Not able to make a class in Python and output it to excel
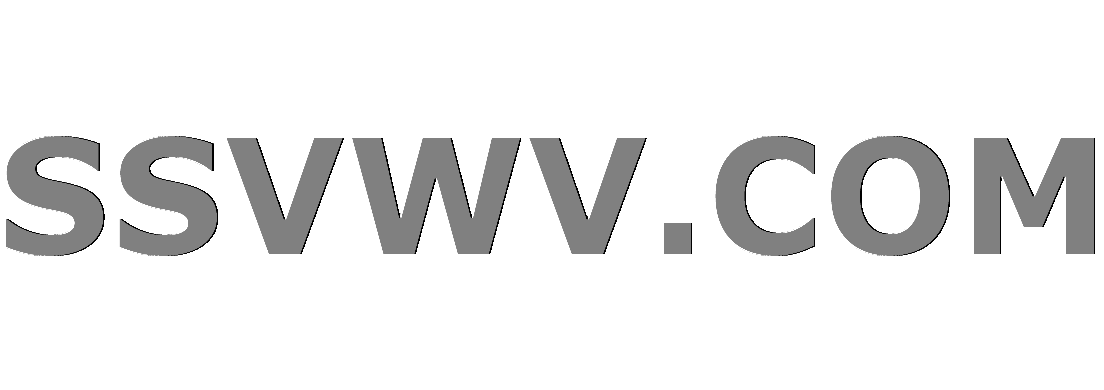
Multi tool use
up vote
1
down vote
favorite
I'm fairly new in Python
and working in a inventory management position.
One important thing in inventory management is calculating the safety stock.
So, this is what I'm trying to achieve.
I have imported a file with 3 columns; FR
, sigma
and LT
for 3 rows. See hereunder the code and the output:
code:
import pandas as pd
df = pd.read_excel("Desktop\TestPython4.xlsx")
xcol=["FR","sigma","LT"]
x=df[xcol].values
output:
snapshot
To calculate the safety stock, I have the following (simplified) formula of it;
CDF(FR)*sigma*sqrt(LT)
where CDF
is the cumulative distribution function of the normal distribution and FR
is a number between 0 and 1 (thus the well-knowned z-value is the output).
I want to output a the file with an extra column that displays the safety stock.
For this I made a class safetystock
with the following code:
class Safetystock:
def __init__(self,FR,sigma,LT):
self.FR = FR
self.sigma = sigma
self.LT = LT
pass
def calculate():
SS=st.norm.ppf(FR)
return print(SS*sigma*np.sqrt(LT))
pass
Then I made the variable: "output"
Output = Safetystock(df.FR,df.sigma,df.LT)
I said that the data in the file needs to be taken into account.
Then I added a column to df
, named output
that needs to contain the variable "Output":
df["output"]=Output
Now, when I want to call df
, it gives me this:
actual output
What am I doing wrong?
Cheers,
Steven
python-3.x inventory-management
add a comment |
up vote
1
down vote
favorite
I'm fairly new in Python
and working in a inventory management position.
One important thing in inventory management is calculating the safety stock.
So, this is what I'm trying to achieve.
I have imported a file with 3 columns; FR
, sigma
and LT
for 3 rows. See hereunder the code and the output:
code:
import pandas as pd
df = pd.read_excel("Desktop\TestPython4.xlsx")
xcol=["FR","sigma","LT"]
x=df[xcol].values
output:
snapshot
To calculate the safety stock, I have the following (simplified) formula of it;
CDF(FR)*sigma*sqrt(LT)
where CDF
is the cumulative distribution function of the normal distribution and FR
is a number between 0 and 1 (thus the well-knowned z-value is the output).
I want to output a the file with an extra column that displays the safety stock.
For this I made a class safetystock
with the following code:
class Safetystock:
def __init__(self,FR,sigma,LT):
self.FR = FR
self.sigma = sigma
self.LT = LT
pass
def calculate():
SS=st.norm.ppf(FR)
return print(SS*sigma*np.sqrt(LT))
pass
Then I made the variable: "output"
Output = Safetystock(df.FR,df.sigma,df.LT)
I said that the data in the file needs to be taken into account.
Then I added a column to df
, named output
that needs to contain the variable "Output":
df["output"]=Output
Now, when I want to call df
, it gives me this:
actual output
What am I doing wrong?
Cheers,
Steven
python-3.x inventory-management
It's working exactly like should be, you are saving class instances on Dataframe as output. What do you mean by variable output? BTW pass on init? what is the reason?
– Tzomas
Nov 20 at 11:27
variable output= output per row (so 3 outputs which displays the safety stock based on the FR, sigma and LT). Pass on init: don't remember it. But even without it doesnt work
– Steven Pauly
Nov 20 at 15:07
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm fairly new in Python
and working in a inventory management position.
One important thing in inventory management is calculating the safety stock.
So, this is what I'm trying to achieve.
I have imported a file with 3 columns; FR
, sigma
and LT
for 3 rows. See hereunder the code and the output:
code:
import pandas as pd
df = pd.read_excel("Desktop\TestPython4.xlsx")
xcol=["FR","sigma","LT"]
x=df[xcol].values
output:
snapshot
To calculate the safety stock, I have the following (simplified) formula of it;
CDF(FR)*sigma*sqrt(LT)
where CDF
is the cumulative distribution function of the normal distribution and FR
is a number between 0 and 1 (thus the well-knowned z-value is the output).
I want to output a the file with an extra column that displays the safety stock.
For this I made a class safetystock
with the following code:
class Safetystock:
def __init__(self,FR,sigma,LT):
self.FR = FR
self.sigma = sigma
self.LT = LT
pass
def calculate():
SS=st.norm.ppf(FR)
return print(SS*sigma*np.sqrt(LT))
pass
Then I made the variable: "output"
Output = Safetystock(df.FR,df.sigma,df.LT)
I said that the data in the file needs to be taken into account.
Then I added a column to df
, named output
that needs to contain the variable "Output":
df["output"]=Output
Now, when I want to call df
, it gives me this:
actual output
What am I doing wrong?
Cheers,
Steven
python-3.x inventory-management
I'm fairly new in Python
and working in a inventory management position.
One important thing in inventory management is calculating the safety stock.
So, this is what I'm trying to achieve.
I have imported a file with 3 columns; FR
, sigma
and LT
for 3 rows. See hereunder the code and the output:
code:
import pandas as pd
df = pd.read_excel("Desktop\TestPython4.xlsx")
xcol=["FR","sigma","LT"]
x=df[xcol].values
output:
snapshot
To calculate the safety stock, I have the following (simplified) formula of it;
CDF(FR)*sigma*sqrt(LT)
where CDF
is the cumulative distribution function of the normal distribution and FR
is a number between 0 and 1 (thus the well-knowned z-value is the output).
I want to output a the file with an extra column that displays the safety stock.
For this I made a class safetystock
with the following code:
class Safetystock:
def __init__(self,FR,sigma,LT):
self.FR = FR
self.sigma = sigma
self.LT = LT
pass
def calculate():
SS=st.norm.ppf(FR)
return print(SS*sigma*np.sqrt(LT))
pass
Then I made the variable: "output"
Output = Safetystock(df.FR,df.sigma,df.LT)
I said that the data in the file needs to be taken into account.
Then I added a column to df
, named output
that needs to contain the variable "Output":
df["output"]=Output
Now, when I want to call df
, it gives me this:
actual output
What am I doing wrong?
Cheers,
Steven
python-3.x inventory-management
python-3.x inventory-management
edited Nov 20 at 13:04
Ned
1,0821422
1,0821422
asked Nov 20 at 11:19
Steven Pauly
428
428
It's working exactly like should be, you are saving class instances on Dataframe as output. What do you mean by variable output? BTW pass on init? what is the reason?
– Tzomas
Nov 20 at 11:27
variable output= output per row (so 3 outputs which displays the safety stock based on the FR, sigma and LT). Pass on init: don't remember it. But even without it doesnt work
– Steven Pauly
Nov 20 at 15:07
add a comment |
It's working exactly like should be, you are saving class instances on Dataframe as output. What do you mean by variable output? BTW pass on init? what is the reason?
– Tzomas
Nov 20 at 11:27
variable output= output per row (so 3 outputs which displays the safety stock based on the FR, sigma and LT). Pass on init: don't remember it. But even without it doesnt work
– Steven Pauly
Nov 20 at 15:07
It's working exactly like should be, you are saving class instances on Dataframe as output. What do you mean by variable output? BTW pass on init? what is the reason?
– Tzomas
Nov 20 at 11:27
It's working exactly like should be, you are saving class instances on Dataframe as output. What do you mean by variable output? BTW pass on init? what is the reason?
– Tzomas
Nov 20 at 11:27
variable output= output per row (so 3 outputs which displays the safety stock based on the FR, sigma and LT). Pass on init: don't remember it. But even without it doesnt work
– Steven Pauly
Nov 20 at 15:07
variable output= output per row (so 3 outputs which displays the safety stock based on the FR, sigma and LT). Pass on init: don't remember it. But even without it doesnt work
– Steven Pauly
Nov 20 at 15:07
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
What about
import pandas as pd
import numpy as np
import scipy.stats as st
df = pd.read_excel("Desktop\TestPython4.xlsx")
df["output"] = st.norm.ppf(df.FR)*df.sigma*np.sqrt(df.LT)
But how do I do it when I want to use a class?
– Steven Pauly
Nov 20 at 14:59
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391885%2fnot-able-to-make-a-class-in-python-and-output-it-to-excel%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
What about
import pandas as pd
import numpy as np
import scipy.stats as st
df = pd.read_excel("Desktop\TestPython4.xlsx")
df["output"] = st.norm.ppf(df.FR)*df.sigma*np.sqrt(df.LT)
But how do I do it when I want to use a class?
– Steven Pauly
Nov 20 at 14:59
add a comment |
up vote
0
down vote
accepted
What about
import pandas as pd
import numpy as np
import scipy.stats as st
df = pd.read_excel("Desktop\TestPython4.xlsx")
df["output"] = st.norm.ppf(df.FR)*df.sigma*np.sqrt(df.LT)
But how do I do it when I want to use a class?
– Steven Pauly
Nov 20 at 14:59
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
What about
import pandas as pd
import numpy as np
import scipy.stats as st
df = pd.read_excel("Desktop\TestPython4.xlsx")
df["output"] = st.norm.ppf(df.FR)*df.sigma*np.sqrt(df.LT)
What about
import pandas as pd
import numpy as np
import scipy.stats as st
df = pd.read_excel("Desktop\TestPython4.xlsx")
df["output"] = st.norm.ppf(df.FR)*df.sigma*np.sqrt(df.LT)
answered Nov 20 at 11:30
Nicolas
969
969
But how do I do it when I want to use a class?
– Steven Pauly
Nov 20 at 14:59
add a comment |
But how do I do it when I want to use a class?
– Steven Pauly
Nov 20 at 14:59
But how do I do it when I want to use a class?
– Steven Pauly
Nov 20 at 14:59
But how do I do it when I want to use a class?
– Steven Pauly
Nov 20 at 14:59
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391885%2fnot-able-to-make-a-class-in-python-and-output-it-to-excel%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Xi0nGEAwHTiM4x4VI,tt9sn4
It's working exactly like should be, you are saving class instances on Dataframe as output. What do you mean by variable output? BTW pass on init? what is the reason?
– Tzomas
Nov 20 at 11:27
variable output= output per row (so 3 outputs which displays the safety stock based on the FR, sigma and LT). Pass on init: don't remember it. But even without it doesnt work
– Steven Pauly
Nov 20 at 15:07