Assign OnClickListener dinamically to custom layouts
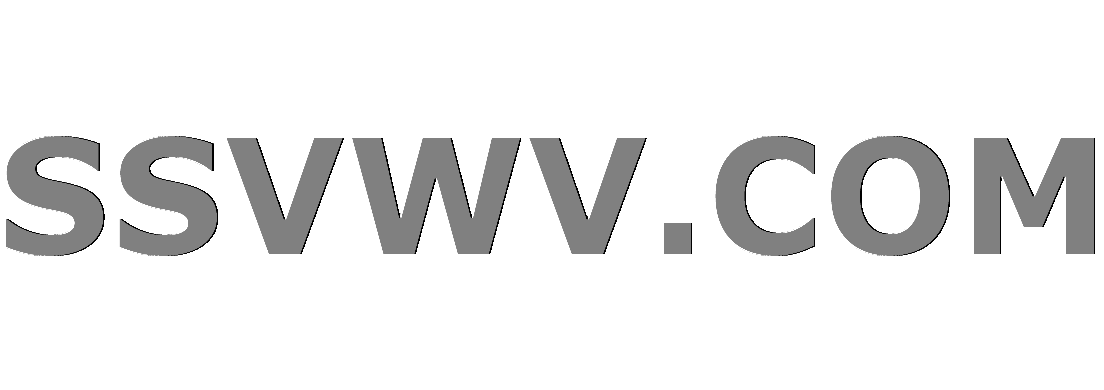
Multi tool use
I'm new to Android, with just a few hours of experience. I have created an interface with 2 main columns. Both columns have custom objects that must act as buttons. These custom objects are based on a simple custom class (ButtonItem) that has 2 textviews. Also I have a layout that contains a LinearLayout (vertical) with the two TextViews inside. In the main view, I fill both columns with and adapter and example content using the custom class and placeholder texts, and the result is that the columns have "buttons" with 2 independent text lines. The problem is to add an OnClickListeners to these objects. My idea was to add some attribute for each ButtonItem to assign an action when clicked, but until now I've had no success. Any ideas? Thanks.
This is how the columns are filled (updated)
public class TicketSaleActivity extends Activity {
private ListView lv;
private LinearLayout ll;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.ticket_sale_layout);
lv = (ListView) findViewById(R.id.buttonList);
ll = (LinearLayout)findViewById(R.id.linearLayout);
ArrayList<ButtonItem> buttonItemList = new ArrayList<>();
ButtonItem buttonItem1 = new ButtonItem();
buttonItem1.setUpperText("1€");
buttonItem1.setBottomText("Billete a Narnia");
ButtonItem buttonItem2 = new ButtonItem();
buttonItem2.setUpperText("1.5€");
buttonItem2.setBottomText("BILLETE A CANGAS DE ONIS");
ButtonItem buttonItem3 = new ButtonItem();
buttonItem3.setUpperText("1.25€");
buttonItem3.setBottomText("Billete a Narnia");
ButtonItem buttonItem4 = new ButtonItem();
buttonItem4.setUpperText("2€");
buttonItem4.setBottomText("BILLETE A CALASPARRA");
buttonItemList.add(buttonItem1);
buttonItemList.add(buttonItem2);
buttonItemList.add(buttonItem3);
buttonItemList.add(buttonItem4);
buttonItemList.add(buttonItem1);
ButtonItemAdapter buttonItemAdapter = new ButtonItemAdapter(this, buttonItemList);
lv.setAdapter(buttonItemAdapter);
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked");
}
});
for (int i=0;i<buttonItemList.size();i++)
{
View item = buttonItemAdapter.getView(i, null, null);
ll.addView(item);
}
}
}
Custom class:
public class ButtonItem{
private String upperText;
private String bottomText;
public String getUpperText() {
return upperText;
}
public void setUpperText(String upperText) {
this.upperText = upperText;
}
public String getBottomText() {
return bottomText;
}
public void setBottomText(String bottomText) {
this.bottomText = bottomText;
}
}
Adapter
public class ButtonItemAdapter extends BaseAdapter {
ArrayList<ButtonItem> buttonItemList;
Context context;
public ButtonItemAdapter(Context context, ArrayList<ButtonItem> buttonList)
{
this.context=context;
this.buttonItemList=buttonList;
}
@Override
public int getCount() {
return buttonItemList.size();
}
@Override
public Object getItem(int position) {
return buttonItemList.get(position);
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder viewHolder;
if(convertView==null)
{
convertView = LayoutInflater.from(context).inflate(R.layout.button_list_item,parent, false);
viewHolder = new ViewHolder();
viewHolder.upperText = convertView.findViewById(R.id.buttonList_upperText);
viewHolder.bottomText = convertView.findViewById(R.id.buttonList_bottomText);
convertView.setTag(viewHolder);//almacenamos los elementos en la vista para reutilizarlos
}
else{
viewHolder = (ViewHolder) convertView.getTag();
}
viewHolder.upperText.setText(buttonItemList.get(position).getUpperText());
viewHolder.bottomText.setText(buttonItemList.get(position).getBottomText());
return convertView;
}
static class ViewHolder{
TextView upperText;
TextView bottomText;
}
}
button_list_item.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
style="@style/list_button"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginEnd="1dp"
android:orientation="vertical"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:id="@+id/buttonList_upperText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_weight="1"
android:text="12€"
android:textSize="16sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/buttonList_bottomText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="8dp"
android:layout_weight="2.5"
android:maxLines="2"
android:minLines="2"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/buttonList_upperText" />
</LinearLayout>
</android.support.constraint.ConstraintLayout>
styles/list_button
<style name="list_button" parent="@android:style/Widget.Button">
<item name="android:gravity">center_vertical|center_horizontal</item>
<item name="android:textColor">#000000</item>
<item name="android:shadowColor">#000000</item>
<item name="android:shadowDx">0</item>
<item name="android:shadowDy">0</item>
<item name="android:layout_margin">0.5dp</item>
<item name="android:shadowRadius">0.1</item>
<item name="android:textSize">20dip</item>
<item name="android:textStyle">normal</item>
<item name="android:background">@drawable/default_button</item>
<item name="android:padding">2dp</item>
</style>
Update: added ticket_sale_layout
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:background="@android:color/darker_gray">
<LinearLayout
android:id="@+id/linearLayout3"
android:layout_width="match_parent"
android:layout_height="0dp"
android:baselineAligned="false"
android:orientation="horizontal"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<LinearLayout
android:id="@+id/linearLayout"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp"
android:layout_weight="1"
android:background="@android:color/darker_gray"
android:orientation="vertical"
android:padding="0dp"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.0">
</LinearLayout>
<ListView
android:id="@id/buttonList"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:layout_weight="1"
android:background="#FFF"
android:divider="@null"
android:dividerHeight="0dp"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout2"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:orientation="horizontal"
app:layout_constraintBottom_toTopOf="@+id/sellButton"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/linearLayout3"
tools:background="@android:color/darker_gray">
<TextView
android:id="@+id/textView3"
android:layout_width="150dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="#FFF"
android:focusableInTouchMode="false"
android:maxLines="2"
android:text="TextView" />
<TextView
android:id="@+id/textView2"
android:layout_width="150dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="#FFF"
android:focusableInTouchMode="false"
android:text="TextView" />
</LinearLayout>
<Button
android:id="@+id/sellButton"
style="@style/sell_button"
android:layout_width="0dp"
android:layout_height="75dp"
android:layout_marginStart="4dp"
android:layout_marginEnd="4dp"
android:text="@string/btn_sell"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>

add a comment |
I'm new to Android, with just a few hours of experience. I have created an interface with 2 main columns. Both columns have custom objects that must act as buttons. These custom objects are based on a simple custom class (ButtonItem) that has 2 textviews. Also I have a layout that contains a LinearLayout (vertical) with the two TextViews inside. In the main view, I fill both columns with and adapter and example content using the custom class and placeholder texts, and the result is that the columns have "buttons" with 2 independent text lines. The problem is to add an OnClickListeners to these objects. My idea was to add some attribute for each ButtonItem to assign an action when clicked, but until now I've had no success. Any ideas? Thanks.
This is how the columns are filled (updated)
public class TicketSaleActivity extends Activity {
private ListView lv;
private LinearLayout ll;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.ticket_sale_layout);
lv = (ListView) findViewById(R.id.buttonList);
ll = (LinearLayout)findViewById(R.id.linearLayout);
ArrayList<ButtonItem> buttonItemList = new ArrayList<>();
ButtonItem buttonItem1 = new ButtonItem();
buttonItem1.setUpperText("1€");
buttonItem1.setBottomText("Billete a Narnia");
ButtonItem buttonItem2 = new ButtonItem();
buttonItem2.setUpperText("1.5€");
buttonItem2.setBottomText("BILLETE A CANGAS DE ONIS");
ButtonItem buttonItem3 = new ButtonItem();
buttonItem3.setUpperText("1.25€");
buttonItem3.setBottomText("Billete a Narnia");
ButtonItem buttonItem4 = new ButtonItem();
buttonItem4.setUpperText("2€");
buttonItem4.setBottomText("BILLETE A CALASPARRA");
buttonItemList.add(buttonItem1);
buttonItemList.add(buttonItem2);
buttonItemList.add(buttonItem3);
buttonItemList.add(buttonItem4);
buttonItemList.add(buttonItem1);
ButtonItemAdapter buttonItemAdapter = new ButtonItemAdapter(this, buttonItemList);
lv.setAdapter(buttonItemAdapter);
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked");
}
});
for (int i=0;i<buttonItemList.size();i++)
{
View item = buttonItemAdapter.getView(i, null, null);
ll.addView(item);
}
}
}
Custom class:
public class ButtonItem{
private String upperText;
private String bottomText;
public String getUpperText() {
return upperText;
}
public void setUpperText(String upperText) {
this.upperText = upperText;
}
public String getBottomText() {
return bottomText;
}
public void setBottomText(String bottomText) {
this.bottomText = bottomText;
}
}
Adapter
public class ButtonItemAdapter extends BaseAdapter {
ArrayList<ButtonItem> buttonItemList;
Context context;
public ButtonItemAdapter(Context context, ArrayList<ButtonItem> buttonList)
{
this.context=context;
this.buttonItemList=buttonList;
}
@Override
public int getCount() {
return buttonItemList.size();
}
@Override
public Object getItem(int position) {
return buttonItemList.get(position);
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder viewHolder;
if(convertView==null)
{
convertView = LayoutInflater.from(context).inflate(R.layout.button_list_item,parent, false);
viewHolder = new ViewHolder();
viewHolder.upperText = convertView.findViewById(R.id.buttonList_upperText);
viewHolder.bottomText = convertView.findViewById(R.id.buttonList_bottomText);
convertView.setTag(viewHolder);//almacenamos los elementos en la vista para reutilizarlos
}
else{
viewHolder = (ViewHolder) convertView.getTag();
}
viewHolder.upperText.setText(buttonItemList.get(position).getUpperText());
viewHolder.bottomText.setText(buttonItemList.get(position).getBottomText());
return convertView;
}
static class ViewHolder{
TextView upperText;
TextView bottomText;
}
}
button_list_item.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
style="@style/list_button"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginEnd="1dp"
android:orientation="vertical"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:id="@+id/buttonList_upperText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_weight="1"
android:text="12€"
android:textSize="16sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/buttonList_bottomText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="8dp"
android:layout_weight="2.5"
android:maxLines="2"
android:minLines="2"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/buttonList_upperText" />
</LinearLayout>
</android.support.constraint.ConstraintLayout>
styles/list_button
<style name="list_button" parent="@android:style/Widget.Button">
<item name="android:gravity">center_vertical|center_horizontal</item>
<item name="android:textColor">#000000</item>
<item name="android:shadowColor">#000000</item>
<item name="android:shadowDx">0</item>
<item name="android:shadowDy">0</item>
<item name="android:layout_margin">0.5dp</item>
<item name="android:shadowRadius">0.1</item>
<item name="android:textSize">20dip</item>
<item name="android:textStyle">normal</item>
<item name="android:background">@drawable/default_button</item>
<item name="android:padding">2dp</item>
</style>
Update: added ticket_sale_layout
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:background="@android:color/darker_gray">
<LinearLayout
android:id="@+id/linearLayout3"
android:layout_width="match_parent"
android:layout_height="0dp"
android:baselineAligned="false"
android:orientation="horizontal"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<LinearLayout
android:id="@+id/linearLayout"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp"
android:layout_weight="1"
android:background="@android:color/darker_gray"
android:orientation="vertical"
android:padding="0dp"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.0">
</LinearLayout>
<ListView
android:id="@id/buttonList"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:layout_weight="1"
android:background="#FFF"
android:divider="@null"
android:dividerHeight="0dp"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout2"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:orientation="horizontal"
app:layout_constraintBottom_toTopOf="@+id/sellButton"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/linearLayout3"
tools:background="@android:color/darker_gray">
<TextView
android:id="@+id/textView3"
android:layout_width="150dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="#FFF"
android:focusableInTouchMode="false"
android:maxLines="2"
android:text="TextView" />
<TextView
android:id="@+id/textView2"
android:layout_width="150dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="#FFF"
android:focusableInTouchMode="false"
android:text="TextView" />
</LinearLayout>
<Button
android:id="@+id/sellButton"
style="@style/sell_button"
android:layout_width="0dp"
android:layout_height="75dp"
android:layout_marginStart="4dp"
android:layout_marginEnd="4dp"
android:text="@string/btn_sell"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>

add a comment |
I'm new to Android, with just a few hours of experience. I have created an interface with 2 main columns. Both columns have custom objects that must act as buttons. These custom objects are based on a simple custom class (ButtonItem) that has 2 textviews. Also I have a layout that contains a LinearLayout (vertical) with the two TextViews inside. In the main view, I fill both columns with and adapter and example content using the custom class and placeholder texts, and the result is that the columns have "buttons" with 2 independent text lines. The problem is to add an OnClickListeners to these objects. My idea was to add some attribute for each ButtonItem to assign an action when clicked, but until now I've had no success. Any ideas? Thanks.
This is how the columns are filled (updated)
public class TicketSaleActivity extends Activity {
private ListView lv;
private LinearLayout ll;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.ticket_sale_layout);
lv = (ListView) findViewById(R.id.buttonList);
ll = (LinearLayout)findViewById(R.id.linearLayout);
ArrayList<ButtonItem> buttonItemList = new ArrayList<>();
ButtonItem buttonItem1 = new ButtonItem();
buttonItem1.setUpperText("1€");
buttonItem1.setBottomText("Billete a Narnia");
ButtonItem buttonItem2 = new ButtonItem();
buttonItem2.setUpperText("1.5€");
buttonItem2.setBottomText("BILLETE A CANGAS DE ONIS");
ButtonItem buttonItem3 = new ButtonItem();
buttonItem3.setUpperText("1.25€");
buttonItem3.setBottomText("Billete a Narnia");
ButtonItem buttonItem4 = new ButtonItem();
buttonItem4.setUpperText("2€");
buttonItem4.setBottomText("BILLETE A CALASPARRA");
buttonItemList.add(buttonItem1);
buttonItemList.add(buttonItem2);
buttonItemList.add(buttonItem3);
buttonItemList.add(buttonItem4);
buttonItemList.add(buttonItem1);
ButtonItemAdapter buttonItemAdapter = new ButtonItemAdapter(this, buttonItemList);
lv.setAdapter(buttonItemAdapter);
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked");
}
});
for (int i=0;i<buttonItemList.size();i++)
{
View item = buttonItemAdapter.getView(i, null, null);
ll.addView(item);
}
}
}
Custom class:
public class ButtonItem{
private String upperText;
private String bottomText;
public String getUpperText() {
return upperText;
}
public void setUpperText(String upperText) {
this.upperText = upperText;
}
public String getBottomText() {
return bottomText;
}
public void setBottomText(String bottomText) {
this.bottomText = bottomText;
}
}
Adapter
public class ButtonItemAdapter extends BaseAdapter {
ArrayList<ButtonItem> buttonItemList;
Context context;
public ButtonItemAdapter(Context context, ArrayList<ButtonItem> buttonList)
{
this.context=context;
this.buttonItemList=buttonList;
}
@Override
public int getCount() {
return buttonItemList.size();
}
@Override
public Object getItem(int position) {
return buttonItemList.get(position);
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder viewHolder;
if(convertView==null)
{
convertView = LayoutInflater.from(context).inflate(R.layout.button_list_item,parent, false);
viewHolder = new ViewHolder();
viewHolder.upperText = convertView.findViewById(R.id.buttonList_upperText);
viewHolder.bottomText = convertView.findViewById(R.id.buttonList_bottomText);
convertView.setTag(viewHolder);//almacenamos los elementos en la vista para reutilizarlos
}
else{
viewHolder = (ViewHolder) convertView.getTag();
}
viewHolder.upperText.setText(buttonItemList.get(position).getUpperText());
viewHolder.bottomText.setText(buttonItemList.get(position).getBottomText());
return convertView;
}
static class ViewHolder{
TextView upperText;
TextView bottomText;
}
}
button_list_item.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
style="@style/list_button"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginEnd="1dp"
android:orientation="vertical"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:id="@+id/buttonList_upperText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_weight="1"
android:text="12€"
android:textSize="16sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/buttonList_bottomText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="8dp"
android:layout_weight="2.5"
android:maxLines="2"
android:minLines="2"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/buttonList_upperText" />
</LinearLayout>
</android.support.constraint.ConstraintLayout>
styles/list_button
<style name="list_button" parent="@android:style/Widget.Button">
<item name="android:gravity">center_vertical|center_horizontal</item>
<item name="android:textColor">#000000</item>
<item name="android:shadowColor">#000000</item>
<item name="android:shadowDx">0</item>
<item name="android:shadowDy">0</item>
<item name="android:layout_margin">0.5dp</item>
<item name="android:shadowRadius">0.1</item>
<item name="android:textSize">20dip</item>
<item name="android:textStyle">normal</item>
<item name="android:background">@drawable/default_button</item>
<item name="android:padding">2dp</item>
</style>
Update: added ticket_sale_layout
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:background="@android:color/darker_gray">
<LinearLayout
android:id="@+id/linearLayout3"
android:layout_width="match_parent"
android:layout_height="0dp"
android:baselineAligned="false"
android:orientation="horizontal"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<LinearLayout
android:id="@+id/linearLayout"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp"
android:layout_weight="1"
android:background="@android:color/darker_gray"
android:orientation="vertical"
android:padding="0dp"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.0">
</LinearLayout>
<ListView
android:id="@id/buttonList"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:layout_weight="1"
android:background="#FFF"
android:divider="@null"
android:dividerHeight="0dp"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout2"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:orientation="horizontal"
app:layout_constraintBottom_toTopOf="@+id/sellButton"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/linearLayout3"
tools:background="@android:color/darker_gray">
<TextView
android:id="@+id/textView3"
android:layout_width="150dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="#FFF"
android:focusableInTouchMode="false"
android:maxLines="2"
android:text="TextView" />
<TextView
android:id="@+id/textView2"
android:layout_width="150dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="#FFF"
android:focusableInTouchMode="false"
android:text="TextView" />
</LinearLayout>
<Button
android:id="@+id/sellButton"
style="@style/sell_button"
android:layout_width="0dp"
android:layout_height="75dp"
android:layout_marginStart="4dp"
android:layout_marginEnd="4dp"
android:text="@string/btn_sell"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>

I'm new to Android, with just a few hours of experience. I have created an interface with 2 main columns. Both columns have custom objects that must act as buttons. These custom objects are based on a simple custom class (ButtonItem) that has 2 textviews. Also I have a layout that contains a LinearLayout (vertical) with the two TextViews inside. In the main view, I fill both columns with and adapter and example content using the custom class and placeholder texts, and the result is that the columns have "buttons" with 2 independent text lines. The problem is to add an OnClickListeners to these objects. My idea was to add some attribute for each ButtonItem to assign an action when clicked, but until now I've had no success. Any ideas? Thanks.
This is how the columns are filled (updated)
public class TicketSaleActivity extends Activity {
private ListView lv;
private LinearLayout ll;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.ticket_sale_layout);
lv = (ListView) findViewById(R.id.buttonList);
ll = (LinearLayout)findViewById(R.id.linearLayout);
ArrayList<ButtonItem> buttonItemList = new ArrayList<>();
ButtonItem buttonItem1 = new ButtonItem();
buttonItem1.setUpperText("1€");
buttonItem1.setBottomText("Billete a Narnia");
ButtonItem buttonItem2 = new ButtonItem();
buttonItem2.setUpperText("1.5€");
buttonItem2.setBottomText("BILLETE A CANGAS DE ONIS");
ButtonItem buttonItem3 = new ButtonItem();
buttonItem3.setUpperText("1.25€");
buttonItem3.setBottomText("Billete a Narnia");
ButtonItem buttonItem4 = new ButtonItem();
buttonItem4.setUpperText("2€");
buttonItem4.setBottomText("BILLETE A CALASPARRA");
buttonItemList.add(buttonItem1);
buttonItemList.add(buttonItem2);
buttonItemList.add(buttonItem3);
buttonItemList.add(buttonItem4);
buttonItemList.add(buttonItem1);
ButtonItemAdapter buttonItemAdapter = new ButtonItemAdapter(this, buttonItemList);
lv.setAdapter(buttonItemAdapter);
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked");
}
});
for (int i=0;i<buttonItemList.size();i++)
{
View item = buttonItemAdapter.getView(i, null, null);
ll.addView(item);
}
}
}
Custom class:
public class ButtonItem{
private String upperText;
private String bottomText;
public String getUpperText() {
return upperText;
}
public void setUpperText(String upperText) {
this.upperText = upperText;
}
public String getBottomText() {
return bottomText;
}
public void setBottomText(String bottomText) {
this.bottomText = bottomText;
}
}
Adapter
public class ButtonItemAdapter extends BaseAdapter {
ArrayList<ButtonItem> buttonItemList;
Context context;
public ButtonItemAdapter(Context context, ArrayList<ButtonItem> buttonList)
{
this.context=context;
this.buttonItemList=buttonList;
}
@Override
public int getCount() {
return buttonItemList.size();
}
@Override
public Object getItem(int position) {
return buttonItemList.get(position);
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder viewHolder;
if(convertView==null)
{
convertView = LayoutInflater.from(context).inflate(R.layout.button_list_item,parent, false);
viewHolder = new ViewHolder();
viewHolder.upperText = convertView.findViewById(R.id.buttonList_upperText);
viewHolder.bottomText = convertView.findViewById(R.id.buttonList_bottomText);
convertView.setTag(viewHolder);//almacenamos los elementos en la vista para reutilizarlos
}
else{
viewHolder = (ViewHolder) convertView.getTag();
}
viewHolder.upperText.setText(buttonItemList.get(position).getUpperText());
viewHolder.bottomText.setText(buttonItemList.get(position).getBottomText());
return convertView;
}
static class ViewHolder{
TextView upperText;
TextView bottomText;
}
}
button_list_item.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
style="@style/list_button"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginEnd="1dp"
android:orientation="vertical"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:id="@+id/buttonList_upperText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_weight="1"
android:text="12€"
android:textSize="16sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/buttonList_bottomText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_marginStart="8dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="8dp"
android:layout_weight="2.5"
android:maxLines="2"
android:minLines="2"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/buttonList_upperText" />
</LinearLayout>
</android.support.constraint.ConstraintLayout>
styles/list_button
<style name="list_button" parent="@android:style/Widget.Button">
<item name="android:gravity">center_vertical|center_horizontal</item>
<item name="android:textColor">#000000</item>
<item name="android:shadowColor">#000000</item>
<item name="android:shadowDx">0</item>
<item name="android:shadowDy">0</item>
<item name="android:layout_margin">0.5dp</item>
<item name="android:shadowRadius">0.1</item>
<item name="android:textSize">20dip</item>
<item name="android:textStyle">normal</item>
<item name="android:background">@drawable/default_button</item>
<item name="android:padding">2dp</item>
</style>
Update: added ticket_sale_layout
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:background="@android:color/darker_gray">
<LinearLayout
android:id="@+id/linearLayout3"
android:layout_width="match_parent"
android:layout_height="0dp"
android:baselineAligned="false"
android:orientation="horizontal"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<LinearLayout
android:id="@+id/linearLayout"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp"
android:layout_weight="1"
android:background="@android:color/darker_gray"
android:orientation="vertical"
android:padding="0dp"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.0">
</LinearLayout>
<ListView
android:id="@id/buttonList"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:layout_weight="1"
android:background="#FFF"
android:divider="@null"
android:dividerHeight="0dp"
app:layout_constraintBottom_toTopOf="@+id/linearLayout2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout2"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:orientation="horizontal"
app:layout_constraintBottom_toTopOf="@+id/sellButton"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/linearLayout3"
tools:background="@android:color/darker_gray">
<TextView
android:id="@+id/textView3"
android:layout_width="150dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="#FFF"
android:focusableInTouchMode="false"
android:maxLines="2"
android:text="TextView" />
<TextView
android:id="@+id/textView2"
android:layout_width="150dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="#FFF"
android:focusableInTouchMode="false"
android:text="TextView" />
</LinearLayout>
<Button
android:id="@+id/sellButton"
style="@style/sell_button"
android:layout_width="0dp"
android:layout_height="75dp"
android:layout_marginStart="4dp"
android:layout_marginEnd="4dp"
android:text="@string/btn_sell"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>


edited Nov 23 '18 at 11:52
Rafa J
asked Nov 23 '18 at 9:25


Rafa JRafa J
188
188
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You could either bind click listener within your adapter's getView
method, or use OnItemClickListener on your AdapterView
:
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// ...
}
});
In order to receive callback in onItemClick
, you should not have any clickable or focusable views in the item views. So try removing @style/list_button
from the item XML layout, or replace it with other styles.
Thanks for your answer but seems I can't put them to work. The second solution seems the correct for me, but seems it's not working: lv.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked"); } });
– Rafa J
Nov 23 '18 at 10:01
Can you please post yourbutton_list_item
layout XML? Just making sure there's no focusable or clickable attributes in your views if you wantsetOnItemClickListener
to work.
– Aaron
Nov 23 '18 at 10:09
For sure, I've edited the question to add the xml.
– Rafa J
Nov 23 '18 at 10:19
I hope your@style/list_button
doesn't contain clickable attribute, otherwise it looks normal to me. Could you describe what is not working? Is it only the clicking issue?
– Aaron
Nov 23 '18 at 10:25
Added @style/list_button. There is no clickable attribute as you can see. I want each "button" to do some action. Writing someting in the log could be a good start ^^. Right now any button is working, and for work I mean write in the log on click. and yeah, it's the only issue I have right now. Maybe I have to re-do all from scratch. Any suggestions to achieve this? And thanks for your time!
– Rafa J
Nov 23 '18 at 10:33
|
show 8 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53443831%2fassign-onclicklistener-dinamically-to-custom-layouts%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could either bind click listener within your adapter's getView
method, or use OnItemClickListener on your AdapterView
:
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// ...
}
});
In order to receive callback in onItemClick
, you should not have any clickable or focusable views in the item views. So try removing @style/list_button
from the item XML layout, or replace it with other styles.
Thanks for your answer but seems I can't put them to work. The second solution seems the correct for me, but seems it's not working: lv.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked"); } });
– Rafa J
Nov 23 '18 at 10:01
Can you please post yourbutton_list_item
layout XML? Just making sure there's no focusable or clickable attributes in your views if you wantsetOnItemClickListener
to work.
– Aaron
Nov 23 '18 at 10:09
For sure, I've edited the question to add the xml.
– Rafa J
Nov 23 '18 at 10:19
I hope your@style/list_button
doesn't contain clickable attribute, otherwise it looks normal to me. Could you describe what is not working? Is it only the clicking issue?
– Aaron
Nov 23 '18 at 10:25
Added @style/list_button. There is no clickable attribute as you can see. I want each "button" to do some action. Writing someting in the log could be a good start ^^. Right now any button is working, and for work I mean write in the log on click. and yeah, it's the only issue I have right now. Maybe I have to re-do all from scratch. Any suggestions to achieve this? And thanks for your time!
– Rafa J
Nov 23 '18 at 10:33
|
show 8 more comments
You could either bind click listener within your adapter's getView
method, or use OnItemClickListener on your AdapterView
:
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// ...
}
});
In order to receive callback in onItemClick
, you should not have any clickable or focusable views in the item views. So try removing @style/list_button
from the item XML layout, or replace it with other styles.
Thanks for your answer but seems I can't put them to work. The second solution seems the correct for me, but seems it's not working: lv.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked"); } });
– Rafa J
Nov 23 '18 at 10:01
Can you please post yourbutton_list_item
layout XML? Just making sure there's no focusable or clickable attributes in your views if you wantsetOnItemClickListener
to work.
– Aaron
Nov 23 '18 at 10:09
For sure, I've edited the question to add the xml.
– Rafa J
Nov 23 '18 at 10:19
I hope your@style/list_button
doesn't contain clickable attribute, otherwise it looks normal to me. Could you describe what is not working? Is it only the clicking issue?
– Aaron
Nov 23 '18 at 10:25
Added @style/list_button. There is no clickable attribute as you can see. I want each "button" to do some action. Writing someting in the log could be a good start ^^. Right now any button is working, and for work I mean write in the log on click. and yeah, it's the only issue I have right now. Maybe I have to re-do all from scratch. Any suggestions to achieve this? And thanks for your time!
– Rafa J
Nov 23 '18 at 10:33
|
show 8 more comments
You could either bind click listener within your adapter's getView
method, or use OnItemClickListener on your AdapterView
:
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// ...
}
});
In order to receive callback in onItemClick
, you should not have any clickable or focusable views in the item views. So try removing @style/list_button
from the item XML layout, or replace it with other styles.
You could either bind click listener within your adapter's getView
method, or use OnItemClickListener on your AdapterView
:
lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// ...
}
});
In order to receive callback in onItemClick
, you should not have any clickable or focusable views in the item views. So try removing @style/list_button
from the item XML layout, or replace it with other styles.
edited Nov 23 '18 at 12:58
answered Nov 23 '18 at 9:35


AaronAaron
1,7332212
1,7332212
Thanks for your answer but seems I can't put them to work. The second solution seems the correct for me, but seems it's not working: lv.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked"); } });
– Rafa J
Nov 23 '18 at 10:01
Can you please post yourbutton_list_item
layout XML? Just making sure there's no focusable or clickable attributes in your views if you wantsetOnItemClickListener
to work.
– Aaron
Nov 23 '18 at 10:09
For sure, I've edited the question to add the xml.
– Rafa J
Nov 23 '18 at 10:19
I hope your@style/list_button
doesn't contain clickable attribute, otherwise it looks normal to me. Could you describe what is not working? Is it only the clicking issue?
– Aaron
Nov 23 '18 at 10:25
Added @style/list_button. There is no clickable attribute as you can see. I want each "button" to do some action. Writing someting in the log could be a good start ^^. Right now any button is working, and for work I mean write in the log on click. and yeah, it's the only issue I have right now. Maybe I have to re-do all from scratch. Any suggestions to achieve this? And thanks for your time!
– Rafa J
Nov 23 '18 at 10:33
|
show 8 more comments
Thanks for your answer but seems I can't put them to work. The second solution seems the correct for me, but seems it's not working: lv.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked"); } });
– Rafa J
Nov 23 '18 at 10:01
Can you please post yourbutton_list_item
layout XML? Just making sure there's no focusable or clickable attributes in your views if you wantsetOnItemClickListener
to work.
– Aaron
Nov 23 '18 at 10:09
For sure, I've edited the question to add the xml.
– Rafa J
Nov 23 '18 at 10:19
I hope your@style/list_button
doesn't contain clickable attribute, otherwise it looks normal to me. Could you describe what is not working? Is it only the clicking issue?
– Aaron
Nov 23 '18 at 10:25
Added @style/list_button. There is no clickable attribute as you can see. I want each "button" to do some action. Writing someting in the log could be a good start ^^. Right now any button is working, and for work I mean write in the log on click. and yeah, it's the only issue I have right now. Maybe I have to re-do all from scratch. Any suggestions to achieve this? And thanks for your time!
– Rafa J
Nov 23 '18 at 10:33
Thanks for your answer but seems I can't put them to work. The second solution seems the correct for me, but seems it's not working: lv.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked"); } });
– Rafa J
Nov 23 '18 at 10:01
Thanks for your answer but seems I can't put them to work. The second solution seems the correct for me, but seems it's not working: lv.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Log.i(TicketSaleActivity.class.getSimpleName(),"Clicked"); } });
– Rafa J
Nov 23 '18 at 10:01
Can you please post your
button_list_item
layout XML? Just making sure there's no focusable or clickable attributes in your views if you want setOnItemClickListener
to work.– Aaron
Nov 23 '18 at 10:09
Can you please post your
button_list_item
layout XML? Just making sure there's no focusable or clickable attributes in your views if you want setOnItemClickListener
to work.– Aaron
Nov 23 '18 at 10:09
For sure, I've edited the question to add the xml.
– Rafa J
Nov 23 '18 at 10:19
For sure, I've edited the question to add the xml.
– Rafa J
Nov 23 '18 at 10:19
I hope your
@style/list_button
doesn't contain clickable attribute, otherwise it looks normal to me. Could you describe what is not working? Is it only the clicking issue?– Aaron
Nov 23 '18 at 10:25
I hope your
@style/list_button
doesn't contain clickable attribute, otherwise it looks normal to me. Could you describe what is not working? Is it only the clicking issue?– Aaron
Nov 23 '18 at 10:25
Added @style/list_button. There is no clickable attribute as you can see. I want each "button" to do some action. Writing someting in the log could be a good start ^^. Right now any button is working, and for work I mean write in the log on click. and yeah, it's the only issue I have right now. Maybe I have to re-do all from scratch. Any suggestions to achieve this? And thanks for your time!
– Rafa J
Nov 23 '18 at 10:33
Added @style/list_button. There is no clickable attribute as you can see. I want each "button" to do some action. Writing someting in the log could be a good start ^^. Right now any button is working, and for work I mean write in the log on click. and yeah, it's the only issue I have right now. Maybe I have to re-do all from scratch. Any suggestions to achieve this? And thanks for your time!
– Rafa J
Nov 23 '18 at 10:33
|
show 8 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53443831%2fassign-onclicklistener-dinamically-to-custom-layouts%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
loTCVDGQGrnBhywIJwlwZg,7pyp Fo DD4aLSPcnrL emNFnBrxRn2UOO1,rEagM0GZ