Comparing Elements within a Multi-Dimensional Array
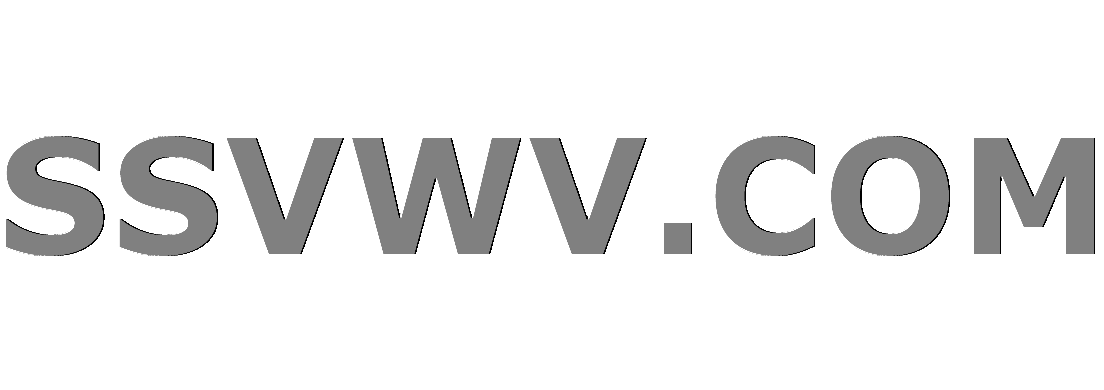
Multi tool use
Assume I have an array of the dimensions comb(x, y)
.
For each element x I need to check if any of the elements y are identical. If so, I will proceed with the next element of x.
If there were 4 elements stored in y for each x the code I would use would be something like this:
For i = 0 To z
j = 0
k = j + 1
l = j + 2
m = j + 3
If comb(i, j) <> comb(i, k) And _
comb(i, j) <> comb(i, l) And _
comb(i, j) <> comb(i, m) And _
comb(i, k) <> comb(i, l) And _
comb(i, k) <> comb(i, m) And _
comb(i, l) <> comb(i, m) Then
MsgBox "success"
End If
Next i
The thing is, that the dimension of y changes depending on the user input.
Is there a way to automate it for an arbitrary number of elements in y?
excel vba
add a comment |
Assume I have an array of the dimensions comb(x, y)
.
For each element x I need to check if any of the elements y are identical. If so, I will proceed with the next element of x.
If there were 4 elements stored in y for each x the code I would use would be something like this:
For i = 0 To z
j = 0
k = j + 1
l = j + 2
m = j + 3
If comb(i, j) <> comb(i, k) And _
comb(i, j) <> comb(i, l) And _
comb(i, j) <> comb(i, m) And _
comb(i, k) <> comb(i, l) And _
comb(i, k) <> comb(i, m) And _
comb(i, l) <> comb(i, m) Then
MsgBox "success"
End If
Next i
The thing is, that the dimension of y changes depending on the user input.
Is there a way to automate it for an arbitrary number of elements in y?
excel vba
Does ubound(comb(2)) not work?
– PGCodeRider
Nov 21 at 7:45
add a comment |
Assume I have an array of the dimensions comb(x, y)
.
For each element x I need to check if any of the elements y are identical. If so, I will proceed with the next element of x.
If there were 4 elements stored in y for each x the code I would use would be something like this:
For i = 0 To z
j = 0
k = j + 1
l = j + 2
m = j + 3
If comb(i, j) <> comb(i, k) And _
comb(i, j) <> comb(i, l) And _
comb(i, j) <> comb(i, m) And _
comb(i, k) <> comb(i, l) And _
comb(i, k) <> comb(i, m) And _
comb(i, l) <> comb(i, m) Then
MsgBox "success"
End If
Next i
The thing is, that the dimension of y changes depending on the user input.
Is there a way to automate it for an arbitrary number of elements in y?
excel vba
Assume I have an array of the dimensions comb(x, y)
.
For each element x I need to check if any of the elements y are identical. If so, I will proceed with the next element of x.
If there were 4 elements stored in y for each x the code I would use would be something like this:
For i = 0 To z
j = 0
k = j + 1
l = j + 2
m = j + 3
If comb(i, j) <> comb(i, k) And _
comb(i, j) <> comb(i, l) And _
comb(i, j) <> comb(i, m) And _
comb(i, k) <> comb(i, l) And _
comb(i, k) <> comb(i, m) And _
comb(i, l) <> comb(i, m) Then
MsgBox "success"
End If
Next i
The thing is, that the dimension of y changes depending on the user input.
Is there a way to automate it for an arbitrary number of elements in y?
excel vba
excel vba
edited Nov 21 at 8:17


K.Dᴀᴠɪs
6,963112139
6,963112139
asked Nov 21 at 7:35
c0k3b0y
112
112
Does ubound(comb(2)) not work?
– PGCodeRider
Nov 21 at 7:45
add a comment |
Does ubound(comb(2)) not work?
– PGCodeRider
Nov 21 at 7:45
Does ubound(comb(2)) not work?
– PGCodeRider
Nov 21 at 7:45
Does ubound(comb(2)) not work?
– PGCodeRider
Nov 21 at 7:45
add a comment |
2 Answers
2
active
oldest
votes
You can create three loops that will check each value, but will skip over each other due to the final loop being y + 1
from the second loop.
Dim x As Long, y As Long, z As Long, comb()
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
msgbox "Match Occurred!"
End If
Next z
Next y
Next x
Essentially, the 2nd loop controls the left side of the equation (the If comb(x, y) =
and the 3rd loop controls the right side (the = comb(x, z)
).
If desired, to prevent errors where there only may be a single value in the 2nd dimension, you could add an extra If..Then statement, like:
If Lbound(comb, 2) <> Ubound(comb, 2) Then ...
due to the nature of how the third loop adds 1 to y
's value, but an error would be raised if there isn't a 1 to add to y
.
Visualization
Here is a good visualization to see how this works. This test will print each iteration to the immediate window, you can see that each number is compared with a different number:
Sub test()
Dim x As Long, y As Long, z As Long, comb(0, 0 To 4)
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
Debug.Print y & "|" & z
End If
Next z
Next y
Next x
End Sub
Which the immediate window prints:
0|1
0|2
0|3
0|4
1|2
1|3
1|4
2|3
2|4
3|4
Thanks for the quick reply! This is headed in the right direction, but lacks a key element. Think of the elements in the 2nd dimension as a set. I want to filter for those sets where there occurs not a single element twice. Assume again the dimension is 4 and I have these elements: comb(0,0)="a" ;comb(0,1)="b"; comb(0,2)="a"; comb(0,3)="b" with your method I would get 2 matches because element 0=2 and element 1=3
– c0k3b0y
Nov 21 at 8:43
add a comment |
With K.Davis' great help I found the following solution to my problem:
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
noMatch = True
End If
Next z
Next y
If noMatch = False Then
MsgBox x
End If
noMatch = False
Next x
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53407228%2fcomparing-elements-within-a-multi-dimensional-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can create three loops that will check each value, but will skip over each other due to the final loop being y + 1
from the second loop.
Dim x As Long, y As Long, z As Long, comb()
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
msgbox "Match Occurred!"
End If
Next z
Next y
Next x
Essentially, the 2nd loop controls the left side of the equation (the If comb(x, y) =
and the 3rd loop controls the right side (the = comb(x, z)
).
If desired, to prevent errors where there only may be a single value in the 2nd dimension, you could add an extra If..Then statement, like:
If Lbound(comb, 2) <> Ubound(comb, 2) Then ...
due to the nature of how the third loop adds 1 to y
's value, but an error would be raised if there isn't a 1 to add to y
.
Visualization
Here is a good visualization to see how this works. This test will print each iteration to the immediate window, you can see that each number is compared with a different number:
Sub test()
Dim x As Long, y As Long, z As Long, comb(0, 0 To 4)
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
Debug.Print y & "|" & z
End If
Next z
Next y
Next x
End Sub
Which the immediate window prints:
0|1
0|2
0|3
0|4
1|2
1|3
1|4
2|3
2|4
3|4
Thanks for the quick reply! This is headed in the right direction, but lacks a key element. Think of the elements in the 2nd dimension as a set. I want to filter for those sets where there occurs not a single element twice. Assume again the dimension is 4 and I have these elements: comb(0,0)="a" ;comb(0,1)="b"; comb(0,2)="a"; comb(0,3)="b" with your method I would get 2 matches because element 0=2 and element 1=3
– c0k3b0y
Nov 21 at 8:43
add a comment |
You can create three loops that will check each value, but will skip over each other due to the final loop being y + 1
from the second loop.
Dim x As Long, y As Long, z As Long, comb()
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
msgbox "Match Occurred!"
End If
Next z
Next y
Next x
Essentially, the 2nd loop controls the left side of the equation (the If comb(x, y) =
and the 3rd loop controls the right side (the = comb(x, z)
).
If desired, to prevent errors where there only may be a single value in the 2nd dimension, you could add an extra If..Then statement, like:
If Lbound(comb, 2) <> Ubound(comb, 2) Then ...
due to the nature of how the third loop adds 1 to y
's value, but an error would be raised if there isn't a 1 to add to y
.
Visualization
Here is a good visualization to see how this works. This test will print each iteration to the immediate window, you can see that each number is compared with a different number:
Sub test()
Dim x As Long, y As Long, z As Long, comb(0, 0 To 4)
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
Debug.Print y & "|" & z
End If
Next z
Next y
Next x
End Sub
Which the immediate window prints:
0|1
0|2
0|3
0|4
1|2
1|3
1|4
2|3
2|4
3|4
Thanks for the quick reply! This is headed in the right direction, but lacks a key element. Think of the elements in the 2nd dimension as a set. I want to filter for those sets where there occurs not a single element twice. Assume again the dimension is 4 and I have these elements: comb(0,0)="a" ;comb(0,1)="b"; comb(0,2)="a"; comb(0,3)="b" with your method I would get 2 matches because element 0=2 and element 1=3
– c0k3b0y
Nov 21 at 8:43
add a comment |
You can create three loops that will check each value, but will skip over each other due to the final loop being y + 1
from the second loop.
Dim x As Long, y As Long, z As Long, comb()
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
msgbox "Match Occurred!"
End If
Next z
Next y
Next x
Essentially, the 2nd loop controls the left side of the equation (the If comb(x, y) =
and the 3rd loop controls the right side (the = comb(x, z)
).
If desired, to prevent errors where there only may be a single value in the 2nd dimension, you could add an extra If..Then statement, like:
If Lbound(comb, 2) <> Ubound(comb, 2) Then ...
due to the nature of how the third loop adds 1 to y
's value, but an error would be raised if there isn't a 1 to add to y
.
Visualization
Here is a good visualization to see how this works. This test will print each iteration to the immediate window, you can see that each number is compared with a different number:
Sub test()
Dim x As Long, y As Long, z As Long, comb(0, 0 To 4)
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
Debug.Print y & "|" & z
End If
Next z
Next y
Next x
End Sub
Which the immediate window prints:
0|1
0|2
0|3
0|4
1|2
1|3
1|4
2|3
2|4
3|4
You can create three loops that will check each value, but will skip over each other due to the final loop being y + 1
from the second loop.
Dim x As Long, y As Long, z As Long, comb()
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
msgbox "Match Occurred!"
End If
Next z
Next y
Next x
Essentially, the 2nd loop controls the left side of the equation (the If comb(x, y) =
and the 3rd loop controls the right side (the = comb(x, z)
).
If desired, to prevent errors where there only may be a single value in the 2nd dimension, you could add an extra If..Then statement, like:
If Lbound(comb, 2) <> Ubound(comb, 2) Then ...
due to the nature of how the third loop adds 1 to y
's value, but an error would be raised if there isn't a 1 to add to y
.
Visualization
Here is a good visualization to see how this works. This test will print each iteration to the immediate window, you can see that each number is compared with a different number:
Sub test()
Dim x As Long, y As Long, z As Long, comb(0, 0 To 4)
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
Debug.Print y & "|" & z
End If
Next z
Next y
Next x
End Sub
Which the immediate window prints:
0|1
0|2
0|3
0|4
1|2
1|3
1|4
2|3
2|4
3|4
edited Nov 21 at 8:38
answered Nov 21 at 7:49


K.Dᴀᴠɪs
6,963112139
6,963112139
Thanks for the quick reply! This is headed in the right direction, but lacks a key element. Think of the elements in the 2nd dimension as a set. I want to filter for those sets where there occurs not a single element twice. Assume again the dimension is 4 and I have these elements: comb(0,0)="a" ;comb(0,1)="b"; comb(0,2)="a"; comb(0,3)="b" with your method I would get 2 matches because element 0=2 and element 1=3
– c0k3b0y
Nov 21 at 8:43
add a comment |
Thanks for the quick reply! This is headed in the right direction, but lacks a key element. Think of the elements in the 2nd dimension as a set. I want to filter for those sets where there occurs not a single element twice. Assume again the dimension is 4 and I have these elements: comb(0,0)="a" ;comb(0,1)="b"; comb(0,2)="a"; comb(0,3)="b" with your method I would get 2 matches because element 0=2 and element 1=3
– c0k3b0y
Nov 21 at 8:43
Thanks for the quick reply! This is headed in the right direction, but lacks a key element. Think of the elements in the 2nd dimension as a set. I want to filter for those sets where there occurs not a single element twice. Assume again the dimension is 4 and I have these elements: comb(0,0)="a" ;comb(0,1)="b"; comb(0,2)="a"; comb(0,3)="b" with your method I would get 2 matches because element 0=2 and element 1=3
– c0k3b0y
Nov 21 at 8:43
Thanks for the quick reply! This is headed in the right direction, but lacks a key element. Think of the elements in the 2nd dimension as a set. I want to filter for those sets where there occurs not a single element twice. Assume again the dimension is 4 and I have these elements: comb(0,0)="a" ;comb(0,1)="b"; comb(0,2)="a"; comb(0,3)="b" with your method I would get 2 matches because element 0=2 and element 1=3
– c0k3b0y
Nov 21 at 8:43
add a comment |
With K.Davis' great help I found the following solution to my problem:
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
noMatch = True
End If
Next z
Next y
If noMatch = False Then
MsgBox x
End If
noMatch = False
Next x
add a comment |
With K.Davis' great help I found the following solution to my problem:
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
noMatch = True
End If
Next z
Next y
If noMatch = False Then
MsgBox x
End If
noMatch = False
Next x
add a comment |
With K.Davis' great help I found the following solution to my problem:
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
noMatch = True
End If
Next z
Next y
If noMatch = False Then
MsgBox x
End If
noMatch = False
Next x
With K.Davis' great help I found the following solution to my problem:
For x = LBound(comb, 1) To UBound(comb, 1)
For y = LBound(comb, 2) To UBound(comb, 2) - 1
For z = y + 1 To UBound(comb, 2)
If comb(x, y) = comb(x, z) Then
noMatch = True
End If
Next z
Next y
If noMatch = False Then
MsgBox x
End If
noMatch = False
Next x
answered Nov 21 at 9:14
c0k3b0y
112
112
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53407228%2fcomparing-elements-within-a-multi-dimensional-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VmEjIo93bkp8 e0QY3 wlM,dbo6lxKLy,XK G5btcBjUvxquM0PbYR,A,xHAuRiX6T8rIy87Ywc,i1Fk,UaWSuTR
Does ubound(comb(2)) not work?
– PGCodeRider
Nov 21 at 7:45