Change variable mapped in Leaflet and Shiny according to user input
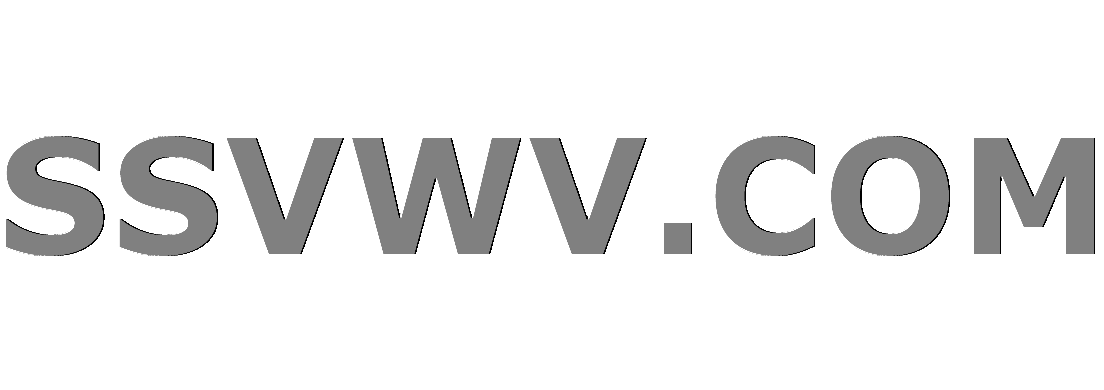
Multi tool use
Update: I have figured out how to do this, but it only works in the HTML viewer pane in R-Studio.
The map pane works fine in the HTML viewer, but is slow and 9/10 times disconnects from the server (I imagine it times out) when deployed.
I have tried to use isolate() and actionButtons(), but this didn't help.
My repo: https://github.com/MirandaLupion/GOV_1005_Final_Project
(app is in navbarApp folder)
Deployed app: https://mlupion.shinyapps.io/Russian_crime_data/
The data itself is large. I use the filter feature in the reactive to filter for only the year needed and then select only the variable needed. This worked fine when the only input option was year. Might is be causing the issue, now that it also facilitates the indicator input? Should I do this in two reactive statements?
Original post:
I am new to Leaflet and Shiny, and I haven't found a suitable answer to this question, but my apologies if someone has already asked this.
I want the user to select the variable, which is then used to generate a choropleth map in my shapefile. The shapefile and map works fine when I manually insert the variable (replacing the variable map_var
here with CRIMESHARE
for instance) in the code. However, when I try to replace that variable with input$y
, Shiny throws an era: no applicable method for 'rescale' applied to an object of class "character"
In the current code, I try to save the input$y
as a variable map_var
. This doesn't work. I have also tried saving the input this way in a reactive statement, but it also failed.
The reactive year input works correctly.
I am not sure I can create a reproducible example, as it involves a shape file. I appreciate any help/suggestions.
Here are the relevant parts of my code below:
Data prep
# Load libraries
library(shiny)
library(tidyverse)
library(stringr)
library(rsconnect)
library(leaflet)
library(rgdal)
library(shinythemes)
library(plotly)
# Read in the data
crime_master <- read_rds("r_4_tidy.rds")
# Prepare two data sets
# one for the plot (not shown) and one for the map
crime_plot <- crime_master
crime_map <- crime_master %>%
mutate(YEAR = as.character(YEAR))
# Prepare the shape file for the map
# Read it in
# Project the shape file
rf_map <- readOGR(dsn = "/Users/me/Desktop/folder
/Project/RUS_adm", layer = "RUS_adm1")
rf_map <- spTransform(rf_map, CRS("+init=epsg:4326"))
crime_options <- c("Road accidents" = "ROADACCIDENT",
"Victims of road accidents" = "ROADVICTIM",
"Crime share" = "CRIMESHARE",
"Murders" = "MURDER",
"Incidences of rape" = "RAPE",
"Robberies" = "ROBBERY",
"Incidences of hooliganism" = "HOOLIGANISM",
"White-collar crimes" = "ECONCRIME",
"Incidences of juvenile crime" = "JUVENILECRIME")
The UI
ui <- fluidPage(theme = shinytheme("cerulean"),
sidebarLayout(
sidebarPanel(
# Let users select the year to map
selectInput(inputId = "year", #internal label
label = "Year to map", #label that user sees
choices = c(crime_map$YEAR), #vector of choices for user to pick from
selected = "1990"),
# Let users select the indicator to map
selectInput(inputId = "y", # internal label
label = "Indicator to display on map", # label that user sees
choices = crime_options, # vector of choices for user to pick from
selected = crime_options[3])),
mainPanel(
tabsetPanel(type = "tabs",
tabPanel("Map an indicator",
leafletOutput("map",
width = "100%",
height = "500px"))))))
The server
server <- function(input, output){
# Reactive that filters the crime_map data for the user-selected year
map_subset <- reactive({
req(input$year)
filter(crime_map, YEAR == input$year)
})
# Map output
# Merge the shapefile with the sub_setted map data
# Color by the selected indicator
# Set the options for the leaflet viewer
# Allow the user to drag
# Add a CartoDB base map
# Set the default view
# Set the max bounds
# Add the shapefile with labels
# Color by the coloring set up
output$map <- renderLeaflet({
map_var <- input$y
rf_map <- merge(rf_map, map_subset(), by = "ID_1", duplicateGeoms =
TRUE)
coloring <- colorNumeric(palette = "Blues",
domain = rf_map@data$map_var)
m <- rf_map %>%
leaflet(options = leafletOptions(dragging = TRUE)) %>%
addProviderTiles(provider = "CartoDB") %>%
setView(lng = 37.618423, lat = 55.751244, zoom = 3) %>%
setMaxBounds(lng1 = 40, lat1 = 30, lng2 = 150, lat2 = 100) %>%
addPolygons(weight = 1,
label = ~paste0(NAME, ", ", map_var),
color = ~coloring(map_var)) %>%
# Add a legend in the bottom
addLegend("bottomright",
pal = coloring,
values = ~map_var,
title = "title here",
opacity = 1)
m})}
shinyApp(ui = ui, server = server)
Thank you!
r shiny leaflet
add a comment |
Update: I have figured out how to do this, but it only works in the HTML viewer pane in R-Studio.
The map pane works fine in the HTML viewer, but is slow and 9/10 times disconnects from the server (I imagine it times out) when deployed.
I have tried to use isolate() and actionButtons(), but this didn't help.
My repo: https://github.com/MirandaLupion/GOV_1005_Final_Project
(app is in navbarApp folder)
Deployed app: https://mlupion.shinyapps.io/Russian_crime_data/
The data itself is large. I use the filter feature in the reactive to filter for only the year needed and then select only the variable needed. This worked fine when the only input option was year. Might is be causing the issue, now that it also facilitates the indicator input? Should I do this in two reactive statements?
Original post:
I am new to Leaflet and Shiny, and I haven't found a suitable answer to this question, but my apologies if someone has already asked this.
I want the user to select the variable, which is then used to generate a choropleth map in my shapefile. The shapefile and map works fine when I manually insert the variable (replacing the variable map_var
here with CRIMESHARE
for instance) in the code. However, when I try to replace that variable with input$y
, Shiny throws an era: no applicable method for 'rescale' applied to an object of class "character"
In the current code, I try to save the input$y
as a variable map_var
. This doesn't work. I have also tried saving the input this way in a reactive statement, but it also failed.
The reactive year input works correctly.
I am not sure I can create a reproducible example, as it involves a shape file. I appreciate any help/suggestions.
Here are the relevant parts of my code below:
Data prep
# Load libraries
library(shiny)
library(tidyverse)
library(stringr)
library(rsconnect)
library(leaflet)
library(rgdal)
library(shinythemes)
library(plotly)
# Read in the data
crime_master <- read_rds("r_4_tidy.rds")
# Prepare two data sets
# one for the plot (not shown) and one for the map
crime_plot <- crime_master
crime_map <- crime_master %>%
mutate(YEAR = as.character(YEAR))
# Prepare the shape file for the map
# Read it in
# Project the shape file
rf_map <- readOGR(dsn = "/Users/me/Desktop/folder
/Project/RUS_adm", layer = "RUS_adm1")
rf_map <- spTransform(rf_map, CRS("+init=epsg:4326"))
crime_options <- c("Road accidents" = "ROADACCIDENT",
"Victims of road accidents" = "ROADVICTIM",
"Crime share" = "CRIMESHARE",
"Murders" = "MURDER",
"Incidences of rape" = "RAPE",
"Robberies" = "ROBBERY",
"Incidences of hooliganism" = "HOOLIGANISM",
"White-collar crimes" = "ECONCRIME",
"Incidences of juvenile crime" = "JUVENILECRIME")
The UI
ui <- fluidPage(theme = shinytheme("cerulean"),
sidebarLayout(
sidebarPanel(
# Let users select the year to map
selectInput(inputId = "year", #internal label
label = "Year to map", #label that user sees
choices = c(crime_map$YEAR), #vector of choices for user to pick from
selected = "1990"),
# Let users select the indicator to map
selectInput(inputId = "y", # internal label
label = "Indicator to display on map", # label that user sees
choices = crime_options, # vector of choices for user to pick from
selected = crime_options[3])),
mainPanel(
tabsetPanel(type = "tabs",
tabPanel("Map an indicator",
leafletOutput("map",
width = "100%",
height = "500px"))))))
The server
server <- function(input, output){
# Reactive that filters the crime_map data for the user-selected year
map_subset <- reactive({
req(input$year)
filter(crime_map, YEAR == input$year)
})
# Map output
# Merge the shapefile with the sub_setted map data
# Color by the selected indicator
# Set the options for the leaflet viewer
# Allow the user to drag
# Add a CartoDB base map
# Set the default view
# Set the max bounds
# Add the shapefile with labels
# Color by the coloring set up
output$map <- renderLeaflet({
map_var <- input$y
rf_map <- merge(rf_map, map_subset(), by = "ID_1", duplicateGeoms =
TRUE)
coloring <- colorNumeric(palette = "Blues",
domain = rf_map@data$map_var)
m <- rf_map %>%
leaflet(options = leafletOptions(dragging = TRUE)) %>%
addProviderTiles(provider = "CartoDB") %>%
setView(lng = 37.618423, lat = 55.751244, zoom = 3) %>%
setMaxBounds(lng1 = 40, lat1 = 30, lng2 = 150, lat2 = 100) %>%
addPolygons(weight = 1,
label = ~paste0(NAME, ", ", map_var),
color = ~coloring(map_var)) %>%
# Add a legend in the bottom
addLegend("bottomright",
pal = coloring,
values = ~map_var,
title = "title here",
opacity = 1)
m})}
shinyApp(ui = ui, server = server)
Thank you!
r shiny leaflet
add a comment |
Update: I have figured out how to do this, but it only works in the HTML viewer pane in R-Studio.
The map pane works fine in the HTML viewer, but is slow and 9/10 times disconnects from the server (I imagine it times out) when deployed.
I have tried to use isolate() and actionButtons(), but this didn't help.
My repo: https://github.com/MirandaLupion/GOV_1005_Final_Project
(app is in navbarApp folder)
Deployed app: https://mlupion.shinyapps.io/Russian_crime_data/
The data itself is large. I use the filter feature in the reactive to filter for only the year needed and then select only the variable needed. This worked fine when the only input option was year. Might is be causing the issue, now that it also facilitates the indicator input? Should I do this in two reactive statements?
Original post:
I am new to Leaflet and Shiny, and I haven't found a suitable answer to this question, but my apologies if someone has already asked this.
I want the user to select the variable, which is then used to generate a choropleth map in my shapefile. The shapefile and map works fine when I manually insert the variable (replacing the variable map_var
here with CRIMESHARE
for instance) in the code. However, when I try to replace that variable with input$y
, Shiny throws an era: no applicable method for 'rescale' applied to an object of class "character"
In the current code, I try to save the input$y
as a variable map_var
. This doesn't work. I have also tried saving the input this way in a reactive statement, but it also failed.
The reactive year input works correctly.
I am not sure I can create a reproducible example, as it involves a shape file. I appreciate any help/suggestions.
Here are the relevant parts of my code below:
Data prep
# Load libraries
library(shiny)
library(tidyverse)
library(stringr)
library(rsconnect)
library(leaflet)
library(rgdal)
library(shinythemes)
library(plotly)
# Read in the data
crime_master <- read_rds("r_4_tidy.rds")
# Prepare two data sets
# one for the plot (not shown) and one for the map
crime_plot <- crime_master
crime_map <- crime_master %>%
mutate(YEAR = as.character(YEAR))
# Prepare the shape file for the map
# Read it in
# Project the shape file
rf_map <- readOGR(dsn = "/Users/me/Desktop/folder
/Project/RUS_adm", layer = "RUS_adm1")
rf_map <- spTransform(rf_map, CRS("+init=epsg:4326"))
crime_options <- c("Road accidents" = "ROADACCIDENT",
"Victims of road accidents" = "ROADVICTIM",
"Crime share" = "CRIMESHARE",
"Murders" = "MURDER",
"Incidences of rape" = "RAPE",
"Robberies" = "ROBBERY",
"Incidences of hooliganism" = "HOOLIGANISM",
"White-collar crimes" = "ECONCRIME",
"Incidences of juvenile crime" = "JUVENILECRIME")
The UI
ui <- fluidPage(theme = shinytheme("cerulean"),
sidebarLayout(
sidebarPanel(
# Let users select the year to map
selectInput(inputId = "year", #internal label
label = "Year to map", #label that user sees
choices = c(crime_map$YEAR), #vector of choices for user to pick from
selected = "1990"),
# Let users select the indicator to map
selectInput(inputId = "y", # internal label
label = "Indicator to display on map", # label that user sees
choices = crime_options, # vector of choices for user to pick from
selected = crime_options[3])),
mainPanel(
tabsetPanel(type = "tabs",
tabPanel("Map an indicator",
leafletOutput("map",
width = "100%",
height = "500px"))))))
The server
server <- function(input, output){
# Reactive that filters the crime_map data for the user-selected year
map_subset <- reactive({
req(input$year)
filter(crime_map, YEAR == input$year)
})
# Map output
# Merge the shapefile with the sub_setted map data
# Color by the selected indicator
# Set the options for the leaflet viewer
# Allow the user to drag
# Add a CartoDB base map
# Set the default view
# Set the max bounds
# Add the shapefile with labels
# Color by the coloring set up
output$map <- renderLeaflet({
map_var <- input$y
rf_map <- merge(rf_map, map_subset(), by = "ID_1", duplicateGeoms =
TRUE)
coloring <- colorNumeric(palette = "Blues",
domain = rf_map@data$map_var)
m <- rf_map %>%
leaflet(options = leafletOptions(dragging = TRUE)) %>%
addProviderTiles(provider = "CartoDB") %>%
setView(lng = 37.618423, lat = 55.751244, zoom = 3) %>%
setMaxBounds(lng1 = 40, lat1 = 30, lng2 = 150, lat2 = 100) %>%
addPolygons(weight = 1,
label = ~paste0(NAME, ", ", map_var),
color = ~coloring(map_var)) %>%
# Add a legend in the bottom
addLegend("bottomright",
pal = coloring,
values = ~map_var,
title = "title here",
opacity = 1)
m})}
shinyApp(ui = ui, server = server)
Thank you!
r shiny leaflet
Update: I have figured out how to do this, but it only works in the HTML viewer pane in R-Studio.
The map pane works fine in the HTML viewer, but is slow and 9/10 times disconnects from the server (I imagine it times out) when deployed.
I have tried to use isolate() and actionButtons(), but this didn't help.
My repo: https://github.com/MirandaLupion/GOV_1005_Final_Project
(app is in navbarApp folder)
Deployed app: https://mlupion.shinyapps.io/Russian_crime_data/
The data itself is large. I use the filter feature in the reactive to filter for only the year needed and then select only the variable needed. This worked fine when the only input option was year. Might is be causing the issue, now that it also facilitates the indicator input? Should I do this in two reactive statements?
Original post:
I am new to Leaflet and Shiny, and I haven't found a suitable answer to this question, but my apologies if someone has already asked this.
I want the user to select the variable, which is then used to generate a choropleth map in my shapefile. The shapefile and map works fine when I manually insert the variable (replacing the variable map_var
here with CRIMESHARE
for instance) in the code. However, when I try to replace that variable with input$y
, Shiny throws an era: no applicable method for 'rescale' applied to an object of class "character"
In the current code, I try to save the input$y
as a variable map_var
. This doesn't work. I have also tried saving the input this way in a reactive statement, but it also failed.
The reactive year input works correctly.
I am not sure I can create a reproducible example, as it involves a shape file. I appreciate any help/suggestions.
Here are the relevant parts of my code below:
Data prep
# Load libraries
library(shiny)
library(tidyverse)
library(stringr)
library(rsconnect)
library(leaflet)
library(rgdal)
library(shinythemes)
library(plotly)
# Read in the data
crime_master <- read_rds("r_4_tidy.rds")
# Prepare two data sets
# one for the plot (not shown) and one for the map
crime_plot <- crime_master
crime_map <- crime_master %>%
mutate(YEAR = as.character(YEAR))
# Prepare the shape file for the map
# Read it in
# Project the shape file
rf_map <- readOGR(dsn = "/Users/me/Desktop/folder
/Project/RUS_adm", layer = "RUS_adm1")
rf_map <- spTransform(rf_map, CRS("+init=epsg:4326"))
crime_options <- c("Road accidents" = "ROADACCIDENT",
"Victims of road accidents" = "ROADVICTIM",
"Crime share" = "CRIMESHARE",
"Murders" = "MURDER",
"Incidences of rape" = "RAPE",
"Robberies" = "ROBBERY",
"Incidences of hooliganism" = "HOOLIGANISM",
"White-collar crimes" = "ECONCRIME",
"Incidences of juvenile crime" = "JUVENILECRIME")
The UI
ui <- fluidPage(theme = shinytheme("cerulean"),
sidebarLayout(
sidebarPanel(
# Let users select the year to map
selectInput(inputId = "year", #internal label
label = "Year to map", #label that user sees
choices = c(crime_map$YEAR), #vector of choices for user to pick from
selected = "1990"),
# Let users select the indicator to map
selectInput(inputId = "y", # internal label
label = "Indicator to display on map", # label that user sees
choices = crime_options, # vector of choices for user to pick from
selected = crime_options[3])),
mainPanel(
tabsetPanel(type = "tabs",
tabPanel("Map an indicator",
leafletOutput("map",
width = "100%",
height = "500px"))))))
The server
server <- function(input, output){
# Reactive that filters the crime_map data for the user-selected year
map_subset <- reactive({
req(input$year)
filter(crime_map, YEAR == input$year)
})
# Map output
# Merge the shapefile with the sub_setted map data
# Color by the selected indicator
# Set the options for the leaflet viewer
# Allow the user to drag
# Add a CartoDB base map
# Set the default view
# Set the max bounds
# Add the shapefile with labels
# Color by the coloring set up
output$map <- renderLeaflet({
map_var <- input$y
rf_map <- merge(rf_map, map_subset(), by = "ID_1", duplicateGeoms =
TRUE)
coloring <- colorNumeric(palette = "Blues",
domain = rf_map@data$map_var)
m <- rf_map %>%
leaflet(options = leafletOptions(dragging = TRUE)) %>%
addProviderTiles(provider = "CartoDB") %>%
setView(lng = 37.618423, lat = 55.751244, zoom = 3) %>%
setMaxBounds(lng1 = 40, lat1 = 30, lng2 = 150, lat2 = 100) %>%
addPolygons(weight = 1,
label = ~paste0(NAME, ", ", map_var),
color = ~coloring(map_var)) %>%
# Add a legend in the bottom
addLegend("bottomright",
pal = coloring,
values = ~map_var,
title = "title here",
opacity = 1)
m})}
shinyApp(ui = ui, server = server)
Thank you!
r shiny leaflet
r shiny leaflet
edited Nov 27 '18 at 14:35
loops
asked Nov 23 '18 at 0:32
loopsloops
53
53
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439410%2fchange-variable-mapped-in-leaflet-and-shiny-according-to-user-input%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439410%2fchange-variable-mapped-in-leaflet-and-shiny-according-to-user-input%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
K hxV,NpIR4o 2S28uPsHSFiuhslZrl ZvkP Lt3K3