How to use 2 higher-order components at the same time? (in order to use injectIntl from react-intl)
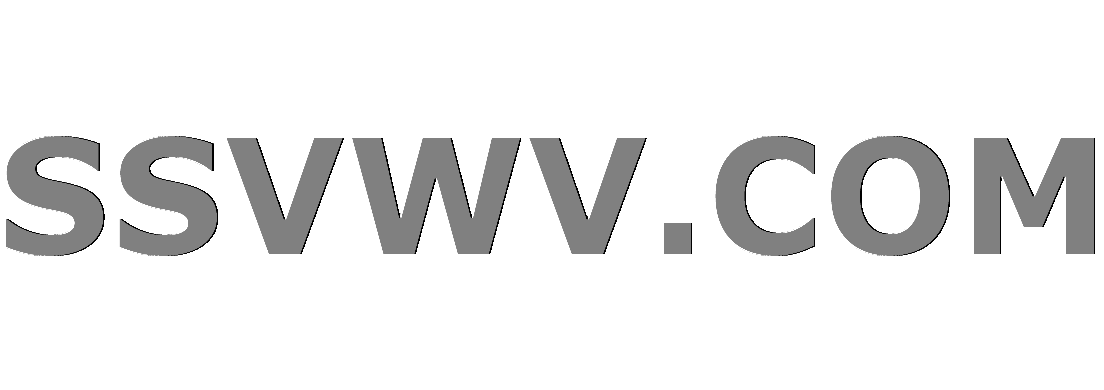
Multi tool use
I know that in order to use injectIntl from the react-intl library I will need something like:
export default injectIntl(SectionNavbars)
However I am already using the withStyles higher order component, how can I combine both?
import React from 'react'
/* more stuff */
import { injectIntl } from 'react-intl'
class SectionNavbars extends React.Component {
render() {
const { classes } = this.props;
const { intl } = this.props;
return (
<div className={classes.section}>
<Header
brand={ <img src={logo}/> }
rightLinks={
<ListItem className={classes.listItem}>
<CustomDropdown
buttonText={intl.formatMessage({ id: 'products' })}
buttonProps={{
className: classes.navLink,
}}
dropdownList={[
/*stuff*/
]}
/>
</ListItem>
}
/>
</div>
);
}
}
export default withStyles(navbarsStyle)(SectionNavbars);
javascript reactjs react-intl
add a comment |
I know that in order to use injectIntl from the react-intl library I will need something like:
export default injectIntl(SectionNavbars)
However I am already using the withStyles higher order component, how can I combine both?
import React from 'react'
/* more stuff */
import { injectIntl } from 'react-intl'
class SectionNavbars extends React.Component {
render() {
const { classes } = this.props;
const { intl } = this.props;
return (
<div className={classes.section}>
<Header
brand={ <img src={logo}/> }
rightLinks={
<ListItem className={classes.listItem}>
<CustomDropdown
buttonText={intl.formatMessage({ id: 'products' })}
buttonProps={{
className: classes.navLink,
}}
dropdownList={[
/*stuff*/
]}
/>
</ListItem>
}
/>
</div>
);
}
}
export default withStyles(navbarsStyle)(SectionNavbars);
javascript reactjs react-intl
add a comment |
I know that in order to use injectIntl from the react-intl library I will need something like:
export default injectIntl(SectionNavbars)
However I am already using the withStyles higher order component, how can I combine both?
import React from 'react'
/* more stuff */
import { injectIntl } from 'react-intl'
class SectionNavbars extends React.Component {
render() {
const { classes } = this.props;
const { intl } = this.props;
return (
<div className={classes.section}>
<Header
brand={ <img src={logo}/> }
rightLinks={
<ListItem className={classes.listItem}>
<CustomDropdown
buttonText={intl.formatMessage({ id: 'products' })}
buttonProps={{
className: classes.navLink,
}}
dropdownList={[
/*stuff*/
]}
/>
</ListItem>
}
/>
</div>
);
}
}
export default withStyles(navbarsStyle)(SectionNavbars);
javascript reactjs react-intl
I know that in order to use injectIntl from the react-intl library I will need something like:
export default injectIntl(SectionNavbars)
However I am already using the withStyles higher order component, how can I combine both?
import React from 'react'
/* more stuff */
import { injectIntl } from 'react-intl'
class SectionNavbars extends React.Component {
render() {
const { classes } = this.props;
const { intl } = this.props;
return (
<div className={classes.section}>
<Header
brand={ <img src={logo}/> }
rightLinks={
<ListItem className={classes.listItem}>
<CustomDropdown
buttonText={intl.formatMessage({ id: 'products' })}
buttonProps={{
className: classes.navLink,
}}
dropdownList={[
/*stuff*/
]}
/>
</ListItem>
}
/>
</div>
);
}
}
export default withStyles(navbarsStyle)(SectionNavbars);
javascript reactjs react-intl
javascript reactjs react-intl
edited Nov 21 at 4:39
asked Nov 21 at 3:41


molusken
135
135
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can combine HOC like this injectIntl(withStyles(navbarsStyle)(SectionNavbars))
Or better
- you can use
compose
from recompose library
- other helpers for functional programming like
flow
andflowRight
from lodash
Also, I would recommend you to read recompose documentation it really helps to understand HOC and provides really useful stuff.
injectIntl(withStyles(navbarsStyle)(SectionNavbars)) is working fine, thanks! While I am at it may I ask why it would be better to use the recompose library?
– molusken
Nov 21 at 4:38
Two reasons: 1. It's better organization of the code. It's easier to read and edit. In your case, there are only two functions but it may be much more. 2. Knowledge of recompose can save you a lot of time. Amoung other HOC I would especialy recommend you to take a closer look atwithStateHandlers()
,withPropsOnChange()
. Having "Pure" component and all state and logic encapsulated into HOC organized withcompose
is a very good practice.
– wanjas
Nov 21 at 5:26
I will look into this, thanks a lot.
– molusken
Nov 21 at 6:11
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404952%2fhow-to-use-2-higher-order-components-at-the-same-time-in-order-to-use-injectin%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can combine HOC like this injectIntl(withStyles(navbarsStyle)(SectionNavbars))
Or better
- you can use
compose
from recompose library
- other helpers for functional programming like
flow
andflowRight
from lodash
Also, I would recommend you to read recompose documentation it really helps to understand HOC and provides really useful stuff.
injectIntl(withStyles(navbarsStyle)(SectionNavbars)) is working fine, thanks! While I am at it may I ask why it would be better to use the recompose library?
– molusken
Nov 21 at 4:38
Two reasons: 1. It's better organization of the code. It's easier to read and edit. In your case, there are only two functions but it may be much more. 2. Knowledge of recompose can save you a lot of time. Amoung other HOC I would especialy recommend you to take a closer look atwithStateHandlers()
,withPropsOnChange()
. Having "Pure" component and all state and logic encapsulated into HOC organized withcompose
is a very good practice.
– wanjas
Nov 21 at 5:26
I will look into this, thanks a lot.
– molusken
Nov 21 at 6:11
add a comment |
You can combine HOC like this injectIntl(withStyles(navbarsStyle)(SectionNavbars))
Or better
- you can use
compose
from recompose library
- other helpers for functional programming like
flow
andflowRight
from lodash
Also, I would recommend you to read recompose documentation it really helps to understand HOC and provides really useful stuff.
injectIntl(withStyles(navbarsStyle)(SectionNavbars)) is working fine, thanks! While I am at it may I ask why it would be better to use the recompose library?
– molusken
Nov 21 at 4:38
Two reasons: 1. It's better organization of the code. It's easier to read and edit. In your case, there are only two functions but it may be much more. 2. Knowledge of recompose can save you a lot of time. Amoung other HOC I would especialy recommend you to take a closer look atwithStateHandlers()
,withPropsOnChange()
. Having "Pure" component and all state and logic encapsulated into HOC organized withcompose
is a very good practice.
– wanjas
Nov 21 at 5:26
I will look into this, thanks a lot.
– molusken
Nov 21 at 6:11
add a comment |
You can combine HOC like this injectIntl(withStyles(navbarsStyle)(SectionNavbars))
Or better
- you can use
compose
from recompose library
- other helpers for functional programming like
flow
andflowRight
from lodash
Also, I would recommend you to read recompose documentation it really helps to understand HOC and provides really useful stuff.
You can combine HOC like this injectIntl(withStyles(navbarsStyle)(SectionNavbars))
Or better
- you can use
compose
from recompose library
- other helpers for functional programming like
flow
andflowRight
from lodash
Also, I would recommend you to read recompose documentation it really helps to understand HOC and provides really useful stuff.
answered Nov 21 at 4:28
wanjas
1447
1447
injectIntl(withStyles(navbarsStyle)(SectionNavbars)) is working fine, thanks! While I am at it may I ask why it would be better to use the recompose library?
– molusken
Nov 21 at 4:38
Two reasons: 1. It's better organization of the code. It's easier to read and edit. In your case, there are only two functions but it may be much more. 2. Knowledge of recompose can save you a lot of time. Amoung other HOC I would especialy recommend you to take a closer look atwithStateHandlers()
,withPropsOnChange()
. Having "Pure" component and all state and logic encapsulated into HOC organized withcompose
is a very good practice.
– wanjas
Nov 21 at 5:26
I will look into this, thanks a lot.
– molusken
Nov 21 at 6:11
add a comment |
injectIntl(withStyles(navbarsStyle)(SectionNavbars)) is working fine, thanks! While I am at it may I ask why it would be better to use the recompose library?
– molusken
Nov 21 at 4:38
Two reasons: 1. It's better organization of the code. It's easier to read and edit. In your case, there are only two functions but it may be much more. 2. Knowledge of recompose can save you a lot of time. Amoung other HOC I would especialy recommend you to take a closer look atwithStateHandlers()
,withPropsOnChange()
. Having "Pure" component and all state and logic encapsulated into HOC organized withcompose
is a very good practice.
– wanjas
Nov 21 at 5:26
I will look into this, thanks a lot.
– molusken
Nov 21 at 6:11
injectIntl(withStyles(navbarsStyle)(SectionNavbars)) is working fine, thanks! While I am at it may I ask why it would be better to use the recompose library?
– molusken
Nov 21 at 4:38
injectIntl(withStyles(navbarsStyle)(SectionNavbars)) is working fine, thanks! While I am at it may I ask why it would be better to use the recompose library?
– molusken
Nov 21 at 4:38
Two reasons: 1. It's better organization of the code. It's easier to read and edit. In your case, there are only two functions but it may be much more. 2. Knowledge of recompose can save you a lot of time. Amoung other HOC I would especialy recommend you to take a closer look at
withStateHandlers()
, withPropsOnChange()
. Having "Pure" component and all state and logic encapsulated into HOC organized with compose
is a very good practice.– wanjas
Nov 21 at 5:26
Two reasons: 1. It's better organization of the code. It's easier to read and edit. In your case, there are only two functions but it may be much more. 2. Knowledge of recompose can save you a lot of time. Amoung other HOC I would especialy recommend you to take a closer look at
withStateHandlers()
, withPropsOnChange()
. Having "Pure" component and all state and logic encapsulated into HOC organized with compose
is a very good practice.– wanjas
Nov 21 at 5:26
I will look into this, thanks a lot.
– molusken
Nov 21 at 6:11
I will look into this, thanks a lot.
– molusken
Nov 21 at 6:11
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404952%2fhow-to-use-2-higher-order-components-at-the-same-time-in-order-to-use-injectin%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pL,iiqf,2h h2t 2fBj6P,R401 k,Q eEOd9goejv MTL H9jhdQrKw6984O6UnqtLPnXnYTjwFkj8 E4selSz,V,ytz3b7pGiXQHV 7,7kk2,pB