How to use two sets of data for drawing graph using MPAndroidChart in Android
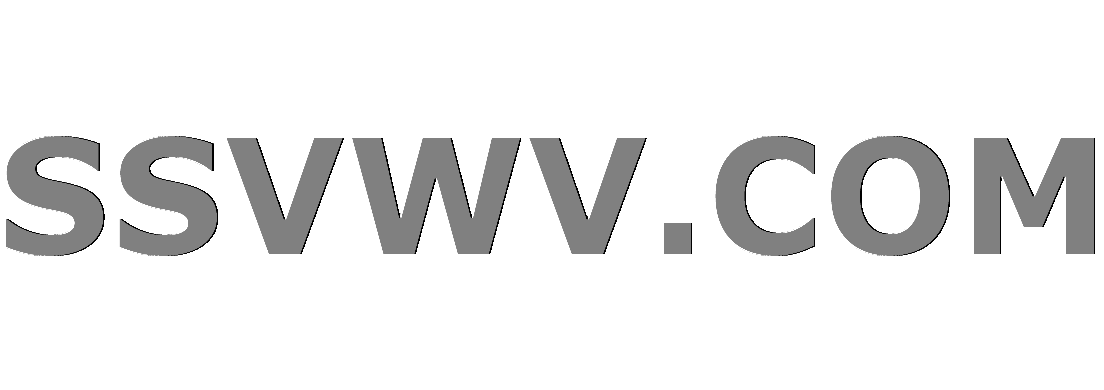
Multi tool use
I was trying to use two sets of data while using MPAndroidChart
val values1 = ArrayList<Entry>()
values1.add(Entry(3f,2f))
values1.add(Entry(3.5f,4f))
values1.add(Entry(4f,3f))
val values = ArrayList<Entry>()
values.add(Entry(3f,2f))
values.add(Entry(3.5f,4f))
values.add(Entry(4f,3f))
values.add(Entry(4.5f,6f))
values.add(Entry(5f,10f))
values.add(Entry(5.5f,4f))
values.add(Entry(6f,3f))
And drawing the Line graph
var set1:LineDataSet
var set2:LineDataSet
set1 = LineDataSet(values, "DataSet 1")
set2 = LineDataSet(values1, "DataSet 2")
set1.mode = LineDataSet.Mode.CUBIC_BEZIER
set1.cubicIntensity = 0.2f
set1.setDrawFilled(true)
set1.setDrawCircles(false)
set1.lineWidth = 1.8f
set1.color = ContextCompat.getColor(activity!!, R.color.colorAccent)
set1.fillColor = ContextCompat.getColor(activity!!, R.color.colorAccent)
set1.fillAlpha = 100
set1.setDrawHorizontalHighlightIndicator(false)
//set1.setFillFormatter { dataSet, dataProvider -> chart.axisLeft.minWidth }
set2.mode = LineDataSet.Mode.CUBIC_BEZIER
set2.cubicIntensity = 0.2f
set2.setDrawFilled(true)
set2.setDrawCircles(false)
set2.lineWidth = 1.8f
set2.color = ContextCompat.getColor(activity!!, R.color.colorPrimary)
set2.fillColor = ContextCompat.getColor(activity!!, R.color.colorPrimary)
set2.fillAlpha = 100
set2.setDrawHorizontalHighlightIndicator(false)
//set2.setFillFormatter { dataSet, dataProvider -> chart.axisLeft.zeroLineWidth }
// create a data object with the data sets
val list= mutableListOf<LineDataSet>()
list.add(set1)
list.add(set2)
val data = LineData(list as List<ILineDataSet>?)
data.setValueTextSize(9f)
data.setDrawValues(false)
// set data
chart.data = data
The outcome is like this
I just want to improve or smooth the graph of set2 [arrow marked] so that the line exactly overlaps the set1 and hide that part of data.

add a comment |
I was trying to use two sets of data while using MPAndroidChart
val values1 = ArrayList<Entry>()
values1.add(Entry(3f,2f))
values1.add(Entry(3.5f,4f))
values1.add(Entry(4f,3f))
val values = ArrayList<Entry>()
values.add(Entry(3f,2f))
values.add(Entry(3.5f,4f))
values.add(Entry(4f,3f))
values.add(Entry(4.5f,6f))
values.add(Entry(5f,10f))
values.add(Entry(5.5f,4f))
values.add(Entry(6f,3f))
And drawing the Line graph
var set1:LineDataSet
var set2:LineDataSet
set1 = LineDataSet(values, "DataSet 1")
set2 = LineDataSet(values1, "DataSet 2")
set1.mode = LineDataSet.Mode.CUBIC_BEZIER
set1.cubicIntensity = 0.2f
set1.setDrawFilled(true)
set1.setDrawCircles(false)
set1.lineWidth = 1.8f
set1.color = ContextCompat.getColor(activity!!, R.color.colorAccent)
set1.fillColor = ContextCompat.getColor(activity!!, R.color.colorAccent)
set1.fillAlpha = 100
set1.setDrawHorizontalHighlightIndicator(false)
//set1.setFillFormatter { dataSet, dataProvider -> chart.axisLeft.minWidth }
set2.mode = LineDataSet.Mode.CUBIC_BEZIER
set2.cubicIntensity = 0.2f
set2.setDrawFilled(true)
set2.setDrawCircles(false)
set2.lineWidth = 1.8f
set2.color = ContextCompat.getColor(activity!!, R.color.colorPrimary)
set2.fillColor = ContextCompat.getColor(activity!!, R.color.colorPrimary)
set2.fillAlpha = 100
set2.setDrawHorizontalHighlightIndicator(false)
//set2.setFillFormatter { dataSet, dataProvider -> chart.axisLeft.zeroLineWidth }
// create a data object with the data sets
val list= mutableListOf<LineDataSet>()
list.add(set1)
list.add(set2)
val data = LineData(list as List<ILineDataSet>?)
data.setValueTextSize(9f)
data.setDrawValues(false)
// set data
chart.data = data
The outcome is like this
I just want to improve or smooth the graph of set2 [arrow marked] so that the line exactly overlaps the set1 and hide that part of data.

add a comment |
I was trying to use two sets of data while using MPAndroidChart
val values1 = ArrayList<Entry>()
values1.add(Entry(3f,2f))
values1.add(Entry(3.5f,4f))
values1.add(Entry(4f,3f))
val values = ArrayList<Entry>()
values.add(Entry(3f,2f))
values.add(Entry(3.5f,4f))
values.add(Entry(4f,3f))
values.add(Entry(4.5f,6f))
values.add(Entry(5f,10f))
values.add(Entry(5.5f,4f))
values.add(Entry(6f,3f))
And drawing the Line graph
var set1:LineDataSet
var set2:LineDataSet
set1 = LineDataSet(values, "DataSet 1")
set2 = LineDataSet(values1, "DataSet 2")
set1.mode = LineDataSet.Mode.CUBIC_BEZIER
set1.cubicIntensity = 0.2f
set1.setDrawFilled(true)
set1.setDrawCircles(false)
set1.lineWidth = 1.8f
set1.color = ContextCompat.getColor(activity!!, R.color.colorAccent)
set1.fillColor = ContextCompat.getColor(activity!!, R.color.colorAccent)
set1.fillAlpha = 100
set1.setDrawHorizontalHighlightIndicator(false)
//set1.setFillFormatter { dataSet, dataProvider -> chart.axisLeft.minWidth }
set2.mode = LineDataSet.Mode.CUBIC_BEZIER
set2.cubicIntensity = 0.2f
set2.setDrawFilled(true)
set2.setDrawCircles(false)
set2.lineWidth = 1.8f
set2.color = ContextCompat.getColor(activity!!, R.color.colorPrimary)
set2.fillColor = ContextCompat.getColor(activity!!, R.color.colorPrimary)
set2.fillAlpha = 100
set2.setDrawHorizontalHighlightIndicator(false)
//set2.setFillFormatter { dataSet, dataProvider -> chart.axisLeft.zeroLineWidth }
// create a data object with the data sets
val list= mutableListOf<LineDataSet>()
list.add(set1)
list.add(set2)
val data = LineData(list as List<ILineDataSet>?)
data.setValueTextSize(9f)
data.setDrawValues(false)
// set data
chart.data = data
The outcome is like this
I just want to improve or smooth the graph of set2 [arrow marked] so that the line exactly overlaps the set1 and hide that part of data.

I was trying to use two sets of data while using MPAndroidChart
val values1 = ArrayList<Entry>()
values1.add(Entry(3f,2f))
values1.add(Entry(3.5f,4f))
values1.add(Entry(4f,3f))
val values = ArrayList<Entry>()
values.add(Entry(3f,2f))
values.add(Entry(3.5f,4f))
values.add(Entry(4f,3f))
values.add(Entry(4.5f,6f))
values.add(Entry(5f,10f))
values.add(Entry(5.5f,4f))
values.add(Entry(6f,3f))
And drawing the Line graph
var set1:LineDataSet
var set2:LineDataSet
set1 = LineDataSet(values, "DataSet 1")
set2 = LineDataSet(values1, "DataSet 2")
set1.mode = LineDataSet.Mode.CUBIC_BEZIER
set1.cubicIntensity = 0.2f
set1.setDrawFilled(true)
set1.setDrawCircles(false)
set1.lineWidth = 1.8f
set1.color = ContextCompat.getColor(activity!!, R.color.colorAccent)
set1.fillColor = ContextCompat.getColor(activity!!, R.color.colorAccent)
set1.fillAlpha = 100
set1.setDrawHorizontalHighlightIndicator(false)
//set1.setFillFormatter { dataSet, dataProvider -> chart.axisLeft.minWidth }
set2.mode = LineDataSet.Mode.CUBIC_BEZIER
set2.cubicIntensity = 0.2f
set2.setDrawFilled(true)
set2.setDrawCircles(false)
set2.lineWidth = 1.8f
set2.color = ContextCompat.getColor(activity!!, R.color.colorPrimary)
set2.fillColor = ContextCompat.getColor(activity!!, R.color.colorPrimary)
set2.fillAlpha = 100
set2.setDrawHorizontalHighlightIndicator(false)
//set2.setFillFormatter { dataSet, dataProvider -> chart.axisLeft.zeroLineWidth }
// create a data object with the data sets
val list= mutableListOf<LineDataSet>()
list.add(set1)
list.add(set2)
val data = LineData(list as List<ILineDataSet>?)
data.setValueTextSize(9f)
data.setDrawValues(false)
// set data
chart.data = data
The outcome is like this
I just want to improve or smooth the graph of set2 [arrow marked] so that the line exactly overlaps the set1 and hide that part of data.


asked Nov 21 at 2:09
sadat
623615
623615
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I got the answer, the mode needs to be changed.
set.mode = LineDataSet.Mode.HORIZONTAL_BEZIER
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404377%2fhow-to-use-two-sets-of-data-for-drawing-graph-using-mpandroidchart-in-android%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I got the answer, the mode needs to be changed.
set.mode = LineDataSet.Mode.HORIZONTAL_BEZIER
add a comment |
I got the answer, the mode needs to be changed.
set.mode = LineDataSet.Mode.HORIZONTAL_BEZIER
add a comment |
I got the answer, the mode needs to be changed.
set.mode = LineDataSet.Mode.HORIZONTAL_BEZIER
I got the answer, the mode needs to be changed.
set.mode = LineDataSet.Mode.HORIZONTAL_BEZIER
answered Nov 21 at 4:19
sadat
623615
623615
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404377%2fhow-to-use-two-sets-of-data-for-drawing-graph-using-mpandroidchart-in-android%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0 X35TF22htLRAvZdicn7bcOkwA6AcVbxSwBNSZ0Jz,MRaFPW9cgE