = '';
this.categories = this.categories.filter( g => {
g.checked = false;
return true;
});
}
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.filter(name => name !== category);
}
getCategories(): void {
this.categoriesService.getCategories().subscribe(categories => this.categories = categories);
}
getCategoryFeeds(myobj): void {
this.categoriesService.getfeedsByCategories(myobj).subscribe( feeds => { this.myService.change(feeds); });
}
check(category, event) {
if (event.target.checked) {
this.myarray.push(category);
this.getCategoryFeeds(this.myobj);
} else if (!event.target.checked) {
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.getCategoryFeeds(this.myobj);
}
if (!event.target.checked && this.myarray.length === 0) {
this.myService.loadAll();
}
}
}
categories.ts
export class Categories {
category_name: string;
checked: false;
}
Once I click on any checkbox It gets added into myarray Array
I am bit confused on the deleteCategory function. When I try to click on the label on the bottom of the checkbox list. Instead of unchecking the checked checkbox it deletes the checkbox itself.
I followed this example: http://www.freakyjolly.com/angular-4-5-typescript-create-filter-list-with-check-boxes-to-select-from-list/
Its a bit different from my code. Help appreciated.
angular checkbox label angular5
add a comment |
Following my previous question: ngFor length in Angular 5
I was able to fix the error in the previous question.
Now I have a list of checkboxes in which I want to uncheck the selected checkbox by on its label click. Something like this: https://run.plnkr.co/plunks/5WkoJK/.
Below is my code
categories.component.html
<input [(ngModel)]="searchText" placeholder="search text goes here">
<span class="filter-clear" *ngIf="searchText.length>0" (click)="clearFilter()">X</span>
<ul class="list-unstyled scrollbar">
<li *ngFor="let category of categories| filter : searchText; let i = index">
<label class="custom-control custom-checkbox">
<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.selected" value="{{category}}" (click)="check(category, $event)" class="custom-control-input">
<small class="custom-control-indicator"></small>
<small class="custom-control-label">{{ category }}</small>
</label>
</li>
</ul>
<div (click)="deleteCategory(category)" class="selected-game" *ngFor="let category of myarray" >
<span>{{category}}</span>
</div>
categories.component.ts
import { Component, NgModule, OnInit, Input, Output, EventEmitter } from '@angular/core';
import { Feeds } from '../shared/services/feeds';
import { FeedsService } from '../shared/services/feeds.service';
import { Categories } from '../shared/services/categories';
import { CategoriesService } from '../shared/services/categories.service';
import { MyService } from '../shared/services/my-service.service';
import { Pipe, PipeTransform } from '@angular/core';
import { FilterPipe } from '../shared/pipes/category-type.pipe';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
@Component({
selector: 'app-categories',
templateUrl: './categories.component.html',
styleUrls: ['./categories.component.scss']
})
export class CategoriesComponent implements OnInit {
categories: Categories;
feeds: Feeds;
myarray = ;
myobj = {
'categories': this.myarray
};
searchText = '';
selected_count = 0;
constructor(private categoriesService: CategoriesService, private myService: MyService) {
}
ngOnInit() {
this.getCategories();
}
clearFilter() {
this.searchText = '';
}
// Clearing All Selections
clearSelection() {
this.searchText = '';
this.categories = this.categories.filter( g => {
g.checked = false;
return true;
});
}
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.filter(name => name !== category);
}
getCategories(): void {
this.categoriesService.getCategories().subscribe(categories => this.categories = categories);
}
getCategoryFeeds(myobj): void {
this.categoriesService.getfeedsByCategories(myobj).subscribe( feeds => { this.myService.change(feeds); });
}
check(category, event) {
if (event.target.checked) {
this.myarray.push(category);
this.getCategoryFeeds(this.myobj);
} else if (!event.target.checked) {
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.getCategoryFeeds(this.myobj);
}
if (!event.target.checked && this.myarray.length === 0) {
this.myService.loadAll();
}
}
}
categories.ts
export class Categories {
category_name: string;
checked: false;
}
Once I click on any checkbox It gets added into myarray Array
I am bit confused on the deleteCategory function. When I try to click on the label on the bottom of the checkbox list. Instead of unchecking the checked checkbox it deletes the checkbox itself.
I followed this example: http://www.freakyjolly.com/angular-4-5-typescript-create-filter-list-with-check-boxes-to-select-from-list/
Its a bit different from my code. Help appreciated.
angular checkbox label angular5
add a comment |
Following my previous question: ngFor length in Angular 5
I was able to fix the error in the previous question.
Now I have a list of checkboxes in which I want to uncheck the selected checkbox by on its label click. Something like this: https://run.plnkr.co/plunks/5WkoJK/.
Below is my code
categories.component.html
<input [(ngModel)]="searchText" placeholder="search text goes here">
<span class="filter-clear" *ngIf="searchText.length>0" (click)="clearFilter()">X</span>
<ul class="list-unstyled scrollbar">
<li *ngFor="let category of categories| filter : searchText; let i = index">
<label class="custom-control custom-checkbox">
<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.selected" value="{{category}}" (click)="check(category, $event)" class="custom-control-input">
<small class="custom-control-indicator"></small>
<small class="custom-control-label">{{ category }}</small>
</label>
</li>
</ul>
<div (click)="deleteCategory(category)" class="selected-game" *ngFor="let category of myarray" >
<span>{{category}}</span>
</div>
categories.component.ts
import { Component, NgModule, OnInit, Input, Output, EventEmitter } from '@angular/core';
import { Feeds } from '../shared/services/feeds';
import { FeedsService } from '../shared/services/feeds.service';
import { Categories } from '../shared/services/categories';
import { CategoriesService } from '../shared/services/categories.service';
import { MyService } from '../shared/services/my-service.service';
import { Pipe, PipeTransform } from '@angular/core';
import { FilterPipe } from '../shared/pipes/category-type.pipe';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
@Component({
selector: 'app-categories',
templateUrl: './categories.component.html',
styleUrls: ['./categories.component.scss']
})
export class CategoriesComponent implements OnInit {
categories: Categories;
feeds: Feeds;
myarray = ;
myobj = {
'categories': this.myarray
};
searchText = '';
selected_count = 0;
constructor(private categoriesService: CategoriesService, private myService: MyService) {
}
ngOnInit() {
this.getCategories();
}
clearFilter() {
this.searchText = '';
}
// Clearing All Selections
clearSelection() {
this.searchText = '';
this.categories = this.categories.filter( g => {
g.checked = false;
return true;
});
}
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.filter(name => name !== category);
}
getCategories(): void {
this.categoriesService.getCategories().subscribe(categories => this.categories = categories);
}
getCategoryFeeds(myobj): void {
this.categoriesService.getfeedsByCategories(myobj).subscribe( feeds => { this.myService.change(feeds); });
}
check(category, event) {
if (event.target.checked) {
this.myarray.push(category);
this.getCategoryFeeds(this.myobj);
} else if (!event.target.checked) {
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.getCategoryFeeds(this.myobj);
}
if (!event.target.checked && this.myarray.length === 0) {
this.myService.loadAll();
}
}
}
categories.ts
export class Categories {
category_name: string;
checked: false;
}
Once I click on any checkbox It gets added into myarray Array
I am bit confused on the deleteCategory function. When I try to click on the label on the bottom of the checkbox list. Instead of unchecking the checked checkbox it deletes the checkbox itself.
I followed this example: http://www.freakyjolly.com/angular-4-5-typescript-create-filter-list-with-check-boxes-to-select-from-list/
Its a bit different from my code. Help appreciated.
angular checkbox label angular5
Following my previous question: ngFor length in Angular 5
I was able to fix the error in the previous question.
Now I have a list of checkboxes in which I want to uncheck the selected checkbox by on its label click. Something like this: https://run.plnkr.co/plunks/5WkoJK/.
Below is my code
categories.component.html
<input [(ngModel)]="searchText" placeholder="search text goes here">
<span class="filter-clear" *ngIf="searchText.length>0" (click)="clearFilter()">X</span>
<ul class="list-unstyled scrollbar">
<li *ngFor="let category of categories| filter : searchText; let i = index">
<label class="custom-control custom-checkbox">
<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.selected" value="{{category}}" (click)="check(category, $event)" class="custom-control-input">
<small class="custom-control-indicator"></small>
<small class="custom-control-label">{{ category }}</small>
</label>
</li>
</ul>
<div (click)="deleteCategory(category)" class="selected-game" *ngFor="let category of myarray" >
<span>{{category}}</span>
</div>
categories.component.ts
import { Component, NgModule, OnInit, Input, Output, EventEmitter } from '@angular/core';
import { Feeds } from '../shared/services/feeds';
import { FeedsService } from '../shared/services/feeds.service';
import { Categories } from '../shared/services/categories';
import { CategoriesService } from '../shared/services/categories.service';
import { MyService } from '../shared/services/my-service.service';
import { Pipe, PipeTransform } from '@angular/core';
import { FilterPipe } from '../shared/pipes/category-type.pipe';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
@Component({
selector: 'app-categories',
templateUrl: './categories.component.html',
styleUrls: ['./categories.component.scss']
})
export class CategoriesComponent implements OnInit {
categories: Categories;
feeds: Feeds;
myarray = ;
myobj = {
'categories': this.myarray
};
searchText = '';
selected_count = 0;
constructor(private categoriesService: CategoriesService, private myService: MyService) {
}
ngOnInit() {
this.getCategories();
}
clearFilter() {
this.searchText = '';
}
// Clearing All Selections
clearSelection() {
this.searchText = '';
this.categories = this.categories.filter( g => {
g.checked = false;
return true;
});
}
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.filter(name => name !== category);
}
getCategories(): void {
this.categoriesService.getCategories().subscribe(categories => this.categories = categories);
}
getCategoryFeeds(myobj): void {
this.categoriesService.getfeedsByCategories(myobj).subscribe( feeds => { this.myService.change(feeds); });
}
check(category, event) {
if (event.target.checked) {
this.myarray.push(category);
this.getCategoryFeeds(this.myobj);
} else if (!event.target.checked) {
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.getCategoryFeeds(this.myobj);
}
if (!event.target.checked && this.myarray.length === 0) {
this.myService.loadAll();
}
}
}
categories.ts
export class Categories {
category_name: string;
checked: false;
}
Once I click on any checkbox It gets added into myarray Array
I am bit confused on the deleteCategory function. When I try to click on the label on the bottom of the checkbox list. Instead of unchecking the checked checkbox it deletes the checkbox itself.
I followed this example: http://www.freakyjolly.com/angular-4-5-typescript-create-filter-list-with-check-boxes-to-select-from-list/
Its a bit different from my code. Help appreciated.
<input [(ngModel)]="searchText" placeholder="search text goes here">
<span class="filter-clear" *ngIf="searchText.length>0" (click)="clearFilter()">X</span>
<ul class="list-unstyled scrollbar">
<li *ngFor="let category of categories| filter : searchText; let i = index">
<label class="custom-control custom-checkbox">
<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.selected" value="{{category}}" (click)="check(category, $event)" class="custom-control-input">
<small class="custom-control-indicator"></small>
<small class="custom-control-label">{{ category }}</small>
</label>
</li>
</ul>
<div (click)="deleteCategory(category)" class="selected-game" *ngFor="let category of myarray" >
<span>{{category}}</span>
</div>
<input [(ngModel)]="searchText" placeholder="search text goes here">
<span class="filter-clear" *ngIf="searchText.length>0" (click)="clearFilter()">X</span>
<ul class="list-unstyled scrollbar">
<li *ngFor="let category of categories| filter : searchText; let i = index">
<label class="custom-control custom-checkbox">
<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.selected" value="{{category}}" (click)="check(category, $event)" class="custom-control-input">
<small class="custom-control-indicator"></small>
<small class="custom-control-label">{{ category }}</small>
</label>
</li>
</ul>
<div (click)="deleteCategory(category)" class="selected-game" *ngFor="let category of myarray" >
<span>{{category}}</span>
</div>
import { Component, NgModule, OnInit, Input, Output, EventEmitter } from '@angular/core';
import { Feeds } from '../shared/services/feeds';
import { FeedsService } from '../shared/services/feeds.service';
import { Categories } from '../shared/services/categories';
import { CategoriesService } from '../shared/services/categories.service';
import { MyService } from '../shared/services/my-service.service';
import { Pipe, PipeTransform } from '@angular/core';
import { FilterPipe } from '../shared/pipes/category-type.pipe';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
@Component({
selector: 'app-categories',
templateUrl: './categories.component.html',
styleUrls: ['./categories.component.scss']
})
export class CategoriesComponent implements OnInit {
categories: Categories;
feeds: Feeds;
myarray = ;
myobj = {
'categories': this.myarray
};
searchText = '';
selected_count = 0;
constructor(private categoriesService: CategoriesService, private myService: MyService) {
}
ngOnInit() {
this.getCategories();
}
clearFilter() {
this.searchText = '';
}
// Clearing All Selections
clearSelection() {
this.searchText = '';
this.categories = this.categories.filter( g => {
g.checked = false;
return true;
});
}
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.filter(name => name !== category);
}
getCategories(): void {
this.categoriesService.getCategories().subscribe(categories => this.categories = categories);
}
getCategoryFeeds(myobj): void {
this.categoriesService.getfeedsByCategories(myobj).subscribe( feeds => { this.myService.change(feeds); });
}
check(category, event) {
if (event.target.checked) {
this.myarray.push(category);
this.getCategoryFeeds(this.myobj);
} else if (!event.target.checked) {
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.getCategoryFeeds(this.myobj);
}
if (!event.target.checked && this.myarray.length === 0) {
this.myService.loadAll();
}
}
}
import { Component, NgModule, OnInit, Input, Output, EventEmitter } from '@angular/core';
import { Feeds } from '../shared/services/feeds';
import { FeedsService } from '../shared/services/feeds.service';
import { Categories } from '../shared/services/categories';
import { CategoriesService } from '../shared/services/categories.service';
import { MyService } from '../shared/services/my-service.service';
import { Pipe, PipeTransform } from '@angular/core';
import { FilterPipe } from '../shared/pipes/category-type.pipe';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
@Component({
selector: 'app-categories',
templateUrl: './categories.component.html',
styleUrls: ['./categories.component.scss']
})
export class CategoriesComponent implements OnInit {
categories: Categories;
feeds: Feeds;
myarray = ;
myobj = {
'categories': this.myarray
};
searchText = '';
selected_count = 0;
constructor(private categoriesService: CategoriesService, private myService: MyService) {
}
ngOnInit() {
this.getCategories();
}
clearFilter() {
this.searchText = '';
}
// Clearing All Selections
clearSelection() {
this.searchText = '';
this.categories = this.categories.filter( g => {
g.checked = false;
return true;
});
}
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.filter(name => name !== category);
}
getCategories(): void {
this.categoriesService.getCategories().subscribe(categories => this.categories = categories);
}
getCategoryFeeds(myobj): void {
this.categoriesService.getfeedsByCategories(myobj).subscribe( feeds => { this.myService.change(feeds); });
}
check(category, event) {
if (event.target.checked) {
this.myarray.push(category);
this.getCategoryFeeds(this.myobj);
} else if (!event.target.checked) {
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.getCategoryFeeds(this.myobj);
}
if (!event.target.checked && this.myarray.length === 0) {
this.myService.loadAll();
}
}
}
export class Categories {
category_name: string;
checked: false;
}
export class Categories {
category_name: string;
checked: false;
}
angular checkbox label angular5
angular checkbox label angular5
edited Nov 21 at 2:10
Samuel Liew♦
44.2k32112148
44.2k32112148
asked Apr 3 at 15:04
Kamlesh Katpara
771118
771118
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
You need to use [checked] property in checkbox tag. The reason to remove checkbox is cause you delete item from your array and *ngFor update.
Or in deleteCategory you can change to catecory.selected = false
edit add code
change deleteCategory to
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.map(name => {
if (name === category)
name.checked = false
return name
});}
and your html to<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.checked" value="{{category}}" (click)="check(category, $event)"
it's work for me
Can you please share an example or a plnkr ?
– Kamlesh Katpara
Apr 3 at 19:45
@KamleshKatpara I'm edit.
– eran hadad
Apr 3 at 20:35
it throws errorERROR TypeError: Cannot create property 'checked' on string 'Mobiles & Tablets' at eval (categories.component.ts:54)
– Kamlesh Katpara
Apr 3 at 21:16
add a comment |
just replace below code in your example....
<input (change)="getSelected()" type="checkbox"
name="games" value="{{g.id}}"
id="{{g.id}}" [(ngModel)]="g.selected"/>
<label class="game-text" for="{{g.id}}">{{g.name}}</label>
in above added id attribute in input and for attribute in label also replaced span with label.
http://plnkr.co/edit/R0bQ4xEDp2vtCrAri6bQ?p=preview
I already tried that it won't work with my code, I need to get the category and event in getSelected() method.
– Kamlesh Katpara
Apr 4 at 4:56
add a comment |
Before removing make category.selected
to false, instead of click
event you can use ngModelChange
which is always safe , and check for the reference leak b/w categories and myarray , use push
or concat
wisely based on your need.
Can you share an example or plnkr ?
– Kamlesh Katpara
Apr 4 at 11:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49633338%2funcheck-checkbox-onclick-of-its-lable-in-angular-5%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to use [checked] property in checkbox tag. The reason to remove checkbox is cause you delete item from your array and *ngFor update.
Or in deleteCategory you can change to catecory.selected = false
edit add code
change deleteCategory to
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.map(name => {
if (name === category)
name.checked = false
return name
});}
and your html to<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.checked" value="{{category}}" (click)="check(category, $event)"
it's work for me
Can you please share an example or a plnkr ?
– Kamlesh Katpara
Apr 3 at 19:45
@KamleshKatpara I'm edit.
– eran hadad
Apr 3 at 20:35
it throws errorERROR TypeError: Cannot create property 'checked' on string 'Mobiles & Tablets' at eval (categories.component.ts:54)
– Kamlesh Katpara
Apr 3 at 21:16
add a comment |
You need to use [checked] property in checkbox tag. The reason to remove checkbox is cause you delete item from your array and *ngFor update.
Or in deleteCategory you can change to catecory.selected = false
edit add code
change deleteCategory to
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.map(name => {
if (name === category)
name.checked = false
return name
});}
and your html to<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.checked" value="{{category}}" (click)="check(category, $event)"
it's work for me
Can you please share an example or a plnkr ?
– Kamlesh Katpara
Apr 3 at 19:45
@KamleshKatpara I'm edit.
– eran hadad
Apr 3 at 20:35
it throws errorERROR TypeError: Cannot create property 'checked' on string 'Mobiles & Tablets' at eval (categories.component.ts:54)
– Kamlesh Katpara
Apr 3 at 21:16
add a comment |
You need to use [checked] property in checkbox tag. The reason to remove checkbox is cause you delete item from your array and *ngFor update.
Or in deleteCategory you can change to catecory.selected = false
edit add code
change deleteCategory to
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.map(name => {
if (name === category)
name.checked = false
return name
});}
and your html to<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.checked" value="{{category}}" (click)="check(category, $event)"
it's work for me
You need to use [checked] property in checkbox tag. The reason to remove checkbox is cause you delete item from your array and *ngFor update.
Or in deleteCategory you can change to catecory.selected = false
edit add code
change deleteCategory to
deleteCategory(category) {
this.searchText = '';
const index = this.myarray.indexOf(category);
this.myarray.splice(index, 1);
this.categories = this.categories.map(name => {
if (name === category)
name.checked = false
return name
});}
and your html to<input type="checkbox" name="category" id="checkbox{{ i +1 }}" [(ngModel)]="category.checked" value="{{category}}" (click)="check(category, $event)"
it's work for me
edited Apr 3 at 20:34
answered Apr 3 at 19:40
eran hadad
12
12
Can you please share an example or a plnkr ?
– Kamlesh Katpara
Apr 3 at 19:45
@KamleshKatpara I'm edit.
– eran hadad
Apr 3 at 20:35
it throws errorERROR TypeError: Cannot create property 'checked' on string 'Mobiles & Tablets' at eval (categories.component.ts:54)
– Kamlesh Katpara
Apr 3 at 21:16
add a comment |
Can you please share an example or a plnkr ?
– Kamlesh Katpara
Apr 3 at 19:45
@KamleshKatpara I'm edit.
– eran hadad
Apr 3 at 20:35
it throws errorERROR TypeError: Cannot create property 'checked' on string 'Mobiles & Tablets' at eval (categories.component.ts:54)
– Kamlesh Katpara
Apr 3 at 21:16
Can you please share an example or a plnkr ?
– Kamlesh Katpara
Apr 3 at 19:45
Can you please share an example or a plnkr ?
– Kamlesh Katpara
Apr 3 at 19:45
@KamleshKatpara I'm edit.
– eran hadad
Apr 3 at 20:35
@KamleshKatpara I'm edit.
– eran hadad
Apr 3 at 20:35
it throws error
ERROR TypeError: Cannot create property 'checked' on string 'Mobiles & Tablets' at eval (categories.component.ts:54)
– Kamlesh Katpara
Apr 3 at 21:16
it throws error
ERROR TypeError: Cannot create property 'checked' on string 'Mobiles & Tablets' at eval (categories.component.ts:54)
– Kamlesh Katpara
Apr 3 at 21:16
add a comment |
just replace below code in your example....
<input (change)="getSelected()" type="checkbox"
name="games" value="{{g.id}}"
id="{{g.id}}" [(ngModel)]="g.selected"/>
<label class="game-text" for="{{g.id}}">{{g.name}}</label>
in above added id attribute in input and for attribute in label also replaced span with label.
http://plnkr.co/edit/R0bQ4xEDp2vtCrAri6bQ?p=preview
I already tried that it won't work with my code, I need to get the category and event in getSelected() method.
– Kamlesh Katpara
Apr 4 at 4:56
add a comment |
just replace below code in your example....
<input (change)="getSelected()" type="checkbox"
name="games" value="{{g.id}}"
id="{{g.id}}" [(ngModel)]="g.selected"/>
<label class="game-text" for="{{g.id}}">{{g.name}}</label>
in above added id attribute in input and for attribute in label also replaced span with label.
http://plnkr.co/edit/R0bQ4xEDp2vtCrAri6bQ?p=preview
I already tried that it won't work with my code, I need to get the category and event in getSelected() method.
– Kamlesh Katpara
Apr 4 at 4:56
add a comment |
just replace below code in your example....
<input (change)="getSelected()" type="checkbox"
name="games" value="{{g.id}}"
id="{{g.id}}" [(ngModel)]="g.selected"/>
<label class="game-text" for="{{g.id}}">{{g.name}}</label>
in above added id attribute in input and for attribute in label also replaced span with label.
http://plnkr.co/edit/R0bQ4xEDp2vtCrAri6bQ?p=preview
just replace below code in your example....
<input (change)="getSelected()" type="checkbox"
name="games" value="{{g.id}}"
id="{{g.id}}" [(ngModel)]="g.selected"/>
<label class="game-text" for="{{g.id}}">{{g.name}}</label>
in above added id attribute in input and for attribute in label also replaced span with label.
http://plnkr.co/edit/R0bQ4xEDp2vtCrAri6bQ?p=preview
<input (change)="getSelected()" type="checkbox"
name="games" value="{{g.id}}"
id="{{g.id}}" [(ngModel)]="g.selected"/>
<label class="game-text" for="{{g.id}}">{{g.name}}</label>
<input (change)="getSelected()" type="checkbox"
name="games" value="{{g.id}}"
id="{{g.id}}" [(ngModel)]="g.selected"/>
<label class="game-text" for="{{g.id}}">{{g.name}}</label>
answered Apr 4 at 4:10
Rahulgiri Goswami
1
1
I already tried that it won't work with my code, I need to get the category and event in getSelected() method.
– Kamlesh Katpara
Apr 4 at 4:56
add a comment |
I already tried that it won't work with my code, I need to get the category and event in getSelected() method.
– Kamlesh Katpara
Apr 4 at 4:56
I already tried that it won't work with my code, I need to get the category and event in getSelected() method.
– Kamlesh Katpara
Apr 4 at 4:56
I already tried that it won't work with my code, I need to get the category and event in getSelected() method.
– Kamlesh Katpara
Apr 4 at 4:56
add a comment |
Before removing make category.selected
to false, instead of click
event you can use ngModelChange
which is always safe , and check for the reference leak b/w categories and myarray , use push
or concat
wisely based on your need.
Can you share an example or plnkr ?
– Kamlesh Katpara
Apr 4 at 11:50
add a comment |
Before removing make category.selected
to false, instead of click
event you can use ngModelChange
which is always safe , and check for the reference leak b/w categories and myarray , use push
or concat
wisely based on your need.
Can you share an example or plnkr ?
– Kamlesh Katpara
Apr 4 at 11:50
add a comment |
Before removing make category.selected
to false, instead of click
event you can use ngModelChange
which is always safe , and check for the reference leak b/w categories and myarray , use push
or concat
wisely based on your need.
Before removing make category.selected
to false, instead of click
event you can use ngModelChange
which is always safe , and check for the reference leak b/w categories and myarray , use push
or concat
wisely based on your need.
answered Apr 4 at 10:52


Ashok Kumar
31
31
Can you share an example or plnkr ?
– Kamlesh Katpara
Apr 4 at 11:50
add a comment |
Can you share an example or plnkr ?
– Kamlesh Katpara
Apr 4 at 11:50
Can you share an example or plnkr ?
– Kamlesh Katpara
Apr 4 at 11:50
Can you share an example or plnkr ?
– Kamlesh Katpara
Apr 4 at 11:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49633338%2funcheck-checkbox-onclick-of-its-lable-in-angular-5%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0yxc733UszF2sn91Cgepjnn K,tmbN3,MsxT ZNE4VCmnut K qD VwYVzg4hZGP9s1g,j QC1PZo,f