problems with the values of the promise Promise { value }
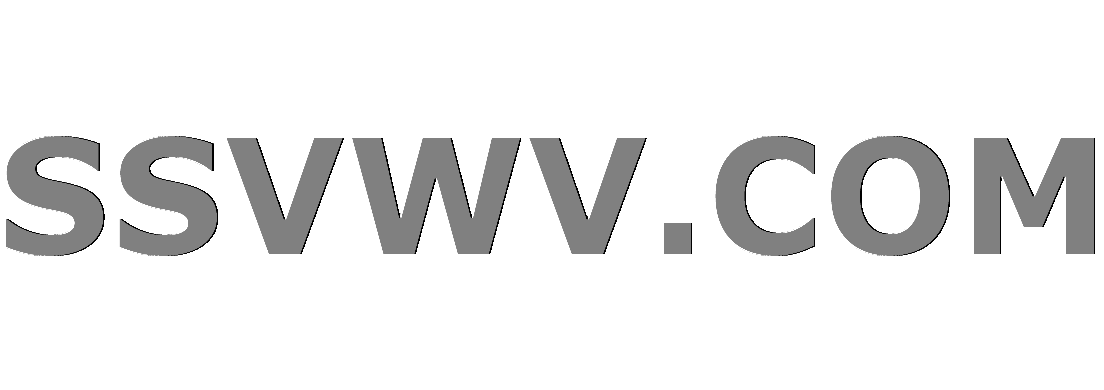
Multi tool use
I'm exporting a function where it returns the value of a query in mysql, when I do the path of the Json and the values that I assign from the function appear as Promise {value} (example Promise {61}), and I do not know how to fix the value of the promise.
query.js
const mysql = require('mysql2/promise');
const vertodo = async function(req){
const connection = await mysql.createConnection({
host: '192.168.0.222',
port: 3306,
user: 'root',
database: 'project',
password : '123456789'
});
return [rows, fields] = await connection.query('Select COUNT(*) total from table1 where id=' +req.id);
connection.end();
}
module.exports.vertodo = vertodo;
index.js
const data_mysql = require("./query")
// value jsonObj
// [{ id: 1,
// name: 'XTRA',
// proceso: 0
// },
// { id: 2,
// name: 'Maq',
// proceso: 0
// }]
for (var i = 0; i < jsonObj.length; i++) {
//jsonObj[i].proceso = 0;
jsonObj[i].procesa = data_mysql.vertodo(jsonObj[i]).then( rows =>{
return rows[0][0].total
})
// jsonObj[i].proceso = Promise { 61 } , Promise { 33 };
}
setTimeout(function(){
for (var i = 0; i < jsonObj.length; i++) {
console.log(jsonObj[i].procesa); // Promise { 61}, Promise { 33 }
}
}, 3000);
I do not know how I can solve it, thanks
javascript node.js promise mysql2
add a comment |
I'm exporting a function where it returns the value of a query in mysql, when I do the path of the Json and the values that I assign from the function appear as Promise {value} (example Promise {61}), and I do not know how to fix the value of the promise.
query.js
const mysql = require('mysql2/promise');
const vertodo = async function(req){
const connection = await mysql.createConnection({
host: '192.168.0.222',
port: 3306,
user: 'root',
database: 'project',
password : '123456789'
});
return [rows, fields] = await connection.query('Select COUNT(*) total from table1 where id=' +req.id);
connection.end();
}
module.exports.vertodo = vertodo;
index.js
const data_mysql = require("./query")
// value jsonObj
// [{ id: 1,
// name: 'XTRA',
// proceso: 0
// },
// { id: 2,
// name: 'Maq',
// proceso: 0
// }]
for (var i = 0; i < jsonObj.length; i++) {
//jsonObj[i].proceso = 0;
jsonObj[i].procesa = data_mysql.vertodo(jsonObj[i]).then( rows =>{
return rows[0][0].total
})
// jsonObj[i].proceso = Promise { 61 } , Promise { 33 };
}
setTimeout(function(){
for (var i = 0; i < jsonObj.length; i++) {
console.log(jsonObj[i].procesa); // Promise { 61}, Promise { 33 }
}
}, 3000);
I do not know how I can solve it, thanks
javascript node.js promise mysql2
add a comment |
I'm exporting a function where it returns the value of a query in mysql, when I do the path of the Json and the values that I assign from the function appear as Promise {value} (example Promise {61}), and I do not know how to fix the value of the promise.
query.js
const mysql = require('mysql2/promise');
const vertodo = async function(req){
const connection = await mysql.createConnection({
host: '192.168.0.222',
port: 3306,
user: 'root',
database: 'project',
password : '123456789'
});
return [rows, fields] = await connection.query('Select COUNT(*) total from table1 where id=' +req.id);
connection.end();
}
module.exports.vertodo = vertodo;
index.js
const data_mysql = require("./query")
// value jsonObj
// [{ id: 1,
// name: 'XTRA',
// proceso: 0
// },
// { id: 2,
// name: 'Maq',
// proceso: 0
// }]
for (var i = 0; i < jsonObj.length; i++) {
//jsonObj[i].proceso = 0;
jsonObj[i].procesa = data_mysql.vertodo(jsonObj[i]).then( rows =>{
return rows[0][0].total
})
// jsonObj[i].proceso = Promise { 61 } , Promise { 33 };
}
setTimeout(function(){
for (var i = 0; i < jsonObj.length; i++) {
console.log(jsonObj[i].procesa); // Promise { 61}, Promise { 33 }
}
}, 3000);
I do not know how I can solve it, thanks
javascript node.js promise mysql2
I'm exporting a function where it returns the value of a query in mysql, when I do the path of the Json and the values that I assign from the function appear as Promise {value} (example Promise {61}), and I do not know how to fix the value of the promise.
query.js
const mysql = require('mysql2/promise');
const vertodo = async function(req){
const connection = await mysql.createConnection({
host: '192.168.0.222',
port: 3306,
user: 'root',
database: 'project',
password : '123456789'
});
return [rows, fields] = await connection.query('Select COUNT(*) total from table1 where id=' +req.id);
connection.end();
}
module.exports.vertodo = vertodo;
index.js
const data_mysql = require("./query")
// value jsonObj
// [{ id: 1,
// name: 'XTRA',
// proceso: 0
// },
// { id: 2,
// name: 'Maq',
// proceso: 0
// }]
for (var i = 0; i < jsonObj.length; i++) {
//jsonObj[i].proceso = 0;
jsonObj[i].procesa = data_mysql.vertodo(jsonObj[i]).then( rows =>{
return rows[0][0].total
})
// jsonObj[i].proceso = Promise { 61 } , Promise { 33 };
}
setTimeout(function(){
for (var i = 0; i < jsonObj.length; i++) {
console.log(jsonObj[i].procesa); // Promise { 61}, Promise { 33 }
}
}, 3000);
I do not know how I can solve it, thanks
javascript node.js promise mysql2
javascript node.js promise mysql2
asked Nov 22 '18 at 17:21
user10597291
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Your function "vertodo" returns a promise. You need to wait for the promise to resolve with the query response data. The easiest way to do this is to use the async/await syntax. See below.
index.js
const data_mysql = require("./query")
runQuery()
async function runQuery() {
for (var i = 0; i < jsonObj.length; i++) {
//jsonObj[i].proceso = 0;
queryResponse = await data_mysql.vertodo(jsonObj[i])
// process queryResponse
}
}
add a comment |
I don't fully understand what you want to do, but I assume that you want to run those queries in loop and wait until all finish and then log results.
If those queries need to be run in sequence.
async function runQueries() {
for (const item of jsonObj) {
item.procesa = await data_mysql.vertodo(item);
}
}
runQueries.then(function () {
jsonObj.forEach(function (item) {
console.log(item.procesa);
});
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435780%2fproblems-with-the-values-of-the-promise-promise-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your function "vertodo" returns a promise. You need to wait for the promise to resolve with the query response data. The easiest way to do this is to use the async/await syntax. See below.
index.js
const data_mysql = require("./query")
runQuery()
async function runQuery() {
for (var i = 0; i < jsonObj.length; i++) {
//jsonObj[i].proceso = 0;
queryResponse = await data_mysql.vertodo(jsonObj[i])
// process queryResponse
}
}
add a comment |
Your function "vertodo" returns a promise. You need to wait for the promise to resolve with the query response data. The easiest way to do this is to use the async/await syntax. See below.
index.js
const data_mysql = require("./query")
runQuery()
async function runQuery() {
for (var i = 0; i < jsonObj.length; i++) {
//jsonObj[i].proceso = 0;
queryResponse = await data_mysql.vertodo(jsonObj[i])
// process queryResponse
}
}
add a comment |
Your function "vertodo" returns a promise. You need to wait for the promise to resolve with the query response data. The easiest way to do this is to use the async/await syntax. See below.
index.js
const data_mysql = require("./query")
runQuery()
async function runQuery() {
for (var i = 0; i < jsonObj.length; i++) {
//jsonObj[i].proceso = 0;
queryResponse = await data_mysql.vertodo(jsonObj[i])
// process queryResponse
}
}
Your function "vertodo" returns a promise. You need to wait for the promise to resolve with the query response data. The easiest way to do this is to use the async/await syntax. See below.
index.js
const data_mysql = require("./query")
runQuery()
async function runQuery() {
for (var i = 0; i < jsonObj.length; i++) {
//jsonObj[i].proceso = 0;
queryResponse = await data_mysql.vertodo(jsonObj[i])
// process queryResponse
}
}
answered Nov 22 '18 at 18:08
FakeFootballFakeFootball
11
11
add a comment |
add a comment |
I don't fully understand what you want to do, but I assume that you want to run those queries in loop and wait until all finish and then log results.
If those queries need to be run in sequence.
async function runQueries() {
for (const item of jsonObj) {
item.procesa = await data_mysql.vertodo(item);
}
}
runQueries.then(function () {
jsonObj.forEach(function (item) {
console.log(item.procesa);
});
});
add a comment |
I don't fully understand what you want to do, but I assume that you want to run those queries in loop and wait until all finish and then log results.
If those queries need to be run in sequence.
async function runQueries() {
for (const item of jsonObj) {
item.procesa = await data_mysql.vertodo(item);
}
}
runQueries.then(function () {
jsonObj.forEach(function (item) {
console.log(item.procesa);
});
});
add a comment |
I don't fully understand what you want to do, but I assume that you want to run those queries in loop and wait until all finish and then log results.
If those queries need to be run in sequence.
async function runQueries() {
for (const item of jsonObj) {
item.procesa = await data_mysql.vertodo(item);
}
}
runQueries.then(function () {
jsonObj.forEach(function (item) {
console.log(item.procesa);
});
});
I don't fully understand what you want to do, but I assume that you want to run those queries in loop and wait until all finish and then log results.
If those queries need to be run in sequence.
async function runQueries() {
for (const item of jsonObj) {
item.procesa = await data_mysql.vertodo(item);
}
}
runQueries.then(function () {
jsonObj.forEach(function (item) {
console.log(item.procesa);
});
});
answered Nov 22 '18 at 23:02


Jake NohJake Noh
615
615
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435780%2fproblems-with-the-values-of-the-promise-promise-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fehScS2 wB rvaXHVhICIvFVmvPKNV,5J4Aq