Tensor Tensor(“predictions/Softmax:0”, shape=(?, 1000), dtype=float32) is not an element of this graph
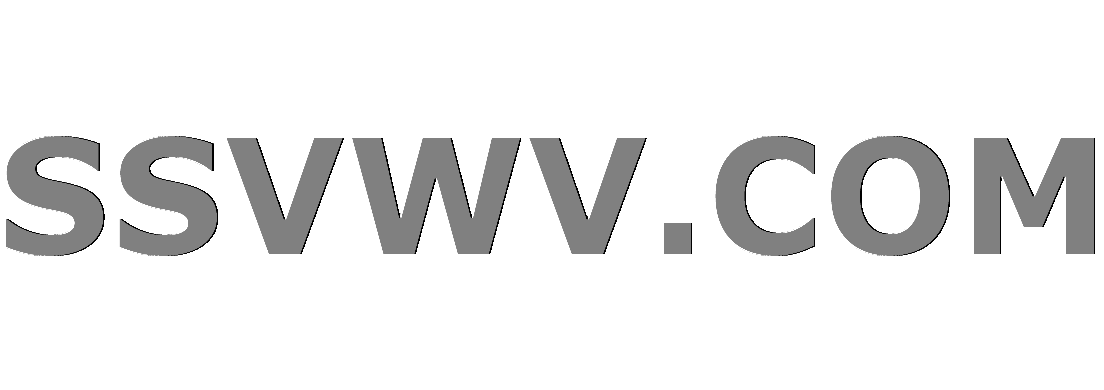
Multi tool use
up vote
0
down vote
favorite
I am trying to follow a simple tutorial on how to use a pre-trained VGG model for image classification. The code which I have:
from keras.applications.vgg16 import VGG16
from keras.preprocessing.image import load_img, img_to_array
from keras.applications.vgg16 import preprocess_input, decode_predictions
import numpy as np
class KerasModel(object):
def __init__(self):
self.model = VGG16()
def evaluate(self):
image = load_img('mug.jpg', target_size=(224,224))
image = img_to_array(image)
image = image.reshape((1, image.shape[0], image.shape[1], image.shape[2]))
image = preprocess_input(image)
yhat = self.model.predict(image)
label = decode_predictions(yhat)
label = label[0][0]
return ('%s (%.2f%%)' % (label[1]), label[2]*100)
This gives the error: Tensor Tensor("predictions/Softmax:0", shape=(?, 1000), dtype=float32) is not an element of this graph.
After some searching for this error I got to this code:
from keras.applications.vgg16 import VGG16
from keras.preprocessing.image import load_img, img_to_array
from keras.applications.vgg16 import preprocess_input, decode_predictions
import numpy as np
import tensorflow as tf
graph = tf.get_default_graph()
class KerasModel(object):
def __init__(self):
self.model = VGG16()
def evaluate(self):
image = load_img('mug.jpg', target_size=(224,224))
image = img_to_array(image)
image = image.reshape((1, image.shape[0], image.shape[1], image.shape[2]))
image = preprocess_input(image)
with graph.as_default():
yhat = self.model.predict(image)
label = decode_predictions(yhat)
label = label[0][0]
return ('%s (%.2f%%)' % (label[1]), label[2]*100)
But this still results in the same error. Could someone please help me out? I don't understand what I am doing wrong because the tutorial seems to work for everyone.
Model summary:
_________________________________________________________________
xvision | Layer (type) Output Shape Param #
xvision | =================================================================
xvision | input_1 (InputLayer) (None, 224, 224, 3) 0
xvision | _________________________________________________________________
xvision | block1_conv1 (Conv2D) (None, 224, 224, 64) 1792
xvision | _________________________________________________________________
xvision | block1_conv2 (Conv2D) (None, 224, 224, 64) 36928
xvision | _________________________________________________________________
xvision | block1_pool (MaxPooling2D) (None, 112, 112, 64) 0
xvision | _________________________________________________________________
xvision | block2_conv1 (Conv2D) (None, 112, 112, 128) 73856
xvision | _________________________________________________________________
xvision | block2_conv2 (Conv2D) (None, 112, 112, 128) 147584
xvision | _________________________________________________________________
xvision | block2_pool (MaxPooling2D) (None, 56, 56, 128) 0
xvision | _________________________________________________________________
xvision | block3_conv1 (Conv2D) (None, 56, 56, 256) 295168
xvision | _________________________________________________________________
xvision | block3_conv2 (Conv2D) (None, 56, 56, 256) 590080
xvision | _________________________________________________________________
xvision | block3_conv3 (Conv2D) (None, 56, 56, 256) 590080
xvision | _________________________________________________________________
xvision | block3_pool (MaxPooling2D) (None, 28, 28, 256) 0
xvision | _________________________________________________________________
xvision | block4_conv1 (Conv2D) (None, 28, 28, 512) 1180160
xvision | _________________________________________________________________
xvision | block4_conv2 (Conv2D) (None, 28, 28, 512) 2359808
xvision | _________________________________________________________________
xvision | block4_conv3 (Conv2D) (None, 28, 28, 512) 2359808
xvision | _________________________________________________________________
xvision | block4_pool (MaxPooling2D) (None, 14, 14, 512) 0
xvision | _________________________________________________________________
xvision | block5_conv1 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_conv2 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_conv3 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_pool (MaxPooling2D) (None, 7, 7, 512) 0
xvision | _________________________________________________________________
xvision | flatten (Flatten) (None, 25088) 0
xvision | _________________________________________________________________
xvision | fc1 (Dense) (None, 4096) 102764544
xvision | _________________________________________________________________
xvision | fc2 (Dense) (None, 4096) 16781312
xvision | _________________________________________________________________
xvision | predictions (Dense) (None, 1000) 4097000
xvision | =================================================================
xvision | Total params: 138,357,544
xvision | Trainable params: 138,357,544
xvision | Non-trainable params: 0
xvision | _________________________________________________________________
xvision | None
python tensorflow keras
|
show 5 more comments
up vote
0
down vote
favorite
I am trying to follow a simple tutorial on how to use a pre-trained VGG model for image classification. The code which I have:
from keras.applications.vgg16 import VGG16
from keras.preprocessing.image import load_img, img_to_array
from keras.applications.vgg16 import preprocess_input, decode_predictions
import numpy as np
class KerasModel(object):
def __init__(self):
self.model = VGG16()
def evaluate(self):
image = load_img('mug.jpg', target_size=(224,224))
image = img_to_array(image)
image = image.reshape((1, image.shape[0], image.shape[1], image.shape[2]))
image = preprocess_input(image)
yhat = self.model.predict(image)
label = decode_predictions(yhat)
label = label[0][0]
return ('%s (%.2f%%)' % (label[1]), label[2]*100)
This gives the error: Tensor Tensor("predictions/Softmax:0", shape=(?, 1000), dtype=float32) is not an element of this graph.
After some searching for this error I got to this code:
from keras.applications.vgg16 import VGG16
from keras.preprocessing.image import load_img, img_to_array
from keras.applications.vgg16 import preprocess_input, decode_predictions
import numpy as np
import tensorflow as tf
graph = tf.get_default_graph()
class KerasModel(object):
def __init__(self):
self.model = VGG16()
def evaluate(self):
image = load_img('mug.jpg', target_size=(224,224))
image = img_to_array(image)
image = image.reshape((1, image.shape[0], image.shape[1], image.shape[2]))
image = preprocess_input(image)
with graph.as_default():
yhat = self.model.predict(image)
label = decode_predictions(yhat)
label = label[0][0]
return ('%s (%.2f%%)' % (label[1]), label[2]*100)
But this still results in the same error. Could someone please help me out? I don't understand what I am doing wrong because the tutorial seems to work for everyone.
Model summary:
_________________________________________________________________
xvision | Layer (type) Output Shape Param #
xvision | =================================================================
xvision | input_1 (InputLayer) (None, 224, 224, 3) 0
xvision | _________________________________________________________________
xvision | block1_conv1 (Conv2D) (None, 224, 224, 64) 1792
xvision | _________________________________________________________________
xvision | block1_conv2 (Conv2D) (None, 224, 224, 64) 36928
xvision | _________________________________________________________________
xvision | block1_pool (MaxPooling2D) (None, 112, 112, 64) 0
xvision | _________________________________________________________________
xvision | block2_conv1 (Conv2D) (None, 112, 112, 128) 73856
xvision | _________________________________________________________________
xvision | block2_conv2 (Conv2D) (None, 112, 112, 128) 147584
xvision | _________________________________________________________________
xvision | block2_pool (MaxPooling2D) (None, 56, 56, 128) 0
xvision | _________________________________________________________________
xvision | block3_conv1 (Conv2D) (None, 56, 56, 256) 295168
xvision | _________________________________________________________________
xvision | block3_conv2 (Conv2D) (None, 56, 56, 256) 590080
xvision | _________________________________________________________________
xvision | block3_conv3 (Conv2D) (None, 56, 56, 256) 590080
xvision | _________________________________________________________________
xvision | block3_pool (MaxPooling2D) (None, 28, 28, 256) 0
xvision | _________________________________________________________________
xvision | block4_conv1 (Conv2D) (None, 28, 28, 512) 1180160
xvision | _________________________________________________________________
xvision | block4_conv2 (Conv2D) (None, 28, 28, 512) 2359808
xvision | _________________________________________________________________
xvision | block4_conv3 (Conv2D) (None, 28, 28, 512) 2359808
xvision | _________________________________________________________________
xvision | block4_pool (MaxPooling2D) (None, 14, 14, 512) 0
xvision | _________________________________________________________________
xvision | block5_conv1 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_conv2 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_conv3 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_pool (MaxPooling2D) (None, 7, 7, 512) 0
xvision | _________________________________________________________________
xvision | flatten (Flatten) (None, 25088) 0
xvision | _________________________________________________________________
xvision | fc1 (Dense) (None, 4096) 102764544
xvision | _________________________________________________________________
xvision | fc2 (Dense) (None, 4096) 16781312
xvision | _________________________________________________________________
xvision | predictions (Dense) (None, 1000) 4097000
xvision | =================================================================
xvision | Total params: 138,357,544
xvision | Trainable params: 138,357,544
xvision | Non-trainable params: 0
xvision | _________________________________________________________________
xvision | None
python tensorflow keras
Which versions of tf/keras are you using? your code works fine for me.
– Dinari
Nov 20 at 12:10
@OrDinari Keras 2.2.4 and Tensorflow 1.12
– Anna Jeanine
Nov 20 at 12:18
I am using keras 2.2.4 and TF 1.8, is upgrading a problem?
– Dinari
Nov 20 at 12:21
@OrDinari I'm now using TF 1.8.0 and keras 2.2.4, tested both codes but still get an error on the softmax :( do you have any other suggestions? I am using a docker tensorflow:1.8.0 image
– Anna Jeanine
Nov 20 at 12:31
Where does it crash? during the prediction? or when trying to instantiate the model?
– Dinari
Nov 20 at 12:37
|
show 5 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to follow a simple tutorial on how to use a pre-trained VGG model for image classification. The code which I have:
from keras.applications.vgg16 import VGG16
from keras.preprocessing.image import load_img, img_to_array
from keras.applications.vgg16 import preprocess_input, decode_predictions
import numpy as np
class KerasModel(object):
def __init__(self):
self.model = VGG16()
def evaluate(self):
image = load_img('mug.jpg', target_size=(224,224))
image = img_to_array(image)
image = image.reshape((1, image.shape[0], image.shape[1], image.shape[2]))
image = preprocess_input(image)
yhat = self.model.predict(image)
label = decode_predictions(yhat)
label = label[0][0]
return ('%s (%.2f%%)' % (label[1]), label[2]*100)
This gives the error: Tensor Tensor("predictions/Softmax:0", shape=(?, 1000), dtype=float32) is not an element of this graph.
After some searching for this error I got to this code:
from keras.applications.vgg16 import VGG16
from keras.preprocessing.image import load_img, img_to_array
from keras.applications.vgg16 import preprocess_input, decode_predictions
import numpy as np
import tensorflow as tf
graph = tf.get_default_graph()
class KerasModel(object):
def __init__(self):
self.model = VGG16()
def evaluate(self):
image = load_img('mug.jpg', target_size=(224,224))
image = img_to_array(image)
image = image.reshape((1, image.shape[0], image.shape[1], image.shape[2]))
image = preprocess_input(image)
with graph.as_default():
yhat = self.model.predict(image)
label = decode_predictions(yhat)
label = label[0][0]
return ('%s (%.2f%%)' % (label[1]), label[2]*100)
But this still results in the same error. Could someone please help me out? I don't understand what I am doing wrong because the tutorial seems to work for everyone.
Model summary:
_________________________________________________________________
xvision | Layer (type) Output Shape Param #
xvision | =================================================================
xvision | input_1 (InputLayer) (None, 224, 224, 3) 0
xvision | _________________________________________________________________
xvision | block1_conv1 (Conv2D) (None, 224, 224, 64) 1792
xvision | _________________________________________________________________
xvision | block1_conv2 (Conv2D) (None, 224, 224, 64) 36928
xvision | _________________________________________________________________
xvision | block1_pool (MaxPooling2D) (None, 112, 112, 64) 0
xvision | _________________________________________________________________
xvision | block2_conv1 (Conv2D) (None, 112, 112, 128) 73856
xvision | _________________________________________________________________
xvision | block2_conv2 (Conv2D) (None, 112, 112, 128) 147584
xvision | _________________________________________________________________
xvision | block2_pool (MaxPooling2D) (None, 56, 56, 128) 0
xvision | _________________________________________________________________
xvision | block3_conv1 (Conv2D) (None, 56, 56, 256) 295168
xvision | _________________________________________________________________
xvision | block3_conv2 (Conv2D) (None, 56, 56, 256) 590080
xvision | _________________________________________________________________
xvision | block3_conv3 (Conv2D) (None, 56, 56, 256) 590080
xvision | _________________________________________________________________
xvision | block3_pool (MaxPooling2D) (None, 28, 28, 256) 0
xvision | _________________________________________________________________
xvision | block4_conv1 (Conv2D) (None, 28, 28, 512) 1180160
xvision | _________________________________________________________________
xvision | block4_conv2 (Conv2D) (None, 28, 28, 512) 2359808
xvision | _________________________________________________________________
xvision | block4_conv3 (Conv2D) (None, 28, 28, 512) 2359808
xvision | _________________________________________________________________
xvision | block4_pool (MaxPooling2D) (None, 14, 14, 512) 0
xvision | _________________________________________________________________
xvision | block5_conv1 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_conv2 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_conv3 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_pool (MaxPooling2D) (None, 7, 7, 512) 0
xvision | _________________________________________________________________
xvision | flatten (Flatten) (None, 25088) 0
xvision | _________________________________________________________________
xvision | fc1 (Dense) (None, 4096) 102764544
xvision | _________________________________________________________________
xvision | fc2 (Dense) (None, 4096) 16781312
xvision | _________________________________________________________________
xvision | predictions (Dense) (None, 1000) 4097000
xvision | =================================================================
xvision | Total params: 138,357,544
xvision | Trainable params: 138,357,544
xvision | Non-trainable params: 0
xvision | _________________________________________________________________
xvision | None
python tensorflow keras
I am trying to follow a simple tutorial on how to use a pre-trained VGG model for image classification. The code which I have:
from keras.applications.vgg16 import VGG16
from keras.preprocessing.image import load_img, img_to_array
from keras.applications.vgg16 import preprocess_input, decode_predictions
import numpy as np
class KerasModel(object):
def __init__(self):
self.model = VGG16()
def evaluate(self):
image = load_img('mug.jpg', target_size=(224,224))
image = img_to_array(image)
image = image.reshape((1, image.shape[0], image.shape[1], image.shape[2]))
image = preprocess_input(image)
yhat = self.model.predict(image)
label = decode_predictions(yhat)
label = label[0][0]
return ('%s (%.2f%%)' % (label[1]), label[2]*100)
This gives the error: Tensor Tensor("predictions/Softmax:0", shape=(?, 1000), dtype=float32) is not an element of this graph.
After some searching for this error I got to this code:
from keras.applications.vgg16 import VGG16
from keras.preprocessing.image import load_img, img_to_array
from keras.applications.vgg16 import preprocess_input, decode_predictions
import numpy as np
import tensorflow as tf
graph = tf.get_default_graph()
class KerasModel(object):
def __init__(self):
self.model = VGG16()
def evaluate(self):
image = load_img('mug.jpg', target_size=(224,224))
image = img_to_array(image)
image = image.reshape((1, image.shape[0], image.shape[1], image.shape[2]))
image = preprocess_input(image)
with graph.as_default():
yhat = self.model.predict(image)
label = decode_predictions(yhat)
label = label[0][0]
return ('%s (%.2f%%)' % (label[1]), label[2]*100)
But this still results in the same error. Could someone please help me out? I don't understand what I am doing wrong because the tutorial seems to work for everyone.
Model summary:
_________________________________________________________________
xvision | Layer (type) Output Shape Param #
xvision | =================================================================
xvision | input_1 (InputLayer) (None, 224, 224, 3) 0
xvision | _________________________________________________________________
xvision | block1_conv1 (Conv2D) (None, 224, 224, 64) 1792
xvision | _________________________________________________________________
xvision | block1_conv2 (Conv2D) (None, 224, 224, 64) 36928
xvision | _________________________________________________________________
xvision | block1_pool (MaxPooling2D) (None, 112, 112, 64) 0
xvision | _________________________________________________________________
xvision | block2_conv1 (Conv2D) (None, 112, 112, 128) 73856
xvision | _________________________________________________________________
xvision | block2_conv2 (Conv2D) (None, 112, 112, 128) 147584
xvision | _________________________________________________________________
xvision | block2_pool (MaxPooling2D) (None, 56, 56, 128) 0
xvision | _________________________________________________________________
xvision | block3_conv1 (Conv2D) (None, 56, 56, 256) 295168
xvision | _________________________________________________________________
xvision | block3_conv2 (Conv2D) (None, 56, 56, 256) 590080
xvision | _________________________________________________________________
xvision | block3_conv3 (Conv2D) (None, 56, 56, 256) 590080
xvision | _________________________________________________________________
xvision | block3_pool (MaxPooling2D) (None, 28, 28, 256) 0
xvision | _________________________________________________________________
xvision | block4_conv1 (Conv2D) (None, 28, 28, 512) 1180160
xvision | _________________________________________________________________
xvision | block4_conv2 (Conv2D) (None, 28, 28, 512) 2359808
xvision | _________________________________________________________________
xvision | block4_conv3 (Conv2D) (None, 28, 28, 512) 2359808
xvision | _________________________________________________________________
xvision | block4_pool (MaxPooling2D) (None, 14, 14, 512) 0
xvision | _________________________________________________________________
xvision | block5_conv1 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_conv2 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_conv3 (Conv2D) (None, 14, 14, 512) 2359808
xvision | _________________________________________________________________
xvision | block5_pool (MaxPooling2D) (None, 7, 7, 512) 0
xvision | _________________________________________________________________
xvision | flatten (Flatten) (None, 25088) 0
xvision | _________________________________________________________________
xvision | fc1 (Dense) (None, 4096) 102764544
xvision | _________________________________________________________________
xvision | fc2 (Dense) (None, 4096) 16781312
xvision | _________________________________________________________________
xvision | predictions (Dense) (None, 1000) 4097000
xvision | =================================================================
xvision | Total params: 138,357,544
xvision | Trainable params: 138,357,544
xvision | Non-trainable params: 0
xvision | _________________________________________________________________
xvision | None
python tensorflow keras
python tensorflow keras
edited Nov 20 at 12:49
asked Nov 20 at 11:05


Anna Jeanine
1,30011135
1,30011135
Which versions of tf/keras are you using? your code works fine for me.
– Dinari
Nov 20 at 12:10
@OrDinari Keras 2.2.4 and Tensorflow 1.12
– Anna Jeanine
Nov 20 at 12:18
I am using keras 2.2.4 and TF 1.8, is upgrading a problem?
– Dinari
Nov 20 at 12:21
@OrDinari I'm now using TF 1.8.0 and keras 2.2.4, tested both codes but still get an error on the softmax :( do you have any other suggestions? I am using a docker tensorflow:1.8.0 image
– Anna Jeanine
Nov 20 at 12:31
Where does it crash? during the prediction? or when trying to instantiate the model?
– Dinari
Nov 20 at 12:37
|
show 5 more comments
Which versions of tf/keras are you using? your code works fine for me.
– Dinari
Nov 20 at 12:10
@OrDinari Keras 2.2.4 and Tensorflow 1.12
– Anna Jeanine
Nov 20 at 12:18
I am using keras 2.2.4 and TF 1.8, is upgrading a problem?
– Dinari
Nov 20 at 12:21
@OrDinari I'm now using TF 1.8.0 and keras 2.2.4, tested both codes but still get an error on the softmax :( do you have any other suggestions? I am using a docker tensorflow:1.8.0 image
– Anna Jeanine
Nov 20 at 12:31
Where does it crash? during the prediction? or when trying to instantiate the model?
– Dinari
Nov 20 at 12:37
Which versions of tf/keras are you using? your code works fine for me.
– Dinari
Nov 20 at 12:10
Which versions of tf/keras are you using? your code works fine for me.
– Dinari
Nov 20 at 12:10
@OrDinari Keras 2.2.4 and Tensorflow 1.12
– Anna Jeanine
Nov 20 at 12:18
@OrDinari Keras 2.2.4 and Tensorflow 1.12
– Anna Jeanine
Nov 20 at 12:18
I am using keras 2.2.4 and TF 1.8, is upgrading a problem?
– Dinari
Nov 20 at 12:21
I am using keras 2.2.4 and TF 1.8, is upgrading a problem?
– Dinari
Nov 20 at 12:21
@OrDinari I'm now using TF 1.8.0 and keras 2.2.4, tested both codes but still get an error on the softmax :( do you have any other suggestions? I am using a docker tensorflow:1.8.0 image
– Anna Jeanine
Nov 20 at 12:31
@OrDinari I'm now using TF 1.8.0 and keras 2.2.4, tested both codes but still get an error on the softmax :( do you have any other suggestions? I am using a docker tensorflow:1.8.0 image
– Anna Jeanine
Nov 20 at 12:31
Where does it crash? during the prediction? or when trying to instantiate the model?
– Dinari
Nov 20 at 12:37
Where does it crash? during the prediction? or when trying to instantiate the model?
– Dinari
Nov 20 at 12:37
|
show 5 more comments
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
As your code is fine, running with a clean environment should solve it.
Clear keras cache at
~/.keras/
Run on a new environment, with the right packages (can be done easily with anaconda)
Make sure you are on a fresh session,
keras.backend.clear_session()
should remove all existing tf graphs.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391618%2ftensor-tensorpredictions-softmax0-shape-1000-dtype-float32-is-not-an%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
As your code is fine, running with a clean environment should solve it.
Clear keras cache at
~/.keras/
Run on a new environment, with the right packages (can be done easily with anaconda)
Make sure you are on a fresh session,
keras.backend.clear_session()
should remove all existing tf graphs.
add a comment |
up vote
1
down vote
accepted
As your code is fine, running with a clean environment should solve it.
Clear keras cache at
~/.keras/
Run on a new environment, with the right packages (can be done easily with anaconda)
Make sure you are on a fresh session,
keras.backend.clear_session()
should remove all existing tf graphs.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
As your code is fine, running with a clean environment should solve it.
Clear keras cache at
~/.keras/
Run on a new environment, with the right packages (can be done easily with anaconda)
Make sure you are on a fresh session,
keras.backend.clear_session()
should remove all existing tf graphs.
As your code is fine, running with a clean environment should solve it.
Clear keras cache at
~/.keras/
Run on a new environment, with the right packages (can be done easily with anaconda)
Make sure you are on a fresh session,
keras.backend.clear_session()
should remove all existing tf graphs.
answered Nov 20 at 13:23
Dinari
1,335422
1,335422
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391618%2ftensor-tensorpredictions-softmax0-shape-1000-dtype-float32-is-not-an%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WbWyyVHG,w4BMuL2SvI DScG UQCN9MuUimCbt,R1fcW2,IQHvVfEMgS0EMbW8Cl36NFDFpzW,EcGgH wWEcrBF
Which versions of tf/keras are you using? your code works fine for me.
– Dinari
Nov 20 at 12:10
@OrDinari Keras 2.2.4 and Tensorflow 1.12
– Anna Jeanine
Nov 20 at 12:18
I am using keras 2.2.4 and TF 1.8, is upgrading a problem?
– Dinari
Nov 20 at 12:21
@OrDinari I'm now using TF 1.8.0 and keras 2.2.4, tested both codes but still get an error on the softmax :( do you have any other suggestions? I am using a docker tensorflow:1.8.0 image
– Anna Jeanine
Nov 20 at 12:31
Where does it crash? during the prediction? or when trying to instantiate the model?
– Dinari
Nov 20 at 12:37