Finding different char from 2 given strings
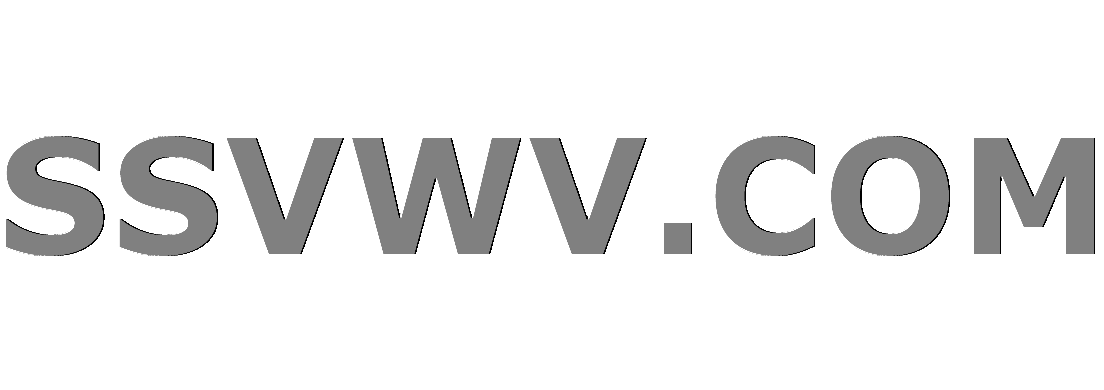
Multi tool use
I believe I have the code right for this particular question already but I do have some follow up questions. I'm still fairly new to this and I got it to spit out what I was looking for. If there are room for improvements I am open to criticisms. I'm just looking to get better.
How would I approach this differently or how would I solve it if the 2 given Strings are VERY large and the memory is limited?
import java.util.ArrayList;
public class DifferChar {
public char diffChar(String str1, String str2)
{
ArrayList<Character> al1 = new ArrayList<Character>();
ArrayList<Character> al2 = new ArrayList<Character>();
String longer;
String shorter;
char c = 'u0000';
if (str1 != null && str2 != null)
{
if (str1.length() > str2.length())
{
longer = str1.toUpperCase();
shorter = str2.toUpperCase();
}
else
{
longer = str2.toUpperCase();
shorter = str1.toUpperCase();
}
if (longer.length() - shorter.length() <= 1)
{
for (char ch : shorter.toCharArray())
al1.add(ch);
for (char ch : longer.toCharArray())
al2.add(ch);
for (int i = al1.size()-1; i >= 0; i--)
{
if (al2.contains(al1.get(i)))
al2.remove(al1.get(i));
}
if (al2.size() == 1)
c = al2.get(0);
}
}
return c;
}
public static void main(String args)
{
String str1 = "aklwejr";
String str2 = "aklwej";
DifferChar diff = new DifferChar();
System.out.println(diff.diffChar(str1, str2));
}
}
java
New contributor
noredlac is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I believe I have the code right for this particular question already but I do have some follow up questions. I'm still fairly new to this and I got it to spit out what I was looking for. If there are room for improvements I am open to criticisms. I'm just looking to get better.
How would I approach this differently or how would I solve it if the 2 given Strings are VERY large and the memory is limited?
import java.util.ArrayList;
public class DifferChar {
public char diffChar(String str1, String str2)
{
ArrayList<Character> al1 = new ArrayList<Character>();
ArrayList<Character> al2 = new ArrayList<Character>();
String longer;
String shorter;
char c = 'u0000';
if (str1 != null && str2 != null)
{
if (str1.length() > str2.length())
{
longer = str1.toUpperCase();
shorter = str2.toUpperCase();
}
else
{
longer = str2.toUpperCase();
shorter = str1.toUpperCase();
}
if (longer.length() - shorter.length() <= 1)
{
for (char ch : shorter.toCharArray())
al1.add(ch);
for (char ch : longer.toCharArray())
al2.add(ch);
for (int i = al1.size()-1; i >= 0; i--)
{
if (al2.contains(al1.get(i)))
al2.remove(al1.get(i));
}
if (al2.size() == 1)
c = al2.get(0);
}
}
return c;
}
public static void main(String args)
{
String str1 = "aklwejr";
String str2 = "aklwej";
DifferChar diff = new DifferChar();
System.out.println(diff.diffChar(str1, str2));
}
}
java
New contributor
noredlac is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Could you clarify exactly what the requirements of the task are?
– 200_success
17 mins ago
add a comment |
I believe I have the code right for this particular question already but I do have some follow up questions. I'm still fairly new to this and I got it to spit out what I was looking for. If there are room for improvements I am open to criticisms. I'm just looking to get better.
How would I approach this differently or how would I solve it if the 2 given Strings are VERY large and the memory is limited?
import java.util.ArrayList;
public class DifferChar {
public char diffChar(String str1, String str2)
{
ArrayList<Character> al1 = new ArrayList<Character>();
ArrayList<Character> al2 = new ArrayList<Character>();
String longer;
String shorter;
char c = 'u0000';
if (str1 != null && str2 != null)
{
if (str1.length() > str2.length())
{
longer = str1.toUpperCase();
shorter = str2.toUpperCase();
}
else
{
longer = str2.toUpperCase();
shorter = str1.toUpperCase();
}
if (longer.length() - shorter.length() <= 1)
{
for (char ch : shorter.toCharArray())
al1.add(ch);
for (char ch : longer.toCharArray())
al2.add(ch);
for (int i = al1.size()-1; i >= 0; i--)
{
if (al2.contains(al1.get(i)))
al2.remove(al1.get(i));
}
if (al2.size() == 1)
c = al2.get(0);
}
}
return c;
}
public static void main(String args)
{
String str1 = "aklwejr";
String str2 = "aklwej";
DifferChar diff = new DifferChar();
System.out.println(diff.diffChar(str1, str2));
}
}
java
New contributor
noredlac is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I believe I have the code right for this particular question already but I do have some follow up questions. I'm still fairly new to this and I got it to spit out what I was looking for. If there are room for improvements I am open to criticisms. I'm just looking to get better.
How would I approach this differently or how would I solve it if the 2 given Strings are VERY large and the memory is limited?
import java.util.ArrayList;
public class DifferChar {
public char diffChar(String str1, String str2)
{
ArrayList<Character> al1 = new ArrayList<Character>();
ArrayList<Character> al2 = new ArrayList<Character>();
String longer;
String shorter;
char c = 'u0000';
if (str1 != null && str2 != null)
{
if (str1.length() > str2.length())
{
longer = str1.toUpperCase();
shorter = str2.toUpperCase();
}
else
{
longer = str2.toUpperCase();
shorter = str1.toUpperCase();
}
if (longer.length() - shorter.length() <= 1)
{
for (char ch : shorter.toCharArray())
al1.add(ch);
for (char ch : longer.toCharArray())
al2.add(ch);
for (int i = al1.size()-1; i >= 0; i--)
{
if (al2.contains(al1.get(i)))
al2.remove(al1.get(i));
}
if (al2.size() == 1)
c = al2.get(0);
}
}
return c;
}
public static void main(String args)
{
String str1 = "aklwejr";
String str2 = "aklwej";
DifferChar diff = new DifferChar();
System.out.println(diff.diffChar(str1, str2));
}
}
java
java
New contributor
noredlac is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
noredlac is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 18 mins ago


Jamal♦
30.3k11116226
30.3k11116226
New contributor
noredlac is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 26 mins ago
noredlacnoredlac
1
1
New contributor
noredlac is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
noredlac is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
noredlac is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Could you clarify exactly what the requirements of the task are?
– 200_success
17 mins ago
add a comment |
1
Could you clarify exactly what the requirements of the task are?
– 200_success
17 mins ago
1
1
Could you clarify exactly what the requirements of the task are?
– 200_success
17 mins ago
Could you clarify exactly what the requirements of the task are?
– 200_success
17 mins ago
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
noredlac is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f211342%2ffinding-different-char-from-2-given-strings%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
noredlac is a new contributor. Be nice, and check out our Code of Conduct.
noredlac is a new contributor. Be nice, and check out our Code of Conduct.
noredlac is a new contributor. Be nice, and check out our Code of Conduct.
noredlac is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f211342%2ffinding-different-char-from-2-given-strings%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vkZl1613T 8w G,Esd,7SUmtjFYH0I28uf1k9Cd mS7KkWTD,Vjd,RamuvzzaRDzQcaarjCOrfgFJThOnhy8WnbTN3E
1
Could you clarify exactly what the requirements of the task are?
– 200_success
17 mins ago