Javascript accessing dynamically created element via getElementbyId
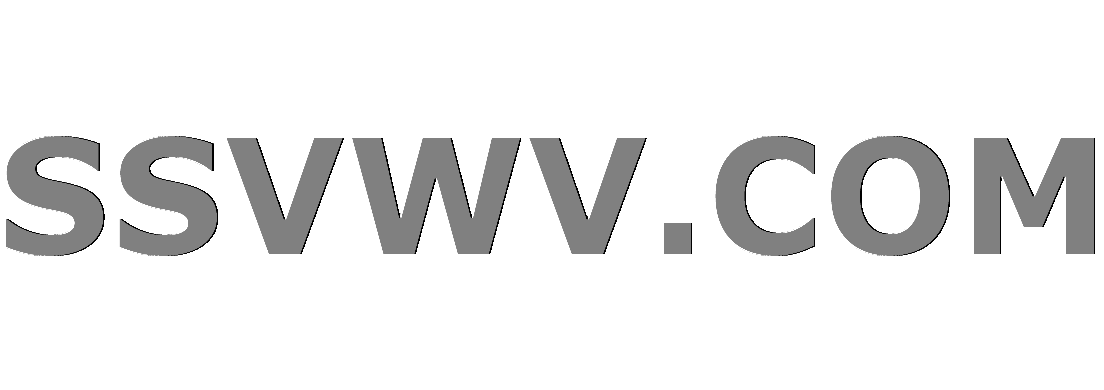
Multi tool use
up vote
0
down vote
favorite
I'm playing around with Javascript as a hobby and I've been having trouble accessing elements that I have dynamically created via another function.
Essentially, I have a link that dynamically creates a couple of dropdown selects with a few options. Then I have a second link which I would try to print some of the selected options onto console.
HTML:
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
Javascript:
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
option.id = 'option' + i + k;
console.log(option.id)
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("option01");
console.log(test.options[test.selectedIndex].value);
}
I've printed to console the option id's as they are created (seems to have no problem printing this), and added them to the DOM via appendChild. However with my second function, I am unable to retrieve the selected value of the options despite explicitly referencing the id.
My guess is that it has something to do with the order the scripts are loaded. Can anyone help me understand what's going on?
Attached is my JSFiddle file,
http://jsfiddle.net/c8h6gx2d/1/
Cheers,
javascript html dynamic
add a comment |
up vote
0
down vote
favorite
I'm playing around with Javascript as a hobby and I've been having trouble accessing elements that I have dynamically created via another function.
Essentially, I have a link that dynamically creates a couple of dropdown selects with a few options. Then I have a second link which I would try to print some of the selected options onto console.
HTML:
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
Javascript:
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
option.id = 'option' + i + k;
console.log(option.id)
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("option01");
console.log(test.options[test.selectedIndex].value);
}
I've printed to console the option id's as they are created (seems to have no problem printing this), and added them to the DOM via appendChild. However with my second function, I am unable to retrieve the selected value of the options despite explicitly referencing the id.
My guess is that it has something to do with the order the scripts are loaded. Can anyone help me understand what's going on?
Attached is my JSFiddle file,
http://jsfiddle.net/c8h6gx2d/1/
Cheers,
javascript html dynamic
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm playing around with Javascript as a hobby and I've been having trouble accessing elements that I have dynamically created via another function.
Essentially, I have a link that dynamically creates a couple of dropdown selects with a few options. Then I have a second link which I would try to print some of the selected options onto console.
HTML:
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
Javascript:
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
option.id = 'option' + i + k;
console.log(option.id)
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("option01");
console.log(test.options[test.selectedIndex].value);
}
I've printed to console the option id's as they are created (seems to have no problem printing this), and added them to the DOM via appendChild. However with my second function, I am unable to retrieve the selected value of the options despite explicitly referencing the id.
My guess is that it has something to do with the order the scripts are loaded. Can anyone help me understand what's going on?
Attached is my JSFiddle file,
http://jsfiddle.net/c8h6gx2d/1/
Cheers,
javascript html dynamic
I'm playing around with Javascript as a hobby and I've been having trouble accessing elements that I have dynamically created via another function.
Essentially, I have a link that dynamically creates a couple of dropdown selects with a few options. Then I have a second link which I would try to print some of the selected options onto console.
HTML:
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
Javascript:
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
option.id = 'option' + i + k;
console.log(option.id)
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("option01");
console.log(test.options[test.selectedIndex].value);
}
I've printed to console the option id's as they are created (seems to have no problem printing this), and added them to the DOM via appendChild. However with my second function, I am unable to retrieve the selected value of the options despite explicitly referencing the id.
My guess is that it has something to do with the order the scripts are loaded. Can anyone help me understand what's going on?
Attached is my JSFiddle file,
http://jsfiddle.net/c8h6gx2d/1/
Cheers,
javascript html dynamic
javascript html dynamic
asked Nov 20 at 11:24
keven ren
716
716
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
3
down vote
accepted
The problem is that <option>
s are nested inside of <select>
s, and it's the *<select>
*s which have a selectedIndex
property. So, test.options[test.selectedIndex].value
won't work when test
is an <option>
element. Try using getElementById
to get one of the <select>
s, for one, and then just access its .value
(which is less cumbersome than checking selectedIndex
):
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
dropdown.id = 'select' + i;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("select0");
console.log(test.value);
// same as:
// console.log(test.options[test.selectedIndex].value);
}
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
Also note that duplicate IDs in a single document is invalid HTML, so if you ever call maker
more than once, for your HTML to be valid, you might have a separate counter outside of maker
that gets incremented:
const makeCount = 0;
function maker() {
// ...
dropdown.id = 'select' + makeCount + '_' + i;
// ...
makeCount++;
}
(or, avoid IDs entirely, if at all possible, numeric ID indicies are a code smell - use classes instead)
AH, I see. I have some code to removeChild before I created the select/option elements, but I had it omitted for this question for readability. Thanks for the suggestion to use classes, I'll give it a look!
– keven ren
Nov 20 at 11:46
add a comment |
up vote
1
down vote
We need to discriminate between option elements and the select element. The select element is the one with which you want to interact most of the time, and the option elements are simply a collection of possible entries for the select element.
Your code as it stands now generates these sort of elements:
<select>
<option value="2" id="option00">2</option>
<option value="3" id="option00">3</option>
<option value="5" id="option00">5</option>
</select>
As you see all the options receive the same ID - which is generally forbidden in HTML documents. You might consider moving the ID indicator to the select element, which also gives you access to the value of the selected option.
Here is the revised JS code with comments before revisions:
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
# Giving the select item an ID instead of each option
dropdown.id = 'select' + i + k;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
option.id = 'option'
console.log(option.id)
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
# Getting the select element instead of the option
var selectElement = document.getElementById("select00");
# The value attribute of the select element is the value of the selected option
console.log(selectElement.value);
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391975%2fjavascript-accessing-dynamically-created-element-via-getelementbyid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
The problem is that <option>
s are nested inside of <select>
s, and it's the *<select>
*s which have a selectedIndex
property. So, test.options[test.selectedIndex].value
won't work when test
is an <option>
element. Try using getElementById
to get one of the <select>
s, for one, and then just access its .value
(which is less cumbersome than checking selectedIndex
):
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
dropdown.id = 'select' + i;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("select0");
console.log(test.value);
// same as:
// console.log(test.options[test.selectedIndex].value);
}
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
Also note that duplicate IDs in a single document is invalid HTML, so if you ever call maker
more than once, for your HTML to be valid, you might have a separate counter outside of maker
that gets incremented:
const makeCount = 0;
function maker() {
// ...
dropdown.id = 'select' + makeCount + '_' + i;
// ...
makeCount++;
}
(or, avoid IDs entirely, if at all possible, numeric ID indicies are a code smell - use classes instead)
AH, I see. I have some code to removeChild before I created the select/option elements, but I had it omitted for this question for readability. Thanks for the suggestion to use classes, I'll give it a look!
– keven ren
Nov 20 at 11:46
add a comment |
up vote
3
down vote
accepted
The problem is that <option>
s are nested inside of <select>
s, and it's the *<select>
*s which have a selectedIndex
property. So, test.options[test.selectedIndex].value
won't work when test
is an <option>
element. Try using getElementById
to get one of the <select>
s, for one, and then just access its .value
(which is less cumbersome than checking selectedIndex
):
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
dropdown.id = 'select' + i;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("select0");
console.log(test.value);
// same as:
// console.log(test.options[test.selectedIndex].value);
}
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
Also note that duplicate IDs in a single document is invalid HTML, so if you ever call maker
more than once, for your HTML to be valid, you might have a separate counter outside of maker
that gets incremented:
const makeCount = 0;
function maker() {
// ...
dropdown.id = 'select' + makeCount + '_' + i;
// ...
makeCount++;
}
(or, avoid IDs entirely, if at all possible, numeric ID indicies are a code smell - use classes instead)
AH, I see. I have some code to removeChild before I created the select/option elements, but I had it omitted for this question for readability. Thanks for the suggestion to use classes, I'll give it a look!
– keven ren
Nov 20 at 11:46
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
The problem is that <option>
s are nested inside of <select>
s, and it's the *<select>
*s which have a selectedIndex
property. So, test.options[test.selectedIndex].value
won't work when test
is an <option>
element. Try using getElementById
to get one of the <select>
s, for one, and then just access its .value
(which is less cumbersome than checking selectedIndex
):
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
dropdown.id = 'select' + i;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("select0");
console.log(test.value);
// same as:
// console.log(test.options[test.selectedIndex].value);
}
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
Also note that duplicate IDs in a single document is invalid HTML, so if you ever call maker
more than once, for your HTML to be valid, you might have a separate counter outside of maker
that gets incremented:
const makeCount = 0;
function maker() {
// ...
dropdown.id = 'select' + makeCount + '_' + i;
// ...
makeCount++;
}
(or, avoid IDs entirely, if at all possible, numeric ID indicies are a code smell - use classes instead)
The problem is that <option>
s are nested inside of <select>
s, and it's the *<select>
*s which have a selectedIndex
property. So, test.options[test.selectedIndex].value
won't work when test
is an <option>
element. Try using getElementById
to get one of the <select>
s, for one, and then just access its .value
(which is less cumbersome than checking selectedIndex
):
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
dropdown.id = 'select' + i;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("select0");
console.log(test.value);
// same as:
// console.log(test.options[test.selectedIndex].value);
}
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
Also note that duplicate IDs in a single document is invalid HTML, so if you ever call maker
more than once, for your HTML to be valid, you might have a separate counter outside of maker
that gets incremented:
const makeCount = 0;
function maker() {
// ...
dropdown.id = 'select' + makeCount + '_' + i;
// ...
makeCount++;
}
(or, avoid IDs entirely, if at all possible, numeric ID indicies are a code smell - use classes instead)
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
dropdown.id = 'select' + i;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("select0");
console.log(test.value);
// same as:
// console.log(test.options[test.selectedIndex].value);
}
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
dropdown.id = 'select' + i;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
var test = document.getElementById("select0");
console.log(test.value);
// same as:
// console.log(test.options[test.selectedIndex].value);
}
<a href="#" id="make" onclick="maker()">create</a>
<a href="#" id="get" onclick="getter()">collect</a>
<div id="box"><br>
answered Nov 20 at 11:31
CertainPerformance
71.5k143453
71.5k143453
AH, I see. I have some code to removeChild before I created the select/option elements, but I had it omitted for this question for readability. Thanks for the suggestion to use classes, I'll give it a look!
– keven ren
Nov 20 at 11:46
add a comment |
AH, I see. I have some code to removeChild before I created the select/option elements, but I had it omitted for this question for readability. Thanks for the suggestion to use classes, I'll give it a look!
– keven ren
Nov 20 at 11:46
AH, I see. I have some code to removeChild before I created the select/option elements, but I had it omitted for this question for readability. Thanks for the suggestion to use classes, I'll give it a look!
– keven ren
Nov 20 at 11:46
AH, I see. I have some code to removeChild before I created the select/option elements, but I had it omitted for this question for readability. Thanks for the suggestion to use classes, I'll give it a look!
– keven ren
Nov 20 at 11:46
add a comment |
up vote
1
down vote
We need to discriminate between option elements and the select element. The select element is the one with which you want to interact most of the time, and the option elements are simply a collection of possible entries for the select element.
Your code as it stands now generates these sort of elements:
<select>
<option value="2" id="option00">2</option>
<option value="3" id="option00">3</option>
<option value="5" id="option00">5</option>
</select>
As you see all the options receive the same ID - which is generally forbidden in HTML documents. You might consider moving the ID indicator to the select element, which also gives you access to the value of the selected option.
Here is the revised JS code with comments before revisions:
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
# Giving the select item an ID instead of each option
dropdown.id = 'select' + i + k;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
option.id = 'option'
console.log(option.id)
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
# Getting the select element instead of the option
var selectElement = document.getElementById("select00");
# The value attribute of the select element is the value of the selected option
console.log(selectElement.value);
}
add a comment |
up vote
1
down vote
We need to discriminate between option elements and the select element. The select element is the one with which you want to interact most of the time, and the option elements are simply a collection of possible entries for the select element.
Your code as it stands now generates these sort of elements:
<select>
<option value="2" id="option00">2</option>
<option value="3" id="option00">3</option>
<option value="5" id="option00">5</option>
</select>
As you see all the options receive the same ID - which is generally forbidden in HTML documents. You might consider moving the ID indicator to the select element, which also gives you access to the value of the selected option.
Here is the revised JS code with comments before revisions:
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
# Giving the select item an ID instead of each option
dropdown.id = 'select' + i + k;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
option.id = 'option'
console.log(option.id)
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
# Getting the select element instead of the option
var selectElement = document.getElementById("select00");
# The value attribute of the select element is the value of the selected option
console.log(selectElement.value);
}
add a comment |
up vote
1
down vote
up vote
1
down vote
We need to discriminate between option elements and the select element. The select element is the one with which you want to interact most of the time, and the option elements are simply a collection of possible entries for the select element.
Your code as it stands now generates these sort of elements:
<select>
<option value="2" id="option00">2</option>
<option value="3" id="option00">3</option>
<option value="5" id="option00">5</option>
</select>
As you see all the options receive the same ID - which is generally forbidden in HTML documents. You might consider moving the ID indicator to the select element, which also gives you access to the value of the selected option.
Here is the revised JS code with comments before revisions:
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
# Giving the select item an ID instead of each option
dropdown.id = 'select' + i + k;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
option.id = 'option'
console.log(option.id)
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
# Getting the select element instead of the option
var selectElement = document.getElementById("select00");
# The value attribute of the select element is the value of the selected option
console.log(selectElement.value);
}
We need to discriminate between option elements and the select element. The select element is the one with which you want to interact most of the time, and the option elements are simply a collection of possible entries for the select element.
Your code as it stands now generates these sort of elements:
<select>
<option value="2" id="option00">2</option>
<option value="3" id="option00">3</option>
<option value="5" id="option00">5</option>
</select>
As you see all the options receive the same ID - which is generally forbidden in HTML documents. You might consider moving the ID indicator to the select element, which also gives you access to the value of the selected option.
Here is the revised JS code with comments before revisions:
var nice = [2, 3, 5];
function maker() {
box.appendChild(document.createElement("br"));
for (i = 0; i < 2; i++) {
box.appendChild(document.createTextNode("test " + (i + 1) + " "));
for (k = 0; k < 2; k++) {
var dropdown = document.createElement("select");
# Giving the select item an ID instead of each option
dropdown.id = 'select' + i + k;
box.appendChild(dropdown);
for (j = 0; j < nice.length; j++) {
var option = document.createElement("option");
option.value = nice[j];
option.text = nice[j];
option.id = 'option'
console.log(option.id)
dropdown.appendChild(option);
}
}
box.appendChild(document.createElement("br"));
}
}
function getter() {
# Getting the select element instead of the option
var selectElement = document.getElementById("select00");
# The value attribute of the select element is the value of the selected option
console.log(selectElement.value);
}
answered Nov 20 at 11:41
Shushan
1,0791614
1,0791614
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391975%2fjavascript-accessing-dynamically-created-element-via-getelementbyid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qq1N6,OK4pqvx7jDHLRrgwCjtM95jlt9 WNWk9Y,B Pt,ohWVYI8578v7LaUJEFDBtEzfq1CtC12d1XX5U1mW6u,nGvg