Create incidence matrix with igraph in C
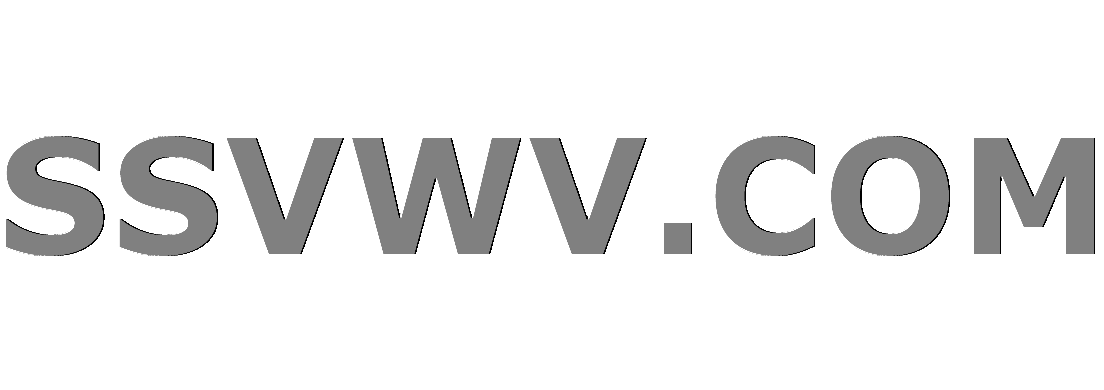
Multi tool use
I'm trying to make an incidence matrix from the graph generated by me:
igraph_t generateGeometricGraph(igraph_integer_t n, igraph_real_t radius){
igraph_t G_graph;
igraph_bool_t connected;
// generate a connected random graph using the geometric model
igraph_grg_game(&G_graph, n, radius, 0, 0, 0);
igraph_is_connected(&G_graph, &connected, IGRAPH_WEAK);
while(!connected){
igraph_destroy(&G_graph);
igraph_grg_game(&G_graph, n, radius, 0, 0, 0);
igraph_is_connected(&G_graph, &connected, IGRAPH_WEAK);
}
return G_graph;
}
This is my graph, but I can't make the matrix: there is a library function to get incidence matrix but it is also for bipartite graph.
I see that there is this function igraph_inclist_init that could be useful but I was unable to obtain the matrix. Thank you for your help!
c igraph
add a comment |
I'm trying to make an incidence matrix from the graph generated by me:
igraph_t generateGeometricGraph(igraph_integer_t n, igraph_real_t radius){
igraph_t G_graph;
igraph_bool_t connected;
// generate a connected random graph using the geometric model
igraph_grg_game(&G_graph, n, radius, 0, 0, 0);
igraph_is_connected(&G_graph, &connected, IGRAPH_WEAK);
while(!connected){
igraph_destroy(&G_graph);
igraph_grg_game(&G_graph, n, radius, 0, 0, 0);
igraph_is_connected(&G_graph, &connected, IGRAPH_WEAK);
}
return G_graph;
}
This is my graph, but I can't make the matrix: there is a library function to get incidence matrix but it is also for bipartite graph.
I see that there is this function igraph_inclist_init that could be useful but I was unable to obtain the matrix. Thank you for your help!
c igraph
The term incidence matrix is used for several different things. Can you define what you mean by it? Do you mean the vertex-edge incidence matrix? If yes, you can loop over edges, and fill out the entries of the matrix as you go (two entries for each edge).
– Szabolcs
Nov 23 '18 at 8:24
Yes, I mean the matrix with vertex-edge
– ciccio
Nov 23 '18 at 8:39
add a comment |
I'm trying to make an incidence matrix from the graph generated by me:
igraph_t generateGeometricGraph(igraph_integer_t n, igraph_real_t radius){
igraph_t G_graph;
igraph_bool_t connected;
// generate a connected random graph using the geometric model
igraph_grg_game(&G_graph, n, radius, 0, 0, 0);
igraph_is_connected(&G_graph, &connected, IGRAPH_WEAK);
while(!connected){
igraph_destroy(&G_graph);
igraph_grg_game(&G_graph, n, radius, 0, 0, 0);
igraph_is_connected(&G_graph, &connected, IGRAPH_WEAK);
}
return G_graph;
}
This is my graph, but I can't make the matrix: there is a library function to get incidence matrix but it is also for bipartite graph.
I see that there is this function igraph_inclist_init that could be useful but I was unable to obtain the matrix. Thank you for your help!
c igraph
I'm trying to make an incidence matrix from the graph generated by me:
igraph_t generateGeometricGraph(igraph_integer_t n, igraph_real_t radius){
igraph_t G_graph;
igraph_bool_t connected;
// generate a connected random graph using the geometric model
igraph_grg_game(&G_graph, n, radius, 0, 0, 0);
igraph_is_connected(&G_graph, &connected, IGRAPH_WEAK);
while(!connected){
igraph_destroy(&G_graph);
igraph_grg_game(&G_graph, n, radius, 0, 0, 0);
igraph_is_connected(&G_graph, &connected, IGRAPH_WEAK);
}
return G_graph;
}
This is my graph, but I can't make the matrix: there is a library function to get incidence matrix but it is also for bipartite graph.
I see that there is this function igraph_inclist_init that could be useful but I was unable to obtain the matrix. Thank you for your help!
c igraph
c igraph
edited Nov 23 '18 at 9:13
Szabolcs
16k361143
16k361143
asked Nov 22 '18 at 23:52
cicciociccio
82
82
The term incidence matrix is used for several different things. Can you define what you mean by it? Do you mean the vertex-edge incidence matrix? If yes, you can loop over edges, and fill out the entries of the matrix as you go (two entries for each edge).
– Szabolcs
Nov 23 '18 at 8:24
Yes, I mean the matrix with vertex-edge
– ciccio
Nov 23 '18 at 8:39
add a comment |
The term incidence matrix is used for several different things. Can you define what you mean by it? Do you mean the vertex-edge incidence matrix? If yes, you can loop over edges, and fill out the entries of the matrix as you go (two entries for each edge).
– Szabolcs
Nov 23 '18 at 8:24
Yes, I mean the matrix with vertex-edge
– ciccio
Nov 23 '18 at 8:39
The term incidence matrix is used for several different things. Can you define what you mean by it? Do you mean the vertex-edge incidence matrix? If yes, you can loop over edges, and fill out the entries of the matrix as you go (two entries for each edge).
– Szabolcs
Nov 23 '18 at 8:24
The term incidence matrix is used for several different things. Can you define what you mean by it? Do you mean the vertex-edge incidence matrix? If yes, you can loop over edges, and fill out the entries of the matrix as you go (two entries for each edge).
– Szabolcs
Nov 23 '18 at 8:24
Yes, I mean the matrix with vertex-edge
– ciccio
Nov 23 '18 at 8:39
Yes, I mean the matrix with vertex-edge
– ciccio
Nov 23 '18 at 8:39
add a comment |
1 Answer
1
active
oldest
votes
A vertex-edge incidence matrix is quite trivial to construct. Just loop over all edges an add the necessary matrix entries for each.
Depending on why you need this matrix, you might want to use a sparse matrix data structure for this. igraph has two sparse matrix types.
For simplicity, here I show an example with the igraph_matrix_t
dense matrix datatype.
#include <igraph.h>
#include <stdio.h>
void print_matrix(igraph_matrix_t *m, FILE *f) {
long int i, j;
for (i=0; i<igraph_matrix_nrow(m); i++) {
for (j=0; j<igraph_matrix_ncol(m); j++) {
fprintf(f, " %li", (long int)MATRIX(*m, i, j));
}
fprintf(f, "n");
}
}
int main() {
igraph_t graph;
igraph_integer_t vcount, ecount, i;
igraph_matrix_t incmat;
igraph_ring(&graph, 10, 0, 0, 0);
vcount = igraph_vcount(&graph);
ecount = igraph_ecount(&graph);
/* this also sets matrix elements to zeros */
igraph_matrix_init(&incmat, vcount, ecount);
for (i=0; i < ecount; ++i) {
/* we increment by one instead of set to 1 to handle self-loops */
MATRIX(incmat, IGRAPH_FROM(&graph, i), i) += 1;
MATRIX(incmat, IGRAPH_TO(&graph, i), i) += 1;
}
print_matrix(&incmat, stdout);
igraph_matrix_destroy(&incmat);
igraph_destroy(&graph);
return 0;
}
Thank you, I had an idea like this but I was unable to code it. One more thing : I'm trying to copy this matrix to a c matrix with this function igraph_matrix_copy_to(const igraph_matrix_t *m, igraph_real_t *to) but I have trouble with pointer to C array, can you help me ? Thanks !
– ciccio
Nov 23 '18 at 11:42
@ciccio If you are not yet comfortable with representing matrices in C, that would be more of a C question than an igraph question. If you want to use a different matrix type than what igraph provides, I suggest you work only with that, not withigraph_matrix_t
. The code I showed does not restrict you to a matrix type.
– Szabolcs
Nov 24 '18 at 10:47
igraph_matrix_copy_to
will simply copy the data in the matrix to the given memory location, as a flat block. It is you resposibility to allocate memory for this.
– Szabolcs
Nov 24 '18 at 10:48
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439201%2fcreate-incidence-matrix-with-igraph-in-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
A vertex-edge incidence matrix is quite trivial to construct. Just loop over all edges an add the necessary matrix entries for each.
Depending on why you need this matrix, you might want to use a sparse matrix data structure for this. igraph has two sparse matrix types.
For simplicity, here I show an example with the igraph_matrix_t
dense matrix datatype.
#include <igraph.h>
#include <stdio.h>
void print_matrix(igraph_matrix_t *m, FILE *f) {
long int i, j;
for (i=0; i<igraph_matrix_nrow(m); i++) {
for (j=0; j<igraph_matrix_ncol(m); j++) {
fprintf(f, " %li", (long int)MATRIX(*m, i, j));
}
fprintf(f, "n");
}
}
int main() {
igraph_t graph;
igraph_integer_t vcount, ecount, i;
igraph_matrix_t incmat;
igraph_ring(&graph, 10, 0, 0, 0);
vcount = igraph_vcount(&graph);
ecount = igraph_ecount(&graph);
/* this also sets matrix elements to zeros */
igraph_matrix_init(&incmat, vcount, ecount);
for (i=0; i < ecount; ++i) {
/* we increment by one instead of set to 1 to handle self-loops */
MATRIX(incmat, IGRAPH_FROM(&graph, i), i) += 1;
MATRIX(incmat, IGRAPH_TO(&graph, i), i) += 1;
}
print_matrix(&incmat, stdout);
igraph_matrix_destroy(&incmat);
igraph_destroy(&graph);
return 0;
}
Thank you, I had an idea like this but I was unable to code it. One more thing : I'm trying to copy this matrix to a c matrix with this function igraph_matrix_copy_to(const igraph_matrix_t *m, igraph_real_t *to) but I have trouble with pointer to C array, can you help me ? Thanks !
– ciccio
Nov 23 '18 at 11:42
@ciccio If you are not yet comfortable with representing matrices in C, that would be more of a C question than an igraph question. If you want to use a different matrix type than what igraph provides, I suggest you work only with that, not withigraph_matrix_t
. The code I showed does not restrict you to a matrix type.
– Szabolcs
Nov 24 '18 at 10:47
igraph_matrix_copy_to
will simply copy the data in the matrix to the given memory location, as a flat block. It is you resposibility to allocate memory for this.
– Szabolcs
Nov 24 '18 at 10:48
add a comment |
A vertex-edge incidence matrix is quite trivial to construct. Just loop over all edges an add the necessary matrix entries for each.
Depending on why you need this matrix, you might want to use a sparse matrix data structure for this. igraph has two sparse matrix types.
For simplicity, here I show an example with the igraph_matrix_t
dense matrix datatype.
#include <igraph.h>
#include <stdio.h>
void print_matrix(igraph_matrix_t *m, FILE *f) {
long int i, j;
for (i=0; i<igraph_matrix_nrow(m); i++) {
for (j=0; j<igraph_matrix_ncol(m); j++) {
fprintf(f, " %li", (long int)MATRIX(*m, i, j));
}
fprintf(f, "n");
}
}
int main() {
igraph_t graph;
igraph_integer_t vcount, ecount, i;
igraph_matrix_t incmat;
igraph_ring(&graph, 10, 0, 0, 0);
vcount = igraph_vcount(&graph);
ecount = igraph_ecount(&graph);
/* this also sets matrix elements to zeros */
igraph_matrix_init(&incmat, vcount, ecount);
for (i=0; i < ecount; ++i) {
/* we increment by one instead of set to 1 to handle self-loops */
MATRIX(incmat, IGRAPH_FROM(&graph, i), i) += 1;
MATRIX(incmat, IGRAPH_TO(&graph, i), i) += 1;
}
print_matrix(&incmat, stdout);
igraph_matrix_destroy(&incmat);
igraph_destroy(&graph);
return 0;
}
Thank you, I had an idea like this but I was unable to code it. One more thing : I'm trying to copy this matrix to a c matrix with this function igraph_matrix_copy_to(const igraph_matrix_t *m, igraph_real_t *to) but I have trouble with pointer to C array, can you help me ? Thanks !
– ciccio
Nov 23 '18 at 11:42
@ciccio If you are not yet comfortable with representing matrices in C, that would be more of a C question than an igraph question. If you want to use a different matrix type than what igraph provides, I suggest you work only with that, not withigraph_matrix_t
. The code I showed does not restrict you to a matrix type.
– Szabolcs
Nov 24 '18 at 10:47
igraph_matrix_copy_to
will simply copy the data in the matrix to the given memory location, as a flat block. It is you resposibility to allocate memory for this.
– Szabolcs
Nov 24 '18 at 10:48
add a comment |
A vertex-edge incidence matrix is quite trivial to construct. Just loop over all edges an add the necessary matrix entries for each.
Depending on why you need this matrix, you might want to use a sparse matrix data structure for this. igraph has two sparse matrix types.
For simplicity, here I show an example with the igraph_matrix_t
dense matrix datatype.
#include <igraph.h>
#include <stdio.h>
void print_matrix(igraph_matrix_t *m, FILE *f) {
long int i, j;
for (i=0; i<igraph_matrix_nrow(m); i++) {
for (j=0; j<igraph_matrix_ncol(m); j++) {
fprintf(f, " %li", (long int)MATRIX(*m, i, j));
}
fprintf(f, "n");
}
}
int main() {
igraph_t graph;
igraph_integer_t vcount, ecount, i;
igraph_matrix_t incmat;
igraph_ring(&graph, 10, 0, 0, 0);
vcount = igraph_vcount(&graph);
ecount = igraph_ecount(&graph);
/* this also sets matrix elements to zeros */
igraph_matrix_init(&incmat, vcount, ecount);
for (i=0; i < ecount; ++i) {
/* we increment by one instead of set to 1 to handle self-loops */
MATRIX(incmat, IGRAPH_FROM(&graph, i), i) += 1;
MATRIX(incmat, IGRAPH_TO(&graph, i), i) += 1;
}
print_matrix(&incmat, stdout);
igraph_matrix_destroy(&incmat);
igraph_destroy(&graph);
return 0;
}
A vertex-edge incidence matrix is quite trivial to construct. Just loop over all edges an add the necessary matrix entries for each.
Depending on why you need this matrix, you might want to use a sparse matrix data structure for this. igraph has two sparse matrix types.
For simplicity, here I show an example with the igraph_matrix_t
dense matrix datatype.
#include <igraph.h>
#include <stdio.h>
void print_matrix(igraph_matrix_t *m, FILE *f) {
long int i, j;
for (i=0; i<igraph_matrix_nrow(m); i++) {
for (j=0; j<igraph_matrix_ncol(m); j++) {
fprintf(f, " %li", (long int)MATRIX(*m, i, j));
}
fprintf(f, "n");
}
}
int main() {
igraph_t graph;
igraph_integer_t vcount, ecount, i;
igraph_matrix_t incmat;
igraph_ring(&graph, 10, 0, 0, 0);
vcount = igraph_vcount(&graph);
ecount = igraph_ecount(&graph);
/* this also sets matrix elements to zeros */
igraph_matrix_init(&incmat, vcount, ecount);
for (i=0; i < ecount; ++i) {
/* we increment by one instead of set to 1 to handle self-loops */
MATRIX(incmat, IGRAPH_FROM(&graph, i), i) += 1;
MATRIX(incmat, IGRAPH_TO(&graph, i), i) += 1;
}
print_matrix(&incmat, stdout);
igraph_matrix_destroy(&incmat);
igraph_destroy(&graph);
return 0;
}
answered Nov 23 '18 at 9:12
SzabolcsSzabolcs
16k361143
16k361143
Thank you, I had an idea like this but I was unable to code it. One more thing : I'm trying to copy this matrix to a c matrix with this function igraph_matrix_copy_to(const igraph_matrix_t *m, igraph_real_t *to) but I have trouble with pointer to C array, can you help me ? Thanks !
– ciccio
Nov 23 '18 at 11:42
@ciccio If you are not yet comfortable with representing matrices in C, that would be more of a C question than an igraph question. If you want to use a different matrix type than what igraph provides, I suggest you work only with that, not withigraph_matrix_t
. The code I showed does not restrict you to a matrix type.
– Szabolcs
Nov 24 '18 at 10:47
igraph_matrix_copy_to
will simply copy the data in the matrix to the given memory location, as a flat block. It is you resposibility to allocate memory for this.
– Szabolcs
Nov 24 '18 at 10:48
add a comment |
Thank you, I had an idea like this but I was unable to code it. One more thing : I'm trying to copy this matrix to a c matrix with this function igraph_matrix_copy_to(const igraph_matrix_t *m, igraph_real_t *to) but I have trouble with pointer to C array, can you help me ? Thanks !
– ciccio
Nov 23 '18 at 11:42
@ciccio If you are not yet comfortable with representing matrices in C, that would be more of a C question than an igraph question. If you want to use a different matrix type than what igraph provides, I suggest you work only with that, not withigraph_matrix_t
. The code I showed does not restrict you to a matrix type.
– Szabolcs
Nov 24 '18 at 10:47
igraph_matrix_copy_to
will simply copy the data in the matrix to the given memory location, as a flat block. It is you resposibility to allocate memory for this.
– Szabolcs
Nov 24 '18 at 10:48
Thank you, I had an idea like this but I was unable to code it. One more thing : I'm trying to copy this matrix to a c matrix with this function igraph_matrix_copy_to(const igraph_matrix_t *m, igraph_real_t *to) but I have trouble with pointer to C array, can you help me ? Thanks !
– ciccio
Nov 23 '18 at 11:42
Thank you, I had an idea like this but I was unable to code it. One more thing : I'm trying to copy this matrix to a c matrix with this function igraph_matrix_copy_to(const igraph_matrix_t *m, igraph_real_t *to) but I have trouble with pointer to C array, can you help me ? Thanks !
– ciccio
Nov 23 '18 at 11:42
@ciccio If you are not yet comfortable with representing matrices in C, that would be more of a C question than an igraph question. If you want to use a different matrix type than what igraph provides, I suggest you work only with that, not with
igraph_matrix_t
. The code I showed does not restrict you to a matrix type.– Szabolcs
Nov 24 '18 at 10:47
@ciccio If you are not yet comfortable with representing matrices in C, that would be more of a C question than an igraph question. If you want to use a different matrix type than what igraph provides, I suggest you work only with that, not with
igraph_matrix_t
. The code I showed does not restrict you to a matrix type.– Szabolcs
Nov 24 '18 at 10:47
igraph_matrix_copy_to
will simply copy the data in the matrix to the given memory location, as a flat block. It is you resposibility to allocate memory for this.– Szabolcs
Nov 24 '18 at 10:48
igraph_matrix_copy_to
will simply copy the data in the matrix to the given memory location, as a flat block. It is you resposibility to allocate memory for this.– Szabolcs
Nov 24 '18 at 10:48
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439201%2fcreate-incidence-matrix-with-igraph-in-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6oU1Zdty irREwvwP x,LgaTFrPk1mbIqO4nqILAyVf,m kM4Ekoz033scE5RcOUAIe3nAppQ8N6jL gi9wGF9Tf0pvryT x
The term incidence matrix is used for several different things. Can you define what you mean by it? Do you mean the vertex-edge incidence matrix? If yes, you can loop over edges, and fill out the entries of the matrix as you go (two entries for each edge).
– Szabolcs
Nov 23 '18 at 8:24
Yes, I mean the matrix with vertex-edge
– ciccio
Nov 23 '18 at 8:39