Aggregating HTML files
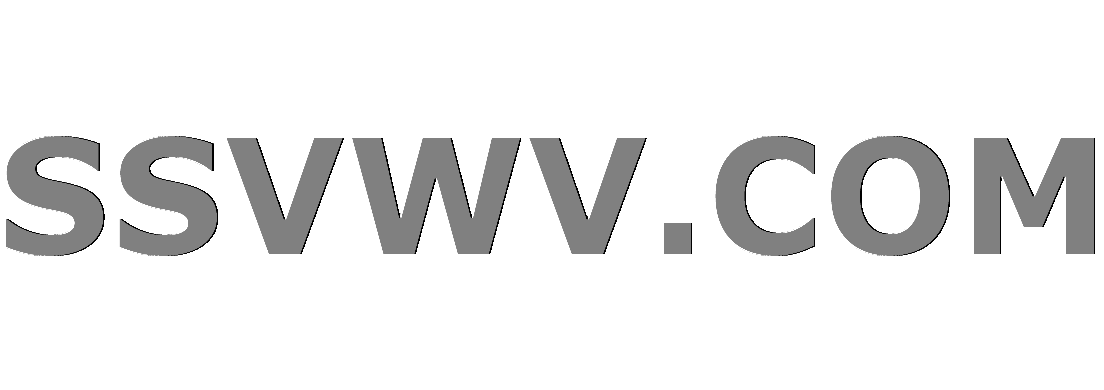
Multi tool use
$begingroup$
I am using PHP to keep my webpage organized.
The directory looks like this:
root/folder/file1
root/folder/file2
root/folder/file3
root/index.php
Each file is very simple html doc with content containing a title tag, which I use to create a nav bar.
I want index.php to aggregate all the content from file1, file2 and file3, in addition to creating a nav bar up at the top.
The code I have achieves this successfully, but I'm not sure if I'm doing this in a practical way.
$domain = "domain/"
$dir = "folder/";
$files = scandir($dir);
// loop over all the files, saving their contents and ids to arrays
$contents = Array();
$titles = Array();
$ids = Array();
for ($x = 0; $x < sizeof($files); $x++) {
if ($files[$x] != "." && $files[$x] != "..") {
$filename = $domain . $dir . $files[$x];
$file = fopen($filename, "r");
$data = file_get_contents($filename);
array_push($contents, $data);
$regex = '#<title>(.*?)</title>#';
preg_match($regex, $data, $match);
$title = $match[1];
array_push($titles, $title);
$regex2 = '#( |-|,)#';
$id = preg_split($regex2, $title)[0];
array_push($ids, $id);
}
}
// the first loop sets the nav bar
echo "<div id='navigation'>";
echo "<ul>";
for ($x = 0; $x < sizeof($contents); $x++) {
echo "<li><a href='#" . $ids[$x] . "'>" . $titles[$x] . "</a></li>";
}
echo "</ul>";
echo "</div>";
// this second loop sets the contents
echo "<div id='content'>";
for ($x = 0; $x < sizeof($contents); $x++) {
echo "<div id='" . $ids[$x] . "'>" . $contents[$x] . "</div>";
}
echo "</div>";
I like the idea of having all the content on one big page and the nav bar helps a lot when I'm viewing it on my phone.
Questions
- Are there any obvious problems with the way I am aggregating my files?
- Are there challenges I may encounter that I have not yet experienced?
- Is there a generally accepted way of doing this while achieving the same result?
php html
New contributor
Jozurcrunch is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
I am using PHP to keep my webpage organized.
The directory looks like this:
root/folder/file1
root/folder/file2
root/folder/file3
root/index.php
Each file is very simple html doc with content containing a title tag, which I use to create a nav bar.
I want index.php to aggregate all the content from file1, file2 and file3, in addition to creating a nav bar up at the top.
The code I have achieves this successfully, but I'm not sure if I'm doing this in a practical way.
$domain = "domain/"
$dir = "folder/";
$files = scandir($dir);
// loop over all the files, saving their contents and ids to arrays
$contents = Array();
$titles = Array();
$ids = Array();
for ($x = 0; $x < sizeof($files); $x++) {
if ($files[$x] != "." && $files[$x] != "..") {
$filename = $domain . $dir . $files[$x];
$file = fopen($filename, "r");
$data = file_get_contents($filename);
array_push($contents, $data);
$regex = '#<title>(.*?)</title>#';
preg_match($regex, $data, $match);
$title = $match[1];
array_push($titles, $title);
$regex2 = '#( |-|,)#';
$id = preg_split($regex2, $title)[0];
array_push($ids, $id);
}
}
// the first loop sets the nav bar
echo "<div id='navigation'>";
echo "<ul>";
for ($x = 0; $x < sizeof($contents); $x++) {
echo "<li><a href='#" . $ids[$x] . "'>" . $titles[$x] . "</a></li>";
}
echo "</ul>";
echo "</div>";
// this second loop sets the contents
echo "<div id='content'>";
for ($x = 0; $x < sizeof($contents); $x++) {
echo "<div id='" . $ids[$x] . "'>" . $contents[$x] . "</div>";
}
echo "</div>";
I like the idea of having all the content on one big page and the nav bar helps a lot when I'm viewing it on my phone.
Questions
- Are there any obvious problems with the way I am aggregating my files?
- Are there challenges I may encounter that I have not yet experienced?
- Is there a generally accepted way of doing this while achieving the same result?
php html
New contributor
Jozurcrunch is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
I am using PHP to keep my webpage organized.
The directory looks like this:
root/folder/file1
root/folder/file2
root/folder/file3
root/index.php
Each file is very simple html doc with content containing a title tag, which I use to create a nav bar.
I want index.php to aggregate all the content from file1, file2 and file3, in addition to creating a nav bar up at the top.
The code I have achieves this successfully, but I'm not sure if I'm doing this in a practical way.
$domain = "domain/"
$dir = "folder/";
$files = scandir($dir);
// loop over all the files, saving their contents and ids to arrays
$contents = Array();
$titles = Array();
$ids = Array();
for ($x = 0; $x < sizeof($files); $x++) {
if ($files[$x] != "." && $files[$x] != "..") {
$filename = $domain . $dir . $files[$x];
$file = fopen($filename, "r");
$data = file_get_contents($filename);
array_push($contents, $data);
$regex = '#<title>(.*?)</title>#';
preg_match($regex, $data, $match);
$title = $match[1];
array_push($titles, $title);
$regex2 = '#( |-|,)#';
$id = preg_split($regex2, $title)[0];
array_push($ids, $id);
}
}
// the first loop sets the nav bar
echo "<div id='navigation'>";
echo "<ul>";
for ($x = 0; $x < sizeof($contents); $x++) {
echo "<li><a href='#" . $ids[$x] . "'>" . $titles[$x] . "</a></li>";
}
echo "</ul>";
echo "</div>";
// this second loop sets the contents
echo "<div id='content'>";
for ($x = 0; $x < sizeof($contents); $x++) {
echo "<div id='" . $ids[$x] . "'>" . $contents[$x] . "</div>";
}
echo "</div>";
I like the idea of having all the content on one big page and the nav bar helps a lot when I'm viewing it on my phone.
Questions
- Are there any obvious problems with the way I am aggregating my files?
- Are there challenges I may encounter that I have not yet experienced?
- Is there a generally accepted way of doing this while achieving the same result?
php html
New contributor
Jozurcrunch is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
I am using PHP to keep my webpage organized.
The directory looks like this:
root/folder/file1
root/folder/file2
root/folder/file3
root/index.php
Each file is very simple html doc with content containing a title tag, which I use to create a nav bar.
I want index.php to aggregate all the content from file1, file2 and file3, in addition to creating a nav bar up at the top.
The code I have achieves this successfully, but I'm not sure if I'm doing this in a practical way.
$domain = "domain/"
$dir = "folder/";
$files = scandir($dir);
// loop over all the files, saving their contents and ids to arrays
$contents = Array();
$titles = Array();
$ids = Array();
for ($x = 0; $x < sizeof($files); $x++) {
if ($files[$x] != "." && $files[$x] != "..") {
$filename = $domain . $dir . $files[$x];
$file = fopen($filename, "r");
$data = file_get_contents($filename);
array_push($contents, $data);
$regex = '#<title>(.*?)</title>#';
preg_match($regex, $data, $match);
$title = $match[1];
array_push($titles, $title);
$regex2 = '#( |-|,)#';
$id = preg_split($regex2, $title)[0];
array_push($ids, $id);
}
}
// the first loop sets the nav bar
echo "<div id='navigation'>";
echo "<ul>";
for ($x = 0; $x < sizeof($contents); $x++) {
echo "<li><a href='#" . $ids[$x] . "'>" . $titles[$x] . "</a></li>";
}
echo "</ul>";
echo "</div>";
// this second loop sets the contents
echo "<div id='content'>";
for ($x = 0; $x < sizeof($contents); $x++) {
echo "<div id='" . $ids[$x] . "'>" . $contents[$x] . "</div>";
}
echo "</div>";
I like the idea of having all the content on one big page and the nav bar helps a lot when I'm viewing it on my phone.
Questions
- Are there any obvious problems with the way I am aggregating my files?
- Are there challenges I may encounter that I have not yet experienced?
- Is there a generally accepted way of doing this while achieving the same result?
php html
php html
New contributor
Jozurcrunch is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Jozurcrunch is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 1 min ago


Jamal♦
30.3k11116227
30.3k11116227
New contributor
Jozurcrunch is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked yesterday
JozurcrunchJozurcrunch
182
182
New contributor
Jozurcrunch is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Jozurcrunch is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Jozurcrunch is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
Basically you need to remove the unused fopen() call and change ponderous operators to handy ones as for some reason you made a peculiar choice in favor of the former, like for vs. foreach, array_push vs simple assignment, etc. Also consider a cleaner way to output HTML
<?php
$pattern = "domain/folder/*.*";
$files = glob($pattern);
// loop over all the files, saving their contents and ids to arrays
$contents = ;
$titles = ;
$ids = ;
foreach ($files as $filename)
{
$data = file_get_contents($filename);
$contents = $data;
preg_match('#<title>(.*?)</title>#', $data, $match);
$titles = $match[1];
$ids = preg_split('#( |-|,)#', $match[1])[0];
}
?>
<div id='navigation'>
<ul>
<?php foreach ($titles as $x => $title): ?>
<li><a href="#<?=$ids[$x]?>"><?=$title?></a></li>
<?php endforeach ?>
</ul>
</div>
<div id='content'>
<?foreach ($contents as $x => $content): ?>
<div id="<?=$ids[$x]?>"><?=$content?></div>
<?php endforeach ?>
</div>
$endgroup$
$begingroup$
This is very interesting. I don't have a lot of experience with php so you've shown me quite a bit. In line 3 of your code I simply changed $dir to $pattern, and in line 18 I changed $title to $match[1], since $title no longer exists. I'm still reviewing it but the result is the same as before which is good. Are the overall mechanics of what I'm doing (looping once to capture data, then looping 2 more times to template the content) reasonable?
$endgroup$
– Jozurcrunch
4 hours ago
$begingroup$
Looping 2 times is the only acceptable way. Data preparation should be always separated from the output as output may vary
$endgroup$
– Your Common Sense
27 mins ago
$begingroup$
Thank you for the corrections!
$endgroup$
– Your Common Sense
25 mins ago
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Jozurcrunch is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f212298%2faggregating-html-files%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Basically you need to remove the unused fopen() call and change ponderous operators to handy ones as for some reason you made a peculiar choice in favor of the former, like for vs. foreach, array_push vs simple assignment, etc. Also consider a cleaner way to output HTML
<?php
$pattern = "domain/folder/*.*";
$files = glob($pattern);
// loop over all the files, saving their contents and ids to arrays
$contents = ;
$titles = ;
$ids = ;
foreach ($files as $filename)
{
$data = file_get_contents($filename);
$contents = $data;
preg_match('#<title>(.*?)</title>#', $data, $match);
$titles = $match[1];
$ids = preg_split('#( |-|,)#', $match[1])[0];
}
?>
<div id='navigation'>
<ul>
<?php foreach ($titles as $x => $title): ?>
<li><a href="#<?=$ids[$x]?>"><?=$title?></a></li>
<?php endforeach ?>
</ul>
</div>
<div id='content'>
<?foreach ($contents as $x => $content): ?>
<div id="<?=$ids[$x]?>"><?=$content?></div>
<?php endforeach ?>
</div>
$endgroup$
$begingroup$
This is very interesting. I don't have a lot of experience with php so you've shown me quite a bit. In line 3 of your code I simply changed $dir to $pattern, and in line 18 I changed $title to $match[1], since $title no longer exists. I'm still reviewing it but the result is the same as before which is good. Are the overall mechanics of what I'm doing (looping once to capture data, then looping 2 more times to template the content) reasonable?
$endgroup$
– Jozurcrunch
4 hours ago
$begingroup$
Looping 2 times is the only acceptable way. Data preparation should be always separated from the output as output may vary
$endgroup$
– Your Common Sense
27 mins ago
$begingroup$
Thank you for the corrections!
$endgroup$
– Your Common Sense
25 mins ago
add a comment |
$begingroup$
Basically you need to remove the unused fopen() call and change ponderous operators to handy ones as for some reason you made a peculiar choice in favor of the former, like for vs. foreach, array_push vs simple assignment, etc. Also consider a cleaner way to output HTML
<?php
$pattern = "domain/folder/*.*";
$files = glob($pattern);
// loop over all the files, saving their contents and ids to arrays
$contents = ;
$titles = ;
$ids = ;
foreach ($files as $filename)
{
$data = file_get_contents($filename);
$contents = $data;
preg_match('#<title>(.*?)</title>#', $data, $match);
$titles = $match[1];
$ids = preg_split('#( |-|,)#', $match[1])[0];
}
?>
<div id='navigation'>
<ul>
<?php foreach ($titles as $x => $title): ?>
<li><a href="#<?=$ids[$x]?>"><?=$title?></a></li>
<?php endforeach ?>
</ul>
</div>
<div id='content'>
<?foreach ($contents as $x => $content): ?>
<div id="<?=$ids[$x]?>"><?=$content?></div>
<?php endforeach ?>
</div>
$endgroup$
$begingroup$
This is very interesting. I don't have a lot of experience with php so you've shown me quite a bit. In line 3 of your code I simply changed $dir to $pattern, and in line 18 I changed $title to $match[1], since $title no longer exists. I'm still reviewing it but the result is the same as before which is good. Are the overall mechanics of what I'm doing (looping once to capture data, then looping 2 more times to template the content) reasonable?
$endgroup$
– Jozurcrunch
4 hours ago
$begingroup$
Looping 2 times is the only acceptable way. Data preparation should be always separated from the output as output may vary
$endgroup$
– Your Common Sense
27 mins ago
$begingroup$
Thank you for the corrections!
$endgroup$
– Your Common Sense
25 mins ago
add a comment |
$begingroup$
Basically you need to remove the unused fopen() call and change ponderous operators to handy ones as for some reason you made a peculiar choice in favor of the former, like for vs. foreach, array_push vs simple assignment, etc. Also consider a cleaner way to output HTML
<?php
$pattern = "domain/folder/*.*";
$files = glob($pattern);
// loop over all the files, saving their contents and ids to arrays
$contents = ;
$titles = ;
$ids = ;
foreach ($files as $filename)
{
$data = file_get_contents($filename);
$contents = $data;
preg_match('#<title>(.*?)</title>#', $data, $match);
$titles = $match[1];
$ids = preg_split('#( |-|,)#', $match[1])[0];
}
?>
<div id='navigation'>
<ul>
<?php foreach ($titles as $x => $title): ?>
<li><a href="#<?=$ids[$x]?>"><?=$title?></a></li>
<?php endforeach ?>
</ul>
</div>
<div id='content'>
<?foreach ($contents as $x => $content): ?>
<div id="<?=$ids[$x]?>"><?=$content?></div>
<?php endforeach ?>
</div>
$endgroup$
Basically you need to remove the unused fopen() call and change ponderous operators to handy ones as for some reason you made a peculiar choice in favor of the former, like for vs. foreach, array_push vs simple assignment, etc. Also consider a cleaner way to output HTML
<?php
$pattern = "domain/folder/*.*";
$files = glob($pattern);
// loop over all the files, saving their contents and ids to arrays
$contents = ;
$titles = ;
$ids = ;
foreach ($files as $filename)
{
$data = file_get_contents($filename);
$contents = $data;
preg_match('#<title>(.*?)</title>#', $data, $match);
$titles = $match[1];
$ids = preg_split('#( |-|,)#', $match[1])[0];
}
?>
<div id='navigation'>
<ul>
<?php foreach ($titles as $x => $title): ?>
<li><a href="#<?=$ids[$x]?>"><?=$title?></a></li>
<?php endforeach ?>
</ul>
</div>
<div id='content'>
<?foreach ($contents as $x => $content): ?>
<div id="<?=$ids[$x]?>"><?=$content?></div>
<?php endforeach ?>
</div>
edited 25 mins ago
answered 23 hours ago


Your Common SenseYour Common Sense
3,6071528
3,6071528
$begingroup$
This is very interesting. I don't have a lot of experience with php so you've shown me quite a bit. In line 3 of your code I simply changed $dir to $pattern, and in line 18 I changed $title to $match[1], since $title no longer exists. I'm still reviewing it but the result is the same as before which is good. Are the overall mechanics of what I'm doing (looping once to capture data, then looping 2 more times to template the content) reasonable?
$endgroup$
– Jozurcrunch
4 hours ago
$begingroup$
Looping 2 times is the only acceptable way. Data preparation should be always separated from the output as output may vary
$endgroup$
– Your Common Sense
27 mins ago
$begingroup$
Thank you for the corrections!
$endgroup$
– Your Common Sense
25 mins ago
add a comment |
$begingroup$
This is very interesting. I don't have a lot of experience with php so you've shown me quite a bit. In line 3 of your code I simply changed $dir to $pattern, and in line 18 I changed $title to $match[1], since $title no longer exists. I'm still reviewing it but the result is the same as before which is good. Are the overall mechanics of what I'm doing (looping once to capture data, then looping 2 more times to template the content) reasonable?
$endgroup$
– Jozurcrunch
4 hours ago
$begingroup$
Looping 2 times is the only acceptable way. Data preparation should be always separated from the output as output may vary
$endgroup$
– Your Common Sense
27 mins ago
$begingroup$
Thank you for the corrections!
$endgroup$
– Your Common Sense
25 mins ago
$begingroup$
This is very interesting. I don't have a lot of experience with php so you've shown me quite a bit. In line 3 of your code I simply changed $dir to $pattern, and in line 18 I changed $title to $match[1], since $title no longer exists. I'm still reviewing it but the result is the same as before which is good. Are the overall mechanics of what I'm doing (looping once to capture data, then looping 2 more times to template the content) reasonable?
$endgroup$
– Jozurcrunch
4 hours ago
$begingroup$
This is very interesting. I don't have a lot of experience with php so you've shown me quite a bit. In line 3 of your code I simply changed $dir to $pattern, and in line 18 I changed $title to $match[1], since $title no longer exists. I'm still reviewing it but the result is the same as before which is good. Are the overall mechanics of what I'm doing (looping once to capture data, then looping 2 more times to template the content) reasonable?
$endgroup$
– Jozurcrunch
4 hours ago
$begingroup$
Looping 2 times is the only acceptable way. Data preparation should be always separated from the output as output may vary
$endgroup$
– Your Common Sense
27 mins ago
$begingroup$
Looping 2 times is the only acceptable way. Data preparation should be always separated from the output as output may vary
$endgroup$
– Your Common Sense
27 mins ago
$begingroup$
Thank you for the corrections!
$endgroup$
– Your Common Sense
25 mins ago
$begingroup$
Thank you for the corrections!
$endgroup$
– Your Common Sense
25 mins ago
add a comment |
Jozurcrunch is a new contributor. Be nice, and check out our Code of Conduct.
Jozurcrunch is a new contributor. Be nice, and check out our Code of Conduct.
Jozurcrunch is a new contributor. Be nice, and check out our Code of Conduct.
Jozurcrunch is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f212298%2faggregating-html-files%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
V0nciCICTWy r8ScBuOA6ewOxTLPnhiAJBJJ d,m2dA9K mrtGqcPpuJrO8fW,dq,ur6pVK9JdfVlsMH