Getting terminal columns and rows in x86_64 assembly
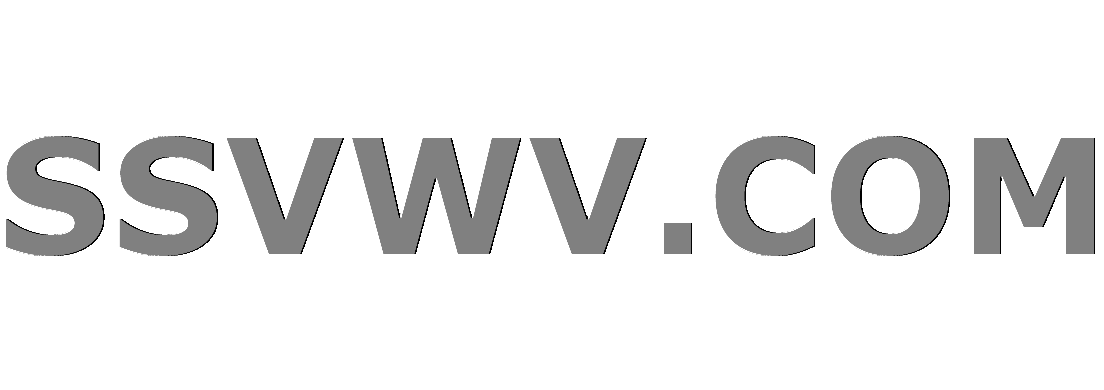
Multi tool use
As the title suggests, is there a way of getting the columns and rows, as a in x86_64 assembly?
EDIT:
I forgot to mention, I am very new to x86_64 assembly (I started last night).
linux assembly terminal x86-64
add a comment |
As the title suggests, is there a way of getting the columns and rows, as a in x86_64 assembly?
EDIT:
I forgot to mention, I am very new to x86_64 assembly (I started last night).
linux assembly terminal x86-64
1
There's nothing special about asm for this. If you're writing a program for Linux or other POSIX OS, you use the normalioctl(1, TIOCGWINSZ, ...)
system call you'd use from C, same asstrace stty -a
shows. Or check an environment variable, if you want to rely on that. If you're writing an EFI bootloader, you'd use an EFI function. If you're writing code for bare metal, it's up to you to set the video hardware to whatever mode you want.
– Peter Cordes
Nov 25 '18 at 5:05
So I should useioctl
, i'll look into it thanks.
– speedxerox
Nov 25 '18 at 5:21
add a comment |
As the title suggests, is there a way of getting the columns and rows, as a in x86_64 assembly?
EDIT:
I forgot to mention, I am very new to x86_64 assembly (I started last night).
linux assembly terminal x86-64
As the title suggests, is there a way of getting the columns and rows, as a in x86_64 assembly?
EDIT:
I forgot to mention, I am very new to x86_64 assembly (I started last night).
linux assembly terminal x86-64
linux assembly terminal x86-64
edited Nov 25 '18 at 5:32


Peter Cordes
128k18190326
128k18190326
asked Nov 25 '18 at 4:55
speedxeroxspeedxerox
184
184
1
There's nothing special about asm for this. If you're writing a program for Linux or other POSIX OS, you use the normalioctl(1, TIOCGWINSZ, ...)
system call you'd use from C, same asstrace stty -a
shows. Or check an environment variable, if you want to rely on that. If you're writing an EFI bootloader, you'd use an EFI function. If you're writing code for bare metal, it's up to you to set the video hardware to whatever mode you want.
– Peter Cordes
Nov 25 '18 at 5:05
So I should useioctl
, i'll look into it thanks.
– speedxerox
Nov 25 '18 at 5:21
add a comment |
1
There's nothing special about asm for this. If you're writing a program for Linux or other POSIX OS, you use the normalioctl(1, TIOCGWINSZ, ...)
system call you'd use from C, same asstrace stty -a
shows. Or check an environment variable, if you want to rely on that. If you're writing an EFI bootloader, you'd use an EFI function. If you're writing code for bare metal, it's up to you to set the video hardware to whatever mode you want.
– Peter Cordes
Nov 25 '18 at 5:05
So I should useioctl
, i'll look into it thanks.
– speedxerox
Nov 25 '18 at 5:21
1
1
There's nothing special about asm for this. If you're writing a program for Linux or other POSIX OS, you use the normal
ioctl(1, TIOCGWINSZ, ...)
system call you'd use from C, same as strace stty -a
shows. Or check an environment variable, if you want to rely on that. If you're writing an EFI bootloader, you'd use an EFI function. If you're writing code for bare metal, it's up to you to set the video hardware to whatever mode you want.– Peter Cordes
Nov 25 '18 at 5:05
There's nothing special about asm for this. If you're writing a program for Linux or other POSIX OS, you use the normal
ioctl(1, TIOCGWINSZ, ...)
system call you'd use from C, same as strace stty -a
shows. Or check an environment variable, if you want to rely on that. If you're writing an EFI bootloader, you'd use an EFI function. If you're writing code for bare metal, it's up to you to set the video hardware to whatever mode you want.– Peter Cordes
Nov 25 '18 at 5:05
So I should use
ioctl
, i'll look into it thanks.– speedxerox
Nov 25 '18 at 5:21
So I should use
ioctl
, i'll look into it thanks.– speedxerox
Nov 25 '18 at 5:21
add a comment |
1 Answer
1
active
oldest
votes
You can get the size of a terminal with the system call no. 16 (sys_ioctl
). Its parameters are identical to the C function ioctl
. The relevant command is TIOCGWINSZ
.
Let's get some informations with C:
// inspired by https://linux.die.net/man/4/tty_ioctl
#include <unistd.h>
#include <sys/ioctl.h>
#include <stdio.h>
int main (void)
{
struct winsize sz;
printf("STDOUT_FILENO = %un", STDOUT_FILENO);
printf("TIOCGWINSZ = 0x%Xn", TIOCGWINSZ);
printf("SIZEOF sz (bytes): %lun", sizeof sz);
ioctl(STDOUT_FILENO, TIOCGWINSZ, &sz);
printf("Screen width: %u Screen height: %un", sz.ws_col, sz.ws_row);
return 0;
}
Call sys_ioctl
with NASM:
; Name: get_terminal_size.asm
; Assemble: nasm -felf64 get_terminal_size.asm
; Link: ld -m elf_x86_64 -dynamic-linker /lib64/ld-linux-x86-64.so.2 -lc -o get_terminal_size get_terminal_size.o
; Run: ./get_terminal_size
section .data
fmt db `Screen width: %u Screen height: %un`,0 ; backticks for 'n'
section .bss
sz RESW 4
section .text
global _start
extern printf, exit
_start:
; http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
; https://linux.die.net/man/4/tty_ioctl
mov eax, 16 ; Kernel function SYS_IOCTL
mov edi, 1 ; STDOUT
mov esi, 0x5413 ; TIOCGWINSZ
mov edx, sz ; struct winsize sz
syscall ; Call Linux
mov rdi, fmt ; string pointer
movzx esi, WORD [sz+2] ; sz.ws_col
movzx edx, WORD [sz+0] ; sz.ws_row
xor eax, eax ; no vector registers used
call printf ; Call libc
xor edi, edi ; Exitcode 0 = exit(0)
call exit ; Call libc
AT&T style:
# Name: get_terminal_size.s
# Compile: gcc -m64 -oget_terminal_size get_terminal_size.s
# Run: ./get_terminal_size
.section .data
fmt: .asciz "Screen width: %u Screen height: %un"
.section .bss
.lcomm sz, 8
.section .text
.globl main
main:
# http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
# https://linux.die.net/man/4/tty_ioctl
mov $16, %eax # Kernel function SYS_IOCTL
mov $1, %edi # STDOUT
mov $0x5413, %esi # TIOCGWINSZ
mov $sz, %edx # struct winsize sz
syscall # Call Linux
mov $fmt, %rdi # string pointer
movswl (sz+2), %esi # sz.ws_col
movswl (sz+0), %edx # sz.ws_row
xor %eax, %eax # no vector registers used
call printf # Call libc
xor %edi, %edi # Exitcode 0 = exit(0)
call exit # Call libc
2
Note that this approach is not guaranteed to give results. It should be used in conjunction to a lookup in the termcap database.
– fuz
Nov 25 '18 at 10:55
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464762%2fgetting-terminal-columns-and-rows-in-x86-64-assembly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can get the size of a terminal with the system call no. 16 (sys_ioctl
). Its parameters are identical to the C function ioctl
. The relevant command is TIOCGWINSZ
.
Let's get some informations with C:
// inspired by https://linux.die.net/man/4/tty_ioctl
#include <unistd.h>
#include <sys/ioctl.h>
#include <stdio.h>
int main (void)
{
struct winsize sz;
printf("STDOUT_FILENO = %un", STDOUT_FILENO);
printf("TIOCGWINSZ = 0x%Xn", TIOCGWINSZ);
printf("SIZEOF sz (bytes): %lun", sizeof sz);
ioctl(STDOUT_FILENO, TIOCGWINSZ, &sz);
printf("Screen width: %u Screen height: %un", sz.ws_col, sz.ws_row);
return 0;
}
Call sys_ioctl
with NASM:
; Name: get_terminal_size.asm
; Assemble: nasm -felf64 get_terminal_size.asm
; Link: ld -m elf_x86_64 -dynamic-linker /lib64/ld-linux-x86-64.so.2 -lc -o get_terminal_size get_terminal_size.o
; Run: ./get_terminal_size
section .data
fmt db `Screen width: %u Screen height: %un`,0 ; backticks for 'n'
section .bss
sz RESW 4
section .text
global _start
extern printf, exit
_start:
; http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
; https://linux.die.net/man/4/tty_ioctl
mov eax, 16 ; Kernel function SYS_IOCTL
mov edi, 1 ; STDOUT
mov esi, 0x5413 ; TIOCGWINSZ
mov edx, sz ; struct winsize sz
syscall ; Call Linux
mov rdi, fmt ; string pointer
movzx esi, WORD [sz+2] ; sz.ws_col
movzx edx, WORD [sz+0] ; sz.ws_row
xor eax, eax ; no vector registers used
call printf ; Call libc
xor edi, edi ; Exitcode 0 = exit(0)
call exit ; Call libc
AT&T style:
# Name: get_terminal_size.s
# Compile: gcc -m64 -oget_terminal_size get_terminal_size.s
# Run: ./get_terminal_size
.section .data
fmt: .asciz "Screen width: %u Screen height: %un"
.section .bss
.lcomm sz, 8
.section .text
.globl main
main:
# http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
# https://linux.die.net/man/4/tty_ioctl
mov $16, %eax # Kernel function SYS_IOCTL
mov $1, %edi # STDOUT
mov $0x5413, %esi # TIOCGWINSZ
mov $sz, %edx # struct winsize sz
syscall # Call Linux
mov $fmt, %rdi # string pointer
movswl (sz+2), %esi # sz.ws_col
movswl (sz+0), %edx # sz.ws_row
xor %eax, %eax # no vector registers used
call printf # Call libc
xor %edi, %edi # Exitcode 0 = exit(0)
call exit # Call libc
2
Note that this approach is not guaranteed to give results. It should be used in conjunction to a lookup in the termcap database.
– fuz
Nov 25 '18 at 10:55
add a comment |
You can get the size of a terminal with the system call no. 16 (sys_ioctl
). Its parameters are identical to the C function ioctl
. The relevant command is TIOCGWINSZ
.
Let's get some informations with C:
// inspired by https://linux.die.net/man/4/tty_ioctl
#include <unistd.h>
#include <sys/ioctl.h>
#include <stdio.h>
int main (void)
{
struct winsize sz;
printf("STDOUT_FILENO = %un", STDOUT_FILENO);
printf("TIOCGWINSZ = 0x%Xn", TIOCGWINSZ);
printf("SIZEOF sz (bytes): %lun", sizeof sz);
ioctl(STDOUT_FILENO, TIOCGWINSZ, &sz);
printf("Screen width: %u Screen height: %un", sz.ws_col, sz.ws_row);
return 0;
}
Call sys_ioctl
with NASM:
; Name: get_terminal_size.asm
; Assemble: nasm -felf64 get_terminal_size.asm
; Link: ld -m elf_x86_64 -dynamic-linker /lib64/ld-linux-x86-64.so.2 -lc -o get_terminal_size get_terminal_size.o
; Run: ./get_terminal_size
section .data
fmt db `Screen width: %u Screen height: %un`,0 ; backticks for 'n'
section .bss
sz RESW 4
section .text
global _start
extern printf, exit
_start:
; http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
; https://linux.die.net/man/4/tty_ioctl
mov eax, 16 ; Kernel function SYS_IOCTL
mov edi, 1 ; STDOUT
mov esi, 0x5413 ; TIOCGWINSZ
mov edx, sz ; struct winsize sz
syscall ; Call Linux
mov rdi, fmt ; string pointer
movzx esi, WORD [sz+2] ; sz.ws_col
movzx edx, WORD [sz+0] ; sz.ws_row
xor eax, eax ; no vector registers used
call printf ; Call libc
xor edi, edi ; Exitcode 0 = exit(0)
call exit ; Call libc
AT&T style:
# Name: get_terminal_size.s
# Compile: gcc -m64 -oget_terminal_size get_terminal_size.s
# Run: ./get_terminal_size
.section .data
fmt: .asciz "Screen width: %u Screen height: %un"
.section .bss
.lcomm sz, 8
.section .text
.globl main
main:
# http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
# https://linux.die.net/man/4/tty_ioctl
mov $16, %eax # Kernel function SYS_IOCTL
mov $1, %edi # STDOUT
mov $0x5413, %esi # TIOCGWINSZ
mov $sz, %edx # struct winsize sz
syscall # Call Linux
mov $fmt, %rdi # string pointer
movswl (sz+2), %esi # sz.ws_col
movswl (sz+0), %edx # sz.ws_row
xor %eax, %eax # no vector registers used
call printf # Call libc
xor %edi, %edi # Exitcode 0 = exit(0)
call exit # Call libc
2
Note that this approach is not guaranteed to give results. It should be used in conjunction to a lookup in the termcap database.
– fuz
Nov 25 '18 at 10:55
add a comment |
You can get the size of a terminal with the system call no. 16 (sys_ioctl
). Its parameters are identical to the C function ioctl
. The relevant command is TIOCGWINSZ
.
Let's get some informations with C:
// inspired by https://linux.die.net/man/4/tty_ioctl
#include <unistd.h>
#include <sys/ioctl.h>
#include <stdio.h>
int main (void)
{
struct winsize sz;
printf("STDOUT_FILENO = %un", STDOUT_FILENO);
printf("TIOCGWINSZ = 0x%Xn", TIOCGWINSZ);
printf("SIZEOF sz (bytes): %lun", sizeof sz);
ioctl(STDOUT_FILENO, TIOCGWINSZ, &sz);
printf("Screen width: %u Screen height: %un", sz.ws_col, sz.ws_row);
return 0;
}
Call sys_ioctl
with NASM:
; Name: get_terminal_size.asm
; Assemble: nasm -felf64 get_terminal_size.asm
; Link: ld -m elf_x86_64 -dynamic-linker /lib64/ld-linux-x86-64.so.2 -lc -o get_terminal_size get_terminal_size.o
; Run: ./get_terminal_size
section .data
fmt db `Screen width: %u Screen height: %un`,0 ; backticks for 'n'
section .bss
sz RESW 4
section .text
global _start
extern printf, exit
_start:
; http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
; https://linux.die.net/man/4/tty_ioctl
mov eax, 16 ; Kernel function SYS_IOCTL
mov edi, 1 ; STDOUT
mov esi, 0x5413 ; TIOCGWINSZ
mov edx, sz ; struct winsize sz
syscall ; Call Linux
mov rdi, fmt ; string pointer
movzx esi, WORD [sz+2] ; sz.ws_col
movzx edx, WORD [sz+0] ; sz.ws_row
xor eax, eax ; no vector registers used
call printf ; Call libc
xor edi, edi ; Exitcode 0 = exit(0)
call exit ; Call libc
AT&T style:
# Name: get_terminal_size.s
# Compile: gcc -m64 -oget_terminal_size get_terminal_size.s
# Run: ./get_terminal_size
.section .data
fmt: .asciz "Screen width: %u Screen height: %un"
.section .bss
.lcomm sz, 8
.section .text
.globl main
main:
# http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
# https://linux.die.net/man/4/tty_ioctl
mov $16, %eax # Kernel function SYS_IOCTL
mov $1, %edi # STDOUT
mov $0x5413, %esi # TIOCGWINSZ
mov $sz, %edx # struct winsize sz
syscall # Call Linux
mov $fmt, %rdi # string pointer
movswl (sz+2), %esi # sz.ws_col
movswl (sz+0), %edx # sz.ws_row
xor %eax, %eax # no vector registers used
call printf # Call libc
xor %edi, %edi # Exitcode 0 = exit(0)
call exit # Call libc
You can get the size of a terminal with the system call no. 16 (sys_ioctl
). Its parameters are identical to the C function ioctl
. The relevant command is TIOCGWINSZ
.
Let's get some informations with C:
// inspired by https://linux.die.net/man/4/tty_ioctl
#include <unistd.h>
#include <sys/ioctl.h>
#include <stdio.h>
int main (void)
{
struct winsize sz;
printf("STDOUT_FILENO = %un", STDOUT_FILENO);
printf("TIOCGWINSZ = 0x%Xn", TIOCGWINSZ);
printf("SIZEOF sz (bytes): %lun", sizeof sz);
ioctl(STDOUT_FILENO, TIOCGWINSZ, &sz);
printf("Screen width: %u Screen height: %un", sz.ws_col, sz.ws_row);
return 0;
}
Call sys_ioctl
with NASM:
; Name: get_terminal_size.asm
; Assemble: nasm -felf64 get_terminal_size.asm
; Link: ld -m elf_x86_64 -dynamic-linker /lib64/ld-linux-x86-64.so.2 -lc -o get_terminal_size get_terminal_size.o
; Run: ./get_terminal_size
section .data
fmt db `Screen width: %u Screen height: %un`,0 ; backticks for 'n'
section .bss
sz RESW 4
section .text
global _start
extern printf, exit
_start:
; http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
; https://linux.die.net/man/4/tty_ioctl
mov eax, 16 ; Kernel function SYS_IOCTL
mov edi, 1 ; STDOUT
mov esi, 0x5413 ; TIOCGWINSZ
mov edx, sz ; struct winsize sz
syscall ; Call Linux
mov rdi, fmt ; string pointer
movzx esi, WORD [sz+2] ; sz.ws_col
movzx edx, WORD [sz+0] ; sz.ws_row
xor eax, eax ; no vector registers used
call printf ; Call libc
xor edi, edi ; Exitcode 0 = exit(0)
call exit ; Call libc
AT&T style:
# Name: get_terminal_size.s
# Compile: gcc -m64 -oget_terminal_size get_terminal_size.s
# Run: ./get_terminal_size
.section .data
fmt: .asciz "Screen width: %u Screen height: %un"
.section .bss
.lcomm sz, 8
.section .text
.globl main
main:
# http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/
# https://linux.die.net/man/4/tty_ioctl
mov $16, %eax # Kernel function SYS_IOCTL
mov $1, %edi # STDOUT
mov $0x5413, %esi # TIOCGWINSZ
mov $sz, %edx # struct winsize sz
syscall # Call Linux
mov $fmt, %rdi # string pointer
movswl (sz+2), %esi # sz.ws_col
movswl (sz+0), %edx # sz.ws_row
xor %eax, %eax # no vector registers used
call printf # Call libc
xor %edi, %edi # Exitcode 0 = exit(0)
call exit # Call libc
edited Nov 28 '18 at 6:23
answered Nov 25 '18 at 10:52


rkhbrkhb
11.4k72142
11.4k72142
2
Note that this approach is not guaranteed to give results. It should be used in conjunction to a lookup in the termcap database.
– fuz
Nov 25 '18 at 10:55
add a comment |
2
Note that this approach is not guaranteed to give results. It should be used in conjunction to a lookup in the termcap database.
– fuz
Nov 25 '18 at 10:55
2
2
Note that this approach is not guaranteed to give results. It should be used in conjunction to a lookup in the termcap database.
– fuz
Nov 25 '18 at 10:55
Note that this approach is not guaranteed to give results. It should be used in conjunction to a lookup in the termcap database.
– fuz
Nov 25 '18 at 10:55
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464762%2fgetting-terminal-columns-and-rows-in-x86-64-assembly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BKNFQZwAy2ESf uKIW7n5rdUM6roclX
1
There's nothing special about asm for this. If you're writing a program for Linux or other POSIX OS, you use the normal
ioctl(1, TIOCGWINSZ, ...)
system call you'd use from C, same asstrace stty -a
shows. Or check an environment variable, if you want to rely on that. If you're writing an EFI bootloader, you'd use an EFI function. If you're writing code for bare metal, it's up to you to set the video hardware to whatever mode you want.– Peter Cordes
Nov 25 '18 at 5:05
So I should use
ioctl
, i'll look into it thanks.– speedxerox
Nov 25 '18 at 5:21