Providing Injection for AndroidX Fragment with Dagger2?
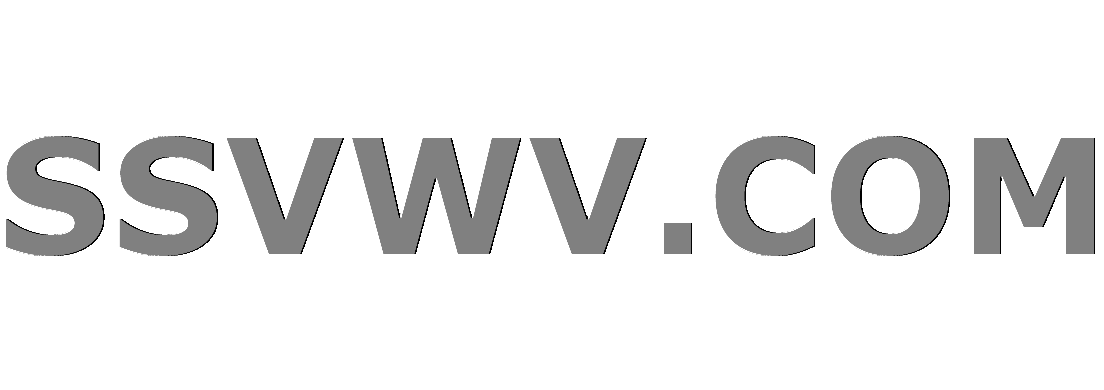
Multi tool use
I'm having some issues trying to provide an Injection to an AndroidX fragment, and I'm not sure what the exact issue is, and how to fix it. The app refuses to build, giving the following error:
error: [Dagger/MissingBinding] java.util.Map<java.lang.Class<? extends androidx.fragment.app.Fragment>,javax.inject.Provider<dagger.android.AndroidInjector.Factory<? extends androidx.fragment.app.Fragment>>> cannot be provided without an @Provides-annotated method.
Here's the method to provide the Injection in the Fragment:
private fun performDependencyInjection() = AndroidSupportInjection.inject(this)
The fragment's parent Activity implements HasSupportFragmentInjector:
class MainActivity : BaseActivity(), MainMVPView, HasSupportFragmentInjector {
@Inject
internal lateinit var dispatchingAndroidInjector: DispatchingAndroidInjector<Fragment>
...
override fun supportFragmentInjector() = dispatchingAndroidInjector
}
I'm completely lost as to where to solve this error from here. It doesn't appear that there is much current documentation for using Dagger2 with AndroidX.
I do feel it is important to note I enabled AndroidX and Jetifier through
gradle.properties:
android.useAndroidX=true
android.enableJetifier=true
However, nothing had changed after a clean and rebuild of the project.
What is the proper way to provide an Injection to an AndroidX fragment using Dagger2?
Edit: For the record, this is on Dagger 2 version 2.19. If I switch to using 2.16, everything works fine.


add a comment |
I'm having some issues trying to provide an Injection to an AndroidX fragment, and I'm not sure what the exact issue is, and how to fix it. The app refuses to build, giving the following error:
error: [Dagger/MissingBinding] java.util.Map<java.lang.Class<? extends androidx.fragment.app.Fragment>,javax.inject.Provider<dagger.android.AndroidInjector.Factory<? extends androidx.fragment.app.Fragment>>> cannot be provided without an @Provides-annotated method.
Here's the method to provide the Injection in the Fragment:
private fun performDependencyInjection() = AndroidSupportInjection.inject(this)
The fragment's parent Activity implements HasSupportFragmentInjector:
class MainActivity : BaseActivity(), MainMVPView, HasSupportFragmentInjector {
@Inject
internal lateinit var dispatchingAndroidInjector: DispatchingAndroidInjector<Fragment>
...
override fun supportFragmentInjector() = dispatchingAndroidInjector
}
I'm completely lost as to where to solve this error from here. It doesn't appear that there is much current documentation for using Dagger2 with AndroidX.
I do feel it is important to note I enabled AndroidX and Jetifier through
gradle.properties:
android.useAndroidX=true
android.enableJetifier=true
However, nothing had changed after a clean and rebuild of the project.
What is the proper way to provide an Injection to an AndroidX fragment using Dagger2?
Edit: For the record, this is on Dagger 2 version 2.19. If I switch to using 2.16, everything works fine.


add a comment |
I'm having some issues trying to provide an Injection to an AndroidX fragment, and I'm not sure what the exact issue is, and how to fix it. The app refuses to build, giving the following error:
error: [Dagger/MissingBinding] java.util.Map<java.lang.Class<? extends androidx.fragment.app.Fragment>,javax.inject.Provider<dagger.android.AndroidInjector.Factory<? extends androidx.fragment.app.Fragment>>> cannot be provided without an @Provides-annotated method.
Here's the method to provide the Injection in the Fragment:
private fun performDependencyInjection() = AndroidSupportInjection.inject(this)
The fragment's parent Activity implements HasSupportFragmentInjector:
class MainActivity : BaseActivity(), MainMVPView, HasSupportFragmentInjector {
@Inject
internal lateinit var dispatchingAndroidInjector: DispatchingAndroidInjector<Fragment>
...
override fun supportFragmentInjector() = dispatchingAndroidInjector
}
I'm completely lost as to where to solve this error from here. It doesn't appear that there is much current documentation for using Dagger2 with AndroidX.
I do feel it is important to note I enabled AndroidX and Jetifier through
gradle.properties:
android.useAndroidX=true
android.enableJetifier=true
However, nothing had changed after a clean and rebuild of the project.
What is the proper way to provide an Injection to an AndroidX fragment using Dagger2?
Edit: For the record, this is on Dagger 2 version 2.19. If I switch to using 2.16, everything works fine.


I'm having some issues trying to provide an Injection to an AndroidX fragment, and I'm not sure what the exact issue is, and how to fix it. The app refuses to build, giving the following error:
error: [Dagger/MissingBinding] java.util.Map<java.lang.Class<? extends androidx.fragment.app.Fragment>,javax.inject.Provider<dagger.android.AndroidInjector.Factory<? extends androidx.fragment.app.Fragment>>> cannot be provided without an @Provides-annotated method.
Here's the method to provide the Injection in the Fragment:
private fun performDependencyInjection() = AndroidSupportInjection.inject(this)
The fragment's parent Activity implements HasSupportFragmentInjector:
class MainActivity : BaseActivity(), MainMVPView, HasSupportFragmentInjector {
@Inject
internal lateinit var dispatchingAndroidInjector: DispatchingAndroidInjector<Fragment>
...
override fun supportFragmentInjector() = dispatchingAndroidInjector
}
I'm completely lost as to where to solve this error from here. It doesn't appear that there is much current documentation for using Dagger2 with AndroidX.
I do feel it is important to note I enabled AndroidX and Jetifier through
gradle.properties:
android.useAndroidX=true
android.enableJetifier=true
However, nothing had changed after a clean and rebuild of the project.
What is the proper way to provide an Injection to an AndroidX fragment using Dagger2?
Edit: For the record, this is on Dagger 2 version 2.19. If I switch to using 2.16, everything works fine.




edited Nov 21 at 4:44
asked Nov 21 at 2:24
josephoneill
425725
425725
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
This is due to a mismatch in the Jetifier sources, as you can see from the below code:
# Androidx compatible dagger
{
"from": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" },
"to": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" }
}
From the release note of dagger-2.19:
In the next release (2.20), we will remove the old format. This will
allow us to support AndroidX packages better.
So for now you either have to stick with version 2.16 or wait for the 2.20 release.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404471%2fproviding-injection-for-androidx-fragment-with-dagger2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is due to a mismatch in the Jetifier sources, as you can see from the below code:
# Androidx compatible dagger
{
"from": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" },
"to": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" }
}
From the release note of dagger-2.19:
In the next release (2.20), we will remove the old format. This will
allow us to support AndroidX packages better.
So for now you either have to stick with version 2.16 or wait for the 2.20 release.
add a comment |
This is due to a mismatch in the Jetifier sources, as you can see from the below code:
# Androidx compatible dagger
{
"from": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" },
"to": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" }
}
From the release note of dagger-2.19:
In the next release (2.20), we will remove the old format. This will
allow us to support AndroidX packages better.
So for now you either have to stick with version 2.16 or wait for the 2.20 release.
add a comment |
This is due to a mismatch in the Jetifier sources, as you can see from the below code:
# Androidx compatible dagger
{
"from": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" },
"to": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" }
}
From the release note of dagger-2.19:
In the next release (2.20), we will remove the old format. This will
allow us to support AndroidX packages better.
So for now you either have to stick with version 2.16 or wait for the 2.20 release.
This is due to a mismatch in the Jetifier sources, as you can see from the below code:
# Androidx compatible dagger
{
"from": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" },
"to": { "groupId": "com.google.dagger", "artifactId": "dagger-android-processor", "version": "2.16" }
}
From the release note of dagger-2.19:
In the next release (2.20), we will remove the old format. This will
allow us to support AndroidX packages better.
So for now you either have to stick with version 2.16 or wait for the 2.20 release.
answered Nov 21 at 13:22


Benjamin
2,48321533
2,48321533
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404471%2fproviding-injection-for-androidx-fragment-with-dagger2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VV1oXimCPuDvNgnF2qSe VSpj2Quxg2C7y1KrHwP C8Bn,l