How to create URL for Logout action in WebApi
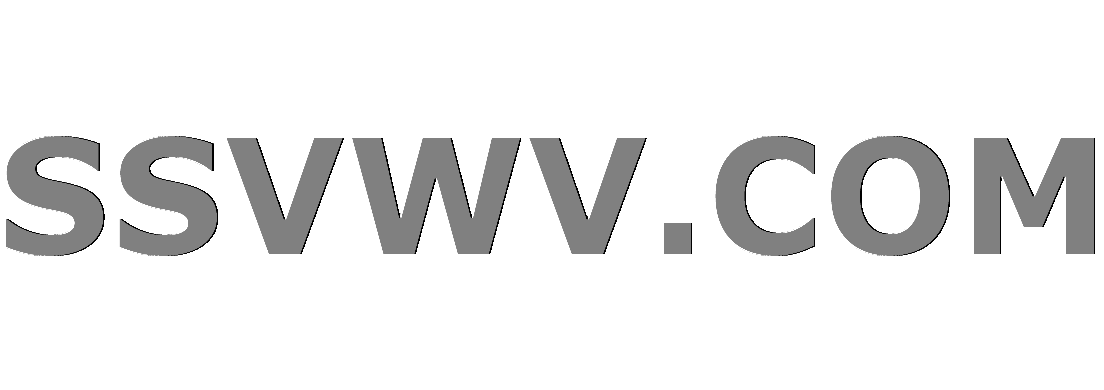
Multi tool use
I am using Visual Studio 2017..... when I created the project, an AccountController was created with this action:
// POST api/Account/Logout
[Route("Logout")]
public IHttpActionResult Logout()
{
Authentication.SignOut(CookieAuthenticationDefaults.AuthenticationType);
return Ok();
}
On the other hand, this route was created by default:
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
I need to do a very simple thing. How can I get the Logout URL in a view?
I tried
@Url.Action("Logout", "Account", new { httproute = "DefaultApi" })
But it did not work since DefaultApi does not contain the action, causing the action to be added as a query string parameter.
If I don't use httproute property, the URL is built but without the "api" part causing the framework to not find it.
I have even tried
@Url.RouteUrl("DefaultApi", new { httproute = "Logout", controller = "Account" })">
with no success either.
asp.net-mvc asp.net-web-api routes
add a comment |
I am using Visual Studio 2017..... when I created the project, an AccountController was created with this action:
// POST api/Account/Logout
[Route("Logout")]
public IHttpActionResult Logout()
{
Authentication.SignOut(CookieAuthenticationDefaults.AuthenticationType);
return Ok();
}
On the other hand, this route was created by default:
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
I need to do a very simple thing. How can I get the Logout URL in a view?
I tried
@Url.Action("Logout", "Account", new { httproute = "DefaultApi" })
But it did not work since DefaultApi does not contain the action, causing the action to be added as a query string parameter.
If I don't use httproute property, the URL is built but without the "api" part causing the framework to not find it.
I have even tried
@Url.RouteUrl("DefaultApi", new { httproute = "Logout", controller = "Account" })">
with no success either.
asp.net-mvc asp.net-web-api routes
How about using@Url.HttpRouteUrl()
? I see that you're not tried this helper before, see related issue here: stackoverflow.com/questions/19868148/…. Also make sure that the routes are in proper order.
– Tetsuya Yamamoto
Nov 21 at 1:41
add a comment |
I am using Visual Studio 2017..... when I created the project, an AccountController was created with this action:
// POST api/Account/Logout
[Route("Logout")]
public IHttpActionResult Logout()
{
Authentication.SignOut(CookieAuthenticationDefaults.AuthenticationType);
return Ok();
}
On the other hand, this route was created by default:
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
I need to do a very simple thing. How can I get the Logout URL in a view?
I tried
@Url.Action("Logout", "Account", new { httproute = "DefaultApi" })
But it did not work since DefaultApi does not contain the action, causing the action to be added as a query string parameter.
If I don't use httproute property, the URL is built but without the "api" part causing the framework to not find it.
I have even tried
@Url.RouteUrl("DefaultApi", new { httproute = "Logout", controller = "Account" })">
with no success either.
asp.net-mvc asp.net-web-api routes
I am using Visual Studio 2017..... when I created the project, an AccountController was created with this action:
// POST api/Account/Logout
[Route("Logout")]
public IHttpActionResult Logout()
{
Authentication.SignOut(CookieAuthenticationDefaults.AuthenticationType);
return Ok();
}
On the other hand, this route was created by default:
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
I need to do a very simple thing. How can I get the Logout URL in a view?
I tried
@Url.Action("Logout", "Account", new { httproute = "DefaultApi" })
But it did not work since DefaultApi does not contain the action, causing the action to be added as a query string parameter.
If I don't use httproute property, the URL is built but without the "api" part causing the framework to not find it.
I have even tried
@Url.RouteUrl("DefaultApi", new { httproute = "Logout", controller = "Account" })">
with no success either.
asp.net-mvc asp.net-web-api routes
asp.net-mvc asp.net-web-api routes
edited Nov 21 at 0:39
asked Nov 21 at 0:31
jstuardo
97352860
97352860
How about using@Url.HttpRouteUrl()
? I see that you're not tried this helper before, see related issue here: stackoverflow.com/questions/19868148/…. Also make sure that the routes are in proper order.
– Tetsuya Yamamoto
Nov 21 at 1:41
add a comment |
How about using@Url.HttpRouteUrl()
? I see that you're not tried this helper before, see related issue here: stackoverflow.com/questions/19868148/…. Also make sure that the routes are in proper order.
– Tetsuya Yamamoto
Nov 21 at 1:41
How about using
@Url.HttpRouteUrl()
? I see that you're not tried this helper before, see related issue here: stackoverflow.com/questions/19868148/…. Also make sure that the routes are in proper order.– Tetsuya Yamamoto
Nov 21 at 1:41
How about using
@Url.HttpRouteUrl()
? I see that you're not tried this helper before, see related issue here: stackoverflow.com/questions/19868148/…. Also make sure that the routes are in proper order.– Tetsuya Yamamoto
Nov 21 at 1:41
add a comment |
1 Answer
1
active
oldest
votes
You can define a new route to define your action name:
routes.MapHttpRoute(
name: "ActionApi",
routeTemplate: "api/{controller}/{action}/{id}",
defaults: new { id = RouteParameter.Optional }
);
If you don't include the action name, Web API tries to find a suitable action for you based on your HTTP verb... for example if you send a Get
request Web API tries to find an action starting with 'Get'... since your action name is Logout
, the default API routing convention cannot match it to a request. see here for more info
Then this link should call the action (see here):
@Url.HttpRouteUrl("ActionApi", new {controller = "Account", action = "Logout"})
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403659%2fhow-to-create-url-for-logout-action-in-webapi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can define a new route to define your action name:
routes.MapHttpRoute(
name: "ActionApi",
routeTemplate: "api/{controller}/{action}/{id}",
defaults: new { id = RouteParameter.Optional }
);
If you don't include the action name, Web API tries to find a suitable action for you based on your HTTP verb... for example if you send a Get
request Web API tries to find an action starting with 'Get'... since your action name is Logout
, the default API routing convention cannot match it to a request. see here for more info
Then this link should call the action (see here):
@Url.HttpRouteUrl("ActionApi", new {controller = "Account", action = "Logout"})
add a comment |
You can define a new route to define your action name:
routes.MapHttpRoute(
name: "ActionApi",
routeTemplate: "api/{controller}/{action}/{id}",
defaults: new { id = RouteParameter.Optional }
);
If you don't include the action name, Web API tries to find a suitable action for you based on your HTTP verb... for example if you send a Get
request Web API tries to find an action starting with 'Get'... since your action name is Logout
, the default API routing convention cannot match it to a request. see here for more info
Then this link should call the action (see here):
@Url.HttpRouteUrl("ActionApi", new {controller = "Account", action = "Logout"})
add a comment |
You can define a new route to define your action name:
routes.MapHttpRoute(
name: "ActionApi",
routeTemplate: "api/{controller}/{action}/{id}",
defaults: new { id = RouteParameter.Optional }
);
If you don't include the action name, Web API tries to find a suitable action for you based on your HTTP verb... for example if you send a Get
request Web API tries to find an action starting with 'Get'... since your action name is Logout
, the default API routing convention cannot match it to a request. see here for more info
Then this link should call the action (see here):
@Url.HttpRouteUrl("ActionApi", new {controller = "Account", action = "Logout"})
You can define a new route to define your action name:
routes.MapHttpRoute(
name: "ActionApi",
routeTemplate: "api/{controller}/{action}/{id}",
defaults: new { id = RouteParameter.Optional }
);
If you don't include the action name, Web API tries to find a suitable action for you based on your HTTP verb... for example if you send a Get
request Web API tries to find an action starting with 'Get'... since your action name is Logout
, the default API routing convention cannot match it to a request. see here for more info
Then this link should call the action (see here):
@Url.HttpRouteUrl("ActionApi", new {controller = "Account", action = "Logout"})
edited Nov 21 at 4:16
answered Nov 21 at 3:42
Hooman
3,1723730
3,1723730
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403659%2fhow-to-create-url-for-logout-action-in-webapi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tQjMfmPcgLv82qANj6os kvOg1K9dT 7ILdiBbhWDIgNIP4Z
How about using
@Url.HttpRouteUrl()
? I see that you're not tried this helper before, see related issue here: stackoverflow.com/questions/19868148/…. Also make sure that the routes are in proper order.– Tetsuya Yamamoto
Nov 21 at 1:41