Unable to locate tables in Derby database
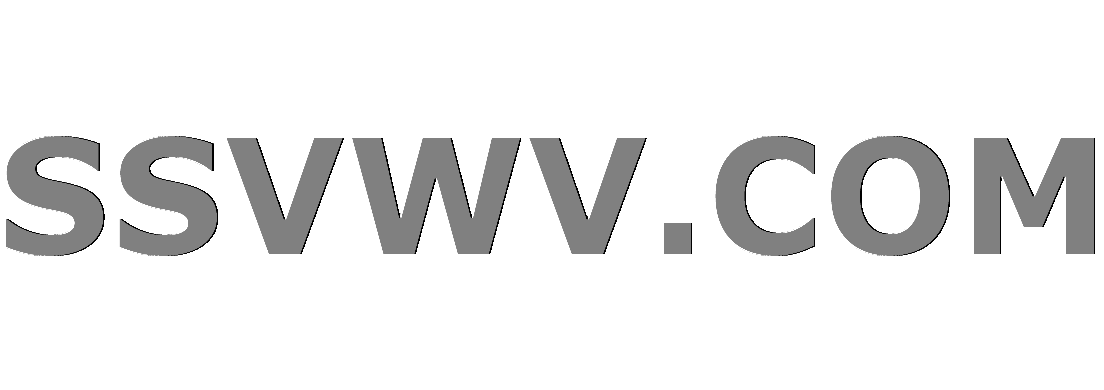
Multi tool use
I can't work out why the following program is unable to locate tables in my derby database:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Properties;
import java.util.logging.Level;
import java.util.logging.Logger;
public class NdConnect
{
public final static String SETUP_FILE_PATH = "/AppData/Local/NewdawnTest";
private static final String CONNECTION_URL = "jdbc:derby:" + "C:/Users/" + System.getenv("USERNAME") + SETUP_FILE_PATH + "db" + ";create=true";
private static Connection conn = null;
private final static Properties dbProperties = new Properties();
private static PreparedStatement pstmtSelectTxns;
public static void connect() {
try {
conn = DriverManager.getConnection(CONNECTION_URL + ";create=true", dbProperties);
pstmtSelectTxns = conn.prepareStatement("SELECT * from TXNS");
System.out.println("Connected OK to " + CONNECTION_URL);
}
catch (SQLException sqle) {
System.out.println("SQL exception");
System.out.println("Connected NOK:The connection URL is " + CONNECTION_URL);
Logger.getLogger(NdConnect.class.getName()).log(Level.SEVERE, null, sqle);
}
}
public static void disconnect()
{
try {
if (conn != null) {
conn.close();
conn = null;
System.out.println("0048 NDC OK:DB closed ");
}
} catch (SQLException sqle) {
Logger.getLogger(NdConnect.class.getName()).log(Level.SEVERE, null, sqle);
}
}
public static void main(String args)
{
// NdConnect nd = new NdConnect();
NdConnect.connect();
NdConnect.disconnect();
// System.out.println("NdConnect finished");
}
}
The program throws a SQLSyntaxErrorException:
run:
SQL exception
Connected NOK:The connection URL is jdbc:derby:C:/Users/Administrator/AppData/Local/NewdawnTestdb;create=true
Nov 25, 2018 6:58:42 AM NdConnect connect
0048 NDC OK:DB closed
SEVERE: null
java.sql.SQLSyntaxErrorException: Table/View 'TXNS' does not exist.
at org.apache.derby.impl.jdbc.SQLExceptionFactory.getSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.Util.generateCsSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.TransactionResourceImpl.wrapInSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.TransactionResourceImpl.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.ConnectionChild.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedPreparedStatement.<init>(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedPreparedStatement42.<init>(Unknown Source)
at org.apache.derby.jdbc.Driver42.newEmbedPreparedStatement(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.prepareStatement(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.prepareStatement(Unknown Source)
at NdConnect.connect(NdConnect.java:21)
at NdConnect.main(NdConnect.java:47)
Caused by: ERROR 42X05: Table/View 'TXNS' does not exist.
at org.apache.derby.iapi.error.StandardException.newException(Unknown Source)
at org.apache.derby.iapi.error.StandardException.newException(Unknown Source)
at org.apache.derby.impl.sql.compile.FromBaseTable.bindTableDescriptor(Unknown Source)
at org.apache.derby.impl.sql.compile.FromBaseTable.bindNonVTITables(Unknown Source)
at org.apache.derby.impl.sql.compile.FromList.bindTables(Unknown Source)
at org.apache.derby.impl.sql.compile.SelectNode.bindNonVTITables(Unknown Source)
at org.apache.derby.impl.sql.compile.DMLStatementNode.bindTables(Unknown Source)
at org.apache.derby.impl.sql.compile.DMLStatementNode.bind(Unknown Source)
at org.apache.derby.impl.sql.compile.CursorNode.bindStatement(Unknown Source)
at org.apache.derby.impl.sql.GenericStatement.prepMinion(Unknown Source)
at org.apache.derby.impl.sql.GenericStatement.prepare(Unknown Source)
at org.apache.derby.impl.sql.conn.GenericLanguageConnectionContext.prepareInternalStatement(Unknown Source)
... 7 more
However the database and table do exist. I have verified this by creating a connection to the database in Netbeans > Services. When I run this command in Services:
SELECT * from TXNS
the desired table is correctly displayed and the output window returns:
Executed successfully in 0 s.
Fetching resultset took 0.016 s.
Line 1, column 1
Execution finished after 0.254 s, no errors occurred.
Looking at the properties of this connection via Netbeans services shows the following attribute values:
Display name NewDawn – TEST DB
Database URL jdbc:derby:C:UsersAdministratorAppDataLocalNewdawnTestdb
Driver apache_derby_embedded
Driver class org.apache.derby.jdbc.EmbeddedDriver
This seems to correspond to the setup I have in the java code example so I don't understand why the code doesn't work.
java derby netbeans-8
add a comment |
I can't work out why the following program is unable to locate tables in my derby database:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Properties;
import java.util.logging.Level;
import java.util.logging.Logger;
public class NdConnect
{
public final static String SETUP_FILE_PATH = "/AppData/Local/NewdawnTest";
private static final String CONNECTION_URL = "jdbc:derby:" + "C:/Users/" + System.getenv("USERNAME") + SETUP_FILE_PATH + "db" + ";create=true";
private static Connection conn = null;
private final static Properties dbProperties = new Properties();
private static PreparedStatement pstmtSelectTxns;
public static void connect() {
try {
conn = DriverManager.getConnection(CONNECTION_URL + ";create=true", dbProperties);
pstmtSelectTxns = conn.prepareStatement("SELECT * from TXNS");
System.out.println("Connected OK to " + CONNECTION_URL);
}
catch (SQLException sqle) {
System.out.println("SQL exception");
System.out.println("Connected NOK:The connection URL is " + CONNECTION_URL);
Logger.getLogger(NdConnect.class.getName()).log(Level.SEVERE, null, sqle);
}
}
public static void disconnect()
{
try {
if (conn != null) {
conn.close();
conn = null;
System.out.println("0048 NDC OK:DB closed ");
}
} catch (SQLException sqle) {
Logger.getLogger(NdConnect.class.getName()).log(Level.SEVERE, null, sqle);
}
}
public static void main(String args)
{
// NdConnect nd = new NdConnect();
NdConnect.connect();
NdConnect.disconnect();
// System.out.println("NdConnect finished");
}
}
The program throws a SQLSyntaxErrorException:
run:
SQL exception
Connected NOK:The connection URL is jdbc:derby:C:/Users/Administrator/AppData/Local/NewdawnTestdb;create=true
Nov 25, 2018 6:58:42 AM NdConnect connect
0048 NDC OK:DB closed
SEVERE: null
java.sql.SQLSyntaxErrorException: Table/View 'TXNS' does not exist.
at org.apache.derby.impl.jdbc.SQLExceptionFactory.getSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.Util.generateCsSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.TransactionResourceImpl.wrapInSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.TransactionResourceImpl.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.ConnectionChild.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedPreparedStatement.<init>(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedPreparedStatement42.<init>(Unknown Source)
at org.apache.derby.jdbc.Driver42.newEmbedPreparedStatement(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.prepareStatement(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.prepareStatement(Unknown Source)
at NdConnect.connect(NdConnect.java:21)
at NdConnect.main(NdConnect.java:47)
Caused by: ERROR 42X05: Table/View 'TXNS' does not exist.
at org.apache.derby.iapi.error.StandardException.newException(Unknown Source)
at org.apache.derby.iapi.error.StandardException.newException(Unknown Source)
at org.apache.derby.impl.sql.compile.FromBaseTable.bindTableDescriptor(Unknown Source)
at org.apache.derby.impl.sql.compile.FromBaseTable.bindNonVTITables(Unknown Source)
at org.apache.derby.impl.sql.compile.FromList.bindTables(Unknown Source)
at org.apache.derby.impl.sql.compile.SelectNode.bindNonVTITables(Unknown Source)
at org.apache.derby.impl.sql.compile.DMLStatementNode.bindTables(Unknown Source)
at org.apache.derby.impl.sql.compile.DMLStatementNode.bind(Unknown Source)
at org.apache.derby.impl.sql.compile.CursorNode.bindStatement(Unknown Source)
at org.apache.derby.impl.sql.GenericStatement.prepMinion(Unknown Source)
at org.apache.derby.impl.sql.GenericStatement.prepare(Unknown Source)
at org.apache.derby.impl.sql.conn.GenericLanguageConnectionContext.prepareInternalStatement(Unknown Source)
... 7 more
However the database and table do exist. I have verified this by creating a connection to the database in Netbeans > Services. When I run this command in Services:
SELECT * from TXNS
the desired table is correctly displayed and the output window returns:
Executed successfully in 0 s.
Fetching resultset took 0.016 s.
Line 1, column 1
Execution finished after 0.254 s, no errors occurred.
Looking at the properties of this connection via Netbeans services shows the following attribute values:
Display name NewDawn – TEST DB
Database URL jdbc:derby:C:UsersAdministratorAppDataLocalNewdawnTestdb
Driver apache_derby_embedded
Driver class org.apache.derby.jdbc.EmbeddedDriver
This seems to correspond to the setup I have in the java code example so I don't understand why the code doesn't work.
java derby netbeans-8
where'spstmtSelectTxns.execute()
? orexecuteQuery()
?
– user7294900
Nov 25 '18 at 7:37
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191. Whether or not I implement the prepared statement seems immaterial - the SQL exception is thrown in every situation. In the example I posted Im simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 7:58
1
Removecreate=true
– user7294900
Nov 25 '18 at 8:00
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191 and this exception is thrown whether or not I implement the prepared statement. In the example I posted I'm simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 8:05
@user7294900 Removing create=true has no effect.
– Rusty
Nov 25 '18 at 8:09
add a comment |
I can't work out why the following program is unable to locate tables in my derby database:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Properties;
import java.util.logging.Level;
import java.util.logging.Logger;
public class NdConnect
{
public final static String SETUP_FILE_PATH = "/AppData/Local/NewdawnTest";
private static final String CONNECTION_URL = "jdbc:derby:" + "C:/Users/" + System.getenv("USERNAME") + SETUP_FILE_PATH + "db" + ";create=true";
private static Connection conn = null;
private final static Properties dbProperties = new Properties();
private static PreparedStatement pstmtSelectTxns;
public static void connect() {
try {
conn = DriverManager.getConnection(CONNECTION_URL + ";create=true", dbProperties);
pstmtSelectTxns = conn.prepareStatement("SELECT * from TXNS");
System.out.println("Connected OK to " + CONNECTION_URL);
}
catch (SQLException sqle) {
System.out.println("SQL exception");
System.out.println("Connected NOK:The connection URL is " + CONNECTION_URL);
Logger.getLogger(NdConnect.class.getName()).log(Level.SEVERE, null, sqle);
}
}
public static void disconnect()
{
try {
if (conn != null) {
conn.close();
conn = null;
System.out.println("0048 NDC OK:DB closed ");
}
} catch (SQLException sqle) {
Logger.getLogger(NdConnect.class.getName()).log(Level.SEVERE, null, sqle);
}
}
public static void main(String args)
{
// NdConnect nd = new NdConnect();
NdConnect.connect();
NdConnect.disconnect();
// System.out.println("NdConnect finished");
}
}
The program throws a SQLSyntaxErrorException:
run:
SQL exception
Connected NOK:The connection URL is jdbc:derby:C:/Users/Administrator/AppData/Local/NewdawnTestdb;create=true
Nov 25, 2018 6:58:42 AM NdConnect connect
0048 NDC OK:DB closed
SEVERE: null
java.sql.SQLSyntaxErrorException: Table/View 'TXNS' does not exist.
at org.apache.derby.impl.jdbc.SQLExceptionFactory.getSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.Util.generateCsSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.TransactionResourceImpl.wrapInSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.TransactionResourceImpl.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.ConnectionChild.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedPreparedStatement.<init>(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedPreparedStatement42.<init>(Unknown Source)
at org.apache.derby.jdbc.Driver42.newEmbedPreparedStatement(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.prepareStatement(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.prepareStatement(Unknown Source)
at NdConnect.connect(NdConnect.java:21)
at NdConnect.main(NdConnect.java:47)
Caused by: ERROR 42X05: Table/View 'TXNS' does not exist.
at org.apache.derby.iapi.error.StandardException.newException(Unknown Source)
at org.apache.derby.iapi.error.StandardException.newException(Unknown Source)
at org.apache.derby.impl.sql.compile.FromBaseTable.bindTableDescriptor(Unknown Source)
at org.apache.derby.impl.sql.compile.FromBaseTable.bindNonVTITables(Unknown Source)
at org.apache.derby.impl.sql.compile.FromList.bindTables(Unknown Source)
at org.apache.derby.impl.sql.compile.SelectNode.bindNonVTITables(Unknown Source)
at org.apache.derby.impl.sql.compile.DMLStatementNode.bindTables(Unknown Source)
at org.apache.derby.impl.sql.compile.DMLStatementNode.bind(Unknown Source)
at org.apache.derby.impl.sql.compile.CursorNode.bindStatement(Unknown Source)
at org.apache.derby.impl.sql.GenericStatement.prepMinion(Unknown Source)
at org.apache.derby.impl.sql.GenericStatement.prepare(Unknown Source)
at org.apache.derby.impl.sql.conn.GenericLanguageConnectionContext.prepareInternalStatement(Unknown Source)
... 7 more
However the database and table do exist. I have verified this by creating a connection to the database in Netbeans > Services. When I run this command in Services:
SELECT * from TXNS
the desired table is correctly displayed and the output window returns:
Executed successfully in 0 s.
Fetching resultset took 0.016 s.
Line 1, column 1
Execution finished after 0.254 s, no errors occurred.
Looking at the properties of this connection via Netbeans services shows the following attribute values:
Display name NewDawn – TEST DB
Database URL jdbc:derby:C:UsersAdministratorAppDataLocalNewdawnTestdb
Driver apache_derby_embedded
Driver class org.apache.derby.jdbc.EmbeddedDriver
This seems to correspond to the setup I have in the java code example so I don't understand why the code doesn't work.
java derby netbeans-8
I can't work out why the following program is unable to locate tables in my derby database:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Properties;
import java.util.logging.Level;
import java.util.logging.Logger;
public class NdConnect
{
public final static String SETUP_FILE_PATH = "/AppData/Local/NewdawnTest";
private static final String CONNECTION_URL = "jdbc:derby:" + "C:/Users/" + System.getenv("USERNAME") + SETUP_FILE_PATH + "db" + ";create=true";
private static Connection conn = null;
private final static Properties dbProperties = new Properties();
private static PreparedStatement pstmtSelectTxns;
public static void connect() {
try {
conn = DriverManager.getConnection(CONNECTION_URL + ";create=true", dbProperties);
pstmtSelectTxns = conn.prepareStatement("SELECT * from TXNS");
System.out.println("Connected OK to " + CONNECTION_URL);
}
catch (SQLException sqle) {
System.out.println("SQL exception");
System.out.println("Connected NOK:The connection URL is " + CONNECTION_URL);
Logger.getLogger(NdConnect.class.getName()).log(Level.SEVERE, null, sqle);
}
}
public static void disconnect()
{
try {
if (conn != null) {
conn.close();
conn = null;
System.out.println("0048 NDC OK:DB closed ");
}
} catch (SQLException sqle) {
Logger.getLogger(NdConnect.class.getName()).log(Level.SEVERE, null, sqle);
}
}
public static void main(String args)
{
// NdConnect nd = new NdConnect();
NdConnect.connect();
NdConnect.disconnect();
// System.out.println("NdConnect finished");
}
}
The program throws a SQLSyntaxErrorException:
run:
SQL exception
Connected NOK:The connection URL is jdbc:derby:C:/Users/Administrator/AppData/Local/NewdawnTestdb;create=true
Nov 25, 2018 6:58:42 AM NdConnect connect
0048 NDC OK:DB closed
SEVERE: null
java.sql.SQLSyntaxErrorException: Table/View 'TXNS' does not exist.
at org.apache.derby.impl.jdbc.SQLExceptionFactory.getSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.Util.generateCsSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.TransactionResourceImpl.wrapInSQLException(Unknown Source)
at org.apache.derby.impl.jdbc.TransactionResourceImpl.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.ConnectionChild.handleException(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedPreparedStatement.<init>(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedPreparedStatement42.<init>(Unknown Source)
at org.apache.derby.jdbc.Driver42.newEmbedPreparedStatement(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.prepareStatement(Unknown Source)
at org.apache.derby.impl.jdbc.EmbedConnection.prepareStatement(Unknown Source)
at NdConnect.connect(NdConnect.java:21)
at NdConnect.main(NdConnect.java:47)
Caused by: ERROR 42X05: Table/View 'TXNS' does not exist.
at org.apache.derby.iapi.error.StandardException.newException(Unknown Source)
at org.apache.derby.iapi.error.StandardException.newException(Unknown Source)
at org.apache.derby.impl.sql.compile.FromBaseTable.bindTableDescriptor(Unknown Source)
at org.apache.derby.impl.sql.compile.FromBaseTable.bindNonVTITables(Unknown Source)
at org.apache.derby.impl.sql.compile.FromList.bindTables(Unknown Source)
at org.apache.derby.impl.sql.compile.SelectNode.bindNonVTITables(Unknown Source)
at org.apache.derby.impl.sql.compile.DMLStatementNode.bindTables(Unknown Source)
at org.apache.derby.impl.sql.compile.DMLStatementNode.bind(Unknown Source)
at org.apache.derby.impl.sql.compile.CursorNode.bindStatement(Unknown Source)
at org.apache.derby.impl.sql.GenericStatement.prepMinion(Unknown Source)
at org.apache.derby.impl.sql.GenericStatement.prepare(Unknown Source)
at org.apache.derby.impl.sql.conn.GenericLanguageConnectionContext.prepareInternalStatement(Unknown Source)
... 7 more
However the database and table do exist. I have verified this by creating a connection to the database in Netbeans > Services. When I run this command in Services:
SELECT * from TXNS
the desired table is correctly displayed and the output window returns:
Executed successfully in 0 s.
Fetching resultset took 0.016 s.
Line 1, column 1
Execution finished after 0.254 s, no errors occurred.
Looking at the properties of this connection via Netbeans services shows the following attribute values:
Display name NewDawn – TEST DB
Database URL jdbc:derby:C:UsersAdministratorAppDataLocalNewdawnTestdb
Driver apache_derby_embedded
Driver class org.apache.derby.jdbc.EmbeddedDriver
This seems to correspond to the setup I have in the java code example so I don't understand why the code doesn't work.
java derby netbeans-8
java derby netbeans-8
asked Nov 25 '18 at 7:33
RustyRusty
407
407
where'spstmtSelectTxns.execute()
? orexecuteQuery()
?
– user7294900
Nov 25 '18 at 7:37
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191. Whether or not I implement the prepared statement seems immaterial - the SQL exception is thrown in every situation. In the example I posted Im simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 7:58
1
Removecreate=true
– user7294900
Nov 25 '18 at 8:00
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191 and this exception is thrown whether or not I implement the prepared statement. In the example I posted I'm simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 8:05
@user7294900 Removing create=true has no effect.
– Rusty
Nov 25 '18 at 8:09
add a comment |
where'spstmtSelectTxns.execute()
? orexecuteQuery()
?
– user7294900
Nov 25 '18 at 7:37
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191. Whether or not I implement the prepared statement seems immaterial - the SQL exception is thrown in every situation. In the example I posted Im simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 7:58
1
Removecreate=true
– user7294900
Nov 25 '18 at 8:00
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191 and this exception is thrown whether or not I implement the prepared statement. In the example I posted I'm simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 8:05
@user7294900 Removing create=true has no effect.
– Rusty
Nov 25 '18 at 8:09
where's
pstmtSelectTxns.execute()
? or executeQuery()
?– user7294900
Nov 25 '18 at 7:37
where's
pstmtSelectTxns.execute()
? or executeQuery()
?– user7294900
Nov 25 '18 at 7:37
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191. Whether or not I implement the prepared statement seems immaterial - the SQL exception is thrown in every situation. In the example I posted Im simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 7:58
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191. Whether or not I implement the prepared statement seems immaterial - the SQL exception is thrown in every situation. In the example I posted Im simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 7:58
1
1
Remove
create=true
– user7294900
Nov 25 '18 at 8:00
Remove
create=true
– user7294900
Nov 25 '18 at 8:00
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191 and this exception is thrown whether or not I implement the prepared statement. In the example I posted I'm simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 8:05
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191 and this exception is thrown whether or not I implement the prepared statement. In the example I posted I'm simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 8:05
@user7294900 Removing create=true has no effect.
– Rusty
Nov 25 '18 at 8:09
@user7294900 Removing create=true has no effect.
– Rusty
Nov 25 '18 at 8:09
add a comment |
1 Answer
1
active
oldest
votes
The URL is not the same:
In Netbeans: jdbc:derby:C:UsersAdministratorAppDataLocalNewdawnTestdb
In Java: jdbc:derby:C:/Users/Administrator/AppData/Local/NewdawnTestdb;create=true
Change the CONNECTION_URL
line to:
private static final String CONNECTION_URL = "jdbc:derby:" + "C:/Users/" + System.getenv("USERNAME") + SETUP_FILE_PATH + "/db"
thank you very much, problem solved. A bad miss on my part not picking up on the forward slash.
– Rusty
Nov 26 '18 at 5:37
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53465530%2funable-to-locate-tables-in-derby-database%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The URL is not the same:
In Netbeans: jdbc:derby:C:UsersAdministratorAppDataLocalNewdawnTestdb
In Java: jdbc:derby:C:/Users/Administrator/AppData/Local/NewdawnTestdb;create=true
Change the CONNECTION_URL
line to:
private static final String CONNECTION_URL = "jdbc:derby:" + "C:/Users/" + System.getenv("USERNAME") + SETUP_FILE_PATH + "/db"
thank you very much, problem solved. A bad miss on my part not picking up on the forward slash.
– Rusty
Nov 26 '18 at 5:37
add a comment |
The URL is not the same:
In Netbeans: jdbc:derby:C:UsersAdministratorAppDataLocalNewdawnTestdb
In Java: jdbc:derby:C:/Users/Administrator/AppData/Local/NewdawnTestdb;create=true
Change the CONNECTION_URL
line to:
private static final String CONNECTION_URL = "jdbc:derby:" + "C:/Users/" + System.getenv("USERNAME") + SETUP_FILE_PATH + "/db"
thank you very much, problem solved. A bad miss on my part not picking up on the forward slash.
– Rusty
Nov 26 '18 at 5:37
add a comment |
The URL is not the same:
In Netbeans: jdbc:derby:C:UsersAdministratorAppDataLocalNewdawnTestdb
In Java: jdbc:derby:C:/Users/Administrator/AppData/Local/NewdawnTestdb;create=true
Change the CONNECTION_URL
line to:
private static final String CONNECTION_URL = "jdbc:derby:" + "C:/Users/" + System.getenv("USERNAME") + SETUP_FILE_PATH + "/db"
The URL is not the same:
In Netbeans: jdbc:derby:C:UsersAdministratorAppDataLocalNewdawnTestdb
In Java: jdbc:derby:C:/Users/Administrator/AppData/Local/NewdawnTestdb;create=true
Change the CONNECTION_URL
line to:
private static final String CONNECTION_URL = "jdbc:derby:" + "C:/Users/" + System.getenv("USERNAME") + SETUP_FILE_PATH + "/db"
answered Nov 25 '18 at 9:39
Robin GreenRobin Green
22.5k875155
22.5k875155
thank you very much, problem solved. A bad miss on my part not picking up on the forward slash.
– Rusty
Nov 26 '18 at 5:37
add a comment |
thank you very much, problem solved. A bad miss on my part not picking up on the forward slash.
– Rusty
Nov 26 '18 at 5:37
thank you very much, problem solved. A bad miss on my part not picking up on the forward slash.
– Rusty
Nov 26 '18 at 5:37
thank you very much, problem solved. A bad miss on my part not picking up on the forward slash.
– Rusty
Nov 26 '18 at 5:37
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53465530%2funable-to-locate-tables-in-derby-database%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
R,8 ZZfECR8vtxT,GTl,Qe0sh7iJgLkSp4ovOFdZB508RECZeFW
where's
pstmtSelectTxns.execute()
? orexecuteQuery()
?– user7294900
Nov 25 '18 at 7:37
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191. Whether or not I implement the prepared statement seems immaterial - the SQL exception is thrown in every situation. In the example I posted Im simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 7:58
1
Remove
create=true
– user7294900
Nov 25 '18 at 8:00
These statements would be included in the code if I could get past this SQL exception. I have five other projects that worked without problems using jdk1.7_ but I have just migrated to a new laptop running jdk1.8_191 and this exception is thrown whether or not I implement the prepared statement. In the example I posted I'm simply trying to strip down to the bare essentials of connecting successfully.
– Rusty
Nov 25 '18 at 8:05
@user7294900 Removing create=true has no effect.
– Rusty
Nov 25 '18 at 8:09