Still don't get how to mock an imported library - Python
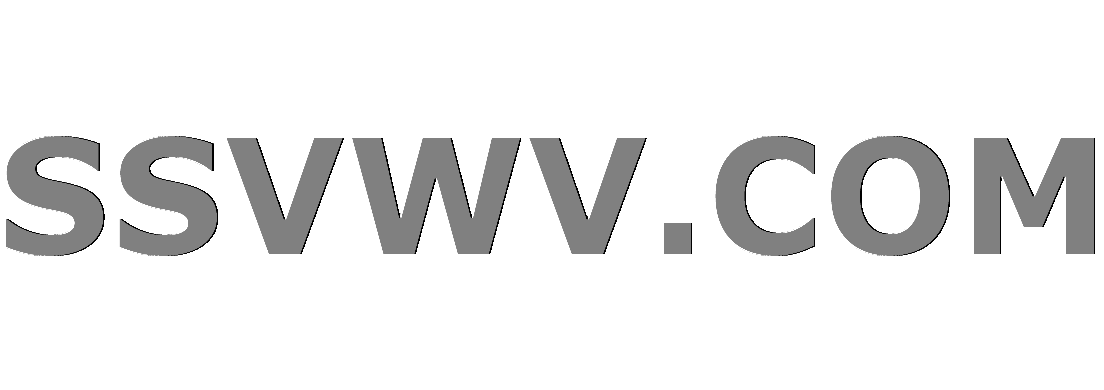
Multi tool use
I've looked around but still don't get how to mock a library used inside a function and assert that its been called properly.
a.py
import win32clipboard
def copy():
win32clipboard.OpenClipboard()
win32clipboard.EmptyClipboard()
win32clipboard.SetClipboardText('dummy')
win32clipboard.CloseClipboard()
test_a.py
import a
import pytest
def test_copy():
# Mock win32clipboard somehow
# Run a.copy()
# assert mock win32clipboard.call_count == 4
python mocking pytest
add a comment |
I've looked around but still don't get how to mock a library used inside a function and assert that its been called properly.
a.py
import win32clipboard
def copy():
win32clipboard.OpenClipboard()
win32clipboard.EmptyClipboard()
win32clipboard.SetClipboardText('dummy')
win32clipboard.CloseClipboard()
test_a.py
import a
import pytest
def test_copy():
# Mock win32clipboard somehow
# Run a.copy()
# assert mock win32clipboard.call_count == 4
python mocking pytest
add a comment |
I've looked around but still don't get how to mock a library used inside a function and assert that its been called properly.
a.py
import win32clipboard
def copy():
win32clipboard.OpenClipboard()
win32clipboard.EmptyClipboard()
win32clipboard.SetClipboardText('dummy')
win32clipboard.CloseClipboard()
test_a.py
import a
import pytest
def test_copy():
# Mock win32clipboard somehow
# Run a.copy()
# assert mock win32clipboard.call_count == 4
python mocking pytest
I've looked around but still don't get how to mock a library used inside a function and assert that its been called properly.
a.py
import win32clipboard
def copy():
win32clipboard.OpenClipboard()
win32clipboard.EmptyClipboard()
win32clipboard.SetClipboardText('dummy')
win32clipboard.CloseClipboard()
test_a.py
import a
import pytest
def test_copy():
# Mock win32clipboard somehow
# Run a.copy()
# assert mock win32clipboard.call_count == 4
python mocking pytest
python mocking pytest
asked Nov 4 '18 at 21:17
curiousgeorgecuriousgeorge
417
417
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
There is a mistake in your approach.
win32clipboard
is a library, with some classes and methods. You must mock every class from this library you want to use (OpenClipboard
, EmptyClipboard
, SetClipboardText
and CloseClipboard
)
import a
import pytest
from unittest.mock import patch
@patch('win32clipboard.OpenClipboard')
@patch('win32clipboard.EmptyClipboard')
@patch('win32clipboard.SetClipboardText')
@patch('win32clipboard.CloseClipboard')
def test_copy(mock_close, mock_set, mock_empty, mock_open):
a.copy()
assert mock_close.called
assert mock_set.called
assert mock_empty.called
assert mock_open.called
1
I actually found out the answer to my own question here some time ago already but my post got deleted for not clarifying enough, apparently. I wasn't even aware since I never came back to check this thread but thanks for giving a perfect example (using decorators) for others to see how its done @MauroBaraldi!
– curiousgeorge
Nov 24 '18 at 21:36
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53145554%2fstill-dont-get-how-to-mock-an-imported-library-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is a mistake in your approach.
win32clipboard
is a library, with some classes and methods. You must mock every class from this library you want to use (OpenClipboard
, EmptyClipboard
, SetClipboardText
and CloseClipboard
)
import a
import pytest
from unittest.mock import patch
@patch('win32clipboard.OpenClipboard')
@patch('win32clipboard.EmptyClipboard')
@patch('win32clipboard.SetClipboardText')
@patch('win32clipboard.CloseClipboard')
def test_copy(mock_close, mock_set, mock_empty, mock_open):
a.copy()
assert mock_close.called
assert mock_set.called
assert mock_empty.called
assert mock_open.called
1
I actually found out the answer to my own question here some time ago already but my post got deleted for not clarifying enough, apparently. I wasn't even aware since I never came back to check this thread but thanks for giving a perfect example (using decorators) for others to see how its done @MauroBaraldi!
– curiousgeorge
Nov 24 '18 at 21:36
add a comment |
There is a mistake in your approach.
win32clipboard
is a library, with some classes and methods. You must mock every class from this library you want to use (OpenClipboard
, EmptyClipboard
, SetClipboardText
and CloseClipboard
)
import a
import pytest
from unittest.mock import patch
@patch('win32clipboard.OpenClipboard')
@patch('win32clipboard.EmptyClipboard')
@patch('win32clipboard.SetClipboardText')
@patch('win32clipboard.CloseClipboard')
def test_copy(mock_close, mock_set, mock_empty, mock_open):
a.copy()
assert mock_close.called
assert mock_set.called
assert mock_empty.called
assert mock_open.called
1
I actually found out the answer to my own question here some time ago already but my post got deleted for not clarifying enough, apparently. I wasn't even aware since I never came back to check this thread but thanks for giving a perfect example (using decorators) for others to see how its done @MauroBaraldi!
– curiousgeorge
Nov 24 '18 at 21:36
add a comment |
There is a mistake in your approach.
win32clipboard
is a library, with some classes and methods. You must mock every class from this library you want to use (OpenClipboard
, EmptyClipboard
, SetClipboardText
and CloseClipboard
)
import a
import pytest
from unittest.mock import patch
@patch('win32clipboard.OpenClipboard')
@patch('win32clipboard.EmptyClipboard')
@patch('win32clipboard.SetClipboardText')
@patch('win32clipboard.CloseClipboard')
def test_copy(mock_close, mock_set, mock_empty, mock_open):
a.copy()
assert mock_close.called
assert mock_set.called
assert mock_empty.called
assert mock_open.called
There is a mistake in your approach.
win32clipboard
is a library, with some classes and methods. You must mock every class from this library you want to use (OpenClipboard
, EmptyClipboard
, SetClipboardText
and CloseClipboard
)
import a
import pytest
from unittest.mock import patch
@patch('win32clipboard.OpenClipboard')
@patch('win32clipboard.EmptyClipboard')
@patch('win32clipboard.SetClipboardText')
@patch('win32clipboard.CloseClipboard')
def test_copy(mock_close, mock_set, mock_empty, mock_open):
a.copy()
assert mock_close.called
assert mock_set.called
assert mock_empty.called
assert mock_open.called
answered Nov 23 '18 at 20:08
Mauro BaraldiMauro Baraldi
4,03112032
4,03112032
1
I actually found out the answer to my own question here some time ago already but my post got deleted for not clarifying enough, apparently. I wasn't even aware since I never came back to check this thread but thanks for giving a perfect example (using decorators) for others to see how its done @MauroBaraldi!
– curiousgeorge
Nov 24 '18 at 21:36
add a comment |
1
I actually found out the answer to my own question here some time ago already but my post got deleted for not clarifying enough, apparently. I wasn't even aware since I never came back to check this thread but thanks for giving a perfect example (using decorators) for others to see how its done @MauroBaraldi!
– curiousgeorge
Nov 24 '18 at 21:36
1
1
I actually found out the answer to my own question here some time ago already but my post got deleted for not clarifying enough, apparently. I wasn't even aware since I never came back to check this thread but thanks for giving a perfect example (using decorators) for others to see how its done @MauroBaraldi!
– curiousgeorge
Nov 24 '18 at 21:36
I actually found out the answer to my own question here some time ago already but my post got deleted for not clarifying enough, apparently. I wasn't even aware since I never came back to check this thread but thanks for giving a perfect example (using decorators) for others to see how its done @MauroBaraldi!
– curiousgeorge
Nov 24 '18 at 21:36
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53145554%2fstill-dont-get-how-to-mock-an-imported-library-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S,3opx y1Y NCidWLwSJnD8SDlaQgNujD212SeEhOyc y nPVkw6uCSsRfKFvaQZNH5es6 gBkY3 aK 532KybYXM5CAJ3JkD