How to use external json file for my quiz
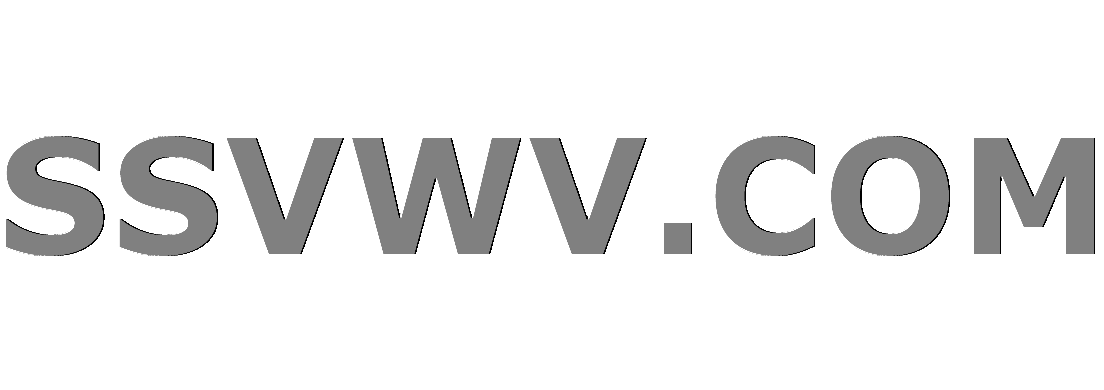
Multi tool use
So as the title says. How do I use an external json file for my quiz data instead of using a an array within javascript code. So using the var questions array and putting into a seperate json file, which is then called into javascript to use and all the radio buttons represents the choices. I am not sure on how to do this
<!DOCTYPE html>
<html>
<head>
<title> aa</title>
<link rel="stylesheet" href="styles.css">
</head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<body>
<header id="main-header">
<h1>
<center> quiz </center>
</h1>
</header>
<div id='container'>
<div id='title'>
<h1>Learning Js Properly: Project #1 - Dynamic Quiz</h1>
</div>
<br />
<div id='quiz'></div>
<div class='button' id='next'><a href=''>Next</a></div>
<div class='button' id='prev'><a href='#'>Prev</a></div>
<div class='button' id='start'> <a href='#'>Start Over</a></div>
<!-- <button class='' id='next'>Next</a></button>
<button class='' id='prev'>Prev</a></button>
<button class='' id='start'> Start Over</a></button> -->
</div>
<script type='text/javascript' src='https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js'></script>
<script type="text/javascript" src='questions.json'></script>
<script type='text/javascript' src='jsquiz.js'></script>
<script>
(function() {
var questions = [{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
}, {
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
}, {
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
}, {
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
}, {
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}];
var questionCounter = 0; //Tracks question number
var selections = ; //Array containing user choices
var quiz = $('#quiz'); //Quiz div object
// Display initial question
displayNext();
// Click handler for the 'next' button
$('#next').on('click', function(e) {
e.preventDefault();
// Suspend click listener during fade animation
if (quiz.is(':animated')) {
return false;
}
choose();
// If no user selection, progress is stopped
if (isNaN(selections[questionCounter])) {
alert('Please make a selection!');
} else {
questionCounter++;
displayNext();
}
});
// Click handler for the 'prev' button
$('#prev').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
choose();
questionCounter--;
displayNext();
});
// Click handler for the 'Start Over' button
$('#start').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
questionCounter = 0;
selections = ;
displayNext();
$('#start').hide();
});
// Animates buttons on hover
$('.button').on('mouseenter', function() {
$(this).addClass('active');
});
$('.button').on('mouseleave', function() {
$(this).removeClass('active');
});
// Creates and returns the div that contains the questions and
// the answer selections
function createQuestionElement(index) {
var qElement = $('<div>', {
id: 'question'
});
var header = $('<h2>Question ' + (index + 1) + ':</h2>');
qElement.append(header);
var question = $('<p>').append(questions[index].question);
qElement.append(question);
var radioButtons = createRadios(index);
qElement.append(radioButtons);
return qElement;
}
// Creates a list of the answer choices as radio inputs
function createRadios(index) {
var radioList = $('<ul>');
var item;
var input = '';
for (var i = 0; i < questions[index].choices.length; i++) {
item = $('<li>');
input = '<input type="radio" name="answer" value=' + i + ' />';
input += questions[index].choices[i];
item.append(input);
radioList.append(item);
}
return radioList;
}
// Reads the user selection and pushes the value to an array
function choose() {
selections[questionCounter] = +$('input[name="answer"]:checked').val();
}
// Displays next requested element
function displayNext() {
quiz.fadeOut(function() {
$('#question').remove();
if (questionCounter < questions.length) {
var nextQuestion = createQuestionElement(questionCounter);
quiz.append(nextQuestion).fadeIn();
if (!(isNaN(selections[questionCounter]))) {
$('input[value=' + selections[questionCounter] + ']').prop('checked', true);
}
// Controls display of 'prev' button
if (questionCounter === 1) {
$('#prev').show();
} else if (questionCounter === 0) {
$('#prev').hide();
$('#next').show();
}
} else {
var scoreElem = displayScore();
quiz.append(scoreElem).fadeIn();
$('#next').hide();
$('#prev').hide();
$('#start').show();
}
});
}
// Computes score and returns a paragraph element to be displayed
function displayScore() {
var score = $('<p>', {
id: 'question'
});
var numCorrect = 0;
for (var i = 0; i < selections.length; i++) {
if (selections[i] === questions[i].correctAnswer) {
numCorrect++;
}
}
score.append('You got ' + numCorrect + ' questions out of ' +
questions.length + ' right!!!');
return score;
}
})();
</script>
</body>
</html>
javascript json
add a comment |
So as the title says. How do I use an external json file for my quiz data instead of using a an array within javascript code. So using the var questions array and putting into a seperate json file, which is then called into javascript to use and all the radio buttons represents the choices. I am not sure on how to do this
<!DOCTYPE html>
<html>
<head>
<title> aa</title>
<link rel="stylesheet" href="styles.css">
</head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<body>
<header id="main-header">
<h1>
<center> quiz </center>
</h1>
</header>
<div id='container'>
<div id='title'>
<h1>Learning Js Properly: Project #1 - Dynamic Quiz</h1>
</div>
<br />
<div id='quiz'></div>
<div class='button' id='next'><a href=''>Next</a></div>
<div class='button' id='prev'><a href='#'>Prev</a></div>
<div class='button' id='start'> <a href='#'>Start Over</a></div>
<!-- <button class='' id='next'>Next</a></button>
<button class='' id='prev'>Prev</a></button>
<button class='' id='start'> Start Over</a></button> -->
</div>
<script type='text/javascript' src='https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js'></script>
<script type="text/javascript" src='questions.json'></script>
<script type='text/javascript' src='jsquiz.js'></script>
<script>
(function() {
var questions = [{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
}, {
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
}, {
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
}, {
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
}, {
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}];
var questionCounter = 0; //Tracks question number
var selections = ; //Array containing user choices
var quiz = $('#quiz'); //Quiz div object
// Display initial question
displayNext();
// Click handler for the 'next' button
$('#next').on('click', function(e) {
e.preventDefault();
// Suspend click listener during fade animation
if (quiz.is(':animated')) {
return false;
}
choose();
// If no user selection, progress is stopped
if (isNaN(selections[questionCounter])) {
alert('Please make a selection!');
} else {
questionCounter++;
displayNext();
}
});
// Click handler for the 'prev' button
$('#prev').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
choose();
questionCounter--;
displayNext();
});
// Click handler for the 'Start Over' button
$('#start').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
questionCounter = 0;
selections = ;
displayNext();
$('#start').hide();
});
// Animates buttons on hover
$('.button').on('mouseenter', function() {
$(this).addClass('active');
});
$('.button').on('mouseleave', function() {
$(this).removeClass('active');
});
// Creates and returns the div that contains the questions and
// the answer selections
function createQuestionElement(index) {
var qElement = $('<div>', {
id: 'question'
});
var header = $('<h2>Question ' + (index + 1) + ':</h2>');
qElement.append(header);
var question = $('<p>').append(questions[index].question);
qElement.append(question);
var radioButtons = createRadios(index);
qElement.append(radioButtons);
return qElement;
}
// Creates a list of the answer choices as radio inputs
function createRadios(index) {
var radioList = $('<ul>');
var item;
var input = '';
for (var i = 0; i < questions[index].choices.length; i++) {
item = $('<li>');
input = '<input type="radio" name="answer" value=' + i + ' />';
input += questions[index].choices[i];
item.append(input);
radioList.append(item);
}
return radioList;
}
// Reads the user selection and pushes the value to an array
function choose() {
selections[questionCounter] = +$('input[name="answer"]:checked').val();
}
// Displays next requested element
function displayNext() {
quiz.fadeOut(function() {
$('#question').remove();
if (questionCounter < questions.length) {
var nextQuestion = createQuestionElement(questionCounter);
quiz.append(nextQuestion).fadeIn();
if (!(isNaN(selections[questionCounter]))) {
$('input[value=' + selections[questionCounter] + ']').prop('checked', true);
}
// Controls display of 'prev' button
if (questionCounter === 1) {
$('#prev').show();
} else if (questionCounter === 0) {
$('#prev').hide();
$('#next').show();
}
} else {
var scoreElem = displayScore();
quiz.append(scoreElem).fadeIn();
$('#next').hide();
$('#prev').hide();
$('#start').show();
}
});
}
// Computes score and returns a paragraph element to be displayed
function displayScore() {
var score = $('<p>', {
id: 'question'
});
var numCorrect = 0;
for (var i = 0; i < selections.length; i++) {
if (selections[i] === questions[i].correctAnswer) {
numCorrect++;
}
}
score.append('You got ' + numCorrect + ' questions out of ' +
questions.length + ' right!!!');
return score;
}
})();
</script>
</body>
</html>
javascript json
This could be as simple aslet json_text = fetch(theURL); let questions = JSON.parse(json_text);
- sorry, don't have time to write an answer right now.
– Stephen P
Nov 21 at 1:06
add a comment |
So as the title says. How do I use an external json file for my quiz data instead of using a an array within javascript code. So using the var questions array and putting into a seperate json file, which is then called into javascript to use and all the radio buttons represents the choices. I am not sure on how to do this
<!DOCTYPE html>
<html>
<head>
<title> aa</title>
<link rel="stylesheet" href="styles.css">
</head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<body>
<header id="main-header">
<h1>
<center> quiz </center>
</h1>
</header>
<div id='container'>
<div id='title'>
<h1>Learning Js Properly: Project #1 - Dynamic Quiz</h1>
</div>
<br />
<div id='quiz'></div>
<div class='button' id='next'><a href=''>Next</a></div>
<div class='button' id='prev'><a href='#'>Prev</a></div>
<div class='button' id='start'> <a href='#'>Start Over</a></div>
<!-- <button class='' id='next'>Next</a></button>
<button class='' id='prev'>Prev</a></button>
<button class='' id='start'> Start Over</a></button> -->
</div>
<script type='text/javascript' src='https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js'></script>
<script type="text/javascript" src='questions.json'></script>
<script type='text/javascript' src='jsquiz.js'></script>
<script>
(function() {
var questions = [{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
}, {
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
}, {
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
}, {
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
}, {
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}];
var questionCounter = 0; //Tracks question number
var selections = ; //Array containing user choices
var quiz = $('#quiz'); //Quiz div object
// Display initial question
displayNext();
// Click handler for the 'next' button
$('#next').on('click', function(e) {
e.preventDefault();
// Suspend click listener during fade animation
if (quiz.is(':animated')) {
return false;
}
choose();
// If no user selection, progress is stopped
if (isNaN(selections[questionCounter])) {
alert('Please make a selection!');
} else {
questionCounter++;
displayNext();
}
});
// Click handler for the 'prev' button
$('#prev').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
choose();
questionCounter--;
displayNext();
});
// Click handler for the 'Start Over' button
$('#start').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
questionCounter = 0;
selections = ;
displayNext();
$('#start').hide();
});
// Animates buttons on hover
$('.button').on('mouseenter', function() {
$(this).addClass('active');
});
$('.button').on('mouseleave', function() {
$(this).removeClass('active');
});
// Creates and returns the div that contains the questions and
// the answer selections
function createQuestionElement(index) {
var qElement = $('<div>', {
id: 'question'
});
var header = $('<h2>Question ' + (index + 1) + ':</h2>');
qElement.append(header);
var question = $('<p>').append(questions[index].question);
qElement.append(question);
var radioButtons = createRadios(index);
qElement.append(radioButtons);
return qElement;
}
// Creates a list of the answer choices as radio inputs
function createRadios(index) {
var radioList = $('<ul>');
var item;
var input = '';
for (var i = 0; i < questions[index].choices.length; i++) {
item = $('<li>');
input = '<input type="radio" name="answer" value=' + i + ' />';
input += questions[index].choices[i];
item.append(input);
radioList.append(item);
}
return radioList;
}
// Reads the user selection and pushes the value to an array
function choose() {
selections[questionCounter] = +$('input[name="answer"]:checked').val();
}
// Displays next requested element
function displayNext() {
quiz.fadeOut(function() {
$('#question').remove();
if (questionCounter < questions.length) {
var nextQuestion = createQuestionElement(questionCounter);
quiz.append(nextQuestion).fadeIn();
if (!(isNaN(selections[questionCounter]))) {
$('input[value=' + selections[questionCounter] + ']').prop('checked', true);
}
// Controls display of 'prev' button
if (questionCounter === 1) {
$('#prev').show();
} else if (questionCounter === 0) {
$('#prev').hide();
$('#next').show();
}
} else {
var scoreElem = displayScore();
quiz.append(scoreElem).fadeIn();
$('#next').hide();
$('#prev').hide();
$('#start').show();
}
});
}
// Computes score and returns a paragraph element to be displayed
function displayScore() {
var score = $('<p>', {
id: 'question'
});
var numCorrect = 0;
for (var i = 0; i < selections.length; i++) {
if (selections[i] === questions[i].correctAnswer) {
numCorrect++;
}
}
score.append('You got ' + numCorrect + ' questions out of ' +
questions.length + ' right!!!');
return score;
}
})();
</script>
</body>
</html>
javascript json
So as the title says. How do I use an external json file for my quiz data instead of using a an array within javascript code. So using the var questions array and putting into a seperate json file, which is then called into javascript to use and all the radio buttons represents the choices. I am not sure on how to do this
<!DOCTYPE html>
<html>
<head>
<title> aa</title>
<link rel="stylesheet" href="styles.css">
</head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<body>
<header id="main-header">
<h1>
<center> quiz </center>
</h1>
</header>
<div id='container'>
<div id='title'>
<h1>Learning Js Properly: Project #1 - Dynamic Quiz</h1>
</div>
<br />
<div id='quiz'></div>
<div class='button' id='next'><a href=''>Next</a></div>
<div class='button' id='prev'><a href='#'>Prev</a></div>
<div class='button' id='start'> <a href='#'>Start Over</a></div>
<!-- <button class='' id='next'>Next</a></button>
<button class='' id='prev'>Prev</a></button>
<button class='' id='start'> Start Over</a></button> -->
</div>
<script type='text/javascript' src='https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js'></script>
<script type="text/javascript" src='questions.json'></script>
<script type='text/javascript' src='jsquiz.js'></script>
<script>
(function() {
var questions = [{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
}, {
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
}, {
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
}, {
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
}, {
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}];
var questionCounter = 0; //Tracks question number
var selections = ; //Array containing user choices
var quiz = $('#quiz'); //Quiz div object
// Display initial question
displayNext();
// Click handler for the 'next' button
$('#next').on('click', function(e) {
e.preventDefault();
// Suspend click listener during fade animation
if (quiz.is(':animated')) {
return false;
}
choose();
// If no user selection, progress is stopped
if (isNaN(selections[questionCounter])) {
alert('Please make a selection!');
} else {
questionCounter++;
displayNext();
}
});
// Click handler for the 'prev' button
$('#prev').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
choose();
questionCounter--;
displayNext();
});
// Click handler for the 'Start Over' button
$('#start').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
questionCounter = 0;
selections = ;
displayNext();
$('#start').hide();
});
// Animates buttons on hover
$('.button').on('mouseenter', function() {
$(this).addClass('active');
});
$('.button').on('mouseleave', function() {
$(this).removeClass('active');
});
// Creates and returns the div that contains the questions and
// the answer selections
function createQuestionElement(index) {
var qElement = $('<div>', {
id: 'question'
});
var header = $('<h2>Question ' + (index + 1) + ':</h2>');
qElement.append(header);
var question = $('<p>').append(questions[index].question);
qElement.append(question);
var radioButtons = createRadios(index);
qElement.append(radioButtons);
return qElement;
}
// Creates a list of the answer choices as radio inputs
function createRadios(index) {
var radioList = $('<ul>');
var item;
var input = '';
for (var i = 0; i < questions[index].choices.length; i++) {
item = $('<li>');
input = '<input type="radio" name="answer" value=' + i + ' />';
input += questions[index].choices[i];
item.append(input);
radioList.append(item);
}
return radioList;
}
// Reads the user selection and pushes the value to an array
function choose() {
selections[questionCounter] = +$('input[name="answer"]:checked').val();
}
// Displays next requested element
function displayNext() {
quiz.fadeOut(function() {
$('#question').remove();
if (questionCounter < questions.length) {
var nextQuestion = createQuestionElement(questionCounter);
quiz.append(nextQuestion).fadeIn();
if (!(isNaN(selections[questionCounter]))) {
$('input[value=' + selections[questionCounter] + ']').prop('checked', true);
}
// Controls display of 'prev' button
if (questionCounter === 1) {
$('#prev').show();
} else if (questionCounter === 0) {
$('#prev').hide();
$('#next').show();
}
} else {
var scoreElem = displayScore();
quiz.append(scoreElem).fadeIn();
$('#next').hide();
$('#prev').hide();
$('#start').show();
}
});
}
// Computes score and returns a paragraph element to be displayed
function displayScore() {
var score = $('<p>', {
id: 'question'
});
var numCorrect = 0;
for (var i = 0; i < selections.length; i++) {
if (selections[i] === questions[i].correctAnswer) {
numCorrect++;
}
}
score.append('You got ' + numCorrect + ' questions out of ' +
questions.length + ' right!!!');
return score;
}
})();
</script>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title> aa</title>
<link rel="stylesheet" href="styles.css">
</head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<body>
<header id="main-header">
<h1>
<center> quiz </center>
</h1>
</header>
<div id='container'>
<div id='title'>
<h1>Learning Js Properly: Project #1 - Dynamic Quiz</h1>
</div>
<br />
<div id='quiz'></div>
<div class='button' id='next'><a href=''>Next</a></div>
<div class='button' id='prev'><a href='#'>Prev</a></div>
<div class='button' id='start'> <a href='#'>Start Over</a></div>
<!-- <button class='' id='next'>Next</a></button>
<button class='' id='prev'>Prev</a></button>
<button class='' id='start'> Start Over</a></button> -->
</div>
<script type='text/javascript' src='https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js'></script>
<script type="text/javascript" src='questions.json'></script>
<script type='text/javascript' src='jsquiz.js'></script>
<script>
(function() {
var questions = [{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
}, {
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
}, {
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
}, {
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
}, {
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}];
var questionCounter = 0; //Tracks question number
var selections = ; //Array containing user choices
var quiz = $('#quiz'); //Quiz div object
// Display initial question
displayNext();
// Click handler for the 'next' button
$('#next').on('click', function(e) {
e.preventDefault();
// Suspend click listener during fade animation
if (quiz.is(':animated')) {
return false;
}
choose();
// If no user selection, progress is stopped
if (isNaN(selections[questionCounter])) {
alert('Please make a selection!');
} else {
questionCounter++;
displayNext();
}
});
// Click handler for the 'prev' button
$('#prev').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
choose();
questionCounter--;
displayNext();
});
// Click handler for the 'Start Over' button
$('#start').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
questionCounter = 0;
selections = ;
displayNext();
$('#start').hide();
});
// Animates buttons on hover
$('.button').on('mouseenter', function() {
$(this).addClass('active');
});
$('.button').on('mouseleave', function() {
$(this).removeClass('active');
});
// Creates and returns the div that contains the questions and
// the answer selections
function createQuestionElement(index) {
var qElement = $('<div>', {
id: 'question'
});
var header = $('<h2>Question ' + (index + 1) + ':</h2>');
qElement.append(header);
var question = $('<p>').append(questions[index].question);
qElement.append(question);
var radioButtons = createRadios(index);
qElement.append(radioButtons);
return qElement;
}
// Creates a list of the answer choices as radio inputs
function createRadios(index) {
var radioList = $('<ul>');
var item;
var input = '';
for (var i = 0; i < questions[index].choices.length; i++) {
item = $('<li>');
input = '<input type="radio" name="answer" value=' + i + ' />';
input += questions[index].choices[i];
item.append(input);
radioList.append(item);
}
return radioList;
}
// Reads the user selection and pushes the value to an array
function choose() {
selections[questionCounter] = +$('input[name="answer"]:checked').val();
}
// Displays next requested element
function displayNext() {
quiz.fadeOut(function() {
$('#question').remove();
if (questionCounter < questions.length) {
var nextQuestion = createQuestionElement(questionCounter);
quiz.append(nextQuestion).fadeIn();
if (!(isNaN(selections[questionCounter]))) {
$('input[value=' + selections[questionCounter] + ']').prop('checked', true);
}
// Controls display of 'prev' button
if (questionCounter === 1) {
$('#prev').show();
} else if (questionCounter === 0) {
$('#prev').hide();
$('#next').show();
}
} else {
var scoreElem = displayScore();
quiz.append(scoreElem).fadeIn();
$('#next').hide();
$('#prev').hide();
$('#start').show();
}
});
}
// Computes score and returns a paragraph element to be displayed
function displayScore() {
var score = $('<p>', {
id: 'question'
});
var numCorrect = 0;
for (var i = 0; i < selections.length; i++) {
if (selections[i] === questions[i].correctAnswer) {
numCorrect++;
}
}
score.append('You got ' + numCorrect + ' questions out of ' +
questions.length + ' right!!!');
return score;
}
})();
</script>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title> aa</title>
<link rel="stylesheet" href="styles.css">
</head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<body>
<header id="main-header">
<h1>
<center> quiz </center>
</h1>
</header>
<div id='container'>
<div id='title'>
<h1>Learning Js Properly: Project #1 - Dynamic Quiz</h1>
</div>
<br />
<div id='quiz'></div>
<div class='button' id='next'><a href=''>Next</a></div>
<div class='button' id='prev'><a href='#'>Prev</a></div>
<div class='button' id='start'> <a href='#'>Start Over</a></div>
<!-- <button class='' id='next'>Next</a></button>
<button class='' id='prev'>Prev</a></button>
<button class='' id='start'> Start Over</a></button> -->
</div>
<script type='text/javascript' src='https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js'></script>
<script type="text/javascript" src='questions.json'></script>
<script type='text/javascript' src='jsquiz.js'></script>
<script>
(function() {
var questions = [{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
}, {
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
}, {
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
}, {
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
}, {
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}];
var questionCounter = 0; //Tracks question number
var selections = ; //Array containing user choices
var quiz = $('#quiz'); //Quiz div object
// Display initial question
displayNext();
// Click handler for the 'next' button
$('#next').on('click', function(e) {
e.preventDefault();
// Suspend click listener during fade animation
if (quiz.is(':animated')) {
return false;
}
choose();
// If no user selection, progress is stopped
if (isNaN(selections[questionCounter])) {
alert('Please make a selection!');
} else {
questionCounter++;
displayNext();
}
});
// Click handler for the 'prev' button
$('#prev').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
choose();
questionCounter--;
displayNext();
});
// Click handler for the 'Start Over' button
$('#start').on('click', function(e) {
e.preventDefault();
if (quiz.is(':animated')) {
return false;
}
questionCounter = 0;
selections = ;
displayNext();
$('#start').hide();
});
// Animates buttons on hover
$('.button').on('mouseenter', function() {
$(this).addClass('active');
});
$('.button').on('mouseleave', function() {
$(this).removeClass('active');
});
// Creates and returns the div that contains the questions and
// the answer selections
function createQuestionElement(index) {
var qElement = $('<div>', {
id: 'question'
});
var header = $('<h2>Question ' + (index + 1) + ':</h2>');
qElement.append(header);
var question = $('<p>').append(questions[index].question);
qElement.append(question);
var radioButtons = createRadios(index);
qElement.append(radioButtons);
return qElement;
}
// Creates a list of the answer choices as radio inputs
function createRadios(index) {
var radioList = $('<ul>');
var item;
var input = '';
for (var i = 0; i < questions[index].choices.length; i++) {
item = $('<li>');
input = '<input type="radio" name="answer" value=' + i + ' />';
input += questions[index].choices[i];
item.append(input);
radioList.append(item);
}
return radioList;
}
// Reads the user selection and pushes the value to an array
function choose() {
selections[questionCounter] = +$('input[name="answer"]:checked').val();
}
// Displays next requested element
function displayNext() {
quiz.fadeOut(function() {
$('#question').remove();
if (questionCounter < questions.length) {
var nextQuestion = createQuestionElement(questionCounter);
quiz.append(nextQuestion).fadeIn();
if (!(isNaN(selections[questionCounter]))) {
$('input[value=' + selections[questionCounter] + ']').prop('checked', true);
}
// Controls display of 'prev' button
if (questionCounter === 1) {
$('#prev').show();
} else if (questionCounter === 0) {
$('#prev').hide();
$('#next').show();
}
} else {
var scoreElem = displayScore();
quiz.append(scoreElem).fadeIn();
$('#next').hide();
$('#prev').hide();
$('#start').show();
}
});
}
// Computes score and returns a paragraph element to be displayed
function displayScore() {
var score = $('<p>', {
id: 'question'
});
var numCorrect = 0;
for (var i = 0; i < selections.length; i++) {
if (selections[i] === questions[i].correctAnswer) {
numCorrect++;
}
}
score.append('You got ' + numCorrect + ' questions out of ' +
questions.length + ' right!!!');
return score;
}
})();
</script>
</body>
</html>
javascript json
javascript json
asked Nov 21 at 0:12
Noob Programmer
206
206
This could be as simple aslet json_text = fetch(theURL); let questions = JSON.parse(json_text);
- sorry, don't have time to write an answer right now.
– Stephen P
Nov 21 at 1:06
add a comment |
This could be as simple aslet json_text = fetch(theURL); let questions = JSON.parse(json_text);
- sorry, don't have time to write an answer right now.
– Stephen P
Nov 21 at 1:06
This could be as simple as
let json_text = fetch(theURL); let questions = JSON.parse(json_text);
- sorry, don't have time to write an answer right now.– Stephen P
Nov 21 at 1:06
This could be as simple as
let json_text = fetch(theURL); let questions = JSON.parse(json_text);
- sorry, don't have time to write an answer right now.– Stephen P
Nov 21 at 1:06
add a comment |
2 Answers
2
active
oldest
votes
You can fetch the JSON simply by:
fetch(url)
.then(result => JSON.parse(result))
.then(resultParsed => {
//do something with result after parsing
console.log(resultParsed)
})
See also:
https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API
Sorry, I dont quite understand
– Noob Programmer
Nov 21 at 17:48
add a comment |
It would be as simple as making your questions array into a file called questions.js and looking like so:
questions = [
{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
},
{
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
},
{
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
},
{
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
},
{
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}
];
then have at the bottom of your body a tag <script src="questions.js" type="text/javascript"></script>
then the first time you need the array just call it as questions (no need to declare it with var or let).
This is not valid JSON. It is not what an external json file for my quiz data should look like.
– radarbob
Nov 21 at 2:33
sorry, but the file needs to be in json and not js
– Noob Programmer
Nov 21 at 17:52
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403516%2fhow-to-use-external-json-file-for-my-quiz%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can fetch the JSON simply by:
fetch(url)
.then(result => JSON.parse(result))
.then(resultParsed => {
//do something with result after parsing
console.log(resultParsed)
})
See also:
https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API
Sorry, I dont quite understand
– Noob Programmer
Nov 21 at 17:48
add a comment |
You can fetch the JSON simply by:
fetch(url)
.then(result => JSON.parse(result))
.then(resultParsed => {
//do something with result after parsing
console.log(resultParsed)
})
See also:
https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API
Sorry, I dont quite understand
– Noob Programmer
Nov 21 at 17:48
add a comment |
You can fetch the JSON simply by:
fetch(url)
.then(result => JSON.parse(result))
.then(resultParsed => {
//do something with result after parsing
console.log(resultParsed)
})
See also:
https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API
You can fetch the JSON simply by:
fetch(url)
.then(result => JSON.parse(result))
.then(resultParsed => {
//do something with result after parsing
console.log(resultParsed)
})
See also:
https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API
fetch(url)
.then(result => JSON.parse(result))
.then(resultParsed => {
//do something with result after parsing
console.log(resultParsed)
})
fetch(url)
.then(result => JSON.parse(result))
.then(resultParsed => {
//do something with result after parsing
console.log(resultParsed)
})
answered Nov 21 at 2:46


Microsmsm
1,8851624
1,8851624
Sorry, I dont quite understand
– Noob Programmer
Nov 21 at 17:48
add a comment |
Sorry, I dont quite understand
– Noob Programmer
Nov 21 at 17:48
Sorry, I dont quite understand
– Noob Programmer
Nov 21 at 17:48
Sorry, I dont quite understand
– Noob Programmer
Nov 21 at 17:48
add a comment |
It would be as simple as making your questions array into a file called questions.js and looking like so:
questions = [
{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
},
{
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
},
{
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
},
{
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
},
{
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}
];
then have at the bottom of your body a tag <script src="questions.js" type="text/javascript"></script>
then the first time you need the array just call it as questions (no need to declare it with var or let).
This is not valid JSON. It is not what an external json file for my quiz data should look like.
– radarbob
Nov 21 at 2:33
sorry, but the file needs to be in json and not js
– Noob Programmer
Nov 21 at 17:52
add a comment |
It would be as simple as making your questions array into a file called questions.js and looking like so:
questions = [
{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
},
{
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
},
{
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
},
{
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
},
{
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}
];
then have at the bottom of your body a tag <script src="questions.js" type="text/javascript"></script>
then the first time you need the array just call it as questions (no need to declare it with var or let).
This is not valid JSON. It is not what an external json file for my quiz data should look like.
– radarbob
Nov 21 at 2:33
sorry, but the file needs to be in json and not js
– Noob Programmer
Nov 21 at 17:52
add a comment |
It would be as simple as making your questions array into a file called questions.js and looking like so:
questions = [
{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
},
{
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
},
{
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
},
{
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
},
{
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}
];
then have at the bottom of your body a tag <script src="questions.js" type="text/javascript"></script>
then the first time you need the array just call it as questions (no need to declare it with var or let).
It would be as simple as making your questions array into a file called questions.js and looking like so:
questions = [
{
question: "What is 2*5?",
choices: [2, 5, 10, 15, 20],
correctAnswer: 2
},
{
question: "What is 3*6?",
choices: [3, 6, 9, 12, 18],
correctAnswer: 4
},
{
question: "What is 8*9?",
choices: [72, 99, 108, 134, 156],
correctAnswer: 0
},
{
question: "What is 1*7?",
choices: [4, 5, 6, 7, 8],
correctAnswer: 3
},
{
question: "What is 8*8?",
choices: [20, 30, 40, 50, 64],
correctAnswer: 4
}
];
then have at the bottom of your body a tag <script src="questions.js" type="text/javascript"></script>
then the first time you need the array just call it as questions (no need to declare it with var or let).
answered Nov 21 at 2:13
ecg8
8411515
8411515
This is not valid JSON. It is not what an external json file for my quiz data should look like.
– radarbob
Nov 21 at 2:33
sorry, but the file needs to be in json and not js
– Noob Programmer
Nov 21 at 17:52
add a comment |
This is not valid JSON. It is not what an external json file for my quiz data should look like.
– radarbob
Nov 21 at 2:33
sorry, but the file needs to be in json and not js
– Noob Programmer
Nov 21 at 17:52
This is not valid JSON. It is not what an external json file for my quiz data should look like.
– radarbob
Nov 21 at 2:33
This is not valid JSON. It is not what an external json file for my quiz data should look like.
– radarbob
Nov 21 at 2:33
sorry, but the file needs to be in json and not js
– Noob Programmer
Nov 21 at 17:52
sorry, but the file needs to be in json and not js
– Noob Programmer
Nov 21 at 17:52
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403516%2fhow-to-use-external-json-file-for-my-quiz%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PIPZhj7P389,BqLxl2Cvqrb Zr5o lRT15E,04,gS,MeMacUtk,dPghB2JELBMfnKTg
This could be as simple as
let json_text = fetch(theURL); let questions = JSON.parse(json_text);
- sorry, don't have time to write an answer right now.– Stephen P
Nov 21 at 1:06