Stuck with Unhandled exception at 0x00007FF74F27A526 : Stack Overflow
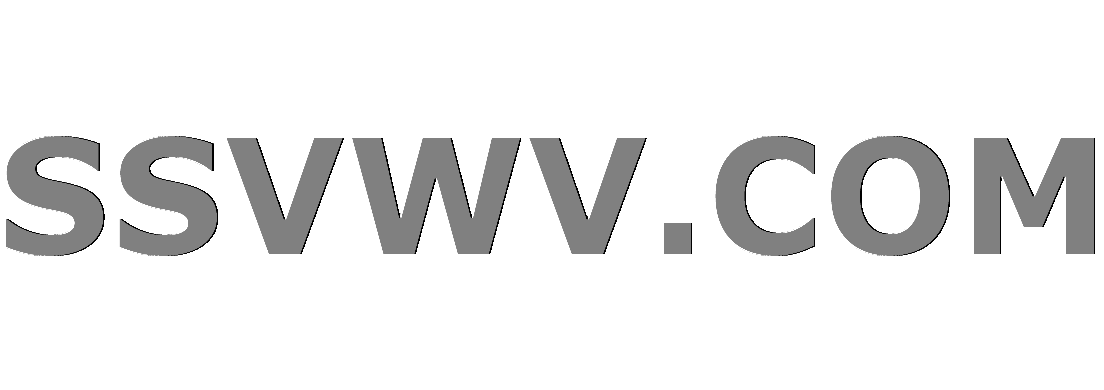
Multi tool use
I am having stack overflow related exception.
To be clear on that, I have no recursive function that can lead to blowing up stack. Although somewhere in my application I am creating a huge array with std::array and I am using class templates.
I thought of doing allocating memory dynamically but that is the last thing I want to do, also in template function which causing me the problem goes like this:
//class Template
template <class T, int Point, int End>
class Node;
private:
int Point;
int End;
std::array <T, Point*End>;
public:
// Rest of the stuff!
//Operator defined in class
//End of Node object1 is equal to Point of Node object2 always so;
template <int entry>
Node<T,Point,entry> operator* (const Node<T,End,entry> &source)
{
Node<T,Point,entry> temporary_node(0.);
//for_loop to multiply each element store in std::array
return temporary_node;
}
It is important to mention that my array size is somewhere around 10000000.
What is the solution if I don't want to use dynamic memory allocation:
Let's say if I do, then how can I allocate memory dynamically for temporary node in * operator defined above, as it takes up memory for vector when I do this:
Node temporary_node;
I am on Windows and I also don't want to increase stack size manually as going over 1MB manually will solve the problem but It will be risky and maybe buggy.
Thank you in advance.
Edit
I just checked it on Linux and its working perfectly fine over there. Maybe because I thought as stack size for linux is around 8MB considering Virtual also. But ugh! This will make the application less cross platform friendly if I don't consider making it work for windows stack default range.
c++ c++11 templates
add a comment |
I am having stack overflow related exception.
To be clear on that, I have no recursive function that can lead to blowing up stack. Although somewhere in my application I am creating a huge array with std::array and I am using class templates.
I thought of doing allocating memory dynamically but that is the last thing I want to do, also in template function which causing me the problem goes like this:
//class Template
template <class T, int Point, int End>
class Node;
private:
int Point;
int End;
std::array <T, Point*End>;
public:
// Rest of the stuff!
//Operator defined in class
//End of Node object1 is equal to Point of Node object2 always so;
template <int entry>
Node<T,Point,entry> operator* (const Node<T,End,entry> &source)
{
Node<T,Point,entry> temporary_node(0.);
//for_loop to multiply each element store in std::array
return temporary_node;
}
It is important to mention that my array size is somewhere around 10000000.
What is the solution if I don't want to use dynamic memory allocation:
Let's say if I do, then how can I allocate memory dynamically for temporary node in * operator defined above, as it takes up memory for vector when I do this:
Node temporary_node;
I am on Windows and I also don't want to increase stack size manually as going over 1MB manually will solve the problem but It will be risky and maybe buggy.
Thank you in advance.
Edit
I just checked it on Linux and its working perfectly fine over there. Maybe because I thought as stack size for linux is around 8MB considering Virtual also. But ugh! This will make the application less cross platform friendly if I don't consider making it work for windows stack default range.
c++ c++11 templates
2
If you're throwing a stack overflow exception, you're blowing the stack. End of. Ergo, don't blow the stack. (Someone with better template experience will have to tell you how you're blowing the stack.)
– Robert Harvey♦
Nov 21 at 2:10
Anything which I didn't mention? But thank you and don't say " Blowing up ", it hurts my feelings.
– Shamraiz
Nov 21 at 3:06
1
@Shamraiz //for_loop to multiply each element store in std::array -- This is a big reason why you should write your templated functions using iterators, not with concrete container types. If you had done that, the user would simply pass to you the start and end iterator, and it wouldn't matter if the container was astd::array
,std::vector
, or just a plain array.
– PaulMcKenzie
Nov 21 at 3:44
@PaulMcKenzie That sounds like decent and logical. I will give it a try
– Shamraiz
Nov 21 at 3:47
add a comment |
I am having stack overflow related exception.
To be clear on that, I have no recursive function that can lead to blowing up stack. Although somewhere in my application I am creating a huge array with std::array and I am using class templates.
I thought of doing allocating memory dynamically but that is the last thing I want to do, also in template function which causing me the problem goes like this:
//class Template
template <class T, int Point, int End>
class Node;
private:
int Point;
int End;
std::array <T, Point*End>;
public:
// Rest of the stuff!
//Operator defined in class
//End of Node object1 is equal to Point of Node object2 always so;
template <int entry>
Node<T,Point,entry> operator* (const Node<T,End,entry> &source)
{
Node<T,Point,entry> temporary_node(0.);
//for_loop to multiply each element store in std::array
return temporary_node;
}
It is important to mention that my array size is somewhere around 10000000.
What is the solution if I don't want to use dynamic memory allocation:
Let's say if I do, then how can I allocate memory dynamically for temporary node in * operator defined above, as it takes up memory for vector when I do this:
Node temporary_node;
I am on Windows and I also don't want to increase stack size manually as going over 1MB manually will solve the problem but It will be risky and maybe buggy.
Thank you in advance.
Edit
I just checked it on Linux and its working perfectly fine over there. Maybe because I thought as stack size for linux is around 8MB considering Virtual also. But ugh! This will make the application less cross platform friendly if I don't consider making it work for windows stack default range.
c++ c++11 templates
I am having stack overflow related exception.
To be clear on that, I have no recursive function that can lead to blowing up stack. Although somewhere in my application I am creating a huge array with std::array and I am using class templates.
I thought of doing allocating memory dynamically but that is the last thing I want to do, also in template function which causing me the problem goes like this:
//class Template
template <class T, int Point, int End>
class Node;
private:
int Point;
int End;
std::array <T, Point*End>;
public:
// Rest of the stuff!
//Operator defined in class
//End of Node object1 is equal to Point of Node object2 always so;
template <int entry>
Node<T,Point,entry> operator* (const Node<T,End,entry> &source)
{
Node<T,Point,entry> temporary_node(0.);
//for_loop to multiply each element store in std::array
return temporary_node;
}
It is important to mention that my array size is somewhere around 10000000.
What is the solution if I don't want to use dynamic memory allocation:
Let's say if I do, then how can I allocate memory dynamically for temporary node in * operator defined above, as it takes up memory for vector when I do this:
Node temporary_node;
I am on Windows and I also don't want to increase stack size manually as going over 1MB manually will solve the problem but It will be risky and maybe buggy.
Thank you in advance.
Edit
I just checked it on Linux and its working perfectly fine over there. Maybe because I thought as stack size for linux is around 8MB considering Virtual also. But ugh! This will make the application less cross platform friendly if I don't consider making it work for windows stack default range.
c++ c++11 templates
c++ c++11 templates
edited Nov 21 at 3:26
asked Nov 21 at 2:09
Shamraiz
236
236
2
If you're throwing a stack overflow exception, you're blowing the stack. End of. Ergo, don't blow the stack. (Someone with better template experience will have to tell you how you're blowing the stack.)
– Robert Harvey♦
Nov 21 at 2:10
Anything which I didn't mention? But thank you and don't say " Blowing up ", it hurts my feelings.
– Shamraiz
Nov 21 at 3:06
1
@Shamraiz //for_loop to multiply each element store in std::array -- This is a big reason why you should write your templated functions using iterators, not with concrete container types. If you had done that, the user would simply pass to you the start and end iterator, and it wouldn't matter if the container was astd::array
,std::vector
, or just a plain array.
– PaulMcKenzie
Nov 21 at 3:44
@PaulMcKenzie That sounds like decent and logical. I will give it a try
– Shamraiz
Nov 21 at 3:47
add a comment |
2
If you're throwing a stack overflow exception, you're blowing the stack. End of. Ergo, don't blow the stack. (Someone with better template experience will have to tell you how you're blowing the stack.)
– Robert Harvey♦
Nov 21 at 2:10
Anything which I didn't mention? But thank you and don't say " Blowing up ", it hurts my feelings.
– Shamraiz
Nov 21 at 3:06
1
@Shamraiz //for_loop to multiply each element store in std::array -- This is a big reason why you should write your templated functions using iterators, not with concrete container types. If you had done that, the user would simply pass to you the start and end iterator, and it wouldn't matter if the container was astd::array
,std::vector
, or just a plain array.
– PaulMcKenzie
Nov 21 at 3:44
@PaulMcKenzie That sounds like decent and logical. I will give it a try
– Shamraiz
Nov 21 at 3:47
2
2
If you're throwing a stack overflow exception, you're blowing the stack. End of. Ergo, don't blow the stack. (Someone with better template experience will have to tell you how you're blowing the stack.)
– Robert Harvey♦
Nov 21 at 2:10
If you're throwing a stack overflow exception, you're blowing the stack. End of. Ergo, don't blow the stack. (Someone with better template experience will have to tell you how you're blowing the stack.)
– Robert Harvey♦
Nov 21 at 2:10
Anything which I didn't mention? But thank you and don't say " Blowing up ", it hurts my feelings.
– Shamraiz
Nov 21 at 3:06
Anything which I didn't mention? But thank you and don't say " Blowing up ", it hurts my feelings.
– Shamraiz
Nov 21 at 3:06
1
1
@Shamraiz //for_loop to multiply each element store in std::array -- This is a big reason why you should write your templated functions using iterators, not with concrete container types. If you had done that, the user would simply pass to you the start and end iterator, and it wouldn't matter if the container was a
std::array
, std::vector
, or just a plain array.– PaulMcKenzie
Nov 21 at 3:44
@Shamraiz //for_loop to multiply each element store in std::array -- This is a big reason why you should write your templated functions using iterators, not with concrete container types. If you had done that, the user would simply pass to you the start and end iterator, and it wouldn't matter if the container was a
std::array
, std::vector
, or just a plain array.– PaulMcKenzie
Nov 21 at 3:44
@PaulMcKenzie That sounds like decent and logical. I will give it a try
– Shamraiz
Nov 21 at 3:47
@PaulMcKenzie That sounds like decent and logical. I will give it a try
– Shamraiz
Nov 21 at 3:47
add a comment |
1 Answer
1
active
oldest
votes
You simply cannot create such large objects on the stack, you must create them on the heap instead.
template <int entry>
std::unique_ptr<Node<T,Point,entry>> operator* (const Node<T,End,entry> &source)
{
auto temporary_node = std::make_unique<Node<T,Point,entry>>(0.);
//for_loop to multiply each element store in std::array
return temporary_node;
}
I appreciate your suggestion but It doesn't help. Since test cases do not expect back pointers.
– Shamraiz
Nov 21 at 3:05
and it is working on Linux though as I heard maybe because stack size on linux is around 8MB considering virtual
– Shamraiz
Nov 21 at 3:24
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404373%2fstuck-with-unhandled-exception-at-0x00007ff74f27a526-stack-overflow%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You simply cannot create such large objects on the stack, you must create them on the heap instead.
template <int entry>
std::unique_ptr<Node<T,Point,entry>> operator* (const Node<T,End,entry> &source)
{
auto temporary_node = std::make_unique<Node<T,Point,entry>>(0.);
//for_loop to multiply each element store in std::array
return temporary_node;
}
I appreciate your suggestion but It doesn't help. Since test cases do not expect back pointers.
– Shamraiz
Nov 21 at 3:05
and it is working on Linux though as I heard maybe because stack size on linux is around 8MB considering virtual
– Shamraiz
Nov 21 at 3:24
add a comment |
You simply cannot create such large objects on the stack, you must create them on the heap instead.
template <int entry>
std::unique_ptr<Node<T,Point,entry>> operator* (const Node<T,End,entry> &source)
{
auto temporary_node = std::make_unique<Node<T,Point,entry>>(0.);
//for_loop to multiply each element store in std::array
return temporary_node;
}
I appreciate your suggestion but It doesn't help. Since test cases do not expect back pointers.
– Shamraiz
Nov 21 at 3:05
and it is working on Linux though as I heard maybe because stack size on linux is around 8MB considering virtual
– Shamraiz
Nov 21 at 3:24
add a comment |
You simply cannot create such large objects on the stack, you must create them on the heap instead.
template <int entry>
std::unique_ptr<Node<T,Point,entry>> operator* (const Node<T,End,entry> &source)
{
auto temporary_node = std::make_unique<Node<T,Point,entry>>(0.);
//for_loop to multiply each element store in std::array
return temporary_node;
}
You simply cannot create such large objects on the stack, you must create them on the heap instead.
template <int entry>
std::unique_ptr<Node<T,Point,entry>> operator* (const Node<T,End,entry> &source)
{
auto temporary_node = std::make_unique<Node<T,Point,entry>>(0.);
//for_loop to multiply each element store in std::array
return temporary_node;
}
answered Nov 21 at 2:48


Peter Ruderman
10.1k2352
10.1k2352
I appreciate your suggestion but It doesn't help. Since test cases do not expect back pointers.
– Shamraiz
Nov 21 at 3:05
and it is working on Linux though as I heard maybe because stack size on linux is around 8MB considering virtual
– Shamraiz
Nov 21 at 3:24
add a comment |
I appreciate your suggestion but It doesn't help. Since test cases do not expect back pointers.
– Shamraiz
Nov 21 at 3:05
and it is working on Linux though as I heard maybe because stack size on linux is around 8MB considering virtual
– Shamraiz
Nov 21 at 3:24
I appreciate your suggestion but It doesn't help. Since test cases do not expect back pointers.
– Shamraiz
Nov 21 at 3:05
I appreciate your suggestion but It doesn't help. Since test cases do not expect back pointers.
– Shamraiz
Nov 21 at 3:05
and it is working on Linux though as I heard maybe because stack size on linux is around 8MB considering virtual
– Shamraiz
Nov 21 at 3:24
and it is working on Linux though as I heard maybe because stack size on linux is around 8MB considering virtual
– Shamraiz
Nov 21 at 3:24
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404373%2fstuck-with-unhandled-exception-at-0x00007ff74f27a526-stack-overflow%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sF8yr,PT5xLoEnIKo,WaYxj9WDn,P3
2
If you're throwing a stack overflow exception, you're blowing the stack. End of. Ergo, don't blow the stack. (Someone with better template experience will have to tell you how you're blowing the stack.)
– Robert Harvey♦
Nov 21 at 2:10
Anything which I didn't mention? But thank you and don't say " Blowing up ", it hurts my feelings.
– Shamraiz
Nov 21 at 3:06
1
@Shamraiz //for_loop to multiply each element store in std::array -- This is a big reason why you should write your templated functions using iterators, not with concrete container types. If you had done that, the user would simply pass to you the start and end iterator, and it wouldn't matter if the container was a
std::array
,std::vector
, or just a plain array.– PaulMcKenzie
Nov 21 at 3:44
@PaulMcKenzie That sounds like decent and logical. I will give it a try
– Shamraiz
Nov 21 at 3:47