Java Merge Sort on Linked List sorting only when smallest number is added first
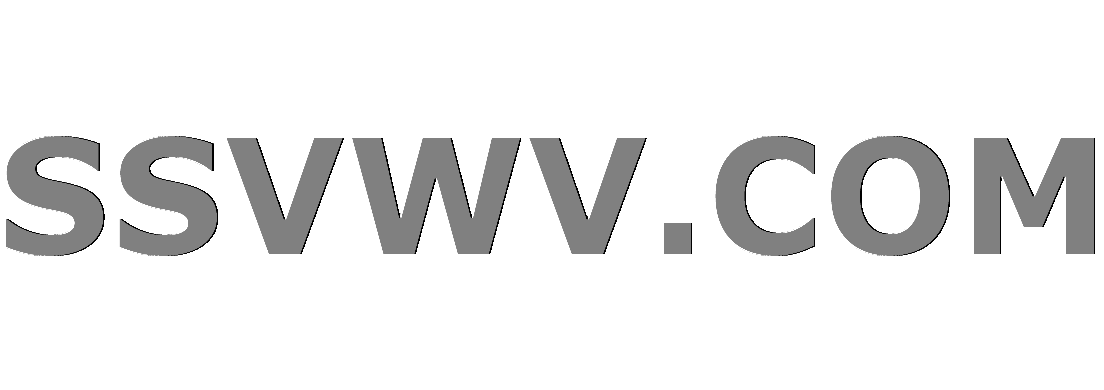
Multi tool use
When I insert numbers in my linked list it only sorts everything when the first number added to it is the smallest. This is the unexpected behavior that I am getting:
I insert the numbers in this order - 200, 25, 473, 23, 390
If I sort the list like that above and display it, I get this - 200, 390, 473
If I change 200 to 900 then sort the list, it only displays 900
It only works when I change 200 to the smallest number in the list like 1 (or anything less than 23), then it gives me the right output 1, 23, 25, 390, 473
For the linked list I insert the elements at the back of the the list, this is the code:
public void insertAtBack(IntegerElement elem) {
if (isFull()) {
System.out.println("Unable to insert node into full list");
} else {
Node temp = new Node(elem);
if (isEmpty()) {
head = temp;
} else {
Node current = head;
while (current.getLink() != null) {
current = current.getLink();
}
current.setLink(temp);
}
}
}
And this is the code I use to merge sort:
public Node getMiddle(Node node) {
if (node == null)
return node;
Node fastptr = node.getLink();
Node slowptr = node;
// Move fastptr by two and slow ptr by one
// Finally slowptr will point to middle node
while (fastptr != null) {
fastptr = fastptr.getLink();
if(fastptr != null) {
slowptr = slowptr.getLink();
fastptr = fastptr.getLink();
}
}
return slowptr;
}
public Node merge(Node left, Node right) {
Node tempHead = new Node(), curr;
curr = tempHead;
while(left != null && right != null) {
if(left.getData().getValue() <= right.getData().getValue()) {
curr.setLink(left);
left = left.getLink();
}else {
curr.setLink(right);
right = right.getLink();
}
curr = curr.getLink();
}
curr.setLink((left == null) ? right : left);
return tempHead.getLink();
}
/**
* This method utilizes merge sort algorithm
*
* @param node - Pass in head first
*
*/
public Node sort(Node node) {
// Base case : if head is null
if (node == null || node.getLink() == null)
return node;
// get the middle of the list
Node middle = getMiddle(node);
Node nextofmiddle = middle.getLink();
// set the next of middle node to null
middle.setLink(null);
// Merge the left and right lists
return merge(sort(node), sort(nextofmiddle));
}
Any help is appreciated
java sorting mergesort
add a comment |
When I insert numbers in my linked list it only sorts everything when the first number added to it is the smallest. This is the unexpected behavior that I am getting:
I insert the numbers in this order - 200, 25, 473, 23, 390
If I sort the list like that above and display it, I get this - 200, 390, 473
If I change 200 to 900 then sort the list, it only displays 900
It only works when I change 200 to the smallest number in the list like 1 (or anything less than 23), then it gives me the right output 1, 23, 25, 390, 473
For the linked list I insert the elements at the back of the the list, this is the code:
public void insertAtBack(IntegerElement elem) {
if (isFull()) {
System.out.println("Unable to insert node into full list");
} else {
Node temp = new Node(elem);
if (isEmpty()) {
head = temp;
} else {
Node current = head;
while (current.getLink() != null) {
current = current.getLink();
}
current.setLink(temp);
}
}
}
And this is the code I use to merge sort:
public Node getMiddle(Node node) {
if (node == null)
return node;
Node fastptr = node.getLink();
Node slowptr = node;
// Move fastptr by two and slow ptr by one
// Finally slowptr will point to middle node
while (fastptr != null) {
fastptr = fastptr.getLink();
if(fastptr != null) {
slowptr = slowptr.getLink();
fastptr = fastptr.getLink();
}
}
return slowptr;
}
public Node merge(Node left, Node right) {
Node tempHead = new Node(), curr;
curr = tempHead;
while(left != null && right != null) {
if(left.getData().getValue() <= right.getData().getValue()) {
curr.setLink(left);
left = left.getLink();
}else {
curr.setLink(right);
right = right.getLink();
}
curr = curr.getLink();
}
curr.setLink((left == null) ? right : left);
return tempHead.getLink();
}
/**
* This method utilizes merge sort algorithm
*
* @param node - Pass in head first
*
*/
public Node sort(Node node) {
// Base case : if head is null
if (node == null || node.getLink() == null)
return node;
// get the middle of the list
Node middle = getMiddle(node);
Node nextofmiddle = middle.getLink();
// set the next of middle node to null
middle.setLink(null);
// Merge the left and right lists
return merge(sort(node), sort(nextofmiddle));
}
Any help is appreciated
java sorting mergesort
1
Please provide an Minimal, Complete, and Verifiable example.
– samabcde
Nov 21 at 4:31
add a comment |
When I insert numbers in my linked list it only sorts everything when the first number added to it is the smallest. This is the unexpected behavior that I am getting:
I insert the numbers in this order - 200, 25, 473, 23, 390
If I sort the list like that above and display it, I get this - 200, 390, 473
If I change 200 to 900 then sort the list, it only displays 900
It only works when I change 200 to the smallest number in the list like 1 (or anything less than 23), then it gives me the right output 1, 23, 25, 390, 473
For the linked list I insert the elements at the back of the the list, this is the code:
public void insertAtBack(IntegerElement elem) {
if (isFull()) {
System.out.println("Unable to insert node into full list");
} else {
Node temp = new Node(elem);
if (isEmpty()) {
head = temp;
} else {
Node current = head;
while (current.getLink() != null) {
current = current.getLink();
}
current.setLink(temp);
}
}
}
And this is the code I use to merge sort:
public Node getMiddle(Node node) {
if (node == null)
return node;
Node fastptr = node.getLink();
Node slowptr = node;
// Move fastptr by two and slow ptr by one
// Finally slowptr will point to middle node
while (fastptr != null) {
fastptr = fastptr.getLink();
if(fastptr != null) {
slowptr = slowptr.getLink();
fastptr = fastptr.getLink();
}
}
return slowptr;
}
public Node merge(Node left, Node right) {
Node tempHead = new Node(), curr;
curr = tempHead;
while(left != null && right != null) {
if(left.getData().getValue() <= right.getData().getValue()) {
curr.setLink(left);
left = left.getLink();
}else {
curr.setLink(right);
right = right.getLink();
}
curr = curr.getLink();
}
curr.setLink((left == null) ? right : left);
return tempHead.getLink();
}
/**
* This method utilizes merge sort algorithm
*
* @param node - Pass in head first
*
*/
public Node sort(Node node) {
// Base case : if head is null
if (node == null || node.getLink() == null)
return node;
// get the middle of the list
Node middle = getMiddle(node);
Node nextofmiddle = middle.getLink();
// set the next of middle node to null
middle.setLink(null);
// Merge the left and right lists
return merge(sort(node), sort(nextofmiddle));
}
Any help is appreciated
java sorting mergesort
When I insert numbers in my linked list it only sorts everything when the first number added to it is the smallest. This is the unexpected behavior that I am getting:
I insert the numbers in this order - 200, 25, 473, 23, 390
If I sort the list like that above and display it, I get this - 200, 390, 473
If I change 200 to 900 then sort the list, it only displays 900
It only works when I change 200 to the smallest number in the list like 1 (or anything less than 23), then it gives me the right output 1, 23, 25, 390, 473
For the linked list I insert the elements at the back of the the list, this is the code:
public void insertAtBack(IntegerElement elem) {
if (isFull()) {
System.out.println("Unable to insert node into full list");
} else {
Node temp = new Node(elem);
if (isEmpty()) {
head = temp;
} else {
Node current = head;
while (current.getLink() != null) {
current = current.getLink();
}
current.setLink(temp);
}
}
}
And this is the code I use to merge sort:
public Node getMiddle(Node node) {
if (node == null)
return node;
Node fastptr = node.getLink();
Node slowptr = node;
// Move fastptr by two and slow ptr by one
// Finally slowptr will point to middle node
while (fastptr != null) {
fastptr = fastptr.getLink();
if(fastptr != null) {
slowptr = slowptr.getLink();
fastptr = fastptr.getLink();
}
}
return slowptr;
}
public Node merge(Node left, Node right) {
Node tempHead = new Node(), curr;
curr = tempHead;
while(left != null && right != null) {
if(left.getData().getValue() <= right.getData().getValue()) {
curr.setLink(left);
left = left.getLink();
}else {
curr.setLink(right);
right = right.getLink();
}
curr = curr.getLink();
}
curr.setLink((left == null) ? right : left);
return tempHead.getLink();
}
/**
* This method utilizes merge sort algorithm
*
* @param node - Pass in head first
*
*/
public Node sort(Node node) {
// Base case : if head is null
if (node == null || node.getLink() == null)
return node;
// get the middle of the list
Node middle = getMiddle(node);
Node nextofmiddle = middle.getLink();
// set the next of middle node to null
middle.setLink(null);
// Merge the left and right lists
return merge(sort(node), sort(nextofmiddle));
}
Any help is appreciated
java sorting mergesort
java sorting mergesort
asked Nov 21 at 3:05


chinloyal
1,02423
1,02423
1
Please provide an Minimal, Complete, and Verifiable example.
– samabcde
Nov 21 at 4:31
add a comment |
1
Please provide an Minimal, Complete, and Verifiable example.
– samabcde
Nov 21 at 4:31
1
1
Please provide an Minimal, Complete, and Verifiable example.
– samabcde
Nov 21 at 4:31
Please provide an Minimal, Complete, and Verifiable example.
– samabcde
Nov 21 at 4:31
add a comment |
1 Answer
1
active
oldest
votes
Your sort routine looks great. The bug appears to be the way you are returning from your sort routine. If you call your sort as if it were an in-place sort, i.e.
sort(head);
System.out.println(head);
the old head is not updated to the new head returned by sort()
. Notice how the nodes that are being displayed in your test cases always begin with whatever the old head was. This appears to work if the old head in the unsorted list happens to be the new head in the sorted list, as in your last example. If, on the other hand, the old head node is moved during the sort, you'll only see the rest of the list from wherever the old head moved to onward. Nodes from the front of the list up to the old head will be garbage collected when they go out of scope.
The fix is to set the head of your list in the caller to the returned head from sort()
, which is the new head of the list:
head = sort(head);
System.out.println(head);
Here's your code re-written which reproduces your errors to illustrate the problem:
class Main {
public static void main(String args) {
Node head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("Example 1 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 1 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 2 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 2 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(1, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 3 (accidentally works, because the old head is still the new head):");
System.out.println(head);
sort(head);
System.out.println(head);
}
static Node getMiddle(Node node) {
Node fastptr = node.link;
Node slowptr = node;
while (fastptr != null) {
fastptr = fastptr.link;
if (fastptr != null) {
slowptr = slowptr.link;
fastptr = fastptr.link;
}
}
return slowptr;
}
static Node merge(Node left, Node right) {
Node temp = new Node(-1, null);
Node curr = temp;
while (left != null && right != null) {
if (left.data < right.data) {
curr.link = left;
left = left.link;
}
else {
curr.link = right;
right = right.link;
}
curr = curr.link;
}
curr.link = left == null ? right : left;
return temp.link;
}
static Node sort(Node node) {
if (node == null || node.link == null) {
return node;
}
Node middle = getMiddle(node);
Node next = middle.link;
middle.link = null;
return merge(sort(node), sort(next));
}
}
class Node {
public int data;
public Node link;
public Node(int data, Node link) {
this.data = data;
this.link = link;
}
public String toString() {
return this.data + (this.link != null ? "->" + this.link : "");
}
}
Output:
Example 1 (correct):
200->25->473->23->390
23->25->200->390->473
Example 1 (incorrect):
200->25->473->23->390
200->390->473
Example 2 (correct):
900->25->473->23->390
23->25->390->473->900
Example 2 (incorrect):
900->25->473->23->390
900
Example 3 (accidentally works, because the old head is still the new head):
1->25->473->23->390
1->23->25->390->473
Try it!
1
Thanks a lot that worked
– chinloyal
Nov 21 at 9:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404719%2fjava-merge-sort-on-linked-list-sorting-only-when-smallest-number-is-added-first%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your sort routine looks great. The bug appears to be the way you are returning from your sort routine. If you call your sort as if it were an in-place sort, i.e.
sort(head);
System.out.println(head);
the old head is not updated to the new head returned by sort()
. Notice how the nodes that are being displayed in your test cases always begin with whatever the old head was. This appears to work if the old head in the unsorted list happens to be the new head in the sorted list, as in your last example. If, on the other hand, the old head node is moved during the sort, you'll only see the rest of the list from wherever the old head moved to onward. Nodes from the front of the list up to the old head will be garbage collected when they go out of scope.
The fix is to set the head of your list in the caller to the returned head from sort()
, which is the new head of the list:
head = sort(head);
System.out.println(head);
Here's your code re-written which reproduces your errors to illustrate the problem:
class Main {
public static void main(String args) {
Node head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("Example 1 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 1 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 2 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 2 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(1, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 3 (accidentally works, because the old head is still the new head):");
System.out.println(head);
sort(head);
System.out.println(head);
}
static Node getMiddle(Node node) {
Node fastptr = node.link;
Node slowptr = node;
while (fastptr != null) {
fastptr = fastptr.link;
if (fastptr != null) {
slowptr = slowptr.link;
fastptr = fastptr.link;
}
}
return slowptr;
}
static Node merge(Node left, Node right) {
Node temp = new Node(-1, null);
Node curr = temp;
while (left != null && right != null) {
if (left.data < right.data) {
curr.link = left;
left = left.link;
}
else {
curr.link = right;
right = right.link;
}
curr = curr.link;
}
curr.link = left == null ? right : left;
return temp.link;
}
static Node sort(Node node) {
if (node == null || node.link == null) {
return node;
}
Node middle = getMiddle(node);
Node next = middle.link;
middle.link = null;
return merge(sort(node), sort(next));
}
}
class Node {
public int data;
public Node link;
public Node(int data, Node link) {
this.data = data;
this.link = link;
}
public String toString() {
return this.data + (this.link != null ? "->" + this.link : "");
}
}
Output:
Example 1 (correct):
200->25->473->23->390
23->25->200->390->473
Example 1 (incorrect):
200->25->473->23->390
200->390->473
Example 2 (correct):
900->25->473->23->390
23->25->390->473->900
Example 2 (incorrect):
900->25->473->23->390
900
Example 3 (accidentally works, because the old head is still the new head):
1->25->473->23->390
1->23->25->390->473
Try it!
1
Thanks a lot that worked
– chinloyal
Nov 21 at 9:15
add a comment |
Your sort routine looks great. The bug appears to be the way you are returning from your sort routine. If you call your sort as if it were an in-place sort, i.e.
sort(head);
System.out.println(head);
the old head is not updated to the new head returned by sort()
. Notice how the nodes that are being displayed in your test cases always begin with whatever the old head was. This appears to work if the old head in the unsorted list happens to be the new head in the sorted list, as in your last example. If, on the other hand, the old head node is moved during the sort, you'll only see the rest of the list from wherever the old head moved to onward. Nodes from the front of the list up to the old head will be garbage collected when they go out of scope.
The fix is to set the head of your list in the caller to the returned head from sort()
, which is the new head of the list:
head = sort(head);
System.out.println(head);
Here's your code re-written which reproduces your errors to illustrate the problem:
class Main {
public static void main(String args) {
Node head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("Example 1 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 1 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 2 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 2 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(1, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 3 (accidentally works, because the old head is still the new head):");
System.out.println(head);
sort(head);
System.out.println(head);
}
static Node getMiddle(Node node) {
Node fastptr = node.link;
Node slowptr = node;
while (fastptr != null) {
fastptr = fastptr.link;
if (fastptr != null) {
slowptr = slowptr.link;
fastptr = fastptr.link;
}
}
return slowptr;
}
static Node merge(Node left, Node right) {
Node temp = new Node(-1, null);
Node curr = temp;
while (left != null && right != null) {
if (left.data < right.data) {
curr.link = left;
left = left.link;
}
else {
curr.link = right;
right = right.link;
}
curr = curr.link;
}
curr.link = left == null ? right : left;
return temp.link;
}
static Node sort(Node node) {
if (node == null || node.link == null) {
return node;
}
Node middle = getMiddle(node);
Node next = middle.link;
middle.link = null;
return merge(sort(node), sort(next));
}
}
class Node {
public int data;
public Node link;
public Node(int data, Node link) {
this.data = data;
this.link = link;
}
public String toString() {
return this.data + (this.link != null ? "->" + this.link : "");
}
}
Output:
Example 1 (correct):
200->25->473->23->390
23->25->200->390->473
Example 1 (incorrect):
200->25->473->23->390
200->390->473
Example 2 (correct):
900->25->473->23->390
23->25->390->473->900
Example 2 (incorrect):
900->25->473->23->390
900
Example 3 (accidentally works, because the old head is still the new head):
1->25->473->23->390
1->23->25->390->473
Try it!
1
Thanks a lot that worked
– chinloyal
Nov 21 at 9:15
add a comment |
Your sort routine looks great. The bug appears to be the way you are returning from your sort routine. If you call your sort as if it were an in-place sort, i.e.
sort(head);
System.out.println(head);
the old head is not updated to the new head returned by sort()
. Notice how the nodes that are being displayed in your test cases always begin with whatever the old head was. This appears to work if the old head in the unsorted list happens to be the new head in the sorted list, as in your last example. If, on the other hand, the old head node is moved during the sort, you'll only see the rest of the list from wherever the old head moved to onward. Nodes from the front of the list up to the old head will be garbage collected when they go out of scope.
The fix is to set the head of your list in the caller to the returned head from sort()
, which is the new head of the list:
head = sort(head);
System.out.println(head);
Here's your code re-written which reproduces your errors to illustrate the problem:
class Main {
public static void main(String args) {
Node head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("Example 1 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 1 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 2 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 2 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(1, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 3 (accidentally works, because the old head is still the new head):");
System.out.println(head);
sort(head);
System.out.println(head);
}
static Node getMiddle(Node node) {
Node fastptr = node.link;
Node slowptr = node;
while (fastptr != null) {
fastptr = fastptr.link;
if (fastptr != null) {
slowptr = slowptr.link;
fastptr = fastptr.link;
}
}
return slowptr;
}
static Node merge(Node left, Node right) {
Node temp = new Node(-1, null);
Node curr = temp;
while (left != null && right != null) {
if (left.data < right.data) {
curr.link = left;
left = left.link;
}
else {
curr.link = right;
right = right.link;
}
curr = curr.link;
}
curr.link = left == null ? right : left;
return temp.link;
}
static Node sort(Node node) {
if (node == null || node.link == null) {
return node;
}
Node middle = getMiddle(node);
Node next = middle.link;
middle.link = null;
return merge(sort(node), sort(next));
}
}
class Node {
public int data;
public Node link;
public Node(int data, Node link) {
this.data = data;
this.link = link;
}
public String toString() {
return this.data + (this.link != null ? "->" + this.link : "");
}
}
Output:
Example 1 (correct):
200->25->473->23->390
23->25->200->390->473
Example 1 (incorrect):
200->25->473->23->390
200->390->473
Example 2 (correct):
900->25->473->23->390
23->25->390->473->900
Example 2 (incorrect):
900->25->473->23->390
900
Example 3 (accidentally works, because the old head is still the new head):
1->25->473->23->390
1->23->25->390->473
Try it!
Your sort routine looks great. The bug appears to be the way you are returning from your sort routine. If you call your sort as if it were an in-place sort, i.e.
sort(head);
System.out.println(head);
the old head is not updated to the new head returned by sort()
. Notice how the nodes that are being displayed in your test cases always begin with whatever the old head was. This appears to work if the old head in the unsorted list happens to be the new head in the sorted list, as in your last example. If, on the other hand, the old head node is moved during the sort, you'll only see the rest of the list from wherever the old head moved to onward. Nodes from the front of the list up to the old head will be garbage collected when they go out of scope.
The fix is to set the head of your list in the caller to the returned head from sort()
, which is the new head of the list:
head = sort(head);
System.out.println(head);
Here's your code re-written which reproduces your errors to illustrate the problem:
class Main {
public static void main(String args) {
Node head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("Example 1 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(200, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 1 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 2 (correct):");
System.out.println(head);
head = sort(head);
System.out.println(head);
head = new Node(900, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nExample 2 (incorrect):");
System.out.println(head);
sort(head);
System.out.println(head);
head = new Node(1, new Node(25, new Node(473, new Node(23, new Node(390, null)))));
System.out.println("nnExample 3 (accidentally works, because the old head is still the new head):");
System.out.println(head);
sort(head);
System.out.println(head);
}
static Node getMiddle(Node node) {
Node fastptr = node.link;
Node slowptr = node;
while (fastptr != null) {
fastptr = fastptr.link;
if (fastptr != null) {
slowptr = slowptr.link;
fastptr = fastptr.link;
}
}
return slowptr;
}
static Node merge(Node left, Node right) {
Node temp = new Node(-1, null);
Node curr = temp;
while (left != null && right != null) {
if (left.data < right.data) {
curr.link = left;
left = left.link;
}
else {
curr.link = right;
right = right.link;
}
curr = curr.link;
}
curr.link = left == null ? right : left;
return temp.link;
}
static Node sort(Node node) {
if (node == null || node.link == null) {
return node;
}
Node middle = getMiddle(node);
Node next = middle.link;
middle.link = null;
return merge(sort(node), sort(next));
}
}
class Node {
public int data;
public Node link;
public Node(int data, Node link) {
this.data = data;
this.link = link;
}
public String toString() {
return this.data + (this.link != null ? "->" + this.link : "");
}
}
Output:
Example 1 (correct):
200->25->473->23->390
23->25->200->390->473
Example 1 (incorrect):
200->25->473->23->390
200->390->473
Example 2 (correct):
900->25->473->23->390
23->25->390->473->900
Example 2 (incorrect):
900->25->473->23->390
900
Example 3 (accidentally works, because the old head is still the new head):
1->25->473->23->390
1->23->25->390->473
Try it!
edited Nov 21 at 6:49
answered Nov 21 at 6:39
ggorlen
6,3963825
6,3963825
1
Thanks a lot that worked
– chinloyal
Nov 21 at 9:15
add a comment |
1
Thanks a lot that worked
– chinloyal
Nov 21 at 9:15
1
1
Thanks a lot that worked
– chinloyal
Nov 21 at 9:15
Thanks a lot that worked
– chinloyal
Nov 21 at 9:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404719%2fjava-merge-sort-on-linked-list-sorting-only-when-smallest-number-is-added-first%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MPKuKct,xstPbLwDdFJ,rJ,MoaYTNnByYN3rMFY6mhDv0,1DAEL0k rZz,h4o6pJ
1
Please provide an Minimal, Complete, and Verifiable example.
– samabcde
Nov 21 at 4:31