Combinations of unique products with itertools
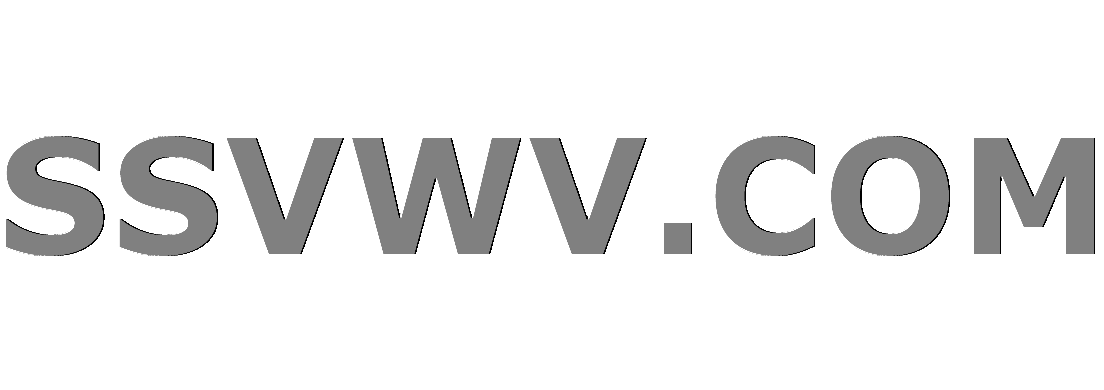
Multi tool use
up vote
1
down vote
favorite
I have a nested list and would like to make a product of two items.
test = [[('juice', 'NOUN'), ('orange', 'FLAVOR')],
[('juice', 'NOUN'), ('orange', 'FLAVOR'), ('lemon', 'FLAVOR')],
[('orange', 'FLAVOR'), ('chip', 'NOUN')]]
What I expect is something like this:
[(('juice', 'NOUN'), ('lemon', 'FLAVOR')),
(('juice', 'NOUN'), ('chip', 'NOUN')),
(('orange', 'FLAVOR'), ('lemon', 'FLAVOR')),
(('orange', 'FLAVOR'), ('chip', 'NOUN')),
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))]
That is to say, I would like to get the permutation across lists but only for unique items. I prefer to use itertools
. Previously, I tried list(itertools.product(*test))
But I realized it would produce the product of the length of a nested list...
My current code:
unique_list = list(set(itertools.chain(*test)))
list(itertools.combinations(unique_list, 2))
My thought process is to get the unique items in the nested list first, so the nested list will be [[('juice', 'NOUN'), ('orange', 'FLAVOR')], [('lemon', 'FLAVOR')], [('chip', 'NOUN')]]
and then use the itertools.combinations
to permute. Yet, it will permute within the list (i.e. juice and orange appear together), which I do not want in my results.
python list permutation itertools
|
show 2 more comments
up vote
1
down vote
favorite
I have a nested list and would like to make a product of two items.
test = [[('juice', 'NOUN'), ('orange', 'FLAVOR')],
[('juice', 'NOUN'), ('orange', 'FLAVOR'), ('lemon', 'FLAVOR')],
[('orange', 'FLAVOR'), ('chip', 'NOUN')]]
What I expect is something like this:
[(('juice', 'NOUN'), ('lemon', 'FLAVOR')),
(('juice', 'NOUN'), ('chip', 'NOUN')),
(('orange', 'FLAVOR'), ('lemon', 'FLAVOR')),
(('orange', 'FLAVOR'), ('chip', 'NOUN')),
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))]
That is to say, I would like to get the permutation across lists but only for unique items. I prefer to use itertools
. Previously, I tried list(itertools.product(*test))
But I realized it would produce the product of the length of a nested list...
My current code:
unique_list = list(set(itertools.chain(*test)))
list(itertools.combinations(unique_list, 2))
My thought process is to get the unique items in the nested list first, so the nested list will be [[('juice', 'NOUN'), ('orange', 'FLAVOR')], [('lemon', 'FLAVOR')], [('chip', 'NOUN')]]
and then use the itertools.combinations
to permute. Yet, it will permute within the list (i.e. juice and orange appear together), which I do not want in my results.
python list permutation itertools
why do you not have(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
in your results?
– Ev. Kounis
Nov 20 at 15:59
@Ev. Kounis oh! Thanks for pointing out! I want that in my results. I just edited my question
– Abbey
Nov 20 at 16:00
Have you tried anything so far? Any code you could show?
– plocks
Nov 20 at 16:02
is the length of the original list fixed?
– Ev. Kounis
Nov 20 at 16:03
I don't understand why(('juice', 'NOUN'), ('orange', 'FLAVOR'))
isn't part of the expected output. It (and its reverse) can be made a whole bunch of ways from your three input lists.
– Blckknght
Nov 21 at 7:54
|
show 2 more comments
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a nested list and would like to make a product of two items.
test = [[('juice', 'NOUN'), ('orange', 'FLAVOR')],
[('juice', 'NOUN'), ('orange', 'FLAVOR'), ('lemon', 'FLAVOR')],
[('orange', 'FLAVOR'), ('chip', 'NOUN')]]
What I expect is something like this:
[(('juice', 'NOUN'), ('lemon', 'FLAVOR')),
(('juice', 'NOUN'), ('chip', 'NOUN')),
(('orange', 'FLAVOR'), ('lemon', 'FLAVOR')),
(('orange', 'FLAVOR'), ('chip', 'NOUN')),
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))]
That is to say, I would like to get the permutation across lists but only for unique items. I prefer to use itertools
. Previously, I tried list(itertools.product(*test))
But I realized it would produce the product of the length of a nested list...
My current code:
unique_list = list(set(itertools.chain(*test)))
list(itertools.combinations(unique_list, 2))
My thought process is to get the unique items in the nested list first, so the nested list will be [[('juice', 'NOUN'), ('orange', 'FLAVOR')], [('lemon', 'FLAVOR')], [('chip', 'NOUN')]]
and then use the itertools.combinations
to permute. Yet, it will permute within the list (i.e. juice and orange appear together), which I do not want in my results.
python list permutation itertools
I have a nested list and would like to make a product of two items.
test = [[('juice', 'NOUN'), ('orange', 'FLAVOR')],
[('juice', 'NOUN'), ('orange', 'FLAVOR'), ('lemon', 'FLAVOR')],
[('orange', 'FLAVOR'), ('chip', 'NOUN')]]
What I expect is something like this:
[(('juice', 'NOUN'), ('lemon', 'FLAVOR')),
(('juice', 'NOUN'), ('chip', 'NOUN')),
(('orange', 'FLAVOR'), ('lemon', 'FLAVOR')),
(('orange', 'FLAVOR'), ('chip', 'NOUN')),
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))]
That is to say, I would like to get the permutation across lists but only for unique items. I prefer to use itertools
. Previously, I tried list(itertools.product(*test))
But I realized it would produce the product of the length of a nested list...
My current code:
unique_list = list(set(itertools.chain(*test)))
list(itertools.combinations(unique_list, 2))
My thought process is to get the unique items in the nested list first, so the nested list will be [[('juice', 'NOUN'), ('orange', 'FLAVOR')], [('lemon', 'FLAVOR')], [('chip', 'NOUN')]]
and then use the itertools.combinations
to permute. Yet, it will permute within the list (i.e. juice and orange appear together), which I do not want in my results.
python list permutation itertools
python list permutation itertools
edited Nov 21 at 7:43


Ev. Kounis
10.5k21545
10.5k21545
asked Nov 20 at 15:52
Abbey
727
727
why do you not have(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
in your results?
– Ev. Kounis
Nov 20 at 15:59
@Ev. Kounis oh! Thanks for pointing out! I want that in my results. I just edited my question
– Abbey
Nov 20 at 16:00
Have you tried anything so far? Any code you could show?
– plocks
Nov 20 at 16:02
is the length of the original list fixed?
– Ev. Kounis
Nov 20 at 16:03
I don't understand why(('juice', 'NOUN'), ('orange', 'FLAVOR'))
isn't part of the expected output. It (and its reverse) can be made a whole bunch of ways from your three input lists.
– Blckknght
Nov 21 at 7:54
|
show 2 more comments
why do you not have(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
in your results?
– Ev. Kounis
Nov 20 at 15:59
@Ev. Kounis oh! Thanks for pointing out! I want that in my results. I just edited my question
– Abbey
Nov 20 at 16:00
Have you tried anything so far? Any code you could show?
– plocks
Nov 20 at 16:02
is the length of the original list fixed?
– Ev. Kounis
Nov 20 at 16:03
I don't understand why(('juice', 'NOUN'), ('orange', 'FLAVOR'))
isn't part of the expected output. It (and its reverse) can be made a whole bunch of ways from your three input lists.
– Blckknght
Nov 21 at 7:54
why do you not have
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
in your results?– Ev. Kounis
Nov 20 at 15:59
why do you not have
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
in your results?– Ev. Kounis
Nov 20 at 15:59
@Ev. Kounis oh! Thanks for pointing out! I want that in my results. I just edited my question
– Abbey
Nov 20 at 16:00
@Ev. Kounis oh! Thanks for pointing out! I want that in my results. I just edited my question
– Abbey
Nov 20 at 16:00
Have you tried anything so far? Any code you could show?
– plocks
Nov 20 at 16:02
Have you tried anything so far? Any code you could show?
– plocks
Nov 20 at 16:02
is the length of the original list fixed?
– Ev. Kounis
Nov 20 at 16:03
is the length of the original list fixed?
– Ev. Kounis
Nov 20 at 16:03
I don't understand why
(('juice', 'NOUN'), ('orange', 'FLAVOR'))
isn't part of the expected output. It (and its reverse) can be made a whole bunch of ways from your three input lists.– Blckknght
Nov 21 at 7:54
I don't understand why
(('juice', 'NOUN'), ('orange', 'FLAVOR'))
isn't part of the expected output. It (and its reverse) can be made a whole bunch of ways from your three input lists.– Blckknght
Nov 21 at 7:54
|
show 2 more comments
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
This does what you want without fixing the size of the original list to 3:
Input:
test = [[('juice', 'NOUN'), ('orange', 'FLAVOR')],
[('juice', 'NOUN'), ('orange', 'FLAVOR'), ('lemon', 'FLAVOR')],
[('juice', 'NOUN'), ('chip', 'NOUN')]]
First, reformat input to remove duplicates (see note 1):
test = [[x for x in sublist if x not in sum(test[:i], )] for i, sublist in enumerate(test)]
Finally, get the product of the combinations.
from itertools import combinations, product
for c in combinations(test, 2):
for x in product(*c):
print(x)
which produces:
(('juice', 'NOUN'), ('lemon', 'FLAVOR'))
(('orange', 'FLAVOR'), ('lemon', 'FLAVOR'))
(('juice', 'NOUN'), ('chip', 'NOUN'))
(('orange', 'FLAVOR'), ('chip', 'NOUN'))
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
- removes inner tuples if they were seen in any of the previous sublists. The magic here is done by the
sum(test[:i], )
which "adds" all the previous sublists together to perform one membership check only.
There is also a list-comprehension version of the above for compactness and style-points:
res = [x for c in combinations(test, 2) for x in product(*c)]
I tested all the solutions you suggested and they worked like magic! Thanks
– Abbey
Nov 20 at 16:17
1
@Abbey Cheers! it was an interesting problem.
– Ev. Kounis
Nov 20 at 16:19
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53396772%2fcombinations-of-unique-products-with-itertools%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
This does what you want without fixing the size of the original list to 3:
Input:
test = [[('juice', 'NOUN'), ('orange', 'FLAVOR')],
[('juice', 'NOUN'), ('orange', 'FLAVOR'), ('lemon', 'FLAVOR')],
[('juice', 'NOUN'), ('chip', 'NOUN')]]
First, reformat input to remove duplicates (see note 1):
test = [[x for x in sublist if x not in sum(test[:i], )] for i, sublist in enumerate(test)]
Finally, get the product of the combinations.
from itertools import combinations, product
for c in combinations(test, 2):
for x in product(*c):
print(x)
which produces:
(('juice', 'NOUN'), ('lemon', 'FLAVOR'))
(('orange', 'FLAVOR'), ('lemon', 'FLAVOR'))
(('juice', 'NOUN'), ('chip', 'NOUN'))
(('orange', 'FLAVOR'), ('chip', 'NOUN'))
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
- removes inner tuples if they were seen in any of the previous sublists. The magic here is done by the
sum(test[:i], )
which "adds" all the previous sublists together to perform one membership check only.
There is also a list-comprehension version of the above for compactness and style-points:
res = [x for c in combinations(test, 2) for x in product(*c)]
I tested all the solutions you suggested and they worked like magic! Thanks
– Abbey
Nov 20 at 16:17
1
@Abbey Cheers! it was an interesting problem.
– Ev. Kounis
Nov 20 at 16:19
add a comment |
up vote
1
down vote
accepted
This does what you want without fixing the size of the original list to 3:
Input:
test = [[('juice', 'NOUN'), ('orange', 'FLAVOR')],
[('juice', 'NOUN'), ('orange', 'FLAVOR'), ('lemon', 'FLAVOR')],
[('juice', 'NOUN'), ('chip', 'NOUN')]]
First, reformat input to remove duplicates (see note 1):
test = [[x for x in sublist if x not in sum(test[:i], )] for i, sublist in enumerate(test)]
Finally, get the product of the combinations.
from itertools import combinations, product
for c in combinations(test, 2):
for x in product(*c):
print(x)
which produces:
(('juice', 'NOUN'), ('lemon', 'FLAVOR'))
(('orange', 'FLAVOR'), ('lemon', 'FLAVOR'))
(('juice', 'NOUN'), ('chip', 'NOUN'))
(('orange', 'FLAVOR'), ('chip', 'NOUN'))
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
- removes inner tuples if they were seen in any of the previous sublists. The magic here is done by the
sum(test[:i], )
which "adds" all the previous sublists together to perform one membership check only.
There is also a list-comprehension version of the above for compactness and style-points:
res = [x for c in combinations(test, 2) for x in product(*c)]
I tested all the solutions you suggested and they worked like magic! Thanks
– Abbey
Nov 20 at 16:17
1
@Abbey Cheers! it was an interesting problem.
– Ev. Kounis
Nov 20 at 16:19
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
This does what you want without fixing the size of the original list to 3:
Input:
test = [[('juice', 'NOUN'), ('orange', 'FLAVOR')],
[('juice', 'NOUN'), ('orange', 'FLAVOR'), ('lemon', 'FLAVOR')],
[('juice', 'NOUN'), ('chip', 'NOUN')]]
First, reformat input to remove duplicates (see note 1):
test = [[x for x in sublist if x not in sum(test[:i], )] for i, sublist in enumerate(test)]
Finally, get the product of the combinations.
from itertools import combinations, product
for c in combinations(test, 2):
for x in product(*c):
print(x)
which produces:
(('juice', 'NOUN'), ('lemon', 'FLAVOR'))
(('orange', 'FLAVOR'), ('lemon', 'FLAVOR'))
(('juice', 'NOUN'), ('chip', 'NOUN'))
(('orange', 'FLAVOR'), ('chip', 'NOUN'))
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
- removes inner tuples if they were seen in any of the previous sublists. The magic here is done by the
sum(test[:i], )
which "adds" all the previous sublists together to perform one membership check only.
There is also a list-comprehension version of the above for compactness and style-points:
res = [x for c in combinations(test, 2) for x in product(*c)]
This does what you want without fixing the size of the original list to 3:
Input:
test = [[('juice', 'NOUN'), ('orange', 'FLAVOR')],
[('juice', 'NOUN'), ('orange', 'FLAVOR'), ('lemon', 'FLAVOR')],
[('juice', 'NOUN'), ('chip', 'NOUN')]]
First, reformat input to remove duplicates (see note 1):
test = [[x for x in sublist if x not in sum(test[:i], )] for i, sublist in enumerate(test)]
Finally, get the product of the combinations.
from itertools import combinations, product
for c in combinations(test, 2):
for x in product(*c):
print(x)
which produces:
(('juice', 'NOUN'), ('lemon', 'FLAVOR'))
(('orange', 'FLAVOR'), ('lemon', 'FLAVOR'))
(('juice', 'NOUN'), ('chip', 'NOUN'))
(('orange', 'FLAVOR'), ('chip', 'NOUN'))
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
- removes inner tuples if they were seen in any of the previous sublists. The magic here is done by the
sum(test[:i], )
which "adds" all the previous sublists together to perform one membership check only.
There is also a list-comprehension version of the above for compactness and style-points:
res = [x for c in combinations(test, 2) for x in product(*c)]
edited Nov 20 at 16:11
answered Nov 20 at 16:05


Ev. Kounis
10.5k21545
10.5k21545
I tested all the solutions you suggested and they worked like magic! Thanks
– Abbey
Nov 20 at 16:17
1
@Abbey Cheers! it was an interesting problem.
– Ev. Kounis
Nov 20 at 16:19
add a comment |
I tested all the solutions you suggested and they worked like magic! Thanks
– Abbey
Nov 20 at 16:17
1
@Abbey Cheers! it was an interesting problem.
– Ev. Kounis
Nov 20 at 16:19
I tested all the solutions you suggested and they worked like magic! Thanks
– Abbey
Nov 20 at 16:17
I tested all the solutions you suggested and they worked like magic! Thanks
– Abbey
Nov 20 at 16:17
1
1
@Abbey Cheers! it was an interesting problem.
– Ev. Kounis
Nov 20 at 16:19
@Abbey Cheers! it was an interesting problem.
– Ev. Kounis
Nov 20 at 16:19
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53396772%2fcombinations-of-unique-products-with-itertools%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
i9qMX pL,2aO8ZiQzoE,mnQjgYv UNUA y w Nq,HSxVKyjFJ8fNfnNbJ FiJ 5rQnirOhxJDZr CcKXhwG0LkQ
why do you not have
(('lemon', 'FLAVOR'), ('chip', 'NOUN'))
in your results?– Ev. Kounis
Nov 20 at 15:59
@Ev. Kounis oh! Thanks for pointing out! I want that in my results. I just edited my question
– Abbey
Nov 20 at 16:00
Have you tried anything so far? Any code you could show?
– plocks
Nov 20 at 16:02
is the length of the original list fixed?
– Ev. Kounis
Nov 20 at 16:03
I don't understand why
(('juice', 'NOUN'), ('orange', 'FLAVOR'))
isn't part of the expected output. It (and its reverse) can be made a whole bunch of ways from your three input lists.– Blckknght
Nov 21 at 7:54