newUser coming up undefined
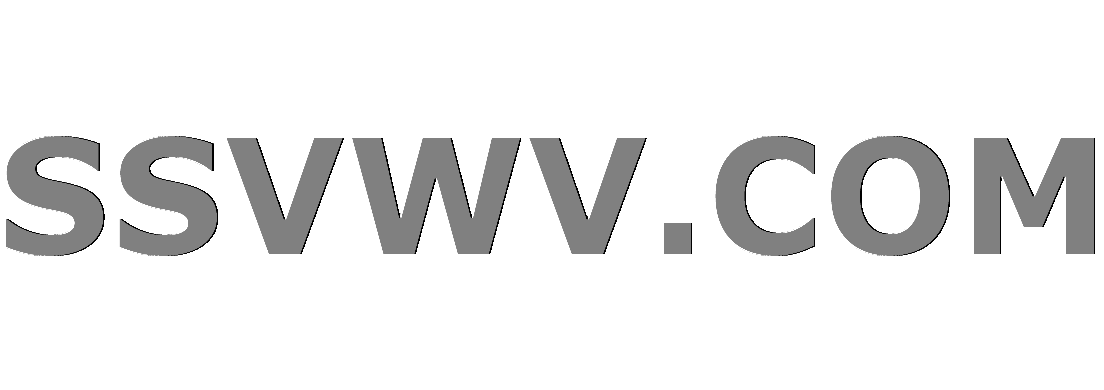
Multi tool use
I am fairly new to Express and am trying to create a simple authentication login/register form. "newUser" is coming up undefined and I am not really sure why.... any suggestions are greatly appreciated. Thanks!
bcrypt.hash(newUser.password, salt, function(err, hash) {
let express = require("express");
let router = express.Router();
let mongojs = require("mongojs");
let db = mongojs("carapp", ["users"]);
let bcrypt = require("bcryptjs");
let passport = require("passport");
let LocalStrategy = require("passport-local").Strategy;
// login GET PAGE
router.get("/login", function(req, res) {
res.render("login");
});
// Register GET PAGE
router.get("/register", function(req, res) {
res.render("register");
});
// Register POST
router.post("/register", function(req, res) {
let name = req.body.name;
let email = req.body.email;
let username = req.body.username;
let password = req.body.password;
let confirm_password = req.body.confirm_password;
// Validation
req.checkBody("name", "Name field is required").notEmpty();
req.checkBody("email", "Email field is required").notEmpty();
req.checkBody("email", "Please use a Valid Email Address").isEmail();
req.checkBody("username", "Username field is required").notEmpty();
req.checkBody("password", "Password field is required").notEmpty();
req.checkBody("confirm_password", "Passwords Do Not
Match".equals(req.body.password);
// Check For Errors
let errors = req.validationErrors();
if (errors) {
console.log("Form has errors");
res.render("register", {
errors: errors,
name: name,
email: email,
username: username,
password: password,
confirm_password: confirm_password
});
} else {
let newUser = {
name: name,
email: email,
username: username,
password: password
};
}
bcrypt.genSalt(10, function(err, salt) {
bcrypt.hash(newUser.password, salt, function(err, hash) {
newUser.password = hash;
db.users.insert(newUser, function(err, doc) {
if (err) {
res.send(err);
} else {
console.log("User Added. Good JOB using MONGODB Matt!");
req.flash("success", "You are registered and can log in");
res.location("/");
res.redirect("/");
}
});
});
});
passport.serializeUser(function(user, done) {
done(null, user._id);
});
passport.deserializeUser(function(id, done) {
db.users.findOne({ _id: mongojs.OnjectId(id) }, function(err, user{
done(err, user);
});
});
passport.use(
new LocalStrategy(function(username, password, done) {
db.users.findOne({ username: username }, function(err, user) {
if (err) {
return done(err);
}
if (!user) {
return done(null, false, { message: "Incorrect Username" });
}
bcrypt.compare(password, user.password, function(err, isMatch) {
if (err) {
return done(err);
}
if (isMatch) {
return done(null, user);
} else {
return done(null, false, { message: "Incorrect Password" });
}
});
});
})
);
// Login Post
router.post("/login",passport.authenticate("local",{
successRedirect: "/",
failureRedirect: "/users/login",
failureFlash: "Invalid Username or Password"
} +
function(req, res) {
console.log("Auth Successful");
res.redirect("/");
}
)
);
});
module.exports = router; //brackets needed here
Here is my package.json file
{
"name": "cars",
"version": "1.0.0",
"description": "car buying application",
"main": "app.js",
"scripts": {
"start" : "nodemon"
},
"author": "",
"license": "ISC",
"dependencies": {
"bcryptjs": "^2.4.3",
"body-parser": "^1.18.3",
"bootstrap": "^4.1.3",
"connect-flash": "^0.1.1",
"ejs": "^2.6.1",
"express": "^4.16.4",
"express-messages": "^1.0.1",
"express-session": "^1.15.6",
"mongojs": "^2.6.0",
"passport": "^0.4.0",
"passport-local": "^1.0.0",
"pug": "^2.0.3"
}
}
javascript node.js express authentication
add a comment |
I am fairly new to Express and am trying to create a simple authentication login/register form. "newUser" is coming up undefined and I am not really sure why.... any suggestions are greatly appreciated. Thanks!
bcrypt.hash(newUser.password, salt, function(err, hash) {
let express = require("express");
let router = express.Router();
let mongojs = require("mongojs");
let db = mongojs("carapp", ["users"]);
let bcrypt = require("bcryptjs");
let passport = require("passport");
let LocalStrategy = require("passport-local").Strategy;
// login GET PAGE
router.get("/login", function(req, res) {
res.render("login");
});
// Register GET PAGE
router.get("/register", function(req, res) {
res.render("register");
});
// Register POST
router.post("/register", function(req, res) {
let name = req.body.name;
let email = req.body.email;
let username = req.body.username;
let password = req.body.password;
let confirm_password = req.body.confirm_password;
// Validation
req.checkBody("name", "Name field is required").notEmpty();
req.checkBody("email", "Email field is required").notEmpty();
req.checkBody("email", "Please use a Valid Email Address").isEmail();
req.checkBody("username", "Username field is required").notEmpty();
req.checkBody("password", "Password field is required").notEmpty();
req.checkBody("confirm_password", "Passwords Do Not
Match".equals(req.body.password);
// Check For Errors
let errors = req.validationErrors();
if (errors) {
console.log("Form has errors");
res.render("register", {
errors: errors,
name: name,
email: email,
username: username,
password: password,
confirm_password: confirm_password
});
} else {
let newUser = {
name: name,
email: email,
username: username,
password: password
};
}
bcrypt.genSalt(10, function(err, salt) {
bcrypt.hash(newUser.password, salt, function(err, hash) {
newUser.password = hash;
db.users.insert(newUser, function(err, doc) {
if (err) {
res.send(err);
} else {
console.log("User Added. Good JOB using MONGODB Matt!");
req.flash("success", "You are registered and can log in");
res.location("/");
res.redirect("/");
}
});
});
});
passport.serializeUser(function(user, done) {
done(null, user._id);
});
passport.deserializeUser(function(id, done) {
db.users.findOne({ _id: mongojs.OnjectId(id) }, function(err, user{
done(err, user);
});
});
passport.use(
new LocalStrategy(function(username, password, done) {
db.users.findOne({ username: username }, function(err, user) {
if (err) {
return done(err);
}
if (!user) {
return done(null, false, { message: "Incorrect Username" });
}
bcrypt.compare(password, user.password, function(err, isMatch) {
if (err) {
return done(err);
}
if (isMatch) {
return done(null, user);
} else {
return done(null, false, { message: "Incorrect Password" });
}
});
});
})
);
// Login Post
router.post("/login",passport.authenticate("local",{
successRedirect: "/",
failureRedirect: "/users/login",
failureFlash: "Invalid Username or Password"
} +
function(req, res) {
console.log("Auth Successful");
res.redirect("/");
}
)
);
});
module.exports = router; //brackets needed here
Here is my package.json file
{
"name": "cars",
"version": "1.0.0",
"description": "car buying application",
"main": "app.js",
"scripts": {
"start" : "nodemon"
},
"author": "",
"license": "ISC",
"dependencies": {
"bcryptjs": "^2.4.3",
"body-parser": "^1.18.3",
"bootstrap": "^4.1.3",
"connect-flash": "^0.1.1",
"ejs": "^2.6.1",
"express": "^4.16.4",
"express-messages": "^1.0.1",
"express-session": "^1.15.6",
"mongojs": "^2.6.0",
"passport": "^0.4.0",
"passport-local": "^1.0.0",
"pug": "^2.0.3"
}
}
javascript node.js express authentication
add a comment |
I am fairly new to Express and am trying to create a simple authentication login/register form. "newUser" is coming up undefined and I am not really sure why.... any suggestions are greatly appreciated. Thanks!
bcrypt.hash(newUser.password, salt, function(err, hash) {
let express = require("express");
let router = express.Router();
let mongojs = require("mongojs");
let db = mongojs("carapp", ["users"]);
let bcrypt = require("bcryptjs");
let passport = require("passport");
let LocalStrategy = require("passport-local").Strategy;
// login GET PAGE
router.get("/login", function(req, res) {
res.render("login");
});
// Register GET PAGE
router.get("/register", function(req, res) {
res.render("register");
});
// Register POST
router.post("/register", function(req, res) {
let name = req.body.name;
let email = req.body.email;
let username = req.body.username;
let password = req.body.password;
let confirm_password = req.body.confirm_password;
// Validation
req.checkBody("name", "Name field is required").notEmpty();
req.checkBody("email", "Email field is required").notEmpty();
req.checkBody("email", "Please use a Valid Email Address").isEmail();
req.checkBody("username", "Username field is required").notEmpty();
req.checkBody("password", "Password field is required").notEmpty();
req.checkBody("confirm_password", "Passwords Do Not
Match".equals(req.body.password);
// Check For Errors
let errors = req.validationErrors();
if (errors) {
console.log("Form has errors");
res.render("register", {
errors: errors,
name: name,
email: email,
username: username,
password: password,
confirm_password: confirm_password
});
} else {
let newUser = {
name: name,
email: email,
username: username,
password: password
};
}
bcrypt.genSalt(10, function(err, salt) {
bcrypt.hash(newUser.password, salt, function(err, hash) {
newUser.password = hash;
db.users.insert(newUser, function(err, doc) {
if (err) {
res.send(err);
} else {
console.log("User Added. Good JOB using MONGODB Matt!");
req.flash("success", "You are registered and can log in");
res.location("/");
res.redirect("/");
}
});
});
});
passport.serializeUser(function(user, done) {
done(null, user._id);
});
passport.deserializeUser(function(id, done) {
db.users.findOne({ _id: mongojs.OnjectId(id) }, function(err, user{
done(err, user);
});
});
passport.use(
new LocalStrategy(function(username, password, done) {
db.users.findOne({ username: username }, function(err, user) {
if (err) {
return done(err);
}
if (!user) {
return done(null, false, { message: "Incorrect Username" });
}
bcrypt.compare(password, user.password, function(err, isMatch) {
if (err) {
return done(err);
}
if (isMatch) {
return done(null, user);
} else {
return done(null, false, { message: "Incorrect Password" });
}
});
});
})
);
// Login Post
router.post("/login",passport.authenticate("local",{
successRedirect: "/",
failureRedirect: "/users/login",
failureFlash: "Invalid Username or Password"
} +
function(req, res) {
console.log("Auth Successful");
res.redirect("/");
}
)
);
});
module.exports = router; //brackets needed here
Here is my package.json file
{
"name": "cars",
"version": "1.0.0",
"description": "car buying application",
"main": "app.js",
"scripts": {
"start" : "nodemon"
},
"author": "",
"license": "ISC",
"dependencies": {
"bcryptjs": "^2.4.3",
"body-parser": "^1.18.3",
"bootstrap": "^4.1.3",
"connect-flash": "^0.1.1",
"ejs": "^2.6.1",
"express": "^4.16.4",
"express-messages": "^1.0.1",
"express-session": "^1.15.6",
"mongojs": "^2.6.0",
"passport": "^0.4.0",
"passport-local": "^1.0.0",
"pug": "^2.0.3"
}
}
javascript node.js express authentication
I am fairly new to Express and am trying to create a simple authentication login/register form. "newUser" is coming up undefined and I am not really sure why.... any suggestions are greatly appreciated. Thanks!
bcrypt.hash(newUser.password, salt, function(err, hash) {
let express = require("express");
let router = express.Router();
let mongojs = require("mongojs");
let db = mongojs("carapp", ["users"]);
let bcrypt = require("bcryptjs");
let passport = require("passport");
let LocalStrategy = require("passport-local").Strategy;
// login GET PAGE
router.get("/login", function(req, res) {
res.render("login");
});
// Register GET PAGE
router.get("/register", function(req, res) {
res.render("register");
});
// Register POST
router.post("/register", function(req, res) {
let name = req.body.name;
let email = req.body.email;
let username = req.body.username;
let password = req.body.password;
let confirm_password = req.body.confirm_password;
// Validation
req.checkBody("name", "Name field is required").notEmpty();
req.checkBody("email", "Email field is required").notEmpty();
req.checkBody("email", "Please use a Valid Email Address").isEmail();
req.checkBody("username", "Username field is required").notEmpty();
req.checkBody("password", "Password field is required").notEmpty();
req.checkBody("confirm_password", "Passwords Do Not
Match".equals(req.body.password);
// Check For Errors
let errors = req.validationErrors();
if (errors) {
console.log("Form has errors");
res.render("register", {
errors: errors,
name: name,
email: email,
username: username,
password: password,
confirm_password: confirm_password
});
} else {
let newUser = {
name: name,
email: email,
username: username,
password: password
};
}
bcrypt.genSalt(10, function(err, salt) {
bcrypt.hash(newUser.password, salt, function(err, hash) {
newUser.password = hash;
db.users.insert(newUser, function(err, doc) {
if (err) {
res.send(err);
} else {
console.log("User Added. Good JOB using MONGODB Matt!");
req.flash("success", "You are registered and can log in");
res.location("/");
res.redirect("/");
}
});
});
});
passport.serializeUser(function(user, done) {
done(null, user._id);
});
passport.deserializeUser(function(id, done) {
db.users.findOne({ _id: mongojs.OnjectId(id) }, function(err, user{
done(err, user);
});
});
passport.use(
new LocalStrategy(function(username, password, done) {
db.users.findOne({ username: username }, function(err, user) {
if (err) {
return done(err);
}
if (!user) {
return done(null, false, { message: "Incorrect Username" });
}
bcrypt.compare(password, user.password, function(err, isMatch) {
if (err) {
return done(err);
}
if (isMatch) {
return done(null, user);
} else {
return done(null, false, { message: "Incorrect Password" });
}
});
});
})
);
// Login Post
router.post("/login",passport.authenticate("local",{
successRedirect: "/",
failureRedirect: "/users/login",
failureFlash: "Invalid Username or Password"
} +
function(req, res) {
console.log("Auth Successful");
res.redirect("/");
}
)
);
});
module.exports = router; //brackets needed here
Here is my package.json file
{
"name": "cars",
"version": "1.0.0",
"description": "car buying application",
"main": "app.js",
"scripts": {
"start" : "nodemon"
},
"author": "",
"license": "ISC",
"dependencies": {
"bcryptjs": "^2.4.3",
"body-parser": "^1.18.3",
"bootstrap": "^4.1.3",
"connect-flash": "^0.1.1",
"ejs": "^2.6.1",
"express": "^4.16.4",
"express-messages": "^1.0.1",
"express-session": "^1.15.6",
"mongojs": "^2.6.0",
"passport": "^0.4.0",
"passport-local": "^1.0.0",
"pug": "^2.0.3"
}
}
javascript node.js express authentication
javascript node.js express authentication
edited Nov 21 at 2:26
asked Nov 21 at 2:16
Matt Grossman
83
83
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
let
only exists in its block scope.
When you declare it in a {}
block but close the block immediately, it is effectively a no-op.
In your original code:
// newUser = undefined
} else {
let newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = undefined
You can try something like:
let newUser;
// newUser = undefined
....
} else {
newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = Object
can you show me where the code should go please?
– Matt Grossman
Nov 21 at 2:51
it was your original code, you should rewrite it usingvar
or putlet newUser
outside the block. Added a simple edited version
– William Chong
Nov 21 at 7:04
add a comment |
Make sure you have installed body-parser. You body may not be in JSON format.
thanks for the reply! i do have body-parser installed- Version "1.18.3"
– Matt Grossman
Nov 21 at 2:24
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404415%2fnewuser-coming-up-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
let
only exists in its block scope.
When you declare it in a {}
block but close the block immediately, it is effectively a no-op.
In your original code:
// newUser = undefined
} else {
let newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = undefined
You can try something like:
let newUser;
// newUser = undefined
....
} else {
newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = Object
can you show me where the code should go please?
– Matt Grossman
Nov 21 at 2:51
it was your original code, you should rewrite it usingvar
or putlet newUser
outside the block. Added a simple edited version
– William Chong
Nov 21 at 7:04
add a comment |
let
only exists in its block scope.
When you declare it in a {}
block but close the block immediately, it is effectively a no-op.
In your original code:
// newUser = undefined
} else {
let newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = undefined
You can try something like:
let newUser;
// newUser = undefined
....
} else {
newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = Object
can you show me where the code should go please?
– Matt Grossman
Nov 21 at 2:51
it was your original code, you should rewrite it usingvar
or putlet newUser
outside the block. Added a simple edited version
– William Chong
Nov 21 at 7:04
add a comment |
let
only exists in its block scope.
When you declare it in a {}
block but close the block immediately, it is effectively a no-op.
In your original code:
// newUser = undefined
} else {
let newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = undefined
You can try something like:
let newUser;
// newUser = undefined
....
} else {
newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = Object
let
only exists in its block scope.
When you declare it in a {}
block but close the block immediately, it is effectively a no-op.
In your original code:
// newUser = undefined
} else {
let newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = undefined
You can try something like:
let newUser;
// newUser = undefined
....
} else {
newUser = {
name: name,
email: email,
username: username,
password: password
};
// newUser = Object
}
// newUser = Object
edited Nov 21 at 7:04
answered Nov 21 at 2:33


William Chong
898416
898416
can you show me where the code should go please?
– Matt Grossman
Nov 21 at 2:51
it was your original code, you should rewrite it usingvar
or putlet newUser
outside the block. Added a simple edited version
– William Chong
Nov 21 at 7:04
add a comment |
can you show me where the code should go please?
– Matt Grossman
Nov 21 at 2:51
it was your original code, you should rewrite it usingvar
or putlet newUser
outside the block. Added a simple edited version
– William Chong
Nov 21 at 7:04
can you show me where the code should go please?
– Matt Grossman
Nov 21 at 2:51
can you show me where the code should go please?
– Matt Grossman
Nov 21 at 2:51
it was your original code, you should rewrite it using
var
or put let newUser
outside the block. Added a simple edited version– William Chong
Nov 21 at 7:04
it was your original code, you should rewrite it using
var
or put let newUser
outside the block. Added a simple edited version– William Chong
Nov 21 at 7:04
add a comment |
Make sure you have installed body-parser. You body may not be in JSON format.
thanks for the reply! i do have body-parser installed- Version "1.18.3"
– Matt Grossman
Nov 21 at 2:24
add a comment |
Make sure you have installed body-parser. You body may not be in JSON format.
thanks for the reply! i do have body-parser installed- Version "1.18.3"
– Matt Grossman
Nov 21 at 2:24
add a comment |
Make sure you have installed body-parser. You body may not be in JSON format.
Make sure you have installed body-parser. You body may not be in JSON format.
answered Nov 21 at 2:21


Ziyo
21629
21629
thanks for the reply! i do have body-parser installed- Version "1.18.3"
– Matt Grossman
Nov 21 at 2:24
add a comment |
thanks for the reply! i do have body-parser installed- Version "1.18.3"
– Matt Grossman
Nov 21 at 2:24
thanks for the reply! i do have body-parser installed- Version "1.18.3"
– Matt Grossman
Nov 21 at 2:24
thanks for the reply! i do have body-parser installed- Version "1.18.3"
– Matt Grossman
Nov 21 at 2:24
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404415%2fnewuser-coming-up-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
TFZ4g m1,5rcKLDhZ8WF,HySuh,naH,sMIAaub,Kwcb3LfuWF I HJW88K,faOV,REC3,zwu2x,Kw