UnauthorizedAccessException - Trying to serialize JSON to a file
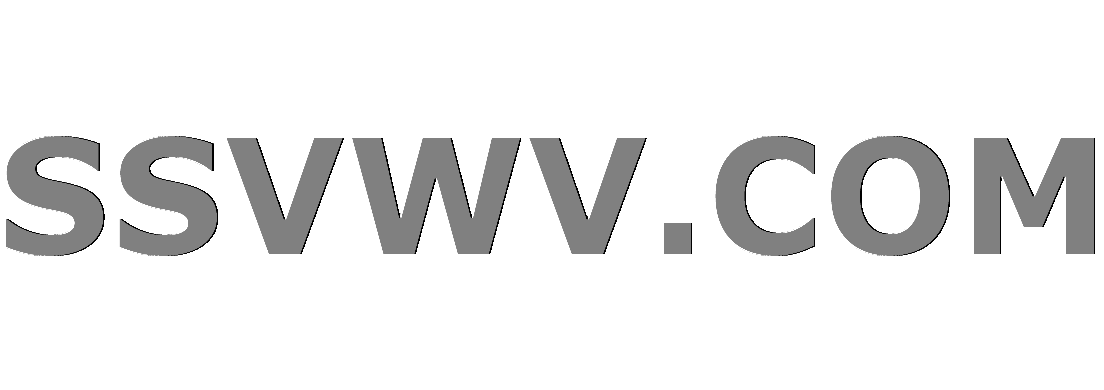
Multi tool use
I am trying to produce a JSON file after parsing it.
In the code below, I am choosing the path to where the specified JSON file is going to appear.
public class Movie
{
public string Name { get; set; }
public int Year { get; set; }
}
class Parsing
{
static void Main(string args)
{
//Just a movie object for the sake of testing
Movie movie = new Movie
{
Name = "Bad Boys",
Year = 1995
};
try
{
// serialize JSON to a string and then write string to a file
File.WriteAllText(@"c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON", JsonConvert.SerializeObject(movie));
// serialize JSON directly to a file
using (StreamWriter file = File.CreateText(@"c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON"))
{
JsonSerializer serializer = new JsonSerializer();
serializer.Serialize(file, movie);
}
}
catch (UnauthorizedAccessException e)
{
Console.WriteLine(e.ToString());
}
}
}
However, the issue is that I keep having an UnauthorizedAccessException
. I have given access to the folder and I have also tried running the program as an administrator.
Everything I found online suggested those two methods, yet they didn't work.
c# .net parsing
add a comment |
I am trying to produce a JSON file after parsing it.
In the code below, I am choosing the path to where the specified JSON file is going to appear.
public class Movie
{
public string Name { get; set; }
public int Year { get; set; }
}
class Parsing
{
static void Main(string args)
{
//Just a movie object for the sake of testing
Movie movie = new Movie
{
Name = "Bad Boys",
Year = 1995
};
try
{
// serialize JSON to a string and then write string to a file
File.WriteAllText(@"c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON", JsonConvert.SerializeObject(movie));
// serialize JSON directly to a file
using (StreamWriter file = File.CreateText(@"c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON"))
{
JsonSerializer serializer = new JsonSerializer();
serializer.Serialize(file, movie);
}
}
catch (UnauthorizedAccessException e)
{
Console.WriteLine(e.ToString());
}
}
}
However, the issue is that I keep having an UnauthorizedAccessException
. I have given access to the folder and I have also tried running the program as an administrator.
Everything I found online suggested those two methods, yet they didn't work.
c# .net parsing
Please update your question with the full exception
– riQQ
Nov 20 at 22:03
add a comment |
I am trying to produce a JSON file after parsing it.
In the code below, I am choosing the path to where the specified JSON file is going to appear.
public class Movie
{
public string Name { get; set; }
public int Year { get; set; }
}
class Parsing
{
static void Main(string args)
{
//Just a movie object for the sake of testing
Movie movie = new Movie
{
Name = "Bad Boys",
Year = 1995
};
try
{
// serialize JSON to a string and then write string to a file
File.WriteAllText(@"c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON", JsonConvert.SerializeObject(movie));
// serialize JSON directly to a file
using (StreamWriter file = File.CreateText(@"c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON"))
{
JsonSerializer serializer = new JsonSerializer();
serializer.Serialize(file, movie);
}
}
catch (UnauthorizedAccessException e)
{
Console.WriteLine(e.ToString());
}
}
}
However, the issue is that I keep having an UnauthorizedAccessException
. I have given access to the folder and I have also tried running the program as an administrator.
Everything I found online suggested those two methods, yet they didn't work.
c# .net parsing
I am trying to produce a JSON file after parsing it.
In the code below, I am choosing the path to where the specified JSON file is going to appear.
public class Movie
{
public string Name { get; set; }
public int Year { get; set; }
}
class Parsing
{
static void Main(string args)
{
//Just a movie object for the sake of testing
Movie movie = new Movie
{
Name = "Bad Boys",
Year = 1995
};
try
{
// serialize JSON to a string and then write string to a file
File.WriteAllText(@"c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON", JsonConvert.SerializeObject(movie));
// serialize JSON directly to a file
using (StreamWriter file = File.CreateText(@"c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON"))
{
JsonSerializer serializer = new JsonSerializer();
serializer.Serialize(file, movie);
}
}
catch (UnauthorizedAccessException e)
{
Console.WriteLine(e.ToString());
}
}
}
However, the issue is that I keep having an UnauthorizedAccessException
. I have given access to the folder and I have also tried running the program as an administrator.
Everything I found online suggested those two methods, yet they didn't work.
c# .net parsing
c# .net parsing
edited Nov 20 at 21:57
Lews Therin
2,41711437
2,41711437
asked Nov 20 at 21:43


Naief Jobsen
75
75
Please update your question with the full exception
– riQQ
Nov 20 at 22:03
add a comment |
Please update your question with the full exception
– riQQ
Nov 20 at 22:03
Please update your question with the full exception
– riQQ
Nov 20 at 22:03
Please update your question with the full exception
– riQQ
Nov 20 at 22:03
add a comment |
2 Answers
2
active
oldest
votes
You are both using a verbatim string literal (@""
) and escaping your path's backslashes. The @
before a string literal will cause it to ignore escaped backslashes. So it's literally trying to write to c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON
, which is an invalid path in Windows.
On top of that, you are only providing a folder in the path. File.WriteAllText()
and File.CreateText()
require a file name and file extension as well.
Also, you should probably move your path to a const
variable:
const string path = @"c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSONtest.json";
// ...
File.WriteAllText(path, JsonConvert.SerializeObject(movie));
// ...
using (StreamWriter file = File.CreateText(path))
// ...
1
Thank you, it was the @. Also, I was going to do create a const variable but thanks for the tip either way!
– Naief Jobsen
Nov 20 at 23:09
Glad I could help.
– Lews Therin
Nov 21 at 14:19
add a comment |
I believe your code should work.
You could try to open the command prompt and type
cd c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSON
then
dir > test.txt
If you get an "Access is denied" message, it's because you really don't have sufficient rights to write into that folder.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402029%2funauthorizedaccessexception-trying-to-serialize-json-to-a-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are both using a verbatim string literal (@""
) and escaping your path's backslashes. The @
before a string literal will cause it to ignore escaped backslashes. So it's literally trying to write to c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON
, which is an invalid path in Windows.
On top of that, you are only providing a folder in the path. File.WriteAllText()
and File.CreateText()
require a file name and file extension as well.
Also, you should probably move your path to a const
variable:
const string path = @"c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSONtest.json";
// ...
File.WriteAllText(path, JsonConvert.SerializeObject(movie));
// ...
using (StreamWriter file = File.CreateText(path))
// ...
1
Thank you, it was the @. Also, I was going to do create a const variable but thanks for the tip either way!
– Naief Jobsen
Nov 20 at 23:09
Glad I could help.
– Lews Therin
Nov 21 at 14:19
add a comment |
You are both using a verbatim string literal (@""
) and escaping your path's backslashes. The @
before a string literal will cause it to ignore escaped backslashes. So it's literally trying to write to c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON
, which is an invalid path in Windows.
On top of that, you are only providing a folder in the path. File.WriteAllText()
and File.CreateText()
require a file name and file extension as well.
Also, you should probably move your path to a const
variable:
const string path = @"c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSONtest.json";
// ...
File.WriteAllText(path, JsonConvert.SerializeObject(movie));
// ...
using (StreamWriter file = File.CreateText(path))
// ...
1
Thank you, it was the @. Also, I was going to do create a const variable but thanks for the tip either way!
– Naief Jobsen
Nov 20 at 23:09
Glad I could help.
– Lews Therin
Nov 21 at 14:19
add a comment |
You are both using a verbatim string literal (@""
) and escaping your path's backslashes. The @
before a string literal will cause it to ignore escaped backslashes. So it's literally trying to write to c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON
, which is an invalid path in Windows.
On top of that, you are only providing a folder in the path. File.WriteAllText()
and File.CreateText()
require a file name and file extension as well.
Also, you should probably move your path to a const
variable:
const string path = @"c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSONtest.json";
// ...
File.WriteAllText(path, JsonConvert.SerializeObject(movie));
// ...
using (StreamWriter file = File.CreateText(path))
// ...
You are both using a verbatim string literal (@""
) and escaping your path's backslashes. The @
before a string literal will cause it to ignore escaped backslashes. So it's literally trying to write to c:\Users\LOrdBenche\source\repos\parsingTest\parsingTest\producedJSON
, which is an invalid path in Windows.
On top of that, you are only providing a folder in the path. File.WriteAllText()
and File.CreateText()
require a file name and file extension as well.
Also, you should probably move your path to a const
variable:
const string path = @"c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSONtest.json";
// ...
File.WriteAllText(path, JsonConvert.SerializeObject(movie));
// ...
using (StreamWriter file = File.CreateText(path))
// ...
answered Nov 20 at 22:05
Lews Therin
2,41711437
2,41711437
1
Thank you, it was the @. Also, I was going to do create a const variable but thanks for the tip either way!
– Naief Jobsen
Nov 20 at 23:09
Glad I could help.
– Lews Therin
Nov 21 at 14:19
add a comment |
1
Thank you, it was the @. Also, I was going to do create a const variable but thanks for the tip either way!
– Naief Jobsen
Nov 20 at 23:09
Glad I could help.
– Lews Therin
Nov 21 at 14:19
1
1
Thank you, it was the @. Also, I was going to do create a const variable but thanks for the tip either way!
– Naief Jobsen
Nov 20 at 23:09
Thank you, it was the @. Also, I was going to do create a const variable but thanks for the tip either way!
– Naief Jobsen
Nov 20 at 23:09
Glad I could help.
– Lews Therin
Nov 21 at 14:19
Glad I could help.
– Lews Therin
Nov 21 at 14:19
add a comment |
I believe your code should work.
You could try to open the command prompt and type
cd c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSON
then
dir > test.txt
If you get an "Access is denied" message, it's because you really don't have sufficient rights to write into that folder.
add a comment |
I believe your code should work.
You could try to open the command prompt and type
cd c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSON
then
dir > test.txt
If you get an "Access is denied" message, it's because you really don't have sufficient rights to write into that folder.
add a comment |
I believe your code should work.
You could try to open the command prompt and type
cd c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSON
then
dir > test.txt
If you get an "Access is denied" message, it's because you really don't have sufficient rights to write into that folder.
I believe your code should work.
You could try to open the command prompt and type
cd c:UsersLOrdBenchesourcereposparsingTestparsingTestproducedJSON
then
dir > test.txt
If you get an "Access is denied" message, it's because you really don't have sufficient rights to write into that folder.
answered Nov 20 at 22:13
slig_3
13
13
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402029%2funauthorizedaccessexception-trying-to-serialize-json-to-a-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yhXGTM Lo7j4y2bobjnY
Please update your question with the full exception
– riQQ
Nov 20 at 22:03