QFileInfo size() is returning shortcut TARGET size
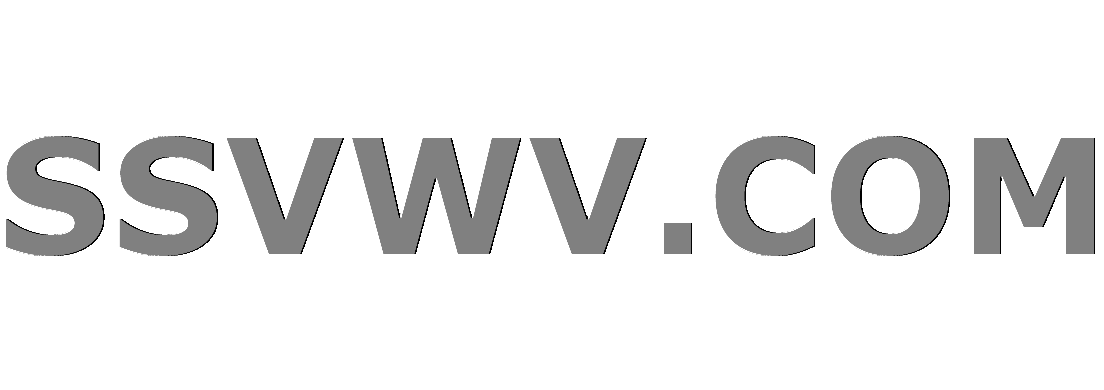
Multi tool use
I am scanning folder size like this:
qint64 dirSize = 0;
int fileCount = 0;
for(QDirIterator itDir(someDir, QDir::NoDotAndDotDot|QDir::Files|QDir::Hidden|QDir::System,
QDirIterator::Subdirectories);
itDir.hasNext(); )
{
itDir.next();
dirSize += itDir.fileInfo().size();
++fileCount;
}
This appears to work fine.
However, I noticed that a folder containing Windows shortcuts (.lnk
) is returning a much larger size than expected. The reason is that the sizes of the shortcut targets are being returned, rather than the sizes of the shortcut files themselves.
But according to QFileInfo documentation:
On Windows, symlinks (shortcuts) are .lnk files. The reported size() is that of the symlink (not the link's target) [...]
So my question is: what am I doing wrong here? How do I get the size of the shortcut file?
c++ qt desktop-shortcut qfileinfo
add a comment |
I am scanning folder size like this:
qint64 dirSize = 0;
int fileCount = 0;
for(QDirIterator itDir(someDir, QDir::NoDotAndDotDot|QDir::Files|QDir::Hidden|QDir::System,
QDirIterator::Subdirectories);
itDir.hasNext(); )
{
itDir.next();
dirSize += itDir.fileInfo().size();
++fileCount;
}
This appears to work fine.
However, I noticed that a folder containing Windows shortcuts (.lnk
) is returning a much larger size than expected. The reason is that the sizes of the shortcut targets are being returned, rather than the sizes of the shortcut files themselves.
But according to QFileInfo documentation:
On Windows, symlinks (shortcuts) are .lnk files. The reported size() is that of the symlink (not the link's target) [...]
So my question is: what am I doing wrong here? How do I get the size of the shortcut file?
c++ qt desktop-shortcut qfileinfo
Is this really a .lnk file? Perhaps you are using a different kind of shortcut.
– drescherjm
Nov 21 '18 at 12:27
Yes, these are definitely Windows shortcut (.lnk
) files.
– bur
Nov 21 '18 at 12:29
In those cases whereitDir
should refer to a link, what is returned byitDir.filePath()
(oritDir.fileInfo().filePath()
) -- is it the path of the link itself or the path of the target?
– G.M.
Nov 21 '18 at 12:32
Both return the path of the link itself as expected.
– bur
Nov 21 '18 at 12:44
add a comment |
I am scanning folder size like this:
qint64 dirSize = 0;
int fileCount = 0;
for(QDirIterator itDir(someDir, QDir::NoDotAndDotDot|QDir::Files|QDir::Hidden|QDir::System,
QDirIterator::Subdirectories);
itDir.hasNext(); )
{
itDir.next();
dirSize += itDir.fileInfo().size();
++fileCount;
}
This appears to work fine.
However, I noticed that a folder containing Windows shortcuts (.lnk
) is returning a much larger size than expected. The reason is that the sizes of the shortcut targets are being returned, rather than the sizes of the shortcut files themselves.
But according to QFileInfo documentation:
On Windows, symlinks (shortcuts) are .lnk files. The reported size() is that of the symlink (not the link's target) [...]
So my question is: what am I doing wrong here? How do I get the size of the shortcut file?
c++ qt desktop-shortcut qfileinfo
I am scanning folder size like this:
qint64 dirSize = 0;
int fileCount = 0;
for(QDirIterator itDir(someDir, QDir::NoDotAndDotDot|QDir::Files|QDir::Hidden|QDir::System,
QDirIterator::Subdirectories);
itDir.hasNext(); )
{
itDir.next();
dirSize += itDir.fileInfo().size();
++fileCount;
}
This appears to work fine.
However, I noticed that a folder containing Windows shortcuts (.lnk
) is returning a much larger size than expected. The reason is that the sizes of the shortcut targets are being returned, rather than the sizes of the shortcut files themselves.
But according to QFileInfo documentation:
On Windows, symlinks (shortcuts) are .lnk files. The reported size() is that of the symlink (not the link's target) [...]
So my question is: what am I doing wrong here? How do I get the size of the shortcut file?
c++ qt desktop-shortcut qfileinfo
c++ qt desktop-shortcut qfileinfo
edited Nov 21 '18 at 13:27
asked Nov 21 '18 at 12:19
bur
17713
17713
Is this really a .lnk file? Perhaps you are using a different kind of shortcut.
– drescherjm
Nov 21 '18 at 12:27
Yes, these are definitely Windows shortcut (.lnk
) files.
– bur
Nov 21 '18 at 12:29
In those cases whereitDir
should refer to a link, what is returned byitDir.filePath()
(oritDir.fileInfo().filePath()
) -- is it the path of the link itself or the path of the target?
– G.M.
Nov 21 '18 at 12:32
Both return the path of the link itself as expected.
– bur
Nov 21 '18 at 12:44
add a comment |
Is this really a .lnk file? Perhaps you are using a different kind of shortcut.
– drescherjm
Nov 21 '18 at 12:27
Yes, these are definitely Windows shortcut (.lnk
) files.
– bur
Nov 21 '18 at 12:29
In those cases whereitDir
should refer to a link, what is returned byitDir.filePath()
(oritDir.fileInfo().filePath()
) -- is it the path of the link itself or the path of the target?
– G.M.
Nov 21 '18 at 12:32
Both return the path of the link itself as expected.
– bur
Nov 21 '18 at 12:44
Is this really a .lnk file? Perhaps you are using a different kind of shortcut.
– drescherjm
Nov 21 '18 at 12:27
Is this really a .lnk file? Perhaps you are using a different kind of shortcut.
– drescherjm
Nov 21 '18 at 12:27
Yes, these are definitely Windows shortcut (
.lnk
) files.– bur
Nov 21 '18 at 12:29
Yes, these are definitely Windows shortcut (
.lnk
) files.– bur
Nov 21 '18 at 12:29
In those cases where
itDir
should refer to a link, what is returned by itDir.filePath()
(or itDir.fileInfo().filePath()
) -- is it the path of the link itself or the path of the target?– G.M.
Nov 21 '18 at 12:32
In those cases where
itDir
should refer to a link, what is returned by itDir.filePath()
(or itDir.fileInfo().filePath()
) -- is it the path of the link itself or the path of the target?– G.M.
Nov 21 '18 at 12:32
Both return the path of the link itself as expected.
– bur
Nov 21 '18 at 12:44
Both return the path of the link itself as expected.
– bur
Nov 21 '18 at 12:44
add a comment |
2 Answers
2
active
oldest
votes
For testing purposes I created a shortcut of one of the Qt's DLL files. I placed this shortcut into an empty folder. I also created a shortcut of Qt's sdktool.exe and placed this into the same folder.
I also noticed that the size() returns the size of the actual file and not the size of shortcut. I remember I had somewhat similiar behaviour in my old project and what I did was that I opened the file before reading the size.
for (QDirIterator itr(someDir, QDir::NoDotAndDotDot|QDir::Files|QDir::Hidden|QDir::System,
QDirIterator::Subdirectories); itr.hasNext();) {
itr.next();
// Shows wrong size
qDebug() << itr.fileName() << ", size (unopened): " << itr.fileInfo().size();
QFile file(itr.filePath());
if (file.exists() && file.open(QIODevice::ReadOnly)) {
// Now the size shows correctly
qDebug() << "Size when opened: " << file.size();
file.close();
}
}
Outputs:
"sdktool.lnk" , size (unopened): 2817024
Size when opened: 1325
"test.lnk" , size (unopened): 4429312
Size when opened: 951
Windows 10's File Property window shows that the size of "test.lnk" is 951 bytes and the size of "sdktool.lnk" is 1325 bytes.
1
Cool, this seems to work. I've implemented this, but first I check ifitr.fileInfo().isSymLink()
and then iffile.size() == 0
I additr.fileInfo().size()
anyway.
– bur
Nov 21 '18 at 13:50
Good catch! I forgot thatQFileInfo::isSymLink()
exists :)
– Rob
Nov 21 '18 at 13:58
I've found that this approach doesn't work for shortcuts with an invalid/nonexistant target. It will return 0 in both cases.
– bur
Dec 1 '18 at 1:57
add a comment |
@Rob's answer works in most cases, but returns 0 when the the shortcut's target doesn't exist/is invalid.
Taking a cue from that approach, you can also copy the shortcut and change the extension.
So combining it all into a function (I'm assuming here that opening the target is cheaper/safer than copying the shortcut):
qint64 getFileSize(const QString &path)
{
qint64 size = 0;
QFileInfo fileInfo(path);
if(fileInfo.isSymLink() && fileInfo.size() == QFileInfo(fileInfo.symLinkTarget()).size())
{
// Try this approach first
QFile file(path);
if(file.exists() && file.open(QIODevice::ReadOnly))
size = file.size();
file.close();
// If that didn't work, try this
if(size == 0)
{
QString tmpPath = path+".tmp";
for(int i=2; QFileInfo().exists(tmpPath); ++i) // Make sure filename is unique
tmpPath = path+".tmp"+QString::number(i);
if(QFile::copy(path, tmpPath))
{
size = QFileInfo(tmpPath).size();
QFile::remove(tmpPath);
}
}
}
else size = fileInfo.size();
return size;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53411886%2fqfileinfo-size-is-returning-shortcut-target-size%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
For testing purposes I created a shortcut of one of the Qt's DLL files. I placed this shortcut into an empty folder. I also created a shortcut of Qt's sdktool.exe and placed this into the same folder.
I also noticed that the size() returns the size of the actual file and not the size of shortcut. I remember I had somewhat similiar behaviour in my old project and what I did was that I opened the file before reading the size.
for (QDirIterator itr(someDir, QDir::NoDotAndDotDot|QDir::Files|QDir::Hidden|QDir::System,
QDirIterator::Subdirectories); itr.hasNext();) {
itr.next();
// Shows wrong size
qDebug() << itr.fileName() << ", size (unopened): " << itr.fileInfo().size();
QFile file(itr.filePath());
if (file.exists() && file.open(QIODevice::ReadOnly)) {
// Now the size shows correctly
qDebug() << "Size when opened: " << file.size();
file.close();
}
}
Outputs:
"sdktool.lnk" , size (unopened): 2817024
Size when opened: 1325
"test.lnk" , size (unopened): 4429312
Size when opened: 951
Windows 10's File Property window shows that the size of "test.lnk" is 951 bytes and the size of "sdktool.lnk" is 1325 bytes.
1
Cool, this seems to work. I've implemented this, but first I check ifitr.fileInfo().isSymLink()
and then iffile.size() == 0
I additr.fileInfo().size()
anyway.
– bur
Nov 21 '18 at 13:50
Good catch! I forgot thatQFileInfo::isSymLink()
exists :)
– Rob
Nov 21 '18 at 13:58
I've found that this approach doesn't work for shortcuts with an invalid/nonexistant target. It will return 0 in both cases.
– bur
Dec 1 '18 at 1:57
add a comment |
For testing purposes I created a shortcut of one of the Qt's DLL files. I placed this shortcut into an empty folder. I also created a shortcut of Qt's sdktool.exe and placed this into the same folder.
I also noticed that the size() returns the size of the actual file and not the size of shortcut. I remember I had somewhat similiar behaviour in my old project and what I did was that I opened the file before reading the size.
for (QDirIterator itr(someDir, QDir::NoDotAndDotDot|QDir::Files|QDir::Hidden|QDir::System,
QDirIterator::Subdirectories); itr.hasNext();) {
itr.next();
// Shows wrong size
qDebug() << itr.fileName() << ", size (unopened): " << itr.fileInfo().size();
QFile file(itr.filePath());
if (file.exists() && file.open(QIODevice::ReadOnly)) {
// Now the size shows correctly
qDebug() << "Size when opened: " << file.size();
file.close();
}
}
Outputs:
"sdktool.lnk" , size (unopened): 2817024
Size when opened: 1325
"test.lnk" , size (unopened): 4429312
Size when opened: 951
Windows 10's File Property window shows that the size of "test.lnk" is 951 bytes and the size of "sdktool.lnk" is 1325 bytes.
1
Cool, this seems to work. I've implemented this, but first I check ifitr.fileInfo().isSymLink()
and then iffile.size() == 0
I additr.fileInfo().size()
anyway.
– bur
Nov 21 '18 at 13:50
Good catch! I forgot thatQFileInfo::isSymLink()
exists :)
– Rob
Nov 21 '18 at 13:58
I've found that this approach doesn't work for shortcuts with an invalid/nonexistant target. It will return 0 in both cases.
– bur
Dec 1 '18 at 1:57
add a comment |
For testing purposes I created a shortcut of one of the Qt's DLL files. I placed this shortcut into an empty folder. I also created a shortcut of Qt's sdktool.exe and placed this into the same folder.
I also noticed that the size() returns the size of the actual file and not the size of shortcut. I remember I had somewhat similiar behaviour in my old project and what I did was that I opened the file before reading the size.
for (QDirIterator itr(someDir, QDir::NoDotAndDotDot|QDir::Files|QDir::Hidden|QDir::System,
QDirIterator::Subdirectories); itr.hasNext();) {
itr.next();
// Shows wrong size
qDebug() << itr.fileName() << ", size (unopened): " << itr.fileInfo().size();
QFile file(itr.filePath());
if (file.exists() && file.open(QIODevice::ReadOnly)) {
// Now the size shows correctly
qDebug() << "Size when opened: " << file.size();
file.close();
}
}
Outputs:
"sdktool.lnk" , size (unopened): 2817024
Size when opened: 1325
"test.lnk" , size (unopened): 4429312
Size when opened: 951
Windows 10's File Property window shows that the size of "test.lnk" is 951 bytes and the size of "sdktool.lnk" is 1325 bytes.
For testing purposes I created a shortcut of one of the Qt's DLL files. I placed this shortcut into an empty folder. I also created a shortcut of Qt's sdktool.exe and placed this into the same folder.
I also noticed that the size() returns the size of the actual file and not the size of shortcut. I remember I had somewhat similiar behaviour in my old project and what I did was that I opened the file before reading the size.
for (QDirIterator itr(someDir, QDir::NoDotAndDotDot|QDir::Files|QDir::Hidden|QDir::System,
QDirIterator::Subdirectories); itr.hasNext();) {
itr.next();
// Shows wrong size
qDebug() << itr.fileName() << ", size (unopened): " << itr.fileInfo().size();
QFile file(itr.filePath());
if (file.exists() && file.open(QIODevice::ReadOnly)) {
// Now the size shows correctly
qDebug() << "Size when opened: " << file.size();
file.close();
}
}
Outputs:
"sdktool.lnk" , size (unopened): 2817024
Size when opened: 1325
"test.lnk" , size (unopened): 4429312
Size when opened: 951
Windows 10's File Property window shows that the size of "test.lnk" is 951 bytes and the size of "sdktool.lnk" is 1325 bytes.
answered Nov 21 '18 at 13:30
Rob
718
718
1
Cool, this seems to work. I've implemented this, but first I check ifitr.fileInfo().isSymLink()
and then iffile.size() == 0
I additr.fileInfo().size()
anyway.
– bur
Nov 21 '18 at 13:50
Good catch! I forgot thatQFileInfo::isSymLink()
exists :)
– Rob
Nov 21 '18 at 13:58
I've found that this approach doesn't work for shortcuts with an invalid/nonexistant target. It will return 0 in both cases.
– bur
Dec 1 '18 at 1:57
add a comment |
1
Cool, this seems to work. I've implemented this, but first I check ifitr.fileInfo().isSymLink()
and then iffile.size() == 0
I additr.fileInfo().size()
anyway.
– bur
Nov 21 '18 at 13:50
Good catch! I forgot thatQFileInfo::isSymLink()
exists :)
– Rob
Nov 21 '18 at 13:58
I've found that this approach doesn't work for shortcuts with an invalid/nonexistant target. It will return 0 in both cases.
– bur
Dec 1 '18 at 1:57
1
1
Cool, this seems to work. I've implemented this, but first I check if
itr.fileInfo().isSymLink()
and then if file.size() == 0
I add itr.fileInfo().size()
anyway.– bur
Nov 21 '18 at 13:50
Cool, this seems to work. I've implemented this, but first I check if
itr.fileInfo().isSymLink()
and then if file.size() == 0
I add itr.fileInfo().size()
anyway.– bur
Nov 21 '18 at 13:50
Good catch! I forgot that
QFileInfo::isSymLink()
exists :)– Rob
Nov 21 '18 at 13:58
Good catch! I forgot that
QFileInfo::isSymLink()
exists :)– Rob
Nov 21 '18 at 13:58
I've found that this approach doesn't work for shortcuts with an invalid/nonexistant target. It will return 0 in both cases.
– bur
Dec 1 '18 at 1:57
I've found that this approach doesn't work for shortcuts with an invalid/nonexistant target. It will return 0 in both cases.
– bur
Dec 1 '18 at 1:57
add a comment |
@Rob's answer works in most cases, but returns 0 when the the shortcut's target doesn't exist/is invalid.
Taking a cue from that approach, you can also copy the shortcut and change the extension.
So combining it all into a function (I'm assuming here that opening the target is cheaper/safer than copying the shortcut):
qint64 getFileSize(const QString &path)
{
qint64 size = 0;
QFileInfo fileInfo(path);
if(fileInfo.isSymLink() && fileInfo.size() == QFileInfo(fileInfo.symLinkTarget()).size())
{
// Try this approach first
QFile file(path);
if(file.exists() && file.open(QIODevice::ReadOnly))
size = file.size();
file.close();
// If that didn't work, try this
if(size == 0)
{
QString tmpPath = path+".tmp";
for(int i=2; QFileInfo().exists(tmpPath); ++i) // Make sure filename is unique
tmpPath = path+".tmp"+QString::number(i);
if(QFile::copy(path, tmpPath))
{
size = QFileInfo(tmpPath).size();
QFile::remove(tmpPath);
}
}
}
else size = fileInfo.size();
return size;
}
add a comment |
@Rob's answer works in most cases, but returns 0 when the the shortcut's target doesn't exist/is invalid.
Taking a cue from that approach, you can also copy the shortcut and change the extension.
So combining it all into a function (I'm assuming here that opening the target is cheaper/safer than copying the shortcut):
qint64 getFileSize(const QString &path)
{
qint64 size = 0;
QFileInfo fileInfo(path);
if(fileInfo.isSymLink() && fileInfo.size() == QFileInfo(fileInfo.symLinkTarget()).size())
{
// Try this approach first
QFile file(path);
if(file.exists() && file.open(QIODevice::ReadOnly))
size = file.size();
file.close();
// If that didn't work, try this
if(size == 0)
{
QString tmpPath = path+".tmp";
for(int i=2; QFileInfo().exists(tmpPath); ++i) // Make sure filename is unique
tmpPath = path+".tmp"+QString::number(i);
if(QFile::copy(path, tmpPath))
{
size = QFileInfo(tmpPath).size();
QFile::remove(tmpPath);
}
}
}
else size = fileInfo.size();
return size;
}
add a comment |
@Rob's answer works in most cases, but returns 0 when the the shortcut's target doesn't exist/is invalid.
Taking a cue from that approach, you can also copy the shortcut and change the extension.
So combining it all into a function (I'm assuming here that opening the target is cheaper/safer than copying the shortcut):
qint64 getFileSize(const QString &path)
{
qint64 size = 0;
QFileInfo fileInfo(path);
if(fileInfo.isSymLink() && fileInfo.size() == QFileInfo(fileInfo.symLinkTarget()).size())
{
// Try this approach first
QFile file(path);
if(file.exists() && file.open(QIODevice::ReadOnly))
size = file.size();
file.close();
// If that didn't work, try this
if(size == 0)
{
QString tmpPath = path+".tmp";
for(int i=2; QFileInfo().exists(tmpPath); ++i) // Make sure filename is unique
tmpPath = path+".tmp"+QString::number(i);
if(QFile::copy(path, tmpPath))
{
size = QFileInfo(tmpPath).size();
QFile::remove(tmpPath);
}
}
}
else size = fileInfo.size();
return size;
}
@Rob's answer works in most cases, but returns 0 when the the shortcut's target doesn't exist/is invalid.
Taking a cue from that approach, you can also copy the shortcut and change the extension.
So combining it all into a function (I'm assuming here that opening the target is cheaper/safer than copying the shortcut):
qint64 getFileSize(const QString &path)
{
qint64 size = 0;
QFileInfo fileInfo(path);
if(fileInfo.isSymLink() && fileInfo.size() == QFileInfo(fileInfo.symLinkTarget()).size())
{
// Try this approach first
QFile file(path);
if(file.exists() && file.open(QIODevice::ReadOnly))
size = file.size();
file.close();
// If that didn't work, try this
if(size == 0)
{
QString tmpPath = path+".tmp";
for(int i=2; QFileInfo().exists(tmpPath); ++i) // Make sure filename is unique
tmpPath = path+".tmp"+QString::number(i);
if(QFile::copy(path, tmpPath))
{
size = QFileInfo(tmpPath).size();
QFile::remove(tmpPath);
}
}
}
else size = fileInfo.size();
return size;
}
edited Dec 1 '18 at 14:27
answered Dec 1 '18 at 2:50
bur
17713
17713
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53411886%2fqfileinfo-size-is-returning-shortcut-target-size%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cwp46qIp 9r,W7oaN,J8s,Mi2dzWjdPEL jp,omK 3a3M0EoTUVdN jziQzkouwT,p,k g,Himvd8ZNe1agP
Is this really a .lnk file? Perhaps you are using a different kind of shortcut.
– drescherjm
Nov 21 '18 at 12:27
Yes, these are definitely Windows shortcut (
.lnk
) files.– bur
Nov 21 '18 at 12:29
In those cases where
itDir
should refer to a link, what is returned byitDir.filePath()
(oritDir.fileInfo().filePath()
) -- is it the path of the link itself or the path of the target?– G.M.
Nov 21 '18 at 12:32
Both return the path of the link itself as expected.
– bur
Nov 21 '18 at 12:44