How to maintain drag shadow opacity after release event
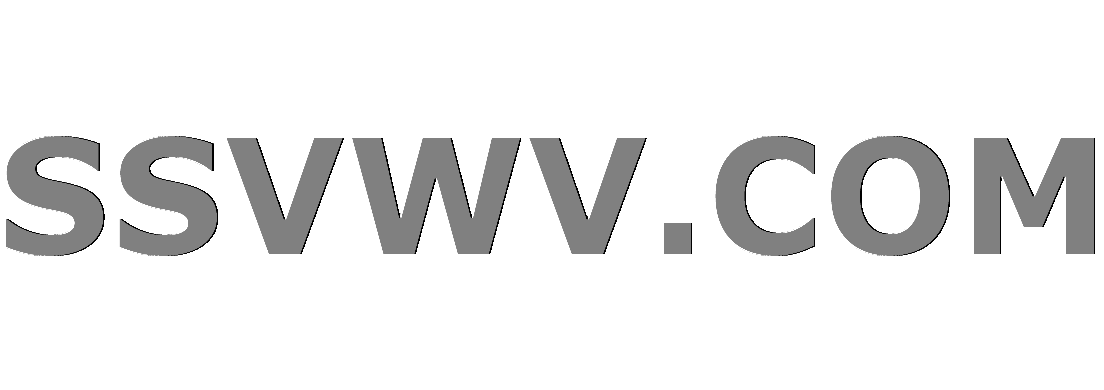
Multi tool use
I was able to make the drag shadow opaque with opaque flag
view.startDrag(data, shadowBuilder, view, DRAG_FLAG_OPAQUE);
However, when I release the drag shadow, it becomes translucent as it floats back to it's touch position.
I would like the drag shadow to maintain 100% opacity throughout its entire life cycle. Is there a way to do this?
@Override
public boolean onTouch(View view, MotionEvent motionEvent)
{
Log.d(TAG,"onTouch()");
switch (motionEvent.getAction())
{
case ACTION_DOWN:
Log.d(TAG, "ACTION_DOWN");
int topLeft = new int[2];
view.getLocationOnScreen(topLeft);
float touchPointX = motionEvent.getRawX() - topLeft[0];
float touchPointY = motionEvent.getRawY() - topLeft[1];
ClipData data = ClipData.newPlainText("", "");
//DragShadowBuilder shadowBuilder = new View.DragShadowBuilder(view);
MyDragShadowBuilder shadowBuilder = new MyDragShadowBuilder((int)touchPointY,(int)touchPointX,view);
view.setAlpha(0); //transparent thus invisible
view.startDrag(data, shadowBuilder, view, DRAG_FLAG_OPAQUE);
break;
case ACTION_MOVE:
Log.d(TAG, "ACTION_MOVE");
break;
default :
return false;
}
return true;
}
private static class MyDragShadowBuilder extends View.DragShadowBuilder {
int mTouchPointY;
int mTouchPointX;
View mView;
// The drag shadow image, defined as a drawable thing
private static Drawable shadow;
// Defines the constructor for myDragShadowBuilder
public MyDragShadowBuilder(int touchPointY, int touchPointX,View view) {
// Stores the View parameter passed to myDragShadowBuilder.
super(view);
mView = view;
mTouchPointY = touchPointY;
mTouchPointX = touchPointX;
// Creates a draggable image that will fill the Canvas provided by the system.
shadow = new ColorDrawable(Color.WHITE);
}
// Defines a callback that sends the drag shadow dimensions and touch point back to the
// system.
@Override
public void onProvideShadowMetrics (Point size, Point touch) {
// Defines local variables
int width, height;
// Sets the width of the shadow to half the width of the original View
width = getView().getWidth();// / 2;
// Sets the height of the shadow to half the height of the original View
height = getView().getHeight();// / 2;
// The drag shadow is a ColorDrawable. This sets its dimensions to be the same as the
// Canvas that the system will provide. As a result, the drag shadow will fill the
// Canvas.
shadow.setBounds(0, 0, width, height);
// Sets the size parameter's width and height values. These get back to the system
// through the size parameter.
size.set(width, height);
// Sets the touch point's position to be in the middle of the drag shadow
touch.set(mTouchPointX, mTouchPointY);
}
// Defines a callback that draws the drag shadow in a Canvas that the system constructs
// from the dimensions passed in onProvideShadowMetrics().
@Override
public void onDrawShadow(Canvas canvas) {
// Draws the ColorDrawable in the Canvas passed in from the system.
//canvas.drawARGB(0,0,0,0);
mView.draw(canvas);
//shadow.draw(canvas);
}
}
@Override
public boolean onDrag(View view, DragEvent event)
{
Log.d(TAG,"onDrag()");
final View viewToDrag = (View) event.getLocalState();
switch (event.getAction()) {
case DragEvent.ACTION_DRAG_STARTED:
Log.d(TAG,"ACTION_DRAG_STARTED");
break;
case DragEvent.ACTION_DRAG_ENTERED:
Log.d(TAG,"ACTION_DRAG_ENTERED");
break;
case DragEvent.ACTION_DRAG_EXITED:
Log.d(TAG,"ACTION_DRAG_EXITED");
break;
case DragEvent.ACTION_DROP:
Log.d(TAG,"ACTION_DROP");
break;
case DragEvent.ACTION_DRAG_ENDED:
Log.d(TAG,"ACTION_DRAG_ENDED");
viewToDrag.setAlpha(1f); //transparent thus invisible
break;
default:
Log.d(TAG,"default");
break;
}
return true;
}


add a comment |
I was able to make the drag shadow opaque with opaque flag
view.startDrag(data, shadowBuilder, view, DRAG_FLAG_OPAQUE);
However, when I release the drag shadow, it becomes translucent as it floats back to it's touch position.
I would like the drag shadow to maintain 100% opacity throughout its entire life cycle. Is there a way to do this?
@Override
public boolean onTouch(View view, MotionEvent motionEvent)
{
Log.d(TAG,"onTouch()");
switch (motionEvent.getAction())
{
case ACTION_DOWN:
Log.d(TAG, "ACTION_DOWN");
int topLeft = new int[2];
view.getLocationOnScreen(topLeft);
float touchPointX = motionEvent.getRawX() - topLeft[0];
float touchPointY = motionEvent.getRawY() - topLeft[1];
ClipData data = ClipData.newPlainText("", "");
//DragShadowBuilder shadowBuilder = new View.DragShadowBuilder(view);
MyDragShadowBuilder shadowBuilder = new MyDragShadowBuilder((int)touchPointY,(int)touchPointX,view);
view.setAlpha(0); //transparent thus invisible
view.startDrag(data, shadowBuilder, view, DRAG_FLAG_OPAQUE);
break;
case ACTION_MOVE:
Log.d(TAG, "ACTION_MOVE");
break;
default :
return false;
}
return true;
}
private static class MyDragShadowBuilder extends View.DragShadowBuilder {
int mTouchPointY;
int mTouchPointX;
View mView;
// The drag shadow image, defined as a drawable thing
private static Drawable shadow;
// Defines the constructor for myDragShadowBuilder
public MyDragShadowBuilder(int touchPointY, int touchPointX,View view) {
// Stores the View parameter passed to myDragShadowBuilder.
super(view);
mView = view;
mTouchPointY = touchPointY;
mTouchPointX = touchPointX;
// Creates a draggable image that will fill the Canvas provided by the system.
shadow = new ColorDrawable(Color.WHITE);
}
// Defines a callback that sends the drag shadow dimensions and touch point back to the
// system.
@Override
public void onProvideShadowMetrics (Point size, Point touch) {
// Defines local variables
int width, height;
// Sets the width of the shadow to half the width of the original View
width = getView().getWidth();// / 2;
// Sets the height of the shadow to half the height of the original View
height = getView().getHeight();// / 2;
// The drag shadow is a ColorDrawable. This sets its dimensions to be the same as the
// Canvas that the system will provide. As a result, the drag shadow will fill the
// Canvas.
shadow.setBounds(0, 0, width, height);
// Sets the size parameter's width and height values. These get back to the system
// through the size parameter.
size.set(width, height);
// Sets the touch point's position to be in the middle of the drag shadow
touch.set(mTouchPointX, mTouchPointY);
}
// Defines a callback that draws the drag shadow in a Canvas that the system constructs
// from the dimensions passed in onProvideShadowMetrics().
@Override
public void onDrawShadow(Canvas canvas) {
// Draws the ColorDrawable in the Canvas passed in from the system.
//canvas.drawARGB(0,0,0,0);
mView.draw(canvas);
//shadow.draw(canvas);
}
}
@Override
public boolean onDrag(View view, DragEvent event)
{
Log.d(TAG,"onDrag()");
final View viewToDrag = (View) event.getLocalState();
switch (event.getAction()) {
case DragEvent.ACTION_DRAG_STARTED:
Log.d(TAG,"ACTION_DRAG_STARTED");
break;
case DragEvent.ACTION_DRAG_ENTERED:
Log.d(TAG,"ACTION_DRAG_ENTERED");
break;
case DragEvent.ACTION_DRAG_EXITED:
Log.d(TAG,"ACTION_DRAG_EXITED");
break;
case DragEvent.ACTION_DROP:
Log.d(TAG,"ACTION_DROP");
break;
case DragEvent.ACTION_DRAG_ENDED:
Log.d(TAG,"ACTION_DRAG_ENDED");
viewToDrag.setAlpha(1f); //transparent thus invisible
break;
default:
Log.d(TAG,"default");
break;
}
return true;
}


add a comment |
I was able to make the drag shadow opaque with opaque flag
view.startDrag(data, shadowBuilder, view, DRAG_FLAG_OPAQUE);
However, when I release the drag shadow, it becomes translucent as it floats back to it's touch position.
I would like the drag shadow to maintain 100% opacity throughout its entire life cycle. Is there a way to do this?
@Override
public boolean onTouch(View view, MotionEvent motionEvent)
{
Log.d(TAG,"onTouch()");
switch (motionEvent.getAction())
{
case ACTION_DOWN:
Log.d(TAG, "ACTION_DOWN");
int topLeft = new int[2];
view.getLocationOnScreen(topLeft);
float touchPointX = motionEvent.getRawX() - topLeft[0];
float touchPointY = motionEvent.getRawY() - topLeft[1];
ClipData data = ClipData.newPlainText("", "");
//DragShadowBuilder shadowBuilder = new View.DragShadowBuilder(view);
MyDragShadowBuilder shadowBuilder = new MyDragShadowBuilder((int)touchPointY,(int)touchPointX,view);
view.setAlpha(0); //transparent thus invisible
view.startDrag(data, shadowBuilder, view, DRAG_FLAG_OPAQUE);
break;
case ACTION_MOVE:
Log.d(TAG, "ACTION_MOVE");
break;
default :
return false;
}
return true;
}
private static class MyDragShadowBuilder extends View.DragShadowBuilder {
int mTouchPointY;
int mTouchPointX;
View mView;
// The drag shadow image, defined as a drawable thing
private static Drawable shadow;
// Defines the constructor for myDragShadowBuilder
public MyDragShadowBuilder(int touchPointY, int touchPointX,View view) {
// Stores the View parameter passed to myDragShadowBuilder.
super(view);
mView = view;
mTouchPointY = touchPointY;
mTouchPointX = touchPointX;
// Creates a draggable image that will fill the Canvas provided by the system.
shadow = new ColorDrawable(Color.WHITE);
}
// Defines a callback that sends the drag shadow dimensions and touch point back to the
// system.
@Override
public void onProvideShadowMetrics (Point size, Point touch) {
// Defines local variables
int width, height;
// Sets the width of the shadow to half the width of the original View
width = getView().getWidth();// / 2;
// Sets the height of the shadow to half the height of the original View
height = getView().getHeight();// / 2;
// The drag shadow is a ColorDrawable. This sets its dimensions to be the same as the
// Canvas that the system will provide. As a result, the drag shadow will fill the
// Canvas.
shadow.setBounds(0, 0, width, height);
// Sets the size parameter's width and height values. These get back to the system
// through the size parameter.
size.set(width, height);
// Sets the touch point's position to be in the middle of the drag shadow
touch.set(mTouchPointX, mTouchPointY);
}
// Defines a callback that draws the drag shadow in a Canvas that the system constructs
// from the dimensions passed in onProvideShadowMetrics().
@Override
public void onDrawShadow(Canvas canvas) {
// Draws the ColorDrawable in the Canvas passed in from the system.
//canvas.drawARGB(0,0,0,0);
mView.draw(canvas);
//shadow.draw(canvas);
}
}
@Override
public boolean onDrag(View view, DragEvent event)
{
Log.d(TAG,"onDrag()");
final View viewToDrag = (View) event.getLocalState();
switch (event.getAction()) {
case DragEvent.ACTION_DRAG_STARTED:
Log.d(TAG,"ACTION_DRAG_STARTED");
break;
case DragEvent.ACTION_DRAG_ENTERED:
Log.d(TAG,"ACTION_DRAG_ENTERED");
break;
case DragEvent.ACTION_DRAG_EXITED:
Log.d(TAG,"ACTION_DRAG_EXITED");
break;
case DragEvent.ACTION_DROP:
Log.d(TAG,"ACTION_DROP");
break;
case DragEvent.ACTION_DRAG_ENDED:
Log.d(TAG,"ACTION_DRAG_ENDED");
viewToDrag.setAlpha(1f); //transparent thus invisible
break;
default:
Log.d(TAG,"default");
break;
}
return true;
}


I was able to make the drag shadow opaque with opaque flag
view.startDrag(data, shadowBuilder, view, DRAG_FLAG_OPAQUE);
However, when I release the drag shadow, it becomes translucent as it floats back to it's touch position.
I would like the drag shadow to maintain 100% opacity throughout its entire life cycle. Is there a way to do this?
@Override
public boolean onTouch(View view, MotionEvent motionEvent)
{
Log.d(TAG,"onTouch()");
switch (motionEvent.getAction())
{
case ACTION_DOWN:
Log.d(TAG, "ACTION_DOWN");
int topLeft = new int[2];
view.getLocationOnScreen(topLeft);
float touchPointX = motionEvent.getRawX() - topLeft[0];
float touchPointY = motionEvent.getRawY() - topLeft[1];
ClipData data = ClipData.newPlainText("", "");
//DragShadowBuilder shadowBuilder = new View.DragShadowBuilder(view);
MyDragShadowBuilder shadowBuilder = new MyDragShadowBuilder((int)touchPointY,(int)touchPointX,view);
view.setAlpha(0); //transparent thus invisible
view.startDrag(data, shadowBuilder, view, DRAG_FLAG_OPAQUE);
break;
case ACTION_MOVE:
Log.d(TAG, "ACTION_MOVE");
break;
default :
return false;
}
return true;
}
private static class MyDragShadowBuilder extends View.DragShadowBuilder {
int mTouchPointY;
int mTouchPointX;
View mView;
// The drag shadow image, defined as a drawable thing
private static Drawable shadow;
// Defines the constructor for myDragShadowBuilder
public MyDragShadowBuilder(int touchPointY, int touchPointX,View view) {
// Stores the View parameter passed to myDragShadowBuilder.
super(view);
mView = view;
mTouchPointY = touchPointY;
mTouchPointX = touchPointX;
// Creates a draggable image that will fill the Canvas provided by the system.
shadow = new ColorDrawable(Color.WHITE);
}
// Defines a callback that sends the drag shadow dimensions and touch point back to the
// system.
@Override
public void onProvideShadowMetrics (Point size, Point touch) {
// Defines local variables
int width, height;
// Sets the width of the shadow to half the width of the original View
width = getView().getWidth();// / 2;
// Sets the height of the shadow to half the height of the original View
height = getView().getHeight();// / 2;
// The drag shadow is a ColorDrawable. This sets its dimensions to be the same as the
// Canvas that the system will provide. As a result, the drag shadow will fill the
// Canvas.
shadow.setBounds(0, 0, width, height);
// Sets the size parameter's width and height values. These get back to the system
// through the size parameter.
size.set(width, height);
// Sets the touch point's position to be in the middle of the drag shadow
touch.set(mTouchPointX, mTouchPointY);
}
// Defines a callback that draws the drag shadow in a Canvas that the system constructs
// from the dimensions passed in onProvideShadowMetrics().
@Override
public void onDrawShadow(Canvas canvas) {
// Draws the ColorDrawable in the Canvas passed in from the system.
//canvas.drawARGB(0,0,0,0);
mView.draw(canvas);
//shadow.draw(canvas);
}
}
@Override
public boolean onDrag(View view, DragEvent event)
{
Log.d(TAG,"onDrag()");
final View viewToDrag = (View) event.getLocalState();
switch (event.getAction()) {
case DragEvent.ACTION_DRAG_STARTED:
Log.d(TAG,"ACTION_DRAG_STARTED");
break;
case DragEvent.ACTION_DRAG_ENTERED:
Log.d(TAG,"ACTION_DRAG_ENTERED");
break;
case DragEvent.ACTION_DRAG_EXITED:
Log.d(TAG,"ACTION_DRAG_EXITED");
break;
case DragEvent.ACTION_DROP:
Log.d(TAG,"ACTION_DROP");
break;
case DragEvent.ACTION_DRAG_ENDED:
Log.d(TAG,"ACTION_DRAG_ENDED");
viewToDrag.setAlpha(1f); //transparent thus invisible
break;
default:
Log.d(TAG,"default");
break;
}
return true;
}




asked Nov 23 '18 at 20:22


the_prolethe_prole
2,87463178
2,87463178
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452558%2fhow-to-maintain-drag-shadow-opacity-after-release-event%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452558%2fhow-to-maintain-drag-shadow-opacity-after-release-event%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9,ag8H 4b,eI0kKazFfHimd,HM9eZ,sFVEruFjZmYich wwoxrHReLYk