Javascript: How can I make Synchronous Loop?
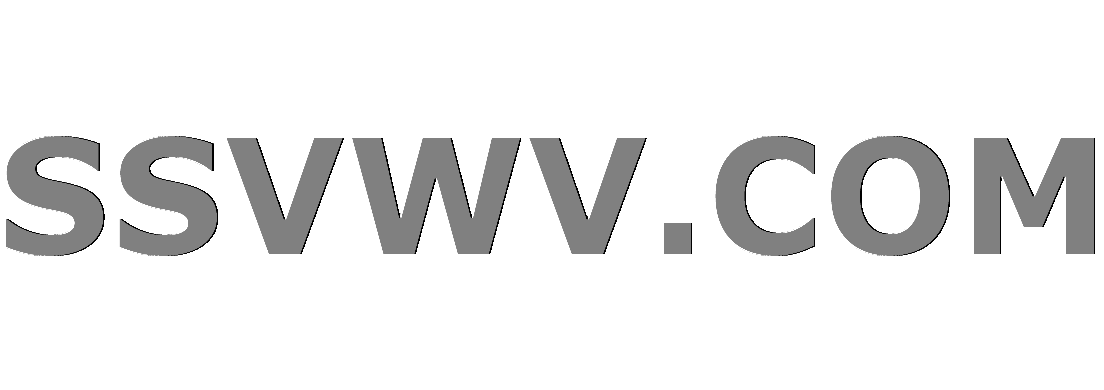
Multi tool use
I'm confused by Synchronous/Asynchronous processing of JavaScript.
What I want to do is below. When self_driving()
is called, then get_direction_by_sensor()
is called and using the direction, moter starts running by move_to_direction(direction)
. This process repeats 5 times.
function get_direction_by_sensor(){
// code for getting direction from sensor
return direction
};
function move_to_direction(direction){
direction = get_direction_by_sensor()
// code for driving motor to the direction
};
function self_driving_loop(maxCount, i) {
if (i <= maxCount) {
move_to_direction();
setTimeout(function(){
self_driving_loop(maxCount, ++i)
}, 1000);
}
};
function self_driving() {
self_driving_loop(5, 1)
};
So I want this code to run like this.
1. get_direction_by_sensor()
1. move_to_direction()
2. get_direction_by_sensor()
2. move_to_direction()
3. get_direction_by_sensor()
3. move_to_direction()
4. get_direction_by_sensor()
4. move_to_direction()
5. get_direction_by_sensor()
5. move_to_direction()
But actually it runs like this.
1. get_direction_by_sensor()
2. get_direction_by_sensor()
3. get_direction_by_sensor()
4. get_direction_by_sensor()
5. get_direction_by_sensor() // this direction is used for moving
5. move_to_direction()
How can I fix this code?
Thanks.
======== MORE DETAILED INFO ========
move_to_direction()
calles Macro of webiopi written by Python.
function move_to_direction() {
w().callMacro('get_direction_to_move', [TRIG_F ,ECHO_F ,TRIG_R ,ECHO_R ,TRIG_L ,ECHO_L ,TRIG_B ,ECHO_B], function(macro, args, resp) {
console.log(resp) // DEBUG
if(resp == "forward") {
change_direction('FOWARD');
} else if(resp == "right") {
change_direction('RIGHT');
} else if(resp == "left") {
change_direction('LEFT');
} else if(resp == "backward") {
change_direction('BACKWARD');
}
});
}
javascript loops synchronization
add a comment |
I'm confused by Synchronous/Asynchronous processing of JavaScript.
What I want to do is below. When self_driving()
is called, then get_direction_by_sensor()
is called and using the direction, moter starts running by move_to_direction(direction)
. This process repeats 5 times.
function get_direction_by_sensor(){
// code for getting direction from sensor
return direction
};
function move_to_direction(direction){
direction = get_direction_by_sensor()
// code for driving motor to the direction
};
function self_driving_loop(maxCount, i) {
if (i <= maxCount) {
move_to_direction();
setTimeout(function(){
self_driving_loop(maxCount, ++i)
}, 1000);
}
};
function self_driving() {
self_driving_loop(5, 1)
};
So I want this code to run like this.
1. get_direction_by_sensor()
1. move_to_direction()
2. get_direction_by_sensor()
2. move_to_direction()
3. get_direction_by_sensor()
3. move_to_direction()
4. get_direction_by_sensor()
4. move_to_direction()
5. get_direction_by_sensor()
5. move_to_direction()
But actually it runs like this.
1. get_direction_by_sensor()
2. get_direction_by_sensor()
3. get_direction_by_sensor()
4. get_direction_by_sensor()
5. get_direction_by_sensor() // this direction is used for moving
5. move_to_direction()
How can I fix this code?
Thanks.
======== MORE DETAILED INFO ========
move_to_direction()
calles Macro of webiopi written by Python.
function move_to_direction() {
w().callMacro('get_direction_to_move', [TRIG_F ,ECHO_F ,TRIG_R ,ECHO_R ,TRIG_L ,ECHO_L ,TRIG_B ,ECHO_B], function(macro, args, resp) {
console.log(resp) // DEBUG
if(resp == "forward") {
change_direction('FOWARD');
} else if(resp == "right") {
change_direction('RIGHT');
} else if(resp == "left") {
change_direction('LEFT');
} else if(resp == "backward") {
change_direction('BACKWARD');
}
});
}
javascript loops synchronization
I guess you'll need to show a little more about those two functions - must have asynchrony in them, right?
– Bravo
Nov 21 at 3:28
the code is doing what you want repl.it/repls/CostlyBonyApplets
– Pablo Fernandez
Nov 21 at 3:29
@Bravo Yeah, some asynchrony inmove_to_direction
function. What I want to do is finish all the (sub) functions inmove_to_direction
and go to nextmove_to_direction
.
– Hiromu Masuda
Nov 21 at 3:36
add a comment |
I'm confused by Synchronous/Asynchronous processing of JavaScript.
What I want to do is below. When self_driving()
is called, then get_direction_by_sensor()
is called and using the direction, moter starts running by move_to_direction(direction)
. This process repeats 5 times.
function get_direction_by_sensor(){
// code for getting direction from sensor
return direction
};
function move_to_direction(direction){
direction = get_direction_by_sensor()
// code for driving motor to the direction
};
function self_driving_loop(maxCount, i) {
if (i <= maxCount) {
move_to_direction();
setTimeout(function(){
self_driving_loop(maxCount, ++i)
}, 1000);
}
};
function self_driving() {
self_driving_loop(5, 1)
};
So I want this code to run like this.
1. get_direction_by_sensor()
1. move_to_direction()
2. get_direction_by_sensor()
2. move_to_direction()
3. get_direction_by_sensor()
3. move_to_direction()
4. get_direction_by_sensor()
4. move_to_direction()
5. get_direction_by_sensor()
5. move_to_direction()
But actually it runs like this.
1. get_direction_by_sensor()
2. get_direction_by_sensor()
3. get_direction_by_sensor()
4. get_direction_by_sensor()
5. get_direction_by_sensor() // this direction is used for moving
5. move_to_direction()
How can I fix this code?
Thanks.
======== MORE DETAILED INFO ========
move_to_direction()
calles Macro of webiopi written by Python.
function move_to_direction() {
w().callMacro('get_direction_to_move', [TRIG_F ,ECHO_F ,TRIG_R ,ECHO_R ,TRIG_L ,ECHO_L ,TRIG_B ,ECHO_B], function(macro, args, resp) {
console.log(resp) // DEBUG
if(resp == "forward") {
change_direction('FOWARD');
} else if(resp == "right") {
change_direction('RIGHT');
} else if(resp == "left") {
change_direction('LEFT');
} else if(resp == "backward") {
change_direction('BACKWARD');
}
});
}
javascript loops synchronization
I'm confused by Synchronous/Asynchronous processing of JavaScript.
What I want to do is below. When self_driving()
is called, then get_direction_by_sensor()
is called and using the direction, moter starts running by move_to_direction(direction)
. This process repeats 5 times.
function get_direction_by_sensor(){
// code for getting direction from sensor
return direction
};
function move_to_direction(direction){
direction = get_direction_by_sensor()
// code for driving motor to the direction
};
function self_driving_loop(maxCount, i) {
if (i <= maxCount) {
move_to_direction();
setTimeout(function(){
self_driving_loop(maxCount, ++i)
}, 1000);
}
};
function self_driving() {
self_driving_loop(5, 1)
};
So I want this code to run like this.
1. get_direction_by_sensor()
1. move_to_direction()
2. get_direction_by_sensor()
2. move_to_direction()
3. get_direction_by_sensor()
3. move_to_direction()
4. get_direction_by_sensor()
4. move_to_direction()
5. get_direction_by_sensor()
5. move_to_direction()
But actually it runs like this.
1. get_direction_by_sensor()
2. get_direction_by_sensor()
3. get_direction_by_sensor()
4. get_direction_by_sensor()
5. get_direction_by_sensor() // this direction is used for moving
5. move_to_direction()
How can I fix this code?
Thanks.
======== MORE DETAILED INFO ========
move_to_direction()
calles Macro of webiopi written by Python.
function move_to_direction() {
w().callMacro('get_direction_to_move', [TRIG_F ,ECHO_F ,TRIG_R ,ECHO_R ,TRIG_L ,ECHO_L ,TRIG_B ,ECHO_B], function(macro, args, resp) {
console.log(resp) // DEBUG
if(resp == "forward") {
change_direction('FOWARD');
} else if(resp == "right") {
change_direction('RIGHT');
} else if(resp == "left") {
change_direction('LEFT');
} else if(resp == "backward") {
change_direction('BACKWARD');
}
});
}
javascript loops synchronization
javascript loops synchronization
edited Nov 21 at 3:34
asked Nov 21 at 3:22
Hiromu Masuda
719
719
I guess you'll need to show a little more about those two functions - must have asynchrony in them, right?
– Bravo
Nov 21 at 3:28
the code is doing what you want repl.it/repls/CostlyBonyApplets
– Pablo Fernandez
Nov 21 at 3:29
@Bravo Yeah, some asynchrony inmove_to_direction
function. What I want to do is finish all the (sub) functions inmove_to_direction
and go to nextmove_to_direction
.
– Hiromu Masuda
Nov 21 at 3:36
add a comment |
I guess you'll need to show a little more about those two functions - must have asynchrony in them, right?
– Bravo
Nov 21 at 3:28
the code is doing what you want repl.it/repls/CostlyBonyApplets
– Pablo Fernandez
Nov 21 at 3:29
@Bravo Yeah, some asynchrony inmove_to_direction
function. What I want to do is finish all the (sub) functions inmove_to_direction
and go to nextmove_to_direction
.
– Hiromu Masuda
Nov 21 at 3:36
I guess you'll need to show a little more about those two functions - must have asynchrony in them, right?
– Bravo
Nov 21 at 3:28
I guess you'll need to show a little more about those two functions - must have asynchrony in them, right?
– Bravo
Nov 21 at 3:28
the code is doing what you want repl.it/repls/CostlyBonyApplets
– Pablo Fernandez
Nov 21 at 3:29
the code is doing what you want repl.it/repls/CostlyBonyApplets
– Pablo Fernandez
Nov 21 at 3:29
@Bravo Yeah, some asynchrony in
move_to_direction
function. What I want to do is finish all the (sub) functions in move_to_direction
and go to next move_to_direction
.– Hiromu Masuda
Nov 21 at 3:36
@Bravo Yeah, some asynchrony in
move_to_direction
function. What I want to do is finish all the (sub) functions in move_to_direction
and go to next move_to_direction
.– Hiromu Masuda
Nov 21 at 3:36
add a comment |
1 Answer
1
active
oldest
votes
settimeout must be wrapped with a promise so that it can be awaited. see
function self_driving_loop(maxCount, i) {
return new Promise(resolve => {
if (i <= maxCount) {
move_to_direction();
setTimeout(function(){
self_driving_loop(maxCount, ++i)
resolve()
}, 1000);
}
})
};
call it this way in an async function
await self_driving_loop(maxCount, i)
Are you sure it works? it looks wrong
– Bravo
Nov 21 at 3:46
did work:self_driving_loop(maxCount, i)
, didnt work:await self_driving_loop(maxCount, i)
– Hiromu Masuda
Nov 21 at 7:14
it will work for async function please see developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– EJL
Nov 22 at 5:00
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404819%2fjavascript-how-can-i-make-synchronous-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
settimeout must be wrapped with a promise so that it can be awaited. see
function self_driving_loop(maxCount, i) {
return new Promise(resolve => {
if (i <= maxCount) {
move_to_direction();
setTimeout(function(){
self_driving_loop(maxCount, ++i)
resolve()
}, 1000);
}
})
};
call it this way in an async function
await self_driving_loop(maxCount, i)
Are you sure it works? it looks wrong
– Bravo
Nov 21 at 3:46
did work:self_driving_loop(maxCount, i)
, didnt work:await self_driving_loop(maxCount, i)
– Hiromu Masuda
Nov 21 at 7:14
it will work for async function please see developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– EJL
Nov 22 at 5:00
add a comment |
settimeout must be wrapped with a promise so that it can be awaited. see
function self_driving_loop(maxCount, i) {
return new Promise(resolve => {
if (i <= maxCount) {
move_to_direction();
setTimeout(function(){
self_driving_loop(maxCount, ++i)
resolve()
}, 1000);
}
})
};
call it this way in an async function
await self_driving_loop(maxCount, i)
Are you sure it works? it looks wrong
– Bravo
Nov 21 at 3:46
did work:self_driving_loop(maxCount, i)
, didnt work:await self_driving_loop(maxCount, i)
– Hiromu Masuda
Nov 21 at 7:14
it will work for async function please see developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– EJL
Nov 22 at 5:00
add a comment |
settimeout must be wrapped with a promise so that it can be awaited. see
function self_driving_loop(maxCount, i) {
return new Promise(resolve => {
if (i <= maxCount) {
move_to_direction();
setTimeout(function(){
self_driving_loop(maxCount, ++i)
resolve()
}, 1000);
}
})
};
call it this way in an async function
await self_driving_loop(maxCount, i)
settimeout must be wrapped with a promise so that it can be awaited. see
function self_driving_loop(maxCount, i) {
return new Promise(resolve => {
if (i <= maxCount) {
move_to_direction();
setTimeout(function(){
self_driving_loop(maxCount, ++i)
resolve()
}, 1000);
}
})
};
call it this way in an async function
await self_driving_loop(maxCount, i)
answered Nov 21 at 3:35


EJL
615
615
Are you sure it works? it looks wrong
– Bravo
Nov 21 at 3:46
did work:self_driving_loop(maxCount, i)
, didnt work:await self_driving_loop(maxCount, i)
– Hiromu Masuda
Nov 21 at 7:14
it will work for async function please see developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– EJL
Nov 22 at 5:00
add a comment |
Are you sure it works? it looks wrong
– Bravo
Nov 21 at 3:46
did work:self_driving_loop(maxCount, i)
, didnt work:await self_driving_loop(maxCount, i)
– Hiromu Masuda
Nov 21 at 7:14
it will work for async function please see developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– EJL
Nov 22 at 5:00
Are you sure it works? it looks wrong
– Bravo
Nov 21 at 3:46
Are you sure it works? it looks wrong
– Bravo
Nov 21 at 3:46
did work:
self_driving_loop(maxCount, i)
, didnt work: await self_driving_loop(maxCount, i)
– Hiromu Masuda
Nov 21 at 7:14
did work:
self_driving_loop(maxCount, i)
, didnt work: await self_driving_loop(maxCount, i)
– Hiromu Masuda
Nov 21 at 7:14
it will work for async function please see developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– EJL
Nov 22 at 5:00
it will work for async function please see developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– EJL
Nov 22 at 5:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404819%2fjavascript-how-can-i-make-synchronous-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9lv8bIC59ffj3ccBkkH
I guess you'll need to show a little more about those two functions - must have asynchrony in them, right?
– Bravo
Nov 21 at 3:28
the code is doing what you want repl.it/repls/CostlyBonyApplets
– Pablo Fernandez
Nov 21 at 3:29
@Bravo Yeah, some asynchrony in
move_to_direction
function. What I want to do is finish all the (sub) functions inmove_to_direction
and go to nextmove_to_direction
.– Hiromu Masuda
Nov 21 at 3:36