Filter v-for on results of mysql join query
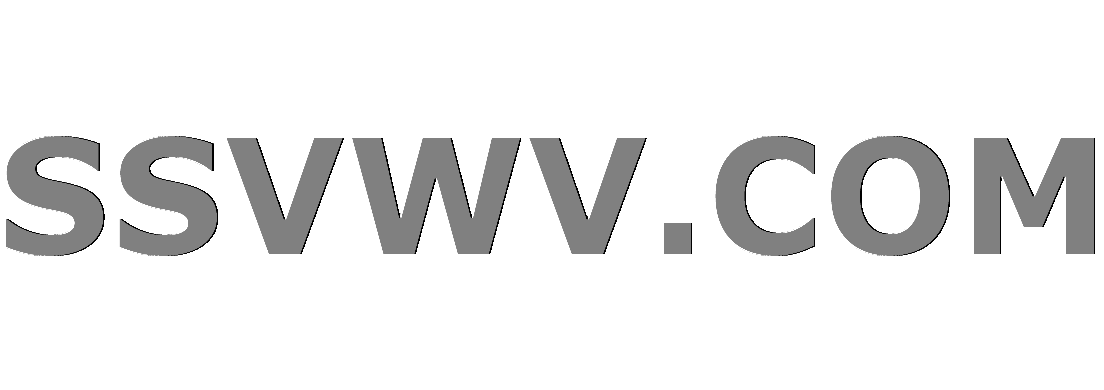
Multi tool use
I'm building a project in Nuxt.js that uses an express API using a mysql database. I have a blog in the project and am setting up comments for each blog post that can have replies to each comment. Each comment can have many replies.
I have set up two database tables for these, 'comments' and 'replys' where 'replys' has a comment_id foreign key relationship with 'comments' id. I query the database using a join like so:
SELECT * FROM comments LEFT JOIN replys ON comments.id = replys.comment_id;
which returns a response like this:
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
| id | post_id | user_id | content | created_at | id | comment_id | reply_user_id | reply_content | reply_created_at |
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
| 1 | 1 | 1 | Well thats a very lovely post | 2018-11-24 19:29:05 | 1 | 1 | 2 | it is indeed | 2018-11-25 15:11:20 |
| 1 | 1 | 1 | Well thats a very lovely post | 2018-11-24 19:29:05 | 2 | 1 | 1 | why thanks | 2018-11-25 15:11:39 |
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
So it is getting all the data I need and I just need to use it now. What I want to do is use a v-for to iterate through data but without the duplicate 'content', so something like:
<div v-for="comment in comments" :key="comment.reply_content">
<p>{{comment.content}}</p>
<p>{{comment.reply_content}}</p>
</div>
but of course this displays the comment.content for each of the replies it has. So I want to limit it to unique comment.content while still showing all the replies. I've tried looking at javascript functions like .map() and .join() but haven't found a way.
After lots of head scratching I'm currently making two queries to get what I need but think there must be a way to use the query I have to do what I need.
javascript mysql express vue.js nuxt.js
add a comment |
I'm building a project in Nuxt.js that uses an express API using a mysql database. I have a blog in the project and am setting up comments for each blog post that can have replies to each comment. Each comment can have many replies.
I have set up two database tables for these, 'comments' and 'replys' where 'replys' has a comment_id foreign key relationship with 'comments' id. I query the database using a join like so:
SELECT * FROM comments LEFT JOIN replys ON comments.id = replys.comment_id;
which returns a response like this:
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
| id | post_id | user_id | content | created_at | id | comment_id | reply_user_id | reply_content | reply_created_at |
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
| 1 | 1 | 1 | Well thats a very lovely post | 2018-11-24 19:29:05 | 1 | 1 | 2 | it is indeed | 2018-11-25 15:11:20 |
| 1 | 1 | 1 | Well thats a very lovely post | 2018-11-24 19:29:05 | 2 | 1 | 1 | why thanks | 2018-11-25 15:11:39 |
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
So it is getting all the data I need and I just need to use it now. What I want to do is use a v-for to iterate through data but without the duplicate 'content', so something like:
<div v-for="comment in comments" :key="comment.reply_content">
<p>{{comment.content}}</p>
<p>{{comment.reply_content}}</p>
</div>
but of course this displays the comment.content for each of the replies it has. So I want to limit it to unique comment.content while still showing all the replies. I've tried looking at javascript functions like .map() and .join() but haven't found a way.
After lots of head scratching I'm currently making two queries to get what I need but think there must be a way to use the query I have to do what I need.
javascript mysql express vue.js nuxt.js
add a comment |
I'm building a project in Nuxt.js that uses an express API using a mysql database. I have a blog in the project and am setting up comments for each blog post that can have replies to each comment. Each comment can have many replies.
I have set up two database tables for these, 'comments' and 'replys' where 'replys' has a comment_id foreign key relationship with 'comments' id. I query the database using a join like so:
SELECT * FROM comments LEFT JOIN replys ON comments.id = replys.comment_id;
which returns a response like this:
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
| id | post_id | user_id | content | created_at | id | comment_id | reply_user_id | reply_content | reply_created_at |
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
| 1 | 1 | 1 | Well thats a very lovely post | 2018-11-24 19:29:05 | 1 | 1 | 2 | it is indeed | 2018-11-25 15:11:20 |
| 1 | 1 | 1 | Well thats a very lovely post | 2018-11-24 19:29:05 | 2 | 1 | 1 | why thanks | 2018-11-25 15:11:39 |
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
So it is getting all the data I need and I just need to use it now. What I want to do is use a v-for to iterate through data but without the duplicate 'content', so something like:
<div v-for="comment in comments" :key="comment.reply_content">
<p>{{comment.content}}</p>
<p>{{comment.reply_content}}</p>
</div>
but of course this displays the comment.content for each of the replies it has. So I want to limit it to unique comment.content while still showing all the replies. I've tried looking at javascript functions like .map() and .join() but haven't found a way.
After lots of head scratching I'm currently making two queries to get what I need but think there must be a way to use the query I have to do what I need.
javascript mysql express vue.js nuxt.js
I'm building a project in Nuxt.js that uses an express API using a mysql database. I have a blog in the project and am setting up comments for each blog post that can have replies to each comment. Each comment can have many replies.
I have set up two database tables for these, 'comments' and 'replys' where 'replys' has a comment_id foreign key relationship with 'comments' id. I query the database using a join like so:
SELECT * FROM comments LEFT JOIN replys ON comments.id = replys.comment_id;
which returns a response like this:
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
| id | post_id | user_id | content | created_at | id | comment_id | reply_user_id | reply_content | reply_created_at |
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
| 1 | 1 | 1 | Well thats a very lovely post | 2018-11-24 19:29:05 | 1 | 1 | 2 | it is indeed | 2018-11-25 15:11:20 |
| 1 | 1 | 1 | Well thats a very lovely post | 2018-11-24 19:29:05 | 2 | 1 | 1 | why thanks | 2018-11-25 15:11:39 |
+----+---------+---------+-------------------------------+---------------------+----+------------+---------------+---------------+---------------------+
So it is getting all the data I need and I just need to use it now. What I want to do is use a v-for to iterate through data but without the duplicate 'content', so something like:
<div v-for="comment in comments" :key="comment.reply_content">
<p>{{comment.content}}</p>
<p>{{comment.reply_content}}</p>
</div>
but of course this displays the comment.content for each of the replies it has. So I want to limit it to unique comment.content while still showing all the replies. I've tried looking at javascript functions like .map() and .join() but haven't found a way.
After lots of head scratching I'm currently making two queries to get what I need but think there must be a way to use the query I have to do what I need.
javascript mysql express vue.js nuxt.js
javascript mysql express vue.js nuxt.js
asked Nov 25 '18 at 4:43


Andrew1325Andrew1325
39329
39329
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Perhaps you could use a computed property with the array method reduce
to sort your comments ..
computed: {
sortedComments() {
return this.comments.reduce((cum, next) => {
const lastCommentArray = cum[cum.length - 1]
if (cum.length == 0 ||
next.content != lastCommentArray[lastCommentArray.length - 1].content) {
cum.push()
}
cum[cum.length - 1].push(next)
return cum
}, )
}
}
Then you could iterate over it like this ..
<div v-for="commentArray in sortedComments" :key="commentArray[0].content">
<p>{{commentArray[0].content}}</p>
<p v-for="reply in commentArray" :key="reply.reply_content">{{reply.reply_content}}</p>
</div>
Yep, that works a treat. Thanks very much.
– Andrew1325
Nov 26 '18 at 8:56
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464716%2ffilter-v-for-on-results-of-mysql-join-query%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Perhaps you could use a computed property with the array method reduce
to sort your comments ..
computed: {
sortedComments() {
return this.comments.reduce((cum, next) => {
const lastCommentArray = cum[cum.length - 1]
if (cum.length == 0 ||
next.content != lastCommentArray[lastCommentArray.length - 1].content) {
cum.push()
}
cum[cum.length - 1].push(next)
return cum
}, )
}
}
Then you could iterate over it like this ..
<div v-for="commentArray in sortedComments" :key="commentArray[0].content">
<p>{{commentArray[0].content}}</p>
<p v-for="reply in commentArray" :key="reply.reply_content">{{reply.reply_content}}</p>
</div>
Yep, that works a treat. Thanks very much.
– Andrew1325
Nov 26 '18 at 8:56
add a comment |
Perhaps you could use a computed property with the array method reduce
to sort your comments ..
computed: {
sortedComments() {
return this.comments.reduce((cum, next) => {
const lastCommentArray = cum[cum.length - 1]
if (cum.length == 0 ||
next.content != lastCommentArray[lastCommentArray.length - 1].content) {
cum.push()
}
cum[cum.length - 1].push(next)
return cum
}, )
}
}
Then you could iterate over it like this ..
<div v-for="commentArray in sortedComments" :key="commentArray[0].content">
<p>{{commentArray[0].content}}</p>
<p v-for="reply in commentArray" :key="reply.reply_content">{{reply.reply_content}}</p>
</div>
Yep, that works a treat. Thanks very much.
– Andrew1325
Nov 26 '18 at 8:56
add a comment |
Perhaps you could use a computed property with the array method reduce
to sort your comments ..
computed: {
sortedComments() {
return this.comments.reduce((cum, next) => {
const lastCommentArray = cum[cum.length - 1]
if (cum.length == 0 ||
next.content != lastCommentArray[lastCommentArray.length - 1].content) {
cum.push()
}
cum[cum.length - 1].push(next)
return cum
}, )
}
}
Then you could iterate over it like this ..
<div v-for="commentArray in sortedComments" :key="commentArray[0].content">
<p>{{commentArray[0].content}}</p>
<p v-for="reply in commentArray" :key="reply.reply_content">{{reply.reply_content}}</p>
</div>
Perhaps you could use a computed property with the array method reduce
to sort your comments ..
computed: {
sortedComments() {
return this.comments.reduce((cum, next) => {
const lastCommentArray = cum[cum.length - 1]
if (cum.length == 0 ||
next.content != lastCommentArray[lastCommentArray.length - 1].content) {
cum.push()
}
cum[cum.length - 1].push(next)
return cum
}, )
}
}
Then you could iterate over it like this ..
<div v-for="commentArray in sortedComments" :key="commentArray[0].content">
<p>{{commentArray[0].content}}</p>
<p v-for="reply in commentArray" :key="reply.reply_content">{{reply.reply_content}}</p>
</div>
edited Nov 25 '18 at 10:28
answered Nov 25 '18 at 10:21


Husam IbrahimHusam Ibrahim
3,198416
3,198416
Yep, that works a treat. Thanks very much.
– Andrew1325
Nov 26 '18 at 8:56
add a comment |
Yep, that works a treat. Thanks very much.
– Andrew1325
Nov 26 '18 at 8:56
Yep, that works a treat. Thanks very much.
– Andrew1325
Nov 26 '18 at 8:56
Yep, that works a treat. Thanks very much.
– Andrew1325
Nov 26 '18 at 8:56
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464716%2ffilter-v-for-on-results-of-mysql-join-query%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tAuALXBYIIYlRSgs9kb UMEHox42,TD03fWM