Measure bandwidth used by web socket in React Native app
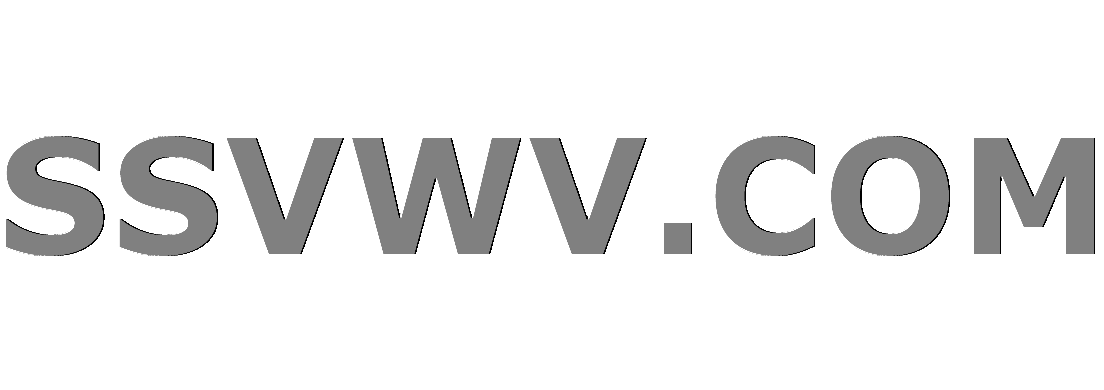
Multi tool use
In our application, staff use their phones to log activities within a business. They end up using 0.5GB-2GB data per month on average.
I'm trying to build functionality into our app that logs data usage so that we can send it back to the business in the form of an expense claim.
In the example code below, how can I determine how much bandwidth/data was used by the device sending the message over a WebSocket
?
var ws = new WebSocket('ws://host.com/path');
ws.onopen = () => {
ws.send('something');
};
javascript react-native networking websocket
add a comment |
In our application, staff use their phones to log activities within a business. They end up using 0.5GB-2GB data per month on average.
I'm trying to build functionality into our app that logs data usage so that we can send it back to the business in the form of an expense claim.
In the example code below, how can I determine how much bandwidth/data was used by the device sending the message over a WebSocket
?
var ws = new WebSocket('ws://host.com/path');
ws.onopen = () => {
ws.send('something');
};
javascript react-native networking websocket
add a comment |
In our application, staff use their phones to log activities within a business. They end up using 0.5GB-2GB data per month on average.
I'm trying to build functionality into our app that logs data usage so that we can send it back to the business in the form of an expense claim.
In the example code below, how can I determine how much bandwidth/data was used by the device sending the message over a WebSocket
?
var ws = new WebSocket('ws://host.com/path');
ws.onopen = () => {
ws.send('something');
};
javascript react-native networking websocket
In our application, staff use their phones to log activities within a business. They end up using 0.5GB-2GB data per month on average.
I'm trying to build functionality into our app that logs data usage so that we can send it back to the business in the form of an expense claim.
In the example code below, how can I determine how much bandwidth/data was used by the device sending the message over a WebSocket
?
var ws = new WebSocket('ws://host.com/path');
ws.onopen = () => {
ws.send('something');
};
javascript react-native networking websocket
javascript react-native networking websocket
asked Nov 22 '18 at 17:23
jskidd3jskidd3
1,84793787
1,84793787
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Assuming that you can identify a client session by unique IP (just the session, they do not always need this IP), I would recommend leveraging lower level tools that are more suited for your application, specifically NetFlow collectors.
NetFlow measures TCP 'conversation', via recording IP src, dst and throughput over a time slice. You can enable this in a Linux kernel or directly in some networking equipment. You will then need a program to collect and store the data.
Assuming you have NetFlow collection enabled and can identify sessions by IP, you can do the following:
- Record the time, user id and their IP address at the beginning of a session
- Using this data you can then query your NetFlow logs and get the throughput
The reason I suggest this instead of some sort of userspace solution that might count bytes received (which you could probably do fairly easily) is because there is a lot of data being abstracted by libraries and the kernel. The kernel handles the TCP stack (including re-sending missed packets), libraries handle the TLS handshakes/encryption and also the WebSocket Handshake. All this data is counted toward the user's used data. How users use the app will effect how much of this overhead data is sent (constantly opening/closing it vs leaving it open).
Many thanks for your answer Liam. Fully makes sense about monitoring this data on the server and matching up with IP from client. My only question would be, we're using Node JS on Google Cloud so don't think we are able to use NetFlow as you suggested. I'm trying to search as I type for a similar solution, perhaps you could steer me in the right path?
– jskidd3
Nov 27 '18 at 19:39
The Google Cloud version of NetFlow is called VPC Flow and provides the same general idea. cloud.google.com/vpc/docs/using-flow-logs
– Liam Kelly
Nov 27 '18 at 19:45
add a comment |
Depends on what precision you need. Simplest way would be to "subclass" existing sockets by something like this:
var inboundTraffic = 0;
var outboundTraffic = 0;
function NewWebSocket(addr) {
var ws = new WebSocket(addr);
var wsSend = ws.send;
ws.send = function(data) {
outboundTraffic += data.length;
return wsSend.call(ws,data);
}
ws.addEventListener("message", function (event) {
inboundTraffic += event.data.length;
});
return ws;
}
Simple and costs pretty much nothing.
Doesn't this just measure the size of the data sent, though? What about the size of the actual request/handshakes etc? I prefer this answer because of its simplicity, but I'm worried about the accuracy. We need to be at least 95% accurate, e.g. if 2GB is transferred 1.9GB needs to be logged minimum. Is it possible 5% could be lost without recording the actual request size? I'll perform some in-depth testing this afternoon!
– jskidd3
Dec 3 '18 at 12:33
Sending 200 bytes of data through an iOS app via a web socket uses 8000 bytes of mobile data. The overhead is very much what needs measuring as it makes up roughly 97.5% of the data usage.
– jskidd3
Dec 3 '18 at 14:11
@jskidd3 You are wrong about 200/8000. Yet is is not clear what that magic "mobile data" means. Anyway I've implemented native WebSockets in my Sciter Engine ( sciter.com ). Yes, there is some header data in each message but it is minimal. All that overhead is defined precisely in WS specification.
– c-smile
Dec 3 '18 at 16:14
Here is relevant part of WebSocket specification tools.ietf.org/html/rfc6455#section-5.6 - as you see overhead is minimal and can be calculated exactly.
– c-smile
Dec 3 '18 at 16:18
Thanks very much for that. That's odd why iOS is reporting such large usage... I'll try and dig into why that might be. Do you think you could use those calculations to modify your answer to include the overhead? If you do that I'll select as best answer as this is then far superior answer.
– jskidd3
Dec 3 '18 at 16:27
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435793%2fmeasure-bandwidth-used-by-web-socket-in-react-native-app%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Assuming that you can identify a client session by unique IP (just the session, they do not always need this IP), I would recommend leveraging lower level tools that are more suited for your application, specifically NetFlow collectors.
NetFlow measures TCP 'conversation', via recording IP src, dst and throughput over a time slice. You can enable this in a Linux kernel or directly in some networking equipment. You will then need a program to collect and store the data.
Assuming you have NetFlow collection enabled and can identify sessions by IP, you can do the following:
- Record the time, user id and their IP address at the beginning of a session
- Using this data you can then query your NetFlow logs and get the throughput
The reason I suggest this instead of some sort of userspace solution that might count bytes received (which you could probably do fairly easily) is because there is a lot of data being abstracted by libraries and the kernel. The kernel handles the TCP stack (including re-sending missed packets), libraries handle the TLS handshakes/encryption and also the WebSocket Handshake. All this data is counted toward the user's used data. How users use the app will effect how much of this overhead data is sent (constantly opening/closing it vs leaving it open).
Many thanks for your answer Liam. Fully makes sense about monitoring this data on the server and matching up with IP from client. My only question would be, we're using Node JS on Google Cloud so don't think we are able to use NetFlow as you suggested. I'm trying to search as I type for a similar solution, perhaps you could steer me in the right path?
– jskidd3
Nov 27 '18 at 19:39
The Google Cloud version of NetFlow is called VPC Flow and provides the same general idea. cloud.google.com/vpc/docs/using-flow-logs
– Liam Kelly
Nov 27 '18 at 19:45
add a comment |
Assuming that you can identify a client session by unique IP (just the session, they do not always need this IP), I would recommend leveraging lower level tools that are more suited for your application, specifically NetFlow collectors.
NetFlow measures TCP 'conversation', via recording IP src, dst and throughput over a time slice. You can enable this in a Linux kernel or directly in some networking equipment. You will then need a program to collect and store the data.
Assuming you have NetFlow collection enabled and can identify sessions by IP, you can do the following:
- Record the time, user id and their IP address at the beginning of a session
- Using this data you can then query your NetFlow logs and get the throughput
The reason I suggest this instead of some sort of userspace solution that might count bytes received (which you could probably do fairly easily) is because there is a lot of data being abstracted by libraries and the kernel. The kernel handles the TCP stack (including re-sending missed packets), libraries handle the TLS handshakes/encryption and also the WebSocket Handshake. All this data is counted toward the user's used data. How users use the app will effect how much of this overhead data is sent (constantly opening/closing it vs leaving it open).
Many thanks for your answer Liam. Fully makes sense about monitoring this data on the server and matching up with IP from client. My only question would be, we're using Node JS on Google Cloud so don't think we are able to use NetFlow as you suggested. I'm trying to search as I type for a similar solution, perhaps you could steer me in the right path?
– jskidd3
Nov 27 '18 at 19:39
The Google Cloud version of NetFlow is called VPC Flow and provides the same general idea. cloud.google.com/vpc/docs/using-flow-logs
– Liam Kelly
Nov 27 '18 at 19:45
add a comment |
Assuming that you can identify a client session by unique IP (just the session, they do not always need this IP), I would recommend leveraging lower level tools that are more suited for your application, specifically NetFlow collectors.
NetFlow measures TCP 'conversation', via recording IP src, dst and throughput over a time slice. You can enable this in a Linux kernel or directly in some networking equipment. You will then need a program to collect and store the data.
Assuming you have NetFlow collection enabled and can identify sessions by IP, you can do the following:
- Record the time, user id and their IP address at the beginning of a session
- Using this data you can then query your NetFlow logs and get the throughput
The reason I suggest this instead of some sort of userspace solution that might count bytes received (which you could probably do fairly easily) is because there is a lot of data being abstracted by libraries and the kernel. The kernel handles the TCP stack (including re-sending missed packets), libraries handle the TLS handshakes/encryption and also the WebSocket Handshake. All this data is counted toward the user's used data. How users use the app will effect how much of this overhead data is sent (constantly opening/closing it vs leaving it open).
Assuming that you can identify a client session by unique IP (just the session, they do not always need this IP), I would recommend leveraging lower level tools that are more suited for your application, specifically NetFlow collectors.
NetFlow measures TCP 'conversation', via recording IP src, dst and throughput over a time slice. You can enable this in a Linux kernel or directly in some networking equipment. You will then need a program to collect and store the data.
Assuming you have NetFlow collection enabled and can identify sessions by IP, you can do the following:
- Record the time, user id and their IP address at the beginning of a session
- Using this data you can then query your NetFlow logs and get the throughput
The reason I suggest this instead of some sort of userspace solution that might count bytes received (which you could probably do fairly easily) is because there is a lot of data being abstracted by libraries and the kernel. The kernel handles the TCP stack (including re-sending missed packets), libraries handle the TLS handshakes/encryption and also the WebSocket Handshake. All this data is counted toward the user's used data. How users use the app will effect how much of this overhead data is sent (constantly opening/closing it vs leaving it open).
answered Nov 26 '18 at 18:11
Liam KellyLiam Kelly
1,513916
1,513916
Many thanks for your answer Liam. Fully makes sense about monitoring this data on the server and matching up with IP from client. My only question would be, we're using Node JS on Google Cloud so don't think we are able to use NetFlow as you suggested. I'm trying to search as I type for a similar solution, perhaps you could steer me in the right path?
– jskidd3
Nov 27 '18 at 19:39
The Google Cloud version of NetFlow is called VPC Flow and provides the same general idea. cloud.google.com/vpc/docs/using-flow-logs
– Liam Kelly
Nov 27 '18 at 19:45
add a comment |
Many thanks for your answer Liam. Fully makes sense about monitoring this data on the server and matching up with IP from client. My only question would be, we're using Node JS on Google Cloud so don't think we are able to use NetFlow as you suggested. I'm trying to search as I type for a similar solution, perhaps you could steer me in the right path?
– jskidd3
Nov 27 '18 at 19:39
The Google Cloud version of NetFlow is called VPC Flow and provides the same general idea. cloud.google.com/vpc/docs/using-flow-logs
– Liam Kelly
Nov 27 '18 at 19:45
Many thanks for your answer Liam. Fully makes sense about monitoring this data on the server and matching up with IP from client. My only question would be, we're using Node JS on Google Cloud so don't think we are able to use NetFlow as you suggested. I'm trying to search as I type for a similar solution, perhaps you could steer me in the right path?
– jskidd3
Nov 27 '18 at 19:39
Many thanks for your answer Liam. Fully makes sense about monitoring this data on the server and matching up with IP from client. My only question would be, we're using Node JS on Google Cloud so don't think we are able to use NetFlow as you suggested. I'm trying to search as I type for a similar solution, perhaps you could steer me in the right path?
– jskidd3
Nov 27 '18 at 19:39
The Google Cloud version of NetFlow is called VPC Flow and provides the same general idea. cloud.google.com/vpc/docs/using-flow-logs
– Liam Kelly
Nov 27 '18 at 19:45
The Google Cloud version of NetFlow is called VPC Flow and provides the same general idea. cloud.google.com/vpc/docs/using-flow-logs
– Liam Kelly
Nov 27 '18 at 19:45
add a comment |
Depends on what precision you need. Simplest way would be to "subclass" existing sockets by something like this:
var inboundTraffic = 0;
var outboundTraffic = 0;
function NewWebSocket(addr) {
var ws = new WebSocket(addr);
var wsSend = ws.send;
ws.send = function(data) {
outboundTraffic += data.length;
return wsSend.call(ws,data);
}
ws.addEventListener("message", function (event) {
inboundTraffic += event.data.length;
});
return ws;
}
Simple and costs pretty much nothing.
Doesn't this just measure the size of the data sent, though? What about the size of the actual request/handshakes etc? I prefer this answer because of its simplicity, but I'm worried about the accuracy. We need to be at least 95% accurate, e.g. if 2GB is transferred 1.9GB needs to be logged minimum. Is it possible 5% could be lost without recording the actual request size? I'll perform some in-depth testing this afternoon!
– jskidd3
Dec 3 '18 at 12:33
Sending 200 bytes of data through an iOS app via a web socket uses 8000 bytes of mobile data. The overhead is very much what needs measuring as it makes up roughly 97.5% of the data usage.
– jskidd3
Dec 3 '18 at 14:11
@jskidd3 You are wrong about 200/8000. Yet is is not clear what that magic "mobile data" means. Anyway I've implemented native WebSockets in my Sciter Engine ( sciter.com ). Yes, there is some header data in each message but it is minimal. All that overhead is defined precisely in WS specification.
– c-smile
Dec 3 '18 at 16:14
Here is relevant part of WebSocket specification tools.ietf.org/html/rfc6455#section-5.6 - as you see overhead is minimal and can be calculated exactly.
– c-smile
Dec 3 '18 at 16:18
Thanks very much for that. That's odd why iOS is reporting such large usage... I'll try and dig into why that might be. Do you think you could use those calculations to modify your answer to include the overhead? If you do that I'll select as best answer as this is then far superior answer.
– jskidd3
Dec 3 '18 at 16:27
add a comment |
Depends on what precision you need. Simplest way would be to "subclass" existing sockets by something like this:
var inboundTraffic = 0;
var outboundTraffic = 0;
function NewWebSocket(addr) {
var ws = new WebSocket(addr);
var wsSend = ws.send;
ws.send = function(data) {
outboundTraffic += data.length;
return wsSend.call(ws,data);
}
ws.addEventListener("message", function (event) {
inboundTraffic += event.data.length;
});
return ws;
}
Simple and costs pretty much nothing.
Doesn't this just measure the size of the data sent, though? What about the size of the actual request/handshakes etc? I prefer this answer because of its simplicity, but I'm worried about the accuracy. We need to be at least 95% accurate, e.g. if 2GB is transferred 1.9GB needs to be logged minimum. Is it possible 5% could be lost without recording the actual request size? I'll perform some in-depth testing this afternoon!
– jskidd3
Dec 3 '18 at 12:33
Sending 200 bytes of data through an iOS app via a web socket uses 8000 bytes of mobile data. The overhead is very much what needs measuring as it makes up roughly 97.5% of the data usage.
– jskidd3
Dec 3 '18 at 14:11
@jskidd3 You are wrong about 200/8000. Yet is is not clear what that magic "mobile data" means. Anyway I've implemented native WebSockets in my Sciter Engine ( sciter.com ). Yes, there is some header data in each message but it is minimal. All that overhead is defined precisely in WS specification.
– c-smile
Dec 3 '18 at 16:14
Here is relevant part of WebSocket specification tools.ietf.org/html/rfc6455#section-5.6 - as you see overhead is minimal and can be calculated exactly.
– c-smile
Dec 3 '18 at 16:18
Thanks very much for that. That's odd why iOS is reporting such large usage... I'll try and dig into why that might be. Do you think you could use those calculations to modify your answer to include the overhead? If you do that I'll select as best answer as this is then far superior answer.
– jskidd3
Dec 3 '18 at 16:27
add a comment |
Depends on what precision you need. Simplest way would be to "subclass" existing sockets by something like this:
var inboundTraffic = 0;
var outboundTraffic = 0;
function NewWebSocket(addr) {
var ws = new WebSocket(addr);
var wsSend = ws.send;
ws.send = function(data) {
outboundTraffic += data.length;
return wsSend.call(ws,data);
}
ws.addEventListener("message", function (event) {
inboundTraffic += event.data.length;
});
return ws;
}
Simple and costs pretty much nothing.
Depends on what precision you need. Simplest way would be to "subclass" existing sockets by something like this:
var inboundTraffic = 0;
var outboundTraffic = 0;
function NewWebSocket(addr) {
var ws = new WebSocket(addr);
var wsSend = ws.send;
ws.send = function(data) {
outboundTraffic += data.length;
return wsSend.call(ws,data);
}
ws.addEventListener("message", function (event) {
inboundTraffic += event.data.length;
});
return ws;
}
Simple and costs pretty much nothing.
answered Dec 1 '18 at 21:29


c-smilec-smile
20.5k44366
20.5k44366
Doesn't this just measure the size of the data sent, though? What about the size of the actual request/handshakes etc? I prefer this answer because of its simplicity, but I'm worried about the accuracy. We need to be at least 95% accurate, e.g. if 2GB is transferred 1.9GB needs to be logged minimum. Is it possible 5% could be lost without recording the actual request size? I'll perform some in-depth testing this afternoon!
– jskidd3
Dec 3 '18 at 12:33
Sending 200 bytes of data through an iOS app via a web socket uses 8000 bytes of mobile data. The overhead is very much what needs measuring as it makes up roughly 97.5% of the data usage.
– jskidd3
Dec 3 '18 at 14:11
@jskidd3 You are wrong about 200/8000. Yet is is not clear what that magic "mobile data" means. Anyway I've implemented native WebSockets in my Sciter Engine ( sciter.com ). Yes, there is some header data in each message but it is minimal. All that overhead is defined precisely in WS specification.
– c-smile
Dec 3 '18 at 16:14
Here is relevant part of WebSocket specification tools.ietf.org/html/rfc6455#section-5.6 - as you see overhead is minimal and can be calculated exactly.
– c-smile
Dec 3 '18 at 16:18
Thanks very much for that. That's odd why iOS is reporting such large usage... I'll try and dig into why that might be. Do you think you could use those calculations to modify your answer to include the overhead? If you do that I'll select as best answer as this is then far superior answer.
– jskidd3
Dec 3 '18 at 16:27
add a comment |
Doesn't this just measure the size of the data sent, though? What about the size of the actual request/handshakes etc? I prefer this answer because of its simplicity, but I'm worried about the accuracy. We need to be at least 95% accurate, e.g. if 2GB is transferred 1.9GB needs to be logged minimum. Is it possible 5% could be lost without recording the actual request size? I'll perform some in-depth testing this afternoon!
– jskidd3
Dec 3 '18 at 12:33
Sending 200 bytes of data through an iOS app via a web socket uses 8000 bytes of mobile data. The overhead is very much what needs measuring as it makes up roughly 97.5% of the data usage.
– jskidd3
Dec 3 '18 at 14:11
@jskidd3 You are wrong about 200/8000. Yet is is not clear what that magic "mobile data" means. Anyway I've implemented native WebSockets in my Sciter Engine ( sciter.com ). Yes, there is some header data in each message but it is minimal. All that overhead is defined precisely in WS specification.
– c-smile
Dec 3 '18 at 16:14
Here is relevant part of WebSocket specification tools.ietf.org/html/rfc6455#section-5.6 - as you see overhead is minimal and can be calculated exactly.
– c-smile
Dec 3 '18 at 16:18
Thanks very much for that. That's odd why iOS is reporting such large usage... I'll try and dig into why that might be. Do you think you could use those calculations to modify your answer to include the overhead? If you do that I'll select as best answer as this is then far superior answer.
– jskidd3
Dec 3 '18 at 16:27
Doesn't this just measure the size of the data sent, though? What about the size of the actual request/handshakes etc? I prefer this answer because of its simplicity, but I'm worried about the accuracy. We need to be at least 95% accurate, e.g. if 2GB is transferred 1.9GB needs to be logged minimum. Is it possible 5% could be lost without recording the actual request size? I'll perform some in-depth testing this afternoon!
– jskidd3
Dec 3 '18 at 12:33
Doesn't this just measure the size of the data sent, though? What about the size of the actual request/handshakes etc? I prefer this answer because of its simplicity, but I'm worried about the accuracy. We need to be at least 95% accurate, e.g. if 2GB is transferred 1.9GB needs to be logged minimum. Is it possible 5% could be lost without recording the actual request size? I'll perform some in-depth testing this afternoon!
– jskidd3
Dec 3 '18 at 12:33
Sending 200 bytes of data through an iOS app via a web socket uses 8000 bytes of mobile data. The overhead is very much what needs measuring as it makes up roughly 97.5% of the data usage.
– jskidd3
Dec 3 '18 at 14:11
Sending 200 bytes of data through an iOS app via a web socket uses 8000 bytes of mobile data. The overhead is very much what needs measuring as it makes up roughly 97.5% of the data usage.
– jskidd3
Dec 3 '18 at 14:11
@jskidd3 You are wrong about 200/8000. Yet is is not clear what that magic "mobile data" means. Anyway I've implemented native WebSockets in my Sciter Engine ( sciter.com ). Yes, there is some header data in each message but it is minimal. All that overhead is defined precisely in WS specification.
– c-smile
Dec 3 '18 at 16:14
@jskidd3 You are wrong about 200/8000. Yet is is not clear what that magic "mobile data" means. Anyway I've implemented native WebSockets in my Sciter Engine ( sciter.com ). Yes, there is some header data in each message but it is minimal. All that overhead is defined precisely in WS specification.
– c-smile
Dec 3 '18 at 16:14
Here is relevant part of WebSocket specification tools.ietf.org/html/rfc6455#section-5.6 - as you see overhead is minimal and can be calculated exactly.
– c-smile
Dec 3 '18 at 16:18
Here is relevant part of WebSocket specification tools.ietf.org/html/rfc6455#section-5.6 - as you see overhead is minimal and can be calculated exactly.
– c-smile
Dec 3 '18 at 16:18
Thanks very much for that. That's odd why iOS is reporting such large usage... I'll try and dig into why that might be. Do you think you could use those calculations to modify your answer to include the overhead? If you do that I'll select as best answer as this is then far superior answer.
– jskidd3
Dec 3 '18 at 16:27
Thanks very much for that. That's odd why iOS is reporting such large usage... I'll try and dig into why that might be. Do you think you could use those calculations to modify your answer to include the overhead? If you do that I'll select as best answer as this is then far superior answer.
– jskidd3
Dec 3 '18 at 16:27
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435793%2fmeasure-bandwidth-used-by-web-socket-in-react-native-app%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
X3O3BKPydusQa0cE,BuGkK0kVoERv,8E7IKvY,nGwk8CmipY,r,okL0