Show all primes in go
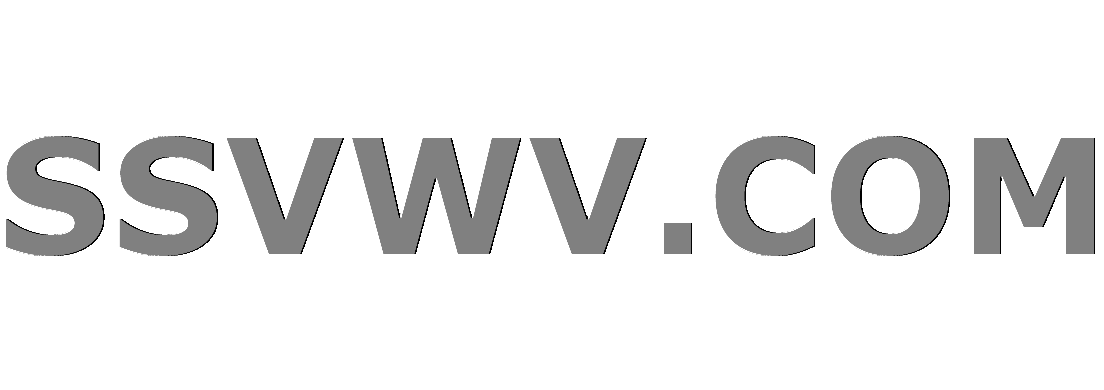
Multi tool use
I'm trying to go through Adrian simple programming tasks; Elementary task 8 It's basically asking for an infinite loop to print every prime number as it finds it.
Are there too many if
statements? Call it intuitive knowledge, but something is just bugging me, like I'm missing something. Like, I can remove one of the if
statements. So I'm wondering what you guys will find.
package main
import "fmt"
func main() {
var current_prime int
var prime bool
current_prime = 0
for {
prime = true
current_prime++
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
i = current_prime
}
}
if prime {
fmt.Println("found prime:", current_prime);
}
}
}
performance primes formatting go
add a comment |
I'm trying to go through Adrian simple programming tasks; Elementary task 8 It's basically asking for an infinite loop to print every prime number as it finds it.
Are there too many if
statements? Call it intuitive knowledge, but something is just bugging me, like I'm missing something. Like, I can remove one of the if
statements. So I'm wondering what you guys will find.
package main
import "fmt"
func main() {
var current_prime int
var prime bool
current_prime = 0
for {
prime = true
current_prime++
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
i = current_prime
}
}
if prime {
fmt.Println("found prime:", current_prime);
}
}
}
performance primes formatting go
add a comment |
I'm trying to go through Adrian simple programming tasks; Elementary task 8 It's basically asking for an infinite loop to print every prime number as it finds it.
Are there too many if
statements? Call it intuitive knowledge, but something is just bugging me, like I'm missing something. Like, I can remove one of the if
statements. So I'm wondering what you guys will find.
package main
import "fmt"
func main() {
var current_prime int
var prime bool
current_prime = 0
for {
prime = true
current_prime++
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
i = current_prime
}
}
if prime {
fmt.Println("found prime:", current_prime);
}
}
}
performance primes formatting go
I'm trying to go through Adrian simple programming tasks; Elementary task 8 It's basically asking for an infinite loop to print every prime number as it finds it.
Are there too many if
statements? Call it intuitive knowledge, but something is just bugging me, like I'm missing something. Like, I can remove one of the if
statements. So I'm wondering what you guys will find.
package main
import "fmt"
func main() {
var current_prime int
var prime bool
current_prime = 0
for {
prime = true
current_prime++
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
i = current_prime
}
}
if prime {
fmt.Println("found prime:", current_prime);
}
}
}
performance primes formatting go
performance primes formatting go
edited Jul 28 at 3:26


Jamal♦
30.2k11116226
30.2k11116226
asked Jul 27 at 14:49
Ibn Rushd
554
554
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Simple Programming Problems
Write a program that prints all prime numbers. (Note: if your
programming language does not support arbitrary size numbers, printing
all primes up to the largest number you can easily represent is fine
too.)
Your program says one is prime. That is not correct.
The only even prime number is two. You don't appear take advantage of that. That is inefficient.
A number can only be divisible by a number less than or equal to its square root. You don't appear take advantage of that. That is inefficient.
You are asked to "[print] all primes up to the largest number you can easily represent." In Go, type int is either 32 or 64 bits. You don't guarantee the largest number by using type int64. Your program is not correct.
You have no program termination condition. Your program is not correct.
And so on.
For example, fixing your code,
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println("prime numbers:")
fmt.Println(2)
for n := int64(3); n > 0; n += 2 {
prime := true
r := int64(math.Sqrt(float64(n))) + 1
for i := int64(3); i < r; i += 2 {
if n%i == 0 {
prime = false
break
}
}
if prime {
fmt.Println(n)
}
}
}
To provide a measure of performance, the results from a Go benchmark for all prime numbers up to 32,771:
BenchmarkPeterSO-4 500 2661556 ns/op
BenchmarkIbnRushd-4 3 492864429 ns/op
Minor possible performance improvement. math.Sqrt is very slow - but we know the previous sqrt
– zaTricky
Jul 31 at 7:01
add a comment |
Instead of trying to get a performance increase with a faster solution let's review your program and try to make it a solid one.
The variables
var current_prime int
var prime bool
current_prime = 0
Check this example:
var a int = 0 // 0
var b int // default int value is 0, similar to above
var c = 0 // type is int, similar to above
d := 0 // same
The latter is short and nice, I suggest you to stick to it. var
notation is required in package block (globals), when explicit type is needed and to overcome variable shading.
You've declared the prime variable, but is first used only within the outer loop, so declare it there. Try to introduce new variables first time you need them.
The outer loop
for {
// ...
}
Your for loop is infinite since there is no loop condition or a break statement. The current_prime will overflow and you'll start printing the same/wrong numbers.
As pointed by peterSO, the first prime number is 2, so we can start with it.
for current_prime := 2; i > 0; i++ {
// ...
}
When overflow occurs the loop condition will be falsy and the loop will terminate.
To take it further it is better not to expect int
overflow and use MaxInt* constants from math package. Apart from guaranteed proper uint
overflow there is nothing solid about int
, so it is better not to abuse its behavior.
The inner loop
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
i = current_prime
}
}
It took me a while to understand the purpose of i = current_prime
line. No. This is not nice, use break
to end the loop.
This one is much cleaner to me:
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
break
}
}
Let's take it further. After the inner loop you test the prime variable and print the current_prime number. So when you get current_prime % i == 0
as true you already know current_prime is not a prime number and you need to continue the outer loop. For such purpose Golang has labels that perfectly solve the task:
outer:
for prime := 2; prime > 0; prime++ {
for i := 2; i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
A continue outer
statement will break the inner loop and continue the outer one.
When you place a label one line before the loop you may break and continue right to it. It helps escaping nested loops a lot.
Finally lets choose the appropriate types for our task. Since we don't need the signed numbers we can use uint
instead of int
. Better, lets use uint64
directly to guaranty the maximum available type. The nice thing about unsigned numbers is that Golang guarantees that they will overflow properly so we can check for zero value to terminate the loop.
Example rewrite
Here follows a possible rewrite of your original program:
package main
import "fmt"
func main() {
outer:
for prime := uint64(2); prime > 0; prime++ {
for i := uint64(2); i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
}
Things accomplished:
- proper types are used
- overflow is handled
- no hacks
1
It will be helpfull if you'll provide comments explaining your downvotes.
– sineemore
Aug 11 at 12:54
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f200424%2fshow-all-primes-in-go%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Simple Programming Problems
Write a program that prints all prime numbers. (Note: if your
programming language does not support arbitrary size numbers, printing
all primes up to the largest number you can easily represent is fine
too.)
Your program says one is prime. That is not correct.
The only even prime number is two. You don't appear take advantage of that. That is inefficient.
A number can only be divisible by a number less than or equal to its square root. You don't appear take advantage of that. That is inefficient.
You are asked to "[print] all primes up to the largest number you can easily represent." In Go, type int is either 32 or 64 bits. You don't guarantee the largest number by using type int64. Your program is not correct.
You have no program termination condition. Your program is not correct.
And so on.
For example, fixing your code,
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println("prime numbers:")
fmt.Println(2)
for n := int64(3); n > 0; n += 2 {
prime := true
r := int64(math.Sqrt(float64(n))) + 1
for i := int64(3); i < r; i += 2 {
if n%i == 0 {
prime = false
break
}
}
if prime {
fmt.Println(n)
}
}
}
To provide a measure of performance, the results from a Go benchmark for all prime numbers up to 32,771:
BenchmarkPeterSO-4 500 2661556 ns/op
BenchmarkIbnRushd-4 3 492864429 ns/op
Minor possible performance improvement. math.Sqrt is very slow - but we know the previous sqrt
– zaTricky
Jul 31 at 7:01
add a comment |
Simple Programming Problems
Write a program that prints all prime numbers. (Note: if your
programming language does not support arbitrary size numbers, printing
all primes up to the largest number you can easily represent is fine
too.)
Your program says one is prime. That is not correct.
The only even prime number is two. You don't appear take advantage of that. That is inefficient.
A number can only be divisible by a number less than or equal to its square root. You don't appear take advantage of that. That is inefficient.
You are asked to "[print] all primes up to the largest number you can easily represent." In Go, type int is either 32 or 64 bits. You don't guarantee the largest number by using type int64. Your program is not correct.
You have no program termination condition. Your program is not correct.
And so on.
For example, fixing your code,
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println("prime numbers:")
fmt.Println(2)
for n := int64(3); n > 0; n += 2 {
prime := true
r := int64(math.Sqrt(float64(n))) + 1
for i := int64(3); i < r; i += 2 {
if n%i == 0 {
prime = false
break
}
}
if prime {
fmt.Println(n)
}
}
}
To provide a measure of performance, the results from a Go benchmark for all prime numbers up to 32,771:
BenchmarkPeterSO-4 500 2661556 ns/op
BenchmarkIbnRushd-4 3 492864429 ns/op
Minor possible performance improvement. math.Sqrt is very slow - but we know the previous sqrt
– zaTricky
Jul 31 at 7:01
add a comment |
Simple Programming Problems
Write a program that prints all prime numbers. (Note: if your
programming language does not support arbitrary size numbers, printing
all primes up to the largest number you can easily represent is fine
too.)
Your program says one is prime. That is not correct.
The only even prime number is two. You don't appear take advantage of that. That is inefficient.
A number can only be divisible by a number less than or equal to its square root. You don't appear take advantage of that. That is inefficient.
You are asked to "[print] all primes up to the largest number you can easily represent." In Go, type int is either 32 or 64 bits. You don't guarantee the largest number by using type int64. Your program is not correct.
You have no program termination condition. Your program is not correct.
And so on.
For example, fixing your code,
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println("prime numbers:")
fmt.Println(2)
for n := int64(3); n > 0; n += 2 {
prime := true
r := int64(math.Sqrt(float64(n))) + 1
for i := int64(3); i < r; i += 2 {
if n%i == 0 {
prime = false
break
}
}
if prime {
fmt.Println(n)
}
}
}
To provide a measure of performance, the results from a Go benchmark for all prime numbers up to 32,771:
BenchmarkPeterSO-4 500 2661556 ns/op
BenchmarkIbnRushd-4 3 492864429 ns/op
Simple Programming Problems
Write a program that prints all prime numbers. (Note: if your
programming language does not support arbitrary size numbers, printing
all primes up to the largest number you can easily represent is fine
too.)
Your program says one is prime. That is not correct.
The only even prime number is two. You don't appear take advantage of that. That is inefficient.
A number can only be divisible by a number less than or equal to its square root. You don't appear take advantage of that. That is inefficient.
You are asked to "[print] all primes up to the largest number you can easily represent." In Go, type int is either 32 or 64 bits. You don't guarantee the largest number by using type int64. Your program is not correct.
You have no program termination condition. Your program is not correct.
And so on.
For example, fixing your code,
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println("prime numbers:")
fmt.Println(2)
for n := int64(3); n > 0; n += 2 {
prime := true
r := int64(math.Sqrt(float64(n))) + 1
for i := int64(3); i < r; i += 2 {
if n%i == 0 {
prime = false
break
}
}
if prime {
fmt.Println(n)
}
}
}
To provide a measure of performance, the results from a Go benchmark for all prime numbers up to 32,771:
BenchmarkPeterSO-4 500 2661556 ns/op
BenchmarkIbnRushd-4 3 492864429 ns/op
edited Jul 28 at 3:20
answered Jul 27 at 15:41
peterSO
1,18958
1,18958
Minor possible performance improvement. math.Sqrt is very slow - but we know the previous sqrt
– zaTricky
Jul 31 at 7:01
add a comment |
Minor possible performance improvement. math.Sqrt is very slow - but we know the previous sqrt
– zaTricky
Jul 31 at 7:01
Minor possible performance improvement. math.Sqrt is very slow - but we know the previous sqrt
– zaTricky
Jul 31 at 7:01
Minor possible performance improvement. math.Sqrt is very slow - but we know the previous sqrt
– zaTricky
Jul 31 at 7:01
add a comment |
Instead of trying to get a performance increase with a faster solution let's review your program and try to make it a solid one.
The variables
var current_prime int
var prime bool
current_prime = 0
Check this example:
var a int = 0 // 0
var b int // default int value is 0, similar to above
var c = 0 // type is int, similar to above
d := 0 // same
The latter is short and nice, I suggest you to stick to it. var
notation is required in package block (globals), when explicit type is needed and to overcome variable shading.
You've declared the prime variable, but is first used only within the outer loop, so declare it there. Try to introduce new variables first time you need them.
The outer loop
for {
// ...
}
Your for loop is infinite since there is no loop condition or a break statement. The current_prime will overflow and you'll start printing the same/wrong numbers.
As pointed by peterSO, the first prime number is 2, so we can start with it.
for current_prime := 2; i > 0; i++ {
// ...
}
When overflow occurs the loop condition will be falsy and the loop will terminate.
To take it further it is better not to expect int
overflow and use MaxInt* constants from math package. Apart from guaranteed proper uint
overflow there is nothing solid about int
, so it is better not to abuse its behavior.
The inner loop
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
i = current_prime
}
}
It took me a while to understand the purpose of i = current_prime
line. No. This is not nice, use break
to end the loop.
This one is much cleaner to me:
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
break
}
}
Let's take it further. After the inner loop you test the prime variable and print the current_prime number. So when you get current_prime % i == 0
as true you already know current_prime is not a prime number and you need to continue the outer loop. For such purpose Golang has labels that perfectly solve the task:
outer:
for prime := 2; prime > 0; prime++ {
for i := 2; i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
A continue outer
statement will break the inner loop and continue the outer one.
When you place a label one line before the loop you may break and continue right to it. It helps escaping nested loops a lot.
Finally lets choose the appropriate types for our task. Since we don't need the signed numbers we can use uint
instead of int
. Better, lets use uint64
directly to guaranty the maximum available type. The nice thing about unsigned numbers is that Golang guarantees that they will overflow properly so we can check for zero value to terminate the loop.
Example rewrite
Here follows a possible rewrite of your original program:
package main
import "fmt"
func main() {
outer:
for prime := uint64(2); prime > 0; prime++ {
for i := uint64(2); i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
}
Things accomplished:
- proper types are used
- overflow is handled
- no hacks
1
It will be helpfull if you'll provide comments explaining your downvotes.
– sineemore
Aug 11 at 12:54
add a comment |
Instead of trying to get a performance increase with a faster solution let's review your program and try to make it a solid one.
The variables
var current_prime int
var prime bool
current_prime = 0
Check this example:
var a int = 0 // 0
var b int // default int value is 0, similar to above
var c = 0 // type is int, similar to above
d := 0 // same
The latter is short and nice, I suggest you to stick to it. var
notation is required in package block (globals), when explicit type is needed and to overcome variable shading.
You've declared the prime variable, but is first used only within the outer loop, so declare it there. Try to introduce new variables first time you need them.
The outer loop
for {
// ...
}
Your for loop is infinite since there is no loop condition or a break statement. The current_prime will overflow and you'll start printing the same/wrong numbers.
As pointed by peterSO, the first prime number is 2, so we can start with it.
for current_prime := 2; i > 0; i++ {
// ...
}
When overflow occurs the loop condition will be falsy and the loop will terminate.
To take it further it is better not to expect int
overflow and use MaxInt* constants from math package. Apart from guaranteed proper uint
overflow there is nothing solid about int
, so it is better not to abuse its behavior.
The inner loop
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
i = current_prime
}
}
It took me a while to understand the purpose of i = current_prime
line. No. This is not nice, use break
to end the loop.
This one is much cleaner to me:
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
break
}
}
Let's take it further. After the inner loop you test the prime variable and print the current_prime number. So when you get current_prime % i == 0
as true you already know current_prime is not a prime number and you need to continue the outer loop. For such purpose Golang has labels that perfectly solve the task:
outer:
for prime := 2; prime > 0; prime++ {
for i := 2; i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
A continue outer
statement will break the inner loop and continue the outer one.
When you place a label one line before the loop you may break and continue right to it. It helps escaping nested loops a lot.
Finally lets choose the appropriate types for our task. Since we don't need the signed numbers we can use uint
instead of int
. Better, lets use uint64
directly to guaranty the maximum available type. The nice thing about unsigned numbers is that Golang guarantees that they will overflow properly so we can check for zero value to terminate the loop.
Example rewrite
Here follows a possible rewrite of your original program:
package main
import "fmt"
func main() {
outer:
for prime := uint64(2); prime > 0; prime++ {
for i := uint64(2); i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
}
Things accomplished:
- proper types are used
- overflow is handled
- no hacks
1
It will be helpfull if you'll provide comments explaining your downvotes.
– sineemore
Aug 11 at 12:54
add a comment |
Instead of trying to get a performance increase with a faster solution let's review your program and try to make it a solid one.
The variables
var current_prime int
var prime bool
current_prime = 0
Check this example:
var a int = 0 // 0
var b int // default int value is 0, similar to above
var c = 0 // type is int, similar to above
d := 0 // same
The latter is short and nice, I suggest you to stick to it. var
notation is required in package block (globals), when explicit type is needed and to overcome variable shading.
You've declared the prime variable, but is first used only within the outer loop, so declare it there. Try to introduce new variables first time you need them.
The outer loop
for {
// ...
}
Your for loop is infinite since there is no loop condition or a break statement. The current_prime will overflow and you'll start printing the same/wrong numbers.
As pointed by peterSO, the first prime number is 2, so we can start with it.
for current_prime := 2; i > 0; i++ {
// ...
}
When overflow occurs the loop condition will be falsy and the loop will terminate.
To take it further it is better not to expect int
overflow and use MaxInt* constants from math package. Apart from guaranteed proper uint
overflow there is nothing solid about int
, so it is better not to abuse its behavior.
The inner loop
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
i = current_prime
}
}
It took me a while to understand the purpose of i = current_prime
line. No. This is not nice, use break
to end the loop.
This one is much cleaner to me:
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
break
}
}
Let's take it further. After the inner loop you test the prime variable and print the current_prime number. So when you get current_prime % i == 0
as true you already know current_prime is not a prime number and you need to continue the outer loop. For such purpose Golang has labels that perfectly solve the task:
outer:
for prime := 2; prime > 0; prime++ {
for i := 2; i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
A continue outer
statement will break the inner loop and continue the outer one.
When you place a label one line before the loop you may break and continue right to it. It helps escaping nested loops a lot.
Finally lets choose the appropriate types for our task. Since we don't need the signed numbers we can use uint
instead of int
. Better, lets use uint64
directly to guaranty the maximum available type. The nice thing about unsigned numbers is that Golang guarantees that they will overflow properly so we can check for zero value to terminate the loop.
Example rewrite
Here follows a possible rewrite of your original program:
package main
import "fmt"
func main() {
outer:
for prime := uint64(2); prime > 0; prime++ {
for i := uint64(2); i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
}
Things accomplished:
- proper types are used
- overflow is handled
- no hacks
Instead of trying to get a performance increase with a faster solution let's review your program and try to make it a solid one.
The variables
var current_prime int
var prime bool
current_prime = 0
Check this example:
var a int = 0 // 0
var b int // default int value is 0, similar to above
var c = 0 // type is int, similar to above
d := 0 // same
The latter is short and nice, I suggest you to stick to it. var
notation is required in package block (globals), when explicit type is needed and to overcome variable shading.
You've declared the prime variable, but is first used only within the outer loop, so declare it there. Try to introduce new variables first time you need them.
The outer loop
for {
// ...
}
Your for loop is infinite since there is no loop condition or a break statement. The current_prime will overflow and you'll start printing the same/wrong numbers.
As pointed by peterSO, the first prime number is 2, so we can start with it.
for current_prime := 2; i > 0; i++ {
// ...
}
When overflow occurs the loop condition will be falsy and the loop will terminate.
To take it further it is better not to expect int
overflow and use MaxInt* constants from math package. Apart from guaranteed proper uint
overflow there is nothing solid about int
, so it is better not to abuse its behavior.
The inner loop
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
i = current_prime
}
}
It took me a while to understand the purpose of i = current_prime
line. No. This is not nice, use break
to end the loop.
This one is much cleaner to me:
for i := 2; i < current_prime; i++ {
if current_prime % i == 0 {
prime = false
break
}
}
Let's take it further. After the inner loop you test the prime variable and print the current_prime number. So when you get current_prime % i == 0
as true you already know current_prime is not a prime number and you need to continue the outer loop. For such purpose Golang has labels that perfectly solve the task:
outer:
for prime := 2; prime > 0; prime++ {
for i := 2; i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
A continue outer
statement will break the inner loop and continue the outer one.
When you place a label one line before the loop you may break and continue right to it. It helps escaping nested loops a lot.
Finally lets choose the appropriate types for our task. Since we don't need the signed numbers we can use uint
instead of int
. Better, lets use uint64
directly to guaranty the maximum available type. The nice thing about unsigned numbers is that Golang guarantees that they will overflow properly so we can check for zero value to terminate the loop.
Example rewrite
Here follows a possible rewrite of your original program:
package main
import "fmt"
func main() {
outer:
for prime := uint64(2); prime > 0; prime++ {
for i := uint64(2); i < prime; i++ {
if prime % i == 0 {
continue outer
}
}
fmt.Println(prime)
}
}
Things accomplished:
- proper types are used
- overflow is handled
- no hacks
edited 4 mins ago
answered Aug 4 at 15:32


sineemore
1,548525
1,548525
1
It will be helpfull if you'll provide comments explaining your downvotes.
– sineemore
Aug 11 at 12:54
add a comment |
1
It will be helpfull if you'll provide comments explaining your downvotes.
– sineemore
Aug 11 at 12:54
1
1
It will be helpfull if you'll provide comments explaining your downvotes.
– sineemore
Aug 11 at 12:54
It will be helpfull if you'll provide comments explaining your downvotes.
– sineemore
Aug 11 at 12:54
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f200424%2fshow-all-primes-in-go%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
I0wfQKdI0nNK5e,jBMBZ XZpOKt U2KIfX7hT 0qRfDtVKjW,lU4KGQFq0KHIMZbGPbv04r,ZM8sJoFIZJqggoWrMgQRJkB